Exercises
7.6 |
Fill in the blanks in each of the following:
- The names of the four elements of array p (int p[ 4 ];) are _________, _________, _________ and __________.
- Naming an array, stating its type and specifying the number of elements in the array is called ____________ the array.
- By convention, the first subscript in a two-dimensional array identifies an element's ____________ and the second subscript identifies an element's ____________.
- An m-by-n array contains ___________ rows, ________ columns and __________elements.
- The name of the element in row 3 and column 5 of array d is ________.
|
7.7 |
Determine whether each of the following is true or false. If false, explain why.
- To refer to a particular location or element within an array, we specify the name of the array and the value of the particular element.
- An array declaration reserves space for the array.
- To indicate that 100 locations should be reserved for integer array p, the programmer writes the declaration
p[ 100 ];
- A for statement must be used to initialize the elements of a 15-element array to zero.
- Nested for statements must be used to total the elements of a two-dimensional array.
|
7.8 |
Write C++ statements to accomplish each of the following:
- Display the value of element 6 of character array f.
- Input a value into element 4 of one-dimensional floating-point array b.
- Initialize each of the 5 elements of one-dimensional integer array g to 8.
- Total and print the elements of floating-point array c of 100 elements.
- Copy array a into the first portion of array b. Assume double a[ 11 ], b[ 34 ];
- Determine and print the smallest and largest values contained in 99-element floating-point array w.
|
7.9 |
Consider a 2-by-3 integer array t.
- Write a declaration for t.
- How many rows does t have?
- How many columns does t have?
- How many elements does t have?
- Write the names of all the elements in row 1 of t.
- Write the names of all the elements in column 2 of t.
- Write a single statement that sets the element of t in row 1 and column 2 to zero.
- Write a series of statements that initialize each element of t to zero. Do not use a loop.
- Write a nested for statement that initializes each element of t to zero.
- Write a statement that inputs the values for the elements of t from the terminal.
- Write a series of statements that determine and print the smallest value in array t.
- Write a statement that displays the elements in row 0 of t.
- Write a statement that totals the elements in column 3 of t.
- Write a series of statements that prints the array t in neat, tabular format. List the column subscripts as headings across the top and list the row subscripts at the left of each row.
|
7.10 |
Use a one-dimensional array to solve the following problem. A company pays its salespeople on a commission basis. The salespeople each receive $200 per week plus 9 percent of their gross sales for that week. For example, a salesperson who grosses $5000 in sales in a week receives $200 plus 9 percent of $5000, or a total of $650. Write a program (using an array of counters) that determines how many of the salespeople earned salaries in each of the following ranges (assume that each salesperson's salary is truncated to an integer amount):
- $200$299
- $300$399
- $400$499
- $500$599
- $600$699
- $700$799
- $800$899
- $900$999
- $1000 and over
|
7.11 |
(Bubble Sort) In the bubble sort algorithm, smaller values gradually "bubble" their way upward to the top of the array like air bubbles rising in water, while the larger values sink to the bottom. The bubble sort makes several passes through the array. On each pass, successive pairs of elements are compared. If a pair is in increasing order (or the values are identical), we leave the values as they are. If a pair is in decreasing order, their values are swapped in the array. Write a program that sorts an array of 10 integers using bubble sort.
|
7.12 |
The bubble sort described in Exercise 7.11 is inefficient for large arrays. Make the following simple modifications to improve the performance of the bubble sort:
- After the first pass, the largest number is guaranteed to be in the highest-numbered element of the array; after the second pass, the two highest numbers are "in place," and so on. Instead of making nine comparisons on every pass, modify the bubble sort to make eight comparisons on the second pass, seven on the third pass, and so on.
- The data in the array may already be in the proper order or near-proper order, so why make nine passes if fewer will suffice? Modify the sort to check at the end of each pass if any swaps have been made. If none have been made, then the data must already be in the proper order, so the program should terminate. If swaps have been made, then at least one more pass is needed.
|
7.13 |
Write single statements that perform the following one-dimensional array operations:
- Initialize the 10 elements of integer array counts to zero.
