The next bit of coding we need to deal with involves creating and displaying a new instance of frmChild, the child form. The user might reasonably expect that when the program is first executed, it opens with a blank document window displayed (as Excel does, for example). In addition, there will be a New command on the File menu to allow the user to open new document windows as needed. Because this functionality (opening and displaying a new document window) needs to be invoked from more than one place in the program, it makes sense to code it as a custom procedure. Creating the NewChild Procedure We will name the custom procedure NewChild, and it is to be coded as a part of the frmParent class. To create the NewChild procedure, right-click frmParent in the Solution Explorer window; then select View Code from the context menu to open frmParent's Code window. Enter the following procedure at the end of the Code window, just above the End Class statement: Private Sub NewChild() Dim f As New frmChild() f.MdiParent = Me f.FileName = "" f.TextSaved = True f.Show() End Sub Here is how the NewChild procedure works: It begins by creating a local object variable named f, which creates a new instance of frmChild. It then sets the MDIParent property of the new instance of frmChild to Me (which represents frmParent because that is the class in which this code resides). This causes f (which represents the new frmChild instance) to be a child form contained by frmParent. Next, the FileName property of the new form instance is set to an empty string, which will trigger the FileName property procedure that we created earlier in frmChild's code (in turn setting the new form's Text property to Untitled); then the new child form's TextSaved property is set to True because an empty document does not need to be saved. Finally, the Show method of the new form is invoked to cause the child form to become visible inside the parent. Displaying a New Child when the Program Starts As you have seen, if you were to run the program at this point, the parent window would be displayed with no child windows inside. As we mentioned, the user probably expects a blank document to be open when the program runs. Because we have already created a NewChild procedure to create a new instance of the child form, we simply need to invoke the NewChild procedure at the appropriate place to cause a new child form to be displayed when the program runs. The proper place to create a new child form when the program runs is in the New procedure of frmParent (the application's startup object). You should already have the Code window for frmParent open. Click the plus sign next to the Windows Form Designer generated code region to display all the code that was generated when the frmParent class was created. Add the following line of code at the end of Public Sub New, just above the End Sub line: NewChild After typing this line of code, click the minus sign next to the Windows Form Designer generated code region to hide the generated code again. Testing the Creation of a New Form Save and run your program. The NewChild procedure executes as part of the startup form's initialization. You should see frmParent open as the container for the application and a single instance of frmChild displayed inside frmParent, as illustrated in Figure 15.5. Note that the title bar for frmChild contains the text Untitled (as defined by the FileName property procedure), and the title bar for frmParent contains MDI Text Editor (the Text property you set at design time). You may also notice that the parent form's menu system contains the commands that we set up for both the parent and child forms because the menus' MergeType properties were set to MergeItems. Maximize the child form and note how it expands to fill the entire parent form. Finally, note that when the child form is maximized, the parent's and child's Text properties are merged into the single caption MDI Text Editor [Untitled], as shown in Figure 15.6. Figure 15.5. A single new instance of the child form is created when the program begins. 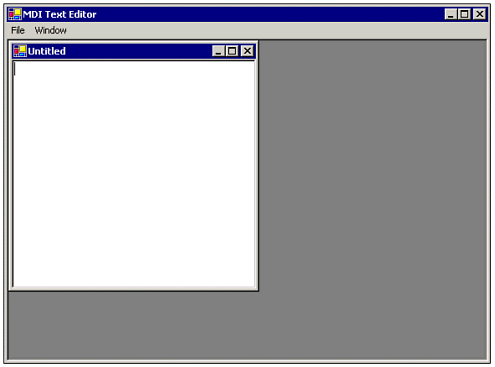 Figure 15.6. Maximizing a child form causes it to fill its parent, and the form's captions merge as well. 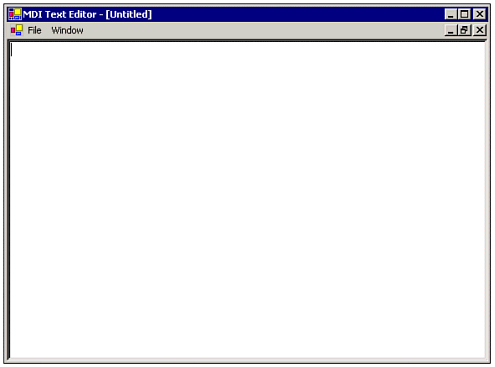 Letting the User Create a New Child Form As we discussed earlier, there are two places where we need to create a new instance of the child form. We have already coded the program to create a new child when the application starts. In addition, the user should be able to create a new child form by selecting File, New from the application's menu system. Coding for this is a simple task. In frmParent's Code window, drop down the Class Name list and select the mnuFileNew menu control; then select Click from the Method Name drop-down. You will see a shell Click event handler for the menu control; simply add a call to the NewChild procedure, as follows: Private Sub mnuFileNew_Click(ByVal sender As Object, _ ByVal e As System.EventArgs) Handles mnuFileNew.Click NewChild() End Sub Save and run the program again. This time you will be able to open multiple child windows by selecting New from the File menu or by pressing the shortcut key for the New menu item (Ctrl+N). |