In this section, we are going to build an applied example of using external AS files by building a creature that will follow the mouse around wherever it goes. We will begin with the Creature object class. 1. | Create a new .as file called Creature.as and save it to the desktop.
| 2. | Place this code within it:
//import the EventDispatcher object class import mx.events.EventDispatcher; class Creature extends MovieClip { //create a couple of properties private var xDist:Number; private var yDist:Number; private var creatureSpeed:Number; //create a couple of methods to use in Flash public var addEventListener:Function; public var removeEventListener:Function; //create the method to trigger the event private var dispatchEvent:Function; function Creature() { this.changeColor(); //initialize the EventDispatcher EventDispatcher.initialize(this); //place it at a random spot this._x = Math.random()*550; this._y = Math.random()*400; //set the initial speed this.speed = 10; //where all the magic happens this.onEnterFrame=function(){ xDist = _root._xmouse-this._x; yDist = _root._ymouse-this._y; this._x+=xDist/creatureSpeed; this._y+=yDist/creatureSpeed; if(this.hitTest(_root._xmouse,_root._ymouse)){ dispatchEvent({type:"onGotcha"}); } } } //create the getter setter methods public function get speed():Number{ return this.creatureSpeed; } public function set speed(amount:Number):Void{ this.creatureSpeed = amount; } //create the method to change its color public function changeColor():Void{ //give it a random color var myColor:Color = new Color(this); myColor.setRGB(Math.random()*0x10000000); delete myColor; } } The preceding code may look like a lot, but it really is just all of the things we have covered in this chapter combined. It first imports the Eventdispatcher class so that we can have listeners. Then we declare the class, which is an extension of the MovieClip class. After that, we create a few properties that we use within the class. Then we start to create some of the methods we will use in Flash. Then, of course, is the constructor function where we call a method to change the object's color to a random color. Then we initialize the Eventdispatcher object so that it will work with our Creature object. We then set the object at a random spot in the stage and using the onEnterFrame event, we move the creature around following the mouse. After that, we declare a couple of getter/setter methods to control the speed, and finally create the method to randomly change the color of the creature.
| 3. | Next comes the Flash part. Create a new Flash document and save it also on the desktop as creatureExample.fla.
| 4. | Draw a circle about 75x75 on the stage.
| 5. | Select the entire circle including the stroke and choose Modify, Convert to Symbol.
| 6. | Give it the same options as in Figure 18.6, which are as follows:
- Name creatureMC
- Behavior Movie clip
- Registration center-center
- Identifier creatureMC
- AS 2.0 Class Creature
- Linkage Export for ActionScript
- Linkage Export in first frame
Figure 18.6. Set ActionScript 2.0 classes directly to movie clips in the Symbol Properties dialog box so they automatically have all of the specified class's methods and properties. 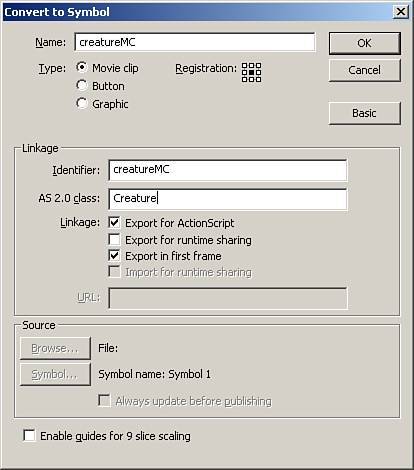
Notice that we are setting the class of this movie clip right in its symbol properties. This means that this symbol will now have all of the characteristics of the Creature object.
| 7. | Give the circle an instance name of myCreature.
| 8. | Create a new layer called action.
| 9. | In the action layer, place this code:
//create the object to listen for the event var creatureListen:Object = new Object(); //create the event creatureListen.onGotcha=function(){ //we will change the color rapidly myCreature.changeColor(); } //add the listener myCreature.addEventListener("onGotcha", creatureListen);
| What the preceding code does is create a new object we will use as a listener for our instance of the Creature object. We then set the event to the listener that, when triggered, will change the color of the creature. Finally, we add the listener to our Creature object. Now, when you test the movie, the circle will follow your mouse around the stage changing color constantly as long as it is touching the mouse. There is another way to set the class of our movie clip without having to do it in the linkage box. Select creatureMC in the Library, right click (Mac: CTRL+click) and select Linkage. Uncheck Export for ActionScript and kit OK. Back in your ActionScript, place this line of code above all of the other code: myCreature.__proto__ = new Creature(); Now test again, and the results will be the same. This extra line of code will reset the class of object our movie clip will think it is. This means that you can reset the object class of the movie clip anytime you want. A good example of using this technique is in a game where a ally might become an enemy, you can simply reset the class the ally was to enemy, and they will act accordingly while still maintaining separate class files. |