- Add 1 to each of the 15 elements of integer array bonus.
- Read 12 values for double array monthlyTemperatures from the keyboard.
- Print the 5 values of integer array bestScores in column format.
|
7.14 |
Find the error(s) in each of the following statements:
- Assume that: char str[ 5 ];
cin >> str; // user types "hello"
- Assume that: int a[ 3 ];
cout << a[ 1 ] << " " << a[ 2 ] << " " << a[ 3 ] << endl;
- double f[ 3 ] = { 1.1, 10.01, 100.001, 1000.0001 };
- Assume that: double d[ 2 ][ 10 ];
d[ 1, 9 ] = 2.345;
|
7.15 |
Use a one-dimensional array to solve the following problem. Read in 20 numbers, each of which is between 10 and 100, inclusive. As each number is read, validate it and store it in the array only if it is not a duplicate of a number already read. After reading all the values, display only the unique values that the user entered. Provide for the "worst case" in which all 20 numbers are different. Use the smallest possible array to solve this problem.
|
7.16 |
Label the elements of a 3-by-5 one-dimensional array sales to indicate the order in which they are set to zero by the following program segment:
for ( row = 0; row < 3; row++ )
for ( column = 0; column < 5; column++ )
sales[ row ][ column ] = 0;
|
7.17 |
Write a program that simulates the rolling of two dice. The program should use rand to roll the first die and should use rand again to roll the second die. The sum of the two values should then be calculated. [ Note: Each die can show an integer value from 1 to 6, so the sum of the two values will vary from 2 to 12, with 7 being the most frequent sum and 2 and 12 being the least frequent sums.] Figure 7.32 shows the 36 possible combinations of the two dice. Your program should roll the two dice 36,000 times. Use a one-dimensional array to tally the numbers of times each possible sum appears. Print the results in a tabular format. Also, determine if the totals are reasonable (i.e., there are six ways to roll a 7, so approximately one-sixth of all the rolls should be 7).
Figure 7.32. The 36 possible outcomes of rolling two dice.
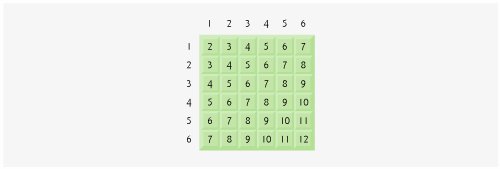
|
7.18 |
What does the following program do?
1 // Ex. 7.18: Ex07_18.cpp
2 // What does this program do?
3 #include
4 using std::cout;
5 using std::endl;
6
7 int whatIsThis( int [], int ); // function prototype
8
9 int main()
10 {
11 const int arraySize = 10;
12 int a[ arraySize ] = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 };
13
14 int result = whatIsThis( a, arraySize );
15
16 cout << "Result is " << result << endl;
17 return 0; // indicates successful termination
18 } // end main
19
20 // What does this function do?
21 int whatIsThis( int b[], int size )
22 {
23 if ( size == 1 ) // base case
24 return b[ 0 ];
25 else // recursive step
26 return b[ size - 1 ] + whatIsThis( b, size - 1 );
27 } // end function whatIsThis
|
7.19 |
Modify the program of Fig. 6.11 to play 1000 games of craps. The program should keep track of the statistics and answer the following questions:
- How many games are won on the 1st roll, 2nd roll, ..., 20th roll, and after the 20th roll?
- How many games are lost on the 1st roll, 2nd roll, ..., 20th roll, and after the 20th roll?
- What are the chances of winning at craps? [ Note: You should discover that craps is one of the fairest casino games. What do you suppose this means?]
- What is the average length of a game of craps?
- Do the chances of winning improve with the length of the game?
|
7.20 |
( Airline Reservations System) A small airline has just purchased a computer for its new automated reservations system. You have been asked to program the new system. You are to write a program to assign seats on each flight of the airline's only plane (capacity: 10 seats).
