5.10 |
Compare and contrast the if single-selection statement and the while repetition statement. How are these two statements similar? How are they different?
|
|
|
5.11 |
Explain what happens when a C# application attempts to divide one integer by another. What happens to the fractional part of the calculation? How can you avoid that outcome?
|
5.12 |
Describe the two ways in which control statements can be combined.
|
5.13 |
What type of repetition would be appropriate for calculating the sum of the first 100 positive integers? What type of repetition would be appropriate for calculating the sum of an arbitrary number of positive integers? Briefly describe how each of these tasks could be performed.
|
5.14 |
What is the difference between the prefix increment operator and the postfix increment operator?
|
5.15 |
Identify and correct the errors in each of the following pieces of code. [Note: There may be more than one error in each piece of code.]
-
if ( age >= 65 );
Console.WriteLine( "Age greater than or equal to 65" );
else
Console.WriteLine( "Age is less than 65 )";
-
int x = 1, total;
while ( x <= 10 )
{
total += x;
++x;
}
-
while ( x <= 100 )
total += x;
++x;
-
while ( y > 0 )
{
Console.WriteLine( y );
++y;
|
5.16 |
What does the following application print?
1 // Ex. 5.16: Mystery.cs
2 using System;
3
4 public class Mystery
5 {
6 public static void Main( string[] args )
7 {
8 int y;
9 int x = 1;
10 int total = 0;
11
12 while ( x <= 10 )
13 {
14 y = x * x;
15 Console.WriteLine( y );
16 total += y;
17 x++;
18 } // end while
19
20 Console.WriteLine( "Total is {0}", total );
21 } // end Main
22 } // end class Mystery
For Exercise 5.17 through Exercise 5.20, perform each of the following steps:
- Read the problem statement.
- Formulate the algorithm using pseudocode and top-down, stepwise refinement.
- Write a C# application.
- Test, debug and execute the C# application.
- Process three complete sets of data.
|
5.17 |
Drivers are concerned with the mileage their automobiles get. One driver has kept track of several tankfuls of gasoline by recording the miles driven and gallons used for each tankful. Develop a C# application that will input the miles driven and gallons used (both as integers) for each tankful. The application should calculate and display the miles per gallon obtained for each tankful and print the combined miles per gallon obtained for all tankfuls up to this point. All averaging calculations should produce floating-point results. Display the results rounded to the nearest hundredth. Use the Console class's ReadLine method and sentinel-controlled repetition to obtain the data from the user.
|
5.18 |
Develop a C# application that will determine whether any of several department-store customers has exceeded the credit limit on a charge account. For each customer, the following facts are available:
- account number
- balance at the beginning of the month
- total of all items charged by the customer this month
- total of all credits applied to the customer's account this month
- allowed credit limit.
The application should input all these facts as integers, calculate the new balance (= beginning balance + charges credits), display the new balance and determine whether the new balance exceeds the customer's credit limit. For those customers whose credit limit is exceeded, the application should display the message "Credit limit exceeded". Use sentinel-controlled repetition to obtain the data for each account.
|
5.19 |
A large company pays its salespeople on a commission basis. The salespeople receive $200 per week plus 9% of their gross sales for that week. For example, a salesperson who sells $5,000 worth of merchandise in a week receives $200 plus 9% of $5,000, or a total of $650. You have been supplied with a list of the items sold by each salesperson. The values of these items are as follows:
Item Value
1 239.99
2 129.75
3 99.95
4 350.89
Develop a C# application that inputs one salesperson's items sold for the last week, then calculates and displays that salesperson's earnings. There is no limit to the number of items that can be sold by a salesperson.
|
5.20 |
Develop a C# application that will determine the gross pay for each of three employees. The company pays straight time for the first 40 hours worked by each employee and time-and-a-half for all hours worked in excess of 40 hours. You are given a list of the three employees of the company, the number of hours each employee worked last week and the hourly rate of each employee. Your application should input this information for each employee, then should determine and display the employee's gross pay. Use the Console class's ReadLine method to input the data.
|
5.21 |
The process of finding the maximum value (i.e., the largest of a group of values) is used frequently in computer applications. For example, an application that determines the winner of a sales contest would input the number of units sold by each salesperson. The salesperson who sells the most units wins the contest. Write a pseudocode application and then a C# application that inputs a series of 10 integers, then determines and prints the largest integer. Your application should use at least the following three variables:
- counter: A counter to count to 10 (i.e., to keep track of how many numbers have been input and to determine when all 10 numbers have been processed).
