Introduction
Functions are used to provide modularity to the software. By using functions, you can divide complex tasks into small manageable tasks. The use of functions can also help avoid duplication of work. For example, if you have written the function for calculating the square root, you can use that function in multiple programs.
Program
#include
int add (int x, int y) //A
{
int z; //B
z = x + y;
return (z); //C
}
main ()
{
int i, j, k;
i = 10;
j = 20;
k = add(i, j); //D
printf ("The value of k is%d
", k); //E
}
Explanation
- This function adds two integers and returns their sum.
- When defining the name of the function, its return data type and parameters must also be defined. For example, when you write
int add (int x, int y)
int is the type of data to be returned, add is the name of the function, and x and y are the parameters of the type int. These are called formal parameters.
- The body of a function is just like the body of main. That means you can have variable declarations and executable statements.
- A function should contain statements that return values compatible with the function's return type.
- The variables within the function are called local variables.
- After executing the return statement, no further statements in the function body are executed.
- The name of the function can come from the arguments that are compatible with the formal parameters, as indicated in statement D.
- The arguments that are used in the call of the function are called actual parameters.
- During the call, the value of the actual parameter is copied into the formal parameter and the function body is executed.
- After the return statement, control returns to the next statement which is after the call of the function.
Points to Remember
- A function provides modularity and readability to the software.
- To define the function, you have to define the function name, the return data type and the formal parameters.
- Functions do not require formal parameters.
- If the function does not return any value, then you have to set the return data type as void.
- A call to a function should be compatible with the function definition.
THE CONCEPT OF STACK
Introduction
A stack is memory in which values are stored and retrieved in "last in first out" manner by using operations called push and pop.
Program
Suppose you want to insert values in a stack and retrieve values from the stack. The operations would proceed in the following manner:
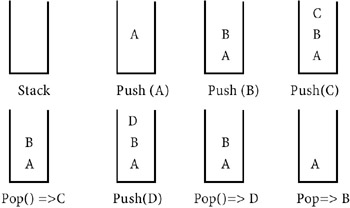
Explanation
- Initially, the stack is empty. When you start push A, A is placed in the stack.
- Similarly, push B and push C put these elements in the stack; the last element pushed is C.
- The pop operation takes the topmost element from the stack. Thus the element C, which was put in last, is retrieved first. This method is called last-in first-out (LIFO).
- The push D operation puts element D in the stack above B.
- Thus push puts the element on the top of the stack and pop takes the element from the top of the stack. The element A which is pushed is the last element taken from the stack.
Point to Remember
The last-in first-out retrieval from the stack is useful for controlling the flow of execution during the function call.
THE SEQUENCE OF EXECUTION DURING A FUNCTION CALL
Introduction
When the function is called, the current execution is temporarily stopped and the control goes to the called function. After the call, the execution resumes from the point at which the execution is stopped.
To get the exact point at which execution is resumed, the address of the next instruction is stored in the stack. When the function call completes, the address at the top of the stack is taken.
Program
main ( )
{
printf ("1
"); // 1
printf ("2
"); // 2
printf ("3
"); // 3
printf ("4
"); // 4
printf ("5
"); // 5
f1 ( );
printf ("6
"); // 6
printf ("7
"); // 7
printf ("8
"); // 8
}
void f1 (void)
{
printf ("f1-9
"); // 9
printf ("f1-10
"); // 10
f2 ( );
printf ("f1-11
"); // 11
printf ("f1-12
"); // 12
}
void f2 (void)
{
printf ("f2-13
"); // 13
printf ("f2-14
"); // 14
printf ("f3-15
"); // 15
}
Explanation
- Statements 1 to 5 are executed and function f1( ) is called.
- The address of the next instruction is pushed into the stack.
- Control goes to function f1( ), which starts executing.
- After the 10th statement, fuction f2 is called and address of the next instruction, 11, is pushed into the stack.
- Execution begins for function f2 and statements 13, 14, and 15 are executed.
- When f2 is finished, the address is popped from the stack. So address 11 is popped.
- Control resumes from statement 11.
- Statements 11 and 12 are executed.
