7.7 |
What is the value of x after each of the following statements is executed?
- x = Math.Abs( 7.5 );
- x = Math.Floor( 7.5 );
- x = Math.Abs( 0.0 );
- x = Math.Ceiling( 0.0 );
- x = Math.Abs( -6.4 );
- x = Math.Ceiling( -6.4 );
- x = Math.Ceiling( -Math.Abs( -8 + Math.Floor( -5.5 ) ) );
|
7.8 |
A parking garage charges a $2.00 minimum fee to park for up to three hours. The garage charges an additional $0.50 per hour for each hour or part thereof in excess of three hours. The maximum charge for any given 24-hour period is $10.00. Assume that no car parks for longer than 24 hours at a time. Write an application that calculates and displays the parking charges for each customer who parked in the garage yesterday. You should enter the hours parked for each customer. The application should display the charge for the current customer and should calculate and display the running total of yesterday's receipts. The application should use method CalculateCharges to determine the charge for each customer.
|
7.9 |
An application of method Math.Floor is rounding a value to the nearest integer. The statement
y = Math.Floor( x + 0.5 );
will round the number x to the nearest integer and assign the result to y. Write an application that reads double values and uses the preceding statement to round each of the numbers to the nearest integer. For each number processed, display both the original number and the rounded number.
|
7.10 |
Math.Floor may be used to round a number to a specific decimal place. The statement
y = Math.Floor( x * 10 + 0.5 ) / 10;
rounds x to the tenths position (i.e., the first position to the right of the decimal point). The statement
y = Math.Floor( x * 100 + 0.5 ) / 100;
rounds x to the hundredths position (i.e., the second position to the right of the decimal point). Write an application that defines four methods for rounding a number x in various ways:
- RoundToInteger( number )
- RoundToTenths( number )
- RoundToHundredths( number )
- RoundToThousandths( number )
For each value read, your application should display the original value, the number rounded to the nearest integer, the number rounded to the nearest tenth, the number rounded to the nearest hundredth and the number rounded to the nearest thousandth.
|
7.11 |
Answer each of the following questions:
- What does it mean to choose numbers "at random?"
- Why is the Random class useful for simulating games of chance?
- Why is it often necessary to scale or shift the values produced by a Random object?
- Why is computerized simulation of real-world situations a useful technique?
|
7.12 |
Write statements that assign random integers to the variable n in the following ranges. Assume Random randomNumbers = new Random() has been defined and use the two-parameter version of the method Random.Next.
- 1
n images/U2264.jpg border=0> 2
- n
images/U2264.jpg border=0> 100
- n
images/U2264.jpg border=0> 9
- n
images/U2264.jpg border=0> 1112
- n
images/U2264.jpg border=0> 1
- images/U2264.jpg border=0> n
images/U2264.jpg border=0> 11
|
7.13 |
For each of the following sets of integers, write a single statement that will display a number at random from the set. Assume Random randomNumbers = new Random() has been defined and use the one-parameter version of method Random.Next.
- 2, 4, 6, 8, 10.
- 3, 5, 7, 9, 11.
- 6, 10, 14, 18, 22.
|
7.14 |
Write a method IntegerPower( base, exponent ) that returns the value of
baseexponent
For example, IntegerPower( 3, 4 ) calculates 34 (or 3 * 3 * 3 * 3). Assume that exponent is a positive, nonzero integer and that base is an integer. Method IntegerPower should use a for or while loop to control the calculation. Do not use any Math-library methods. Incorporate this method into an application that reads integer values for base and exponent and performs the calculation with the IntegerPower method.
|
|
|
7.15 |
Write method Hypotenuse that calculates the length of the hypotenuse of a right triangle when the lengths of the other two sides are given. (Use the sample data in Fig. 7.26.) The method should take two arguments of type double and return the hypotenuse as a double. Incorporate this method into an application that reads values for side1 and side2 and performs the calculation with the Hypotenuse method. Determine the length of the hypotenuse for each of the triangles in Fig. 7.26.
Figure 7.26. Values for the sides of triangles in Exercise 7.15.