Your program should display the following menu of alternativesPlease type 1 for "First Class" and Please type 2 for "Economy". If the person types 1, your program should assign a seat in the first class section (seats 1-5). If the person types 2, your program should assign a seat in the economy section (seats 6-10). Your program should print a boarding pass indicating the person's seat number and whether it is in the first class or economy section of the plane.
Use a one-dimensional array to represent the seating chart of the plane. Initialize all the elements of the array to 0 to indicate that all seats are empty. As each seat is assigned, set the corresponding elements of the array to 1 to indicate that the seat is no longer available.
Your program should, of course, never assign a seat that has already been assigned. When the first class section is full, your program should ask the person if it is acceptable to be placed in the economy section (and vice versa). If yes, then make the appropriate seat assignment. If no, then print the message "Next flight leaves in 3 hours".
|
7.21 |
What does the following program do?
1 // Ex. 7.21: Ex07_21.cpp
2 // What does this program do?
3 #include
4 using std::cout;
5 using std::endl;
6
7 void someFunction( int [], int, int ); // function prototype
8
9 int main()
10 {
11 const int arraySize = 10;
12 int a[ arraySize ] = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 };
13
14 cout << "The values in the array are:" << endl;
15 someFunction( a, 0, arraySize );
16 cout << endl;
17 return 0; // indicates successful termination
18 } // end main
19
20 // What does this function do?
21 void someFunction( int b[], int current, int size )
22 {
23 if ( current < size )
24 {
25 someFunction( b, current + 1, size );
26 cout << b[ current ] << " ";
27 } // end if
28 } // end function someFunction
|
|
|
7.22 |
Use a two-dimensional array to solve the following problem. A company has four salespeople (1 to 4) who sell five different products (1 to 5). Once a day, each salesperson passes in a slip for each different type of product sold. Each slip contains the following:
- The salesperson number
- The product number
- The total dollar value of that product sold that day
Thus, each salesperson passes in between 0 and 5 sales slips per day. Assume that the information from all of the slips for last month is available. Write a program that will read all this information for last month's sales and summarize the total sales by salesperson by product. All totals should be stored in the two-dimensional array sales. After processing all the information for last month, print the results in tabular format with each of the columns representing a particular salesperson and each of the rows representing a particular product. Cross total each row to get the total sales of each product for last month; cross total each column to get the total sales by salesperson for last month. Your tabular printout should include these cross totals to the right of the totaled rows and to the bottom of the totaled columns.
|
7.23 |
( Turtle Graphics ) The Logo language, which is popular among elementary school children, made the concept of turtle graphics famous. Imagine a mechanical turtle that walks around the room under the control of a C++ program. The turtle holds a pen in one of two positions, up or down. While the pen is down, the turtle traces out shapes as it moves; while the pen is up, the turtle moves about freely without writing anything. In this problem, you will simulate the operation of the turtle and create a computerized sketchpad as well.
Use a 20-by-20 array floor that is initialized to zeros. Read commands from an array that contains them. Keep track of the current position of the turtle at all times and whether the pen is currently up or down. Assume that the turtle always starts at position (0, 0) of the floor with its pen up. The set of turtle commands your program must process are shown in Fig. 7.33.
Figure 7.33. Turtle graphics commands.
