Now that you have created the parent form, let's continue by creating a template for the child form. In essence, we will be creating a child form class at design time; later, at runtime, the application will create instances of the child form class as necessary. The child form template will contain a single TextBox control whose properties are set to cause it to completely fill the form. To create the child form template, follow these steps: -
Select Project, Add Windows Form from the Visual Basic .NET menu system. Name the form frmChild before clicking Open. -
Drag a MainMenu control to frmChild, and create the File menu and its submenu items as follows: Name | Text | Shortcut |
---|
mnuFile | File | | mnuFileSave | Save | Ctrl+S | mnuFileSaveAs | Save As... | | mnuFileClose | Close | | mnuFileSep | - (hyphen) | | mnuFilePrint | Print | Ctrl+P | -
Set the MergeType property of mnuFile to MergeItems. This will allow the child's menu items to be combined with those of the parent. -
Drag a TextBox control to the form. Change its Name property to txtMain and clear its Text property. -
Set txtMain's Font property to a monospaced font, such as Courier New. -
So that txtMain will fill up the form no matter how the form is sized, set its MultiLine property to True and its Dock property to Fill. -
Set txtMain's ScrollBars property to Both. This will cause it to automatically display scrollbars if its text takes up more space than is allowed in the control. Also, set its WordWrap property to False so the user can type more text on one line than can be displayed in the control. -
Drag a SaveFileDialog control to the form, and change its Name property to dlgSave. This control will be used to present a Save dialog box to the user to specify a filename. -
Drag a PrintDocument control to the form. We will use this control later in the chapter when we add printing capability to the application. Setting Up the Child Form's Properties We want the child form to provide two properties that can be set and retrieved by the parent form that controls it: TextSaved, which will be a Public Boolean variable reporting whether the text contained in the child form's text box has been saved. A value of True indicates that the text has been saved and has not changed since it was saved. A value of False indicates either that the text has never been saved, or that it has been modified by the user since the last save. FileName, which will will represent the name of the file (and complete path) in which the document represented by this child window is to be saved. If the file has never been saved, the FileName property will contain an empty string (""). Creating the TextSaved Property The TextSaved property is a simple Public variable (of Boolean type) that reports whether the document contained in the child form's text box has been saved (or is in need of saving). Because it is a Public variable, its value can be set or retrieved by the parent form, a need that you will discover a little later. To create the TextSaved property, simply type the following line of code at the bottom of the Code window for frmChild, just above the End Class line: Public TextSaved as Boolean Creating the FileName Property The FileName property is a little more complicated. We will use a Property procedure to handle this property, as a small amount of processing is required when its value is set. The FileName property will be used to expose a Private String variable named sFileName, so begin by typing the following line of code just after the TextSaved declaration you just entered: Private sFileName as String The following Property procedure will handle the FileName property. The Get portion simply returns the current value of sFileName; however, the Set portion performs a secondary function in addition to modifying sFileName it sets the Text property of the current instance of frmChild to the current value of the FileName property, using the InStrRev function to locate and remove the path information. If the FileName property is empty, then the form's Text property is set to Untitled. Enter the following FileName property procedure below the two variable declarations you just entered: Public Property FileName() As String Get Return sFileName End Get Set(ByVal Value As String) sFileName = Value If Trim(sFileName) = "" Then Me.Text = "Untitled" Else Me.Text = Mid(sFileName, InStrRev(sFileName, "\") + 1) End If End Set End Property Save and run the program. Note that you will see the parent form but no instances of the child form, as illustrated in Figure 15.4. Note also (for future reference) that the application's menu system contains only the simple commands we set up for the parent form. Figure 15.4. The parent form shows a simple menu system but no child forms. 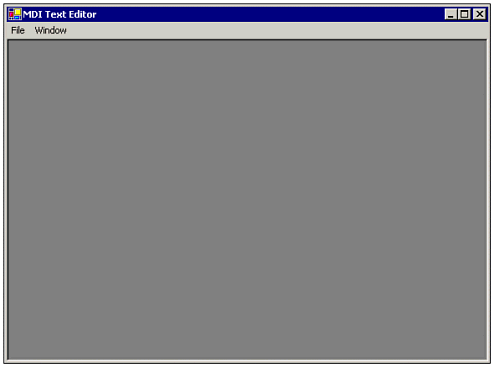 |