Triangle
|
Side 1
|
Side 2
|
1
|
3.0
|
4.0
|
2
|
5.0
|
12.0
|
3
|
8.0
|
15.0
|
|
7.16 |
Write method Multiple that determines, for a pair of integers, whether the second integer is a multiple of the first. The method should take two integer arguments and return true if the second is a multiple of the first and false otherwise. Incorporate this method into an application that inputs a series of pairs of integers (one pair at a time) and determines whether the second value in each pair is a multiple of the first.
|
7.17 |
Write method IsEven that uses the remainder operator (%) to determine whether an integer is even. The method should take an integer argument, and return true if the integer is even and false otherwise. Incorporate this method into an application that inputs a sequence of integers (one at a time) and determines whether each is even or odd.
|
7.18 |
Write method SquareOfAsterisks that displays a solid square (the same number of rows and columns) of asterisks whose side length is specified in integer parameter side. For example, if side is 4, the method should display
****
****
****
****
Incorporate this method into an application that reads an integer value for side from the user and outputs the asterisks with the SquareOfAsterisks method.
|
7.19 |
Modify the method created in Exercise 7.18 to form the square out of whatever character is contained in character parameter FillCharacter. Thus, if side is 5 and FillCharacter is "#," the method should display
#####
#####
#####
#####
#####
[Hint: Use the expression Convert.ToChar( Console.Read() ) to read a character from the user.]
|
7.20 |
Write an application that prompts the user for the radius of a circle and uses method CircleArea to calculate the area of the circle.
|
|
|
7.21 |
Write code segments that accomplish each of the following tasks:
- Calculate the integer part of the quotient when integer a is divided by integer b.
- Calculate the integer remainder when integer a is divided by integer b.
- Use the application pieces developed in parts (a) and (b) to write a method DisplayDigits that receives an integer between 1 and 99999 and displays it as a sequence of digits, separating each pair of digits by two spaces. For example, the integer 4562 should appear as
4 5 6 2
- Incorporate the method developed in part (c) into an application that inputs an integer and calls DisplayDigits by passing the method the integer entered. Display the results.
|
7.22 |
Implement the following integer methods:
- Method Celsius returns the Celsius equivalent of a Fahrenheit temperature, using the calculation
c = 5.0 / 9.0 * ( f - 32 );
- Method Fahrenheit returns the Fahrenheit equivalent of a Celsius temperature, using the calculation
f = 9.0 / 5.0 * c + 32;
- Use the methods from parts (a) and (b) to write an application that enables the user either to enter a Fahrenheit temperature and display the Celsius equivalent or to enter a Celsius temperature and display the Fahrenheit equivalent.
|
7.23 |
Write a method Minimum3 that returns the smallest of three floating-point numbers. Use the Math.Min method to implement Minimum3. Incorporate the method into an application that reads three values from the user, determines the smallest value and displays the result.
|
7.24 |
An integer number is said to be a perfect number if its factors, including 1 (but not the number itself), sum to the number. For example, 6 is a perfect number, because 6 = 1 + 2 + 3. Write method Perfect that determines whether parameter number is a perfect number. Use this method in an application that determines and displays all the perfect numbers between 2 and 1000. Display the factors of each perfect number to confirm that the number is indeed perfect.
|
7.25 |
An integer is said to be prime if it is greater than 1 and divisible by only 1 and itself. For example, 2, 3, 5 and 7 are prime, but 4, 6, 8 and 9 are not.
- Write a method that determines whether a number is prime.
- Use this method in an application that determines and displays all the prime numbers less than 10,000.
- Initially, you might think that n/2 is the upper limit for which you must test to see whether a number is prime, but you need only go as high as the square root of n. Rewrite the application, and run it both ways.
|
7.26 |
Write a method that takes an integer value and returns the number with its digits reversed. For example, given the number 7631, the method should return 1367. Incorporate the method into an application that reads a value from the user and displays the result.
|
7.27 |
The greatest common divisor (GCD) of two integers is the largest integer that evenly divides each of the two numbers. Write method Gcd that returns the greatest common divisor of two integers. Incorporate the method into an application that reads two values from the user and displays the result.
