4.13 |
Drivers are concerned with the mileage obtained by their automobiles. One driver has kept track of several tankfuls of gasoline by recording miles driven and gallons used for each tankful. Develop a C++ program that uses a while statement to input the miles driven and gallons used for each tankful. The program should calculate and display the miles per gallon obtained for each tankful and print the combined miles per gallon obtained for all tankfuls up to this point.
Enter the miles used (-1 to quit): 287
Enter gallons: 13
MPG this tankful: 22.076923
Total MPG: 22.076923
Enter the miles used (-1 to quit): 200
Enter gallons: 10
MPG this tankful: 20.000000
Total MPG: 21.173913
Enter the miles used (-1 to quit): 120
Enter gallons: 5
MPG this tankful: 24.000000
Total MPG: 21.678571
Enter miles (-1 to quit): -1
|
|
4.14 |
Develop a C++ program that will determine whether a department-store customer has exceeded the credit limit on a charge account. For each customer, the following facts are available:
- Account number (an integer)
- Balance at the beginning of the month
- Total of all items charged by this customer this month
- Total of all credits applied to this customer's account this month
- Allowed credit limit
The program should use a while statement to input each of these facts, calculate the new balance (= beginning balance + charges credits) and determine whether the new balance exceeds the customer's credit limit. For those customers whose credit limit is exceeded, the program should display the customer's account number, credit limit, new balance and the message "Credit Limit Exceeded."
Enter account number (-1 to end): 100
Enter beginning balance: 5394.78
Enter total charges: 1000.00
Enter total credits: 500.00
Enter credit limit: 5500.00
New balance is 5894.78
Account: 100
Credit limit: 5500.00
Balance: 5894.78
Credit Limit Exceeded.
Enter Account Number (or -1 to quit): 200
Enter beginning balance: 1000.00
Enter total charges: 123.45
Enter total credits: 321.00
Enter credit limit: 1500.00
New balance is 802.45
Enter Account Number (or -1 to quit): 300
Enter beginning balance: 500.00
Enter total charges: 274.73
Enter total credits: 100.00
Enter credit limit: 800.00
New balance is 674.73
Enter Account Number (or -1 to quit): -1
|
|
4.15 |
One large chemical company pays its salespeople on a commission basis. The salespeople each receive $200 per week plus 9 percent of their gross sales for that week. For example, a salesperson who sells $5000 worth of chemicals in a week receives $200 plus 9 percent of $5000, or a total of $650. Develop a C++ program that uses a while statement to input each salesperson's gross sales for last week and calculates and displays that salesperson's earnings. Process one salesperson's figures at a time.
Enter sales in dollars (-1 to end): 5000.00
Salary is: $650.00
Enter sales in dollars (-1 to end): 6000.00
Salary is: $740.00
Enter sales in dollars (-1 to end): 7000.00
Salary is: $830.00
Enter sales in dollars (-1 to end): -1
|
|
|
|
4.16 |
Develop a C++ program that uses a while statement to determine the gross pay for each of several employees. The company pays "straight time" for the first 40 hours worked by each employee and pays "time-and-a-half" for all hours worked in excess of 40 hours. You are given a list of the employees of the company, the number of hours each employee worked last week and the hourly rate of each employee. Your program should input this information for each employee and should determine and display the employee's gross pay.
Enter hours worked (-1 to end): 39
Enter hourly rate of the worker ($00.00): 10.00
Salary is $390.00
Enter hours worked (-1 to end): 40
Enter hourly rate of the worker ($00.00): 10.00
Salary is $400.00
Enter hours worked (-1 to end): 41
Enter hourly rate of the worker ($00.00): 10.00
Salary is $415.00
Enter hours worked (-1 to end): -1
|
|
4.17 |
The process of finding the largest number (i.e., the maximum of a group of numbers) is used frequently in computer applications. For example, a program that determines the winner of a sales contest inputs the number of units sold by each salesperson. The salesperson who sells the most units wins the contest. Write a pseudocode program, then a C++ program that uses a while statement to determine and print the largest number of 10 numbers input by the user. Your program should use three variables, as follows:
counter:
|
A counter to count to 10 (i.e., to keep track of how many numbers have been input and to determine when all 10 numbers have been processed).