- number: The integer most recently input by the user.
- largest: The largest number found so far.
|
5.22 |
Write a C# application that uses looping to print the following table of values:
N 10*N 100*N 1000*N
1 10 100 1000
2 20 200 2000
3 30 300 3000
4 40 400 4000
5 50 500 5000
|
|
5.23 |
Using an approach similar to that for Exercise 5.21, find the two largest values of the 10 values entered. [Note: You may input each number only once.]
|
5.24 |
Modify the application in Fig. 5.12 to validate its inputs. For any input, if the value entered is other than 1 or 2, keep looping until the user enters a correct value.
|
5.25 |
What does the following application print?
1 // Ex. 5.25: Mystery2.cs
2 using System;
3
4 public class Mystery2
5 {
6 public static void Main( string[] args )
7 {
8 int count = 1;
9
10 while ( count <= 10 )
11 {
12 Console.WriteLine( count % 2 == 1 ? "****" : "++++++++" );
13 count++;
14 } // end while
15 } // end Main
16 } // end class Mystery2
|
5.26 |
What does the following application print?
1 // Ex. 5.26: Mystery3.cs
2 using System;
3
4 public class Mystery3
5 {
6 public static void Main( string[] args )
7 {
8 int row = 10;
9 int column;
10 11 while ( row >= 1 ) 12 { 13 column = 1; 14 15 while ( column <= 10 ) 16 { 17 Console.Write( row % 2 == 1 ? "<" : ">" ); 18 column++; 19 } // end while 20 21 row--; 22 Console.WriteLine(); 23 } // end while 24 } // end Main 25 } // end class Mystery3
|
5.27 |
(Dangling-else Problem) Determine the output for each of the given sets of code when x is 9 and y, is 11 and when x is 11 and y is 9. Note that the compiler ignores the indentation in a C# application. Also, the C# compiler always associates an else with the immediately preceding if unless told to do otherwise by the placement of braces ({}). On first glance, you may not be sure which if an else matchesthis situation is referred to as the "dangling-else problem." We have eliminated the indentation from the following code to make the problem more challenging. [Hint: Apply the indentation conventions you have learned.]
-
if ( x < 10 )
if ( y > 10 )
Console.WriteLine( "*****" );
else
Console.WriteLine( "#####" );
Console.WriteLine( "$$$$$" );
-
if ( x < 10 )
{
if ( y > 10 )
Console.WriteLine( "*****" ); }
else
{
Console.WriteLine( "#####" );
Console.WriteLine( "$$$$$" );
}
|
5.28 |
(Another Dangling-else Problem) Modify the given code to produce the output shown in each part of the problem. Use proper indentation techniques. Make no changes other than inserting braces and changing the indentation of the code. The compiler ignores indentation in a C# application. We have eliminated the indentation from the given code to make the problem more challenging. [Note: It is possible that no modification is necessary for some of the parts.]
if ( y == 8 )
if ( x == 5 )
Console.WriteLine( "@@@@@" );
else
Console.WriteLine( "#####" );
Console.WriteLine( "$$$$$" );
Console.WriteLine( "&&&&&" );
- Assuming that x = 5 and y = 8, the following output is produced:
@@@@@
$$$$$
&&&&&
- Assuming that x = 5 and y = 8, the following output is produced:
@@@@@
- Assuming that x = 5 and y = 8, the following output is produced:
@@@@@
&&&&&
- Assuming that x = 5 and y = 7, the following output is produced. [Note: The last three output statements after the else are all part of a block.]