|
|
|
7.28 |
Write method QualityPoints that inputs a student's average and returns 4 if the student's average is 90100, 3 if the average is 8089, 2 if the average is 7079, 1 if the average is 6069 and 0 if the average is lower than 60. Incorporate the method into an application that reads a value from the user and displays the result.
|
7.29 |
Write an application that simulates coin tossing. Let the application toss a coin each time the user chooses the "Toss Coin" menu option. Count the number of times each side of the coin appears. Display the results. The application should call a separate method Flip that takes no arguments and returns false for tails and true for heads. [Note: If the application realistically simulates coin tossing, each side of the coin should appear approximately half the time.]
|
7.30 |
Computers are playing an increasing role in education. Write an application that will help an elementary school student learn multiplication. Use a Random object to produce two positive onedigit integers. The application should then prompt the user with a question, such as
How much is 6 times 7?
The student then inputs the answer. Next, the application checks the student's answer. If it is correct, display the message "Very good!" and ask another multiplication question. If the answer is wrong, display the message "No. Please try again." and let the student try the same question repeatedly until the student finally gets it right. A separate method should be used to generate each new question. This method should be called once when the application begins execution and each time the user answers the question correctly.
|
7.31 |
The use of computers in education is referred to as computer-assisted instruction (CAI). One problem that develops in CAI environments is student fatigue. This problem can be eliminated by varying the computer's responses to hold the student's attention. Modify the application of Exercise 7.30 so that various comments are displayed for each correct answer and each incorrect answer as follows:
Responses to a correct answer:
Very good!
Excellent!
Nice work!
Keep up the good work!
Responses to an incorrect answer:
No. Please try again.
Wrong. Try once more.
Don't give up!
No. Keep trying.
Use random-number generation to choose a number from 1 to 4 that will be used to select an appropriate response to each answer. Use a switch statement to issue the responses.
|
7.32 |
More sophisticated computer-assisted instruction systems monitor the student's performance over a period of time. The decision to begin a new topic is often based on the student's success with previous topics. Modify the application of Exercise 7.31 to count the number of correct and incorrect responses typed by the student. After the student types 10 answers, your application should calculate the percentage of correct responses. If the percentage is lower than 75%, display Please ask your instructor for extra help and reset the application so another student can try it.
|
7.33 |
Write an application that plays "guess the number" as follows: Your application chooses the number to be guessed by selecting a random integer in the range 1 to 1000. The application displays the prompt Guess a number between 1 and 1000. The player inputs a first guess. If the player's guess is incorrect, your application should display Too high. Try again. or Too low. Try again. to help the player "zero in" on the correct answer. The application should prompt the user for the next guess. When the user enters the correct answer, display Congratulations. You guessed the number! and allow the user to choose whether to play again. [Note: The guessing technique employed in this problem is similar to a binary search, which is discussed in Chapter 24.]
|
7.34 |
Modify the application of Exercise 7.33 to count the number of guesses the player makes. If the number is 10 or fewer, display Either you know the secret or you got lucky! If the player guesses the number in 10 tries, display Aha! You know the secret! If the player makes more than 10 guesses, display Youshould be able to do better! Why should it take no more than 10 guesses? Well, with each "good guess," the player should be able to eliminate half of the numbers. Now show why any number from 1 to 1000 can be guessed in 10 or fewer tries.
|
7.35 |
Exercises 7.307.32 developed a computer-assisted instruction application to teach an elementary school student multiplication. Perform the following enhancements:
- Modify the application to allow the user to choose a "school grade-level" of 1 or 2. Grade level 1 means that the application should use only single-digit numbers in the problems. Grade level 2 means that the application should use numbers as large as two digits.