- After finishing the f1 address is popped from the stack, i.e. 6.
- Statements 6, 7, and 8 are executed.
- The execution sequence is 1 2 3 4 5 f1_9 f1_10 f2_13 f2_14 f2_15 f1_11 f1_12 6 7 8.
Points to Remember
- Functions or sub-programs are implemented using a stack.
- When a function is called, the address of the next instruction is pushed into the stack.
- When the function is finished, the address for execution is taken by using the pop operation.
PARAMETER PASSING
Introduction
Information can be passed from one function to another using parameters.
Program
main ( )
{
int i;
i = 0;
printf (" The value of i before call %d
", i);
f1 (i);
printf (" The value of i after call %d
", i);
}
void f1 (int k)
{
k = k + 10;
}
Explanation
- The parameter used for writing the function is called the formal parameter, k in this case.
- The argument used for calling the function is called the actual parameter.
- The actual and formal parameters may have the same name.
- When the function is called, the value of the actual parameter is copied into the formal parameter. Thus k gets the value 0. This method is called parameter passing by value.
- Since only the value of i is passed to the formal parameter k, and k is changed within the function, the changes are done in k and the value of i remains unaffected.
- Thus i will equal 0 after the call; the value of i before and after the function call remains the same.
Points to Remember
- C uses the method of parameter passing by value.
- In parameter passing by value, the value before and after the call remains the same.
CALL BY REFERENCE
Introduction
Suppose you want to pass a parameter under the following conditions:
- You need to change the value of the parameter inside the function.
- You are interested in the changed value after the function completes.
In languages such as Pascal, you have the option of passing the parameter by reference. C, however, does not support this. As explained in the previous example, you cannot have a changed value after the function call because C uses the method of parameter passing by value. Instead, you'll have to implement the function indirectly. This is done by passing the address of the variable and changing the value of the variable through its address.
Program
main ( )
{
int i;
i = 0;
printf (" The value of i before call %d
", i);
f1 (&i); // A
printf (" The value of i after call %d
", i);
}
void (int *k) // B
{
*k = *k + 10; // C
}
Explanation
- This example is similar to the previous example, except that the function is written using a pointer to an integer as a parameter.
- Statement C changes the value at the location specified by *k.
- The function is called by passing the address of i using notation &i.
- When the function is called, the address of i is copied to k, which holds the address of the integer.
- Statement C increments the value at the address specified by k.
- The value at the address of i is changed to 10. It means the value of i is changed.
- The printf statements after the function call prints the value 10, that is, the changed value of i.
Points to Remember
- Call by reference is implemented indirectly by passing the address of the variable.
- In this example, the address of i is passed during the function call. It does not change; only the value of the address is changed by the function.
THE CONCEPT OF GLOBAL VARIABLES
Introduction
The various modules can share information by using global variables.
Program
#include
int i =0; //Global variable
main()
{
int j; // local variable in main
void f1(void) ;
i =0;
printf("value of i in main %d
",i);
f1();
printf("value of i after call%d
",i);
}
void f1(void)
{
int k; // local variable for f1.
i = 50;
}
Explanation
- When you define a variable inside the function block it is called a local variable.
- The local variable can be accessed only in the block in which it is declared.
- j is the local variable for main and it can be accessed only in the block main. That means you cannot access it in function f1.
- k is the local variable for function f1 and it cannot be accessed in main.
- The variable i, which is outside main, is called a global variable. It can be accessed from function main as well as function f1.
- Any expression in this function is going to operate on the same i.
- When you call function f1, which sets the value of i to 50, it is also reflected in main because main and f1 are referring to the same variable, i.
Points to Remember
- Global variables can be accessed in all the functions in that file.
- Any update to the global variable also affects the other functions, because all functions refer to the same value of i.
- When you want to share information between multiple functions, you can use the concept of global variables.
RESOLVING VARIABLE REFERENCES
Introduction
When the same variable is resolved using both local definition and global definition, the local definition is given preference. This is called the rule of inheritance. It says that when you can resolve a reference to the variable by using multiple definitions, the nearest definition is given preference. Since local definition is the nearest, it gets preference.