(This item is displayed on page 396 in the print version)
Command
|
Meaning
|
1
|
Pen up
|
2
|
Pen down
|
3
|
Turn right
|
4
|
Turn left
|
5,10
|
Move forward 10 spaces (or a number other than 10)
|
6
|
Print the 20-by-20 array
|
9
|
End of data (sentinel)
|
Suppose that the turtle is somewhere near the center of the floor. The following "program" would draw and print a 12-by-12 square and end with the pen in the up position:
2
5,12
3
5,12
3
5,12
3
5,12
1
6
9
As the turtle moves with the pen down, set the appropriate elements of array floor to 1's. When the 6 command (print) is given, wherever there is a 1 in the array, display an asterisk or some other character you choose. Wherever there is a zero, display a blank. Write a program to implement the turtle graphics capabilities discussed here. Write several turtle graphics programs to draw interesting shapes. Add other commands to increase the power of your turtle graphics language.
|
7.24 |
( Knight's Tour ) One of the more interesting puzzlers for chess buffs is the Knight's Tour problem. The question is this: Can the chess piece called the knight move around an empty chessboard and touch each of the 64 squares once and only once? We study this intriguing problem in depth in this exercise.
The knight makes L-shaped moves (over two in one direction and then over one in a perpendicular direction). Thus, from a square in the middle of an empty chessboard, the knight can make eight different moves (numbered 0 through 7) as shown in Fig. 7.34.
Figure 7.34. The eight possible moves of the knight.
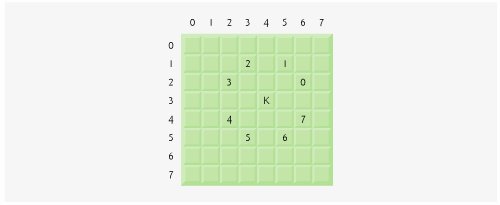
- Draw an 8-by-8 chessboard on a sheet of paper and attempt a Knight's Tour by hand. Put a 1 in the first square you move to, a 2 in the second square, a 3 in the third, etc. Before starting the tour, estimate how far you think you will get, remembering that a full tour consists of 64 moves. How far did you get? Was this close to your estimate?
- Now let us develop a program that will move the knight around a chessboard. The board is represented by an 8-by-8 two-dimensional array board. Each of the squares is initialized to zero. We describe each of the eight possible moves in terms of both their horizontal and vertical components. For example, a move of type 0, as shown in Fig. 7.34, consists of moving two squares horizontally to the right and one square vertically upward. Move 2 consists of moving one square horizontally to the left and two squares vertically upward. Horizontal moves to the left and vertical moves upward are indicated with negative numbers. The eight moves may be described by two one-dimensional arrays, horizontal and vertical, as follows:
horizontal[ 0 ] = 2
horizontal[ 1 ] = 1
horizontal[ 2 ] = -1
horizontal[ 3 ] = -2
horizontal[ 4 ] = -2
horizontal[ 5 ] = -1 horizontal[ 6 ] = 1 horizontal[ 7 ] = 2 vertical[ 0 ] = -1 vertical[ 1 ] = -2 vertical[ 2 ] = -2 vertical[ 3 ] = -1 vertical[ 4 ] = 1 vertical[ 5 ] = 2 vertical[ 6 ] = 2 vertical[ 7 ] = 1
Let the variables currentRow and currentColumn indicate the row and column of the knight's current position. To make a move of type moveNumber, where moveNumber is between 0 and 7, your program uses the statements
currentRow += vertical[ moveNumber ];
currentColumn += horizontal[ moveNumber ];
Keep a counter that varies from 1 to 64. Record the latest count in each square the knight moves to. Remember to test each potential move to see if the knight has already visited that square, and, of course, test every potential move to make sure that the knight does not land off the chessboard. Now write a program to move the knight around the chessboard. Run the program. How many moves did the knight make?
- After attempting to write and run a Knight's Tour program, you have probably developed some valuable insights. We will use these to develop a heuristic (or strategy) for moving the knight. Heuristics do not guarantee success, but a carefully developed heuristic greatly improves the chance of success. You may have observed that the outer squares are more troublesome than the squares nearer the center of the board. In fact, the most troublesome, or inaccessible, squares are the four corners.
Intuition may suggest that you should attempt to move the knight to the most troublesome squares first and leave open those that are easiest to get to, so when the board gets congested near the end of the tour, there will be a greater chance of success.