|
number:
|
The current number input to the program.
|
largest:
|
The largest number found so far.
|
|
4.18 |
Write a C++ program that uses a while statement and the tab escape sequence t to print the following table of values:
N 10*N 100*N 1000*N
1 10 100 1000
2 20 200 2000
3 30 300 3000
4 40 400 4000
5 50 500 5000
|
|
4.19 |
Using an approach similar to that in Exercise 4.17, find the two largest values among the 10 numbers. [Note: You must input each number only once.]
|
4.20 |
The examination-results program of Fig. 4.16Fig. 4.18 assumes that any value input by the user that is not a 1 must be a 2. Modify the application to validate its inputs. On any input, if the value entered is other than 1 or 2, keep looping until the user enters a correct value.
|
|
|
4.21 |
What does the following program print?
1 // Exercise 4.21: ex04_21.cpp
2 // What does this program print?
3 #include
4 using std::cout;
5 using std::endl;
6
7 int main()
8 {
9 int count = 1; // initialize count
10
11 while ( count <= 10 ) // loop 10 times
12 {
13 // output line of text
14 cout << ( count % 2 ? "****" : "++++++++" ) << endl;
15 count++; // increment count
16 } // end while
17
18 return 0; // indicate successful termination
19 } // end main
|
4.22 |
What does the following program print?
1 // Exercise 4.22: ex04_22.cpp
2 // What does this program print?
3 #include
4 using std::cout;
5 using std::endl;
6
7 int main()
8 {
9 int row = 10; // initialize row
10 int column; // declare column
11
12 while ( row >= 1 ) // loop until row < 1
13 {
14 column = 1; // set column to 1 as iteration begins
15
16 while ( column <= 10 ) // loop 10 times
17 {
18 cout << ( row % 2 ? "<" : ">" ); // output
19 column++; // increment column
20 } // end inner while
21
22 row--; // decrement row
23 cout << endl; // begin new output line
24 } // end outer while
25
26 return 0; // indicate successful termination
27 } // end main
|
|
|
4.23 |
(Dangling-Else Problem) State the output for each of the following when x is 9 and y is 11 and when x is 11 and y is 9. Note that the compiler ignores the indentation in a C++ program. The C++ compiler always associates an else with the previous if unless told to do otherwise by the placement of braces {}. On first glance, the programmer may not be sure which if and else match, so this is referred to as the "dangling-else" problem. We eliminated the indentation from the following code to make the problem more challenging. [Hint: Apply indentation conventions you have learned.]
-
if ( x < 10 )
if ( y > 10 )
cout << "*****" << endl;
else
cout << "#####" << endl;
cout << "$$$$$" << endl;
-
if ( x < 10 )
{
if ( y > 10 )
cout << "*****" << endl;
}
else
{
cout << "#####" << endl;
cout << "$$$$$" << endl;
}
|
4.24 |
(Another Dangling-Else Problem) Modify the following code to produce the output shown. Use proper indentation techniques. You must not make any changes other than inserting braces. The compiler ignores indentation in a C++ program. We eliminated the indentation from the following code to make the problem more challenging. [Note: It is possible that no modification is necessary.]
if ( y == 8 )
if ( x == 5 )
cout << "@@@@@" << endl;
else
cout << "#####" << endl;
cout << "$$$$$" << endl;
cout << "&&&&&" << endl;
- Assuming x = 5 and y = 8, the following output is produced.
- Assuming x = 5 and y = 8, the following output is produced.
- Assuming x = 5 and y = 8, the following output is produced.