Program
int i =0; //Global variable /A
main()
{
int i ; // local variable for main / B
void f1(void) ; //C
i =0; // D
printf("value of i in main %d
",i); // E
f1(); // F
printf("value of i after call%d
",i); // G
}
void f1(void) // H
{
int i=0; //local variable for f1 // I
i = 50; // J
}
Explanation
- Here i is declared globally and locally in function main and in function f1, respectively, as given in statements A, B and I.
- Statement D refers to i, which can be resolved by using both local definition and global definition. Local definition is given more preference. So statement D refers to the definition at statement B and all the statements in main refer to the definition at statement B, that is, the local definition.
- When a function is called, statement i = 50 refers to the local definition in that function (definition at statement I).
- Using statement G, the value of i is 0 because both main and function f1 refer to their local copies of i. So the changed value of f1 is not reflected in main.
- Even if you comment local definition of function f1 at statement I the value printed remains the same. This is because main refers to its local copy while f1 refers to the global variable i — the two are different.
Point to Remember
When a variable can be resolved by using multiple references, the local definition is given more preference.
SYNTAX OF FUNCTION DEFINITION
Introduction
When specifying the function, you have to specify the return type, function name, parameter, list, and function body. Within the function body, you can have local definition and return statements.
Program/Example
The general format of a function is
{
executable statements;
Return (expression);
}
For example,
int f1 (int j, float f)
{
int k;
k = l;
return (k);
}
Explanation
- A function returns a value of the type that is specified by the return type. If you don't specify a written type, it is assumed that it returns an int value.
- When the function does not return a value, you have to specify the return data type as void. When the function returns void, you may not write return in the body or you can write the return statement as return; .
- All functions must be named.
- You can specify parameters in the parameter list, separated by commas. While specifying the parameters, you have to specify the parameter data type and parameter name.
- If you don't specify parameters, then you can specify only parentheses as shown here:
int f1( )
- When you want to use variables only for the function then you can declare them just as in main.
- A function returns a value to the caller using the return statement. You may have multiple return statements and the return expression should evaluate to a value that is compatible with the return data type.
- A function returns to the caller after executing the first return statement it encounters during execution.
- A call to a function should match the definition of the function.
- The order of parameters in the call is important because the actual parameter value is copied to the formal parameter value according to the order. It means that the first argument in the call is copied to the first parameter, the second argument is copied to the second parameter, etc.
- When you are using whole numbers as parameters, it is better to declare them by using the data type int. Because all your lower data types' actual parameters can be used for passing the value, your function can be useful for multiple data types.
- When you are using real numbers as parameters, it is better to declare them as double so that the function can be used for both the float and double data types.
Points to Remember
- While specifying the function you have to specify five main functions: written type, function name, parameter, list, function body and return statement.
- Function name and function body are necessary, while the others are optional.
CALLING FUNCTION
Introduction
When a function is written before main it can be called in the body of main. If it is written after main then in the declaration of main you have to write the prototype of the function. The prototype can also be written as a global declaration.
Program
Case 1:
#include
main ( )
{
int i;
void (int *k) // D
i = 0;
printf (" The value of i before call %d
", i);
f1 (&i); // A
printf (" The value of i after call %d
", i);
}
void (int *k) // B
{
*k = *k + 10; // C
}
Case 2:
#include
void (int *k) // B
{
*k = *k + 10; // C
}
main ( )
{
int i;
i = 0;
printf (" The value of i before call %d
", i);
f1 (&i); // A
printf (" The value of i after call %d
", i);
}
Case 3:
#include
void f1(int *k) // B
{
*k = *k + 10; // C
}
.
main ( )
{
int i;
i = 0;
printf ("The value of i before call %d
", i);
f1 (&i); // A
printf ("The value of i after call %d
", i);
}
Explanation
- In Case 1, the function is written after main, so you have to write the prototype definition in main as given in statement D.
- In Case 2, the function is written above the function main, so during the compilation of main the reference of function f1 is resolved. So it is not necessary to write the prototype definition in main.
- In Case 3, the prototype is written as a global declaration. So, during the compilation of main, all the function information is known.