We may develop an "accessibility heuristic" by classifying each square according to how accessible it is and then always moving the knight to the square (within the knight's L-shaped moves, of course) that is most inaccessible. We label a two-dimensional array accessibility with numbers indicating from how many squares each particular square is accessible. On a blank chessboard, each center square is rated as 8, each corner square is rated as 2 and the other squares have accessibility numbers of 3, 4 or 6 as follows:
2 3 4 4 4 4 3 2
3 4 6 6 6 6 4 3
4 6 8 8 8 8 6 4
4 6 8 8 8 8 6 4
4 6 8 8 8 8 6 4
4 6 8 8 8 8 6 4
3 4 6 6 6 6 4 3
2 3 4 4 4 4 3 2
Now write a version of the Knight's Tour program using the accessibility heuristic. At any time, the knight should move to the square with the lowest accessibility number. In case of a tie, the knight may move to any of the tied squares. Therefore, the tour may begin in any of the four corners. [Note: As the knight moves around the chessboard, your program should reduce the accessibility numbers as more and more squares become occupied. In this way, at any given time during the tour, each available square's accessibility number will remain equal to precisely the number of squares from which that square may be reached.] Run this version of your program. Did you get a full tour? Now modify the program to run 64 tours, one starting from each square of the chessboard. How many full tours did you get?
- Write a version of the Knight's Tour program which, when encountering a tie between two or more squares, decides what square to choose by looking ahead to those squares reachable from the "tied" squares. Your program should move to the square for which the next move would arrive at a square with the lowest accessibility number.
|
7.25 |
(Knight's Tour: Brute Force Approaches) In Exercise 7.24, we developed a solution to the Knight's Tour problem. The approach used, called the "accessibility heuristic," generates many solutions and executes efficiently.
As computers continue increasing in power, we will be able to solve more problems with sheer computer power and relatively unsophisticated algorithms. This is the "brute force" approach to problem solving.
- Use random number generation to enable the knight to walk around the chessboard (in its legitimate L-shaped moves, of course) at random. Your program should run one tour and print the final chessboard. How far did the knight get?
- Most likely, the preceding program produced a relatively short tour. Now modify your program to attempt 1000 tours. Use a one-dimensional array to keep track of the number of tours of each length. When your program finishes attempting the 1000 tours, it should print this information in neat tabular format. What was the best result?
- Most likely, the preceding program gave you some "respectable" tours, but no full tours. Now "pull all the stops out" and simply let your program run until it produces a full tour. [Caution: This version of the program could run for hours on a powerful computer.] Once again, keep a table of the number of tours of each length, and print this table when the first full tour is found. How many tours did your program attempt before producing a full tour? How much time did it take?
- Compare the brute force version of the Knight's Tour with the accessibility heuristic version. Which required a more careful study of the problem? Which algorithm was more difficult to develop? Which required more computer power? Could we be certain (in advance) of obtaining a full tour with the accessibility heuristic approach? Could we be certain (in advance) of obtaining a full tour with the brute force approach? Argue the pros and cons of brute force problem solving in general.
|
7.26 |
(Eight Queens) Another puzzler for chess buffs is the Eight Queens problem. Simply stated: Is it possible to place eight queens on an empty chessboard so that no queen is "attacking" any other, i.e., no two queens are in the same row, the same column, or along the same diagonal? Use the thinking developed in Exercise 7.24 to formulate a heuristic for solving the Eight Queens problem. Run your program. [Hint: It is possible to assign a value to each square of the chessboard indicating how many squares of an empty chessboard are "eliminated" if a queen is placed in that square. Each of the corners would be assigned the value 22, as in Fig. 7.35.] Once these "elimination numbers" are placed in all 64 squares, an appropriate heuristic might be: Place the next queen in the square with the smallest elimination number. Why is this strategy intuitively appealing?
Figure 7.35. The 22 squares eliminated by placing a queen in the upper-left corner.