- Assuming x = 5 and y = 7, the following output is produced. [Note: The last three output statements after the else are all part of a block.]
|
4.25 |
Write a program that reads in the size of the side of a square and then prints a hollow square of that size out of asterisks and blanks. Your program should work for squares of all side sizes between 1 and 20. For example, if your program reads a size of 5, it should print
|
4.26 |
A palindrome is a number or a text phrase that reads the same backwards as forwards. For example, each of the following five-digit integers is a palindrome: 12321, 55555, 45554 and 11611. Write a program that reads in a five-digit integer and determines whether it is a palindrome. [Hint: Use the division and modulus operators to separate the number into its individual digits.]
|
4.27 |
Input an integer containing only 0s and 1s (i.e., a "binary" integer) and print its decimal equivalent. Use the modulus and division operators to pick off the "binary" number's digits one at a time from right to left. Much as in the decimal number system, where the rightmost digit has a positional value of 1, the next digit left has a positional value of 10, then 100, then 1000, and so on, in the binary number system the rightmost digit has a positional value of 1, the next digit left has a positional value of 2, then 4, then 8, and so on. Thus the decimal number 234 can be interpreted as 2 * 100 + 3 * 10 + 4 * 1. The decimal equivalent of binary 1101 is 1 * 1 + 0 * 2 + 1 * 4 + 1 * 8 or 1 + 0 + 4 + 8, or 13. [Note: The reader not familiar with binary numbers might wish to refer to Appendix D.]
|
4.28 |
Write a program that displays the checkerboard pattern shown below. Your program must use only three output statements, one of each of the following forms:
cout << "* ";
cout << ' ';
cout << endl;
* * * * * * * *
* * * * * * * *
* * * * * * * *
* * * * * * * *
* * * * * * * *
* * * * * * * *
* * * * * * * *
* * * * * * * *
|
|
4.29 |
Write a program that prints the powers of the integer 2, namely 2, 4, 8, 16, 32, 64, etc. Your while loop should not terminate (i.e., you should create an infinite loop). To do this, simply use the keyword true as the expression for the while statement. What happens when you run this program?
|
4.30 |
Write a program that reads the radius of a circle (as a double value) and computes and prints the diameter, the circumference and the area. Use the value 3.14159 for p.
|
4.31 |
What is wrong with the following statement? Provide the correct statement to accomplish what the programmer was probably trying to do.
cout << ++( x + y );
|
4.32 |
Write a program that reads three nonzero double values and determines and prints whether they could represent the sides of a triangle.
|
4.33 |
Write a program that reads three nonzero integers and determines and prints whether they could be the sides of a right triangle.
|
4.34 |
(Cryptography) A company wants to transmit data over the telephone, but is concerned that its phones could be tapped. All of the data are transmitted as four-digit integers. The company has asked you to write a program that encrypts the data so that it can be transmitted more securely. Your program should read a four-digit integer and encrypt it as follows: Replace each digit by (the sum of that digit plus 7) modulus 10. Then, swap the first digit with the third, swap the second digit with the fourth and print the encrypted integer. Write a separate program that inputs an encrypted fourdigit integer and decrypts it to form the original number.
|
4.35 |
The factorial of a nonnegative integer n is written n! (pronounced "n factorial") and is defined as follows:
n! = n · (n 1) · (n 2) · ... · 1 (for values of n greater than to 1)
and
n! = 1 (for n = 0 or n = 1).
For example, 5! = 5 · 4 · 3 · 2 · 1, which is 120. Use while statements in each of the following:
- Write a program that reads a nonnegative integer and computes and prints its factorial.
- Write a program that estimates the value of the mathematical constant e by using the formula:

Prompt the user for the desired accuracy of e (i.e., the number of terms in the summation).
- Write a program that computes the value of ex by using the formula
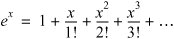
Prompt the user for the desired accuracy of e (i.e., the number of terms in the summation).
|
4.36 |
[Note: This exercise corresponds to Section 4.13, a portion of our software engineering case study.] Describe in 200 words or fewer what an automobile is and does. List the nouns and verbs separately. In the text, we stated that each noun might correspond to an object that will need to be built to implement a system, in this case a car. Pick five of the objects you listed, and, for each, list several attributes and several behaviors. Describe briefly how these objects interact with one another and other objects in your description. You have just performed several of the key steps in a typical object-oriented design.
|