#####
$$$$$
&&&&&
|
5.29 |
Write an application that prompts the user to enter the size of the side of a square, then displays a hollow square of that size made of asterisks. Your application should work for squares of all side lengths between 1 and 20. If the user enters a number less than 1 or greater than 20, your application should display a square of size 1 or 20, respectively.
|
5.30 |
(Palindromes) A palindrome is a sequence of characters that reads the same backward as forward. For example, each of the following five-digit integers is a palindrome: 12321, 55555, 45554 and 11611. Write an application that reads in a five-digit integer and determines whether it is a palindrome. If the number is not five digits long, display an error message and allow the user to enter a new value. [Hint: Use the remainder and division operators to pick off the number's digits one at a time, from right to left.]
|
5.31 |
Write an application that inputs an integer containing only 0s and 1s (i.e., a binary integer) and prints its decimal equivalent. [Hint: Picking the digits of a binary number is similar to picking the digits off a decimal number, which you did in Exercise 5.30. In the decimal number system, the rightmost digit has a positional value of 1 and the next digit to the left has a positional value of 10, then 100, then 1000 and so on. The decimal number 234 can be interpreted as 4 * 1 + 3 * 10 + 2 * 100. In the binary number system, the rightmost digit has a positional value of 1, the next digit to the left has a positional value of 2, then 4, then 8 and so on. The decimal equivalent of binary 1101 is 1 * 1 + 0 * 2 + 1 * 4 + 1 * 8, or 1 + 0 + 4 + 8, or 13.]
|
5.32 |
Write an application that uses only the output statements
Console.Write( "* " );
Console.Write( " " );
Console.WriteLine();
to display the checkerboard pattern that follows. Note that a Console.WriteLine method call with no arguments causes the application to output a single newline character. [Hint: Repetition statements are required.]
* * * * * * * *
* * * * * * * *
* * * * * * * *
* * * * * * * *
* * * * * * * *
* * * * * * * *
* * * * * * * *
* * * * * * * *
|
|
5.33 |
Write an application that keeps displaying in the console window the powers of the integer 2namely, 2, 4, 8, 16, 32, 64 and so on. Loop 40 times. What happens when you run this application?
|
5.34 |
What is wrong with the following statement? Provide the correct statement to add one to the sum of x and y.
Console.WriteLine( ++(x + y) );
|
5.35 |
Write an application that reads three nonzero values entered by the user, then determines and prints whether they could represent the sides of a triangle.
|
5.36 |
Write an application that reads three nonzero integers, then determines and prints whether they could represent the sides of a right triangle.
|
5.37 |
A company wants to transmit data over the telephone, but is concerned that its phones may be tapped. It has asked you to write an application that will encrypt the data so that it may be transmitted more securely. All the data is transmitted as four-digit integers. Your application should read a four-digit integer entered by the user and encrypt it as follows: Replace each digit with the result of adding 7 to the digit and getting the remainder after dividing the new value by 10. Then swap the first digit with the third, and swap the second digit with the fourth. Then print the encrypted integer. Write a separate application that inputs an encrypted four-digit integer and decrypts it to form the original number.
|
5.38 |
The factorial of a non-negative integer n is written as n! (pronounced "n factorial") and is defined as follows:
n! = n · (n 1) · (n 2) · ... · 1 (for values of n greater than or equal to 1)
and
n! = 1 (for n = 0)
For example, 5! = 5 · 4 · 3 · 2 · 1, which is 120.
- Write an application that reads a non-negative integer and computes and prints its factorial.
- Write an application that estimates the value of the mathematical constant e by using the formula
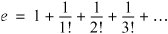
Note that the predefined constant Math.E (class Math is in the System namespace) provides a good approximation of e. Use the WriteLine method to output both your estimated value of e and Math.E for comparison.
- Write an application that computes the value of ex by using the formula
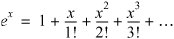
Compare the result of your calculation to the return value of the method call Math.Pow( Math.E, x ). [Note: The predefined method Math.Pow takes two arguments, and raises the first argument to the power of the second. We discuss Math.Pow in Section 6.4.]
|