(This item is displayed on page 399 in the print version)
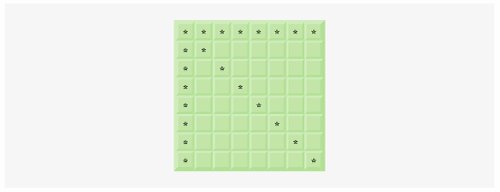
|
7.27 |
(Eight Queens: Brute Force Approaches) In this exercise, you will develop several brute-force approaches to solving the Eight Queens problem introduced in Exercise 7.26.
- Solve the Eight Queens exercise, using the random brute force technique developed in Exercise 7.25.
- Use an exhaustive technique, i.e., try all possible combinations of eight queens on the chessboard.
- Why do you suppose the exhaustive brute force approach may not be appropriate for solving the Knight's Tour problem?
- Compare and contrast the random brute force and exhaustive brute force approaches in general.
|
7.28 |
(Knight's Tour: Closed-Tour Test) In the Knight's Tour, a full tour occurs when the knight makes 64 moves touching each square of the chess board once and only once. A closed tour occurs when the 64th move is one move away from the location in which the knight started the tour. Modify the Knight's Tour program you wrote in Exercise 7.24 to test for a closed tour if a full tour has occurred.
|
7.29 |
(The Sieve of Eratosthenes) A prime integer is any integer that is evenly divisible only by itself and 1. The Sieve of Eratosthenes is a method of finding prime numbers. It operates as follows:
- Create an array with all elements initialized to 1 (true). Array elements with prime subscripts will remain 1. All other array elements will eventually be set to zero. You will ignore elements 0 and 1 in this exercise.
- Starting with array subscript 2, every time an array element is found whose value is 1, loop through the remainder of the array and set to zero every element whose subscript is a multiple of the subscript for the element with value 1. For array subscript 2, all elements beyond 2 in the array that are multiples of 2 will be set to zero (subscripts 4, 6, 8, 10, etc.); for array subscript 3, all elements beyond 3 in the array that are multiples of 3 will be set to zero (subscripts 6, 9, 12, 15, etc.); and so on.
When this process is complete, the array elements that are still set to one indicate that the subscript is a prime number. These subscripts can then be printed. Write a program that uses an array of 1000 elements to determine and print the prime numbers between 2 and 999. Ignore element 0 of the array.
|
7.30 |
(Bucket Sort) A bucket sort begins with a one-dimensional array of positive integers to be sorted and a two-dimensional array of integers with rows subscripted from 0 to 9 and columns subscripted from 0 to n1, where n is the number of values in the array to be sorted. Each row of the two-dimensional array is referred to as a bucket. Write a function bucketSort that takes an integer array and the array size as arguments and performs as follows:
- Place each value of the one-dimensional array into a row of the bucket array based on the value's ones digit. For example, 97 is placed in row 7, 3 is placed in row 3 and 100 is placed in row 0. This is called a "distribution pass."
- Loop through the bucket array row by row, and copy the values back to the original array. This is called a "gathering pass." The new order of the preceding values in the one-dimensional array is 100, 3 and 97.
- Repeat this process for each subsequent digit position (tens, hundreds, thousands, etc.).
On the second pass, 100 is placed in row 0, 3 is placed in row 0 (because 3 has no tens digit) and 97 is placed in row 9. After the gathering pass, the order of the values in the one-dimensional array is 100, 3 and 97. On the third pass, 100 is placed in row 1, 3 is placed in row zero and 97 is placed in row zero (after the 3). After the last gathering pass, the original array is now in sorted order.
Note that the two-dimensional array of buckets is 10 times the size of the integer array being sorted. This sorting technique provides better performance than a insertion sort, but requires much more memory. The insertion sort requires space for only one additional element of data. This is an example of the spacetime trade-off: The bucket sort uses more memory than the insertion sort, but performs better. This version of the bucket sort requires copying all the data back to the original array on each pass. Another possibility is to create a second two-dimensional bucket array and repeatedly swap the data between the two bucket arrays.
|
|