- Modify the application to allow the user to pick the type of arithmetic problems he or she wishes to study. An option of 1 means addition problems only, 2 means subtraction problems only, 3 means multiplication problems only, 4 means division problems only and 5 means a random mixture of problems of all these types.
|
7.36 |
Write method Distance to calculate the distance between two points (x1, y1) and (x2, y2). All numbers and return values should be of type double. Incorporate this method into an application that enables the user to enter the coordinates of the points.
|
7.37 |
Modify the craps application of Fig. 7.9 to allow wagering. Initialize variable balance to 1000 dollars. Prompt the player to enter a wager. Check that wager is less than or equal to balance, and if it is not, have the user re-enter wager until a valid wager is entered. After a correct wager is entered, run one game of craps. If the player wins, increase balance by wager and display the new balance. If the player loses, decrease balance by wager, display the new balance, check whether balance has become zero and, if so, display the message "Sorry. You busted!"
|
7.38 |
Write an application that displays a table of the binary, octal, and hexadecimal equivalents of the decimal numbers in the range 1256. If you are not familiar with these number systems, read Appendix B, Number Systems first.
|
7.39 |
Write recursive method Power( base, exponent ) that, when called, returns
baseexponent
For example, Power( 3, 4 ) = 3 * 3 * 3 * 3. Assume that exponent is an integer greater than or equal to 1. [Hint: The recursion step should use the relationship
base exponent = base · base exponent 1
and the terminating condition occurs when exponent is equal to 1, because
base1 = base]
Incorporate this method into an application that enables the user to enter the base and exponent.
|
7.40 |
(Towers of Hanoi) Every budding computer scientist must grapple with certain classic problems, and the Towers of Hanoi (see Fig. 7.27) is one of the most famous. Legend has it that in a temple in the Far East, priests are attempting to move a stack of disks from one peg to another. The initial stack has 64 disks threaded onto one peg and arranged from bottom to top by decreasing size. The priests are attempting to move the stack from this peg to a second peg under the constraints that exactly one disk is moved at a time and at no time may a larger disk be placed above a smaller disk. A third peg is available for temporarily holding disks. Supposedly, the world will end when the priests complete their task, so there is little incentive for us to facilitate their efforts.
Figure 7.27. The Towers of Hanoi for the case with four disks.
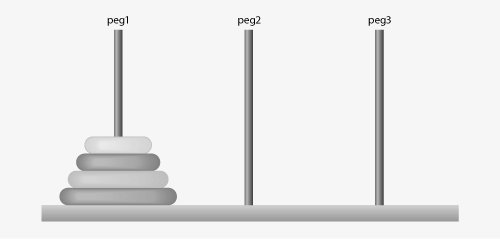
Let us assume that the priests are attempting to move the disks from peg 1 to peg 3. We wish to develop an algorithm that will print the precise sequence of peg-to-peg disk transfers.
If we were to approach this problem with conventional methods, we would rapidly find ourselves hopelessly knotted up in managing the disks. Instead, if we attack the problem with recursion in mind, it immediately becomes tractable. Moving n disks can be viewed in terms of moving only n 1 disks (hence the recursion) as follows:
- Move n 1 disks from peg 1 to peg 2, using peg 3 as a temporary holding area.
- Move the last disk (the largest) from peg 1 to peg 3.
- Move the n 1 disks from peg 2 to peg 3, using peg 1 as a temporary holding area.
The process ends when the last task involves moving n = 1 disk (i.e., the base case). This task is accomplished by simply moving the disk, without the need for a temporary holding area.
Write an application to solve the Towers of Hanoi problem. Allow the user to enter the number of disks. Use a recursive Tower method with four parameters:
- the number of disks to be moved,
- the peg on which these disks are initially threaded,
- the peg to which this stack of disks is to be moved, and
- the peg to be used as a temporary holding area.
Your application should display the precise instructions it will take to move the disks from the starting peg to the destination peg. For example, to move a stack of three disks from peg 1 to peg 3, your application should print the following series of moves:
1 --> 3 (This notation means "Move one disk from peg 1 to peg 3.")
1 --> 2
3 --> 2
1 --> 3
2 --> 1
2 --> 3
1 --> 3
|
7.41 |
What does the following method do?
// Parameter b must be a positive
// integer to prevent infinite recursion
public int Mystery( int a, int b )
{
if ( b == 1 )
return a;
else
return a + Mystery( a, b - 1 );
}
|
7.42 |
Find the error in the following recursive method, and explain how to correct it:
public int Sum( int n )
{
if ( n == 0 )
return 0;
else
return n + Sum( n );
}
|