The final container type that we will cover is the Dialog class. A Frame object or another Dialog always contains a Dialog object. It might be modal, locking out other processes until the dialog is closed, or it might be modeless, allowing it to remain open while the user works in other windows. A Dialog object fires window events when the state of its window changes. The show() method is used to bring the Dialog object to the front and display it. A common use of dialogs is to confirm something. Listing 13.8 shows an example of how this works. Listing 13.8 The TestDialog.java File /* * TestDialog.java * * Created on July 30, 2002, 2:36 PM */ package com.samspublishing.jpp.ch13; import java.awt.*; import java.awt.event.*; /** * * @author Stephen Potts * @version */ public class TestDialog extends Frame implements ActionListener { Button btnExit; Button btnYes; Button btnNo; Dialog dlgConfirm; /** Creates new TestDialog */ public TestDialog() { btnExit = new Button("Exit"); btnExit.addActionListener(this); add(btnExit); this.setLayout(new FlowLayout()); dlgConfirm = new Dialog(this); dlgConfirm.setResizable(false); btnYes = new Button("Yes"); btnYes.addActionListener(this); btnNo = new Button("No"); btnNo.addActionListener(this); dlgConfirm.add(btnYes); dlgConfirm.add(btnNo); dlgConfirm.setTitle("Are you sure?"); dlgConfirm.setSize(200, 100); dlgConfirm.setLayout(new FlowLayout()); addWindowListener(new WinCloser()); setTitle("Using a Dialog"); setBounds( 100, 100, 300, 300); setVisible(true); } public void actionPerformed(ActionEvent ae) { if (ae.getActionCommand().equals("Exit")) dlgConfirm.show(); if (ae.getActionCommand().equals("Yes")) System.exit(0); if (ae.getActionCommand().equals("No")) dlgConfirm.setVisible(false); } public static void main(String[] args) { TestDialog td = new TestDialog(); } } class WinCloser extends WindowAdapter { public void windowClosing(WindowEvent e) { System.exit(0); } } This example introduces several new concepts and foreshadows several others. The TestDialog class implements the ActionListener interface. This interface handles Action events. public class TestDialog extends Frame implements ActionListener Most of the work that is done is in the TestDialog constructor. public TestDialog() { We create an exit button and add a listener class for it. btnExit = new Button("Exit"); btnExit.addActionListener(this); add(btnExit); We set a different layout manager for TestDialog because the default border layout manager would expand the "Exit" button's size until it filled the whole window. this.setLayout(new FlowLayout()); We create a dialog and attach it to this class. dlgConfirm = new Dialog(this); dlgConfirm.setResizable(false); We add buttons for yes and no to the dialog. btnYes = new Button("Yes"); btnYes.addActionListener(this); btnNo = new Button("No"); btnNo.addActionListener(this); dlgConfirm.add(btnYes); dlgConfirm.add(btnNo); We set the title and size of the dialog. dlgConfirm.setTitle("Are you sure?"); dlgConfirm.setSize(200, 100); The default BorderLayout is not appropriate for the dialog because it causes buttons to overlay each other. dlgConfirm.setLayout(new FlowLayout()); The actionPerformed() method is required by the ActionListener interface. It is automatically called when any action event, such as a button click occurs. public void actionPerformed(ActionEvent ae) { if (ae.getActionCommand().equals("Exit")) The show() method makes the dialog appear. dlgConfirm.show(); if (ae.getActionCommand().equals("Yes")) System.exit(0); The setVisible(false) method call causes the dialog to disappear. if (ae.getActionCommand().equals("No")) dlgConfirm.setVisible(false); } When we run this example, the frame appears and shows the Exit button. Clicking this will open the dialog as shown here in Figure 13.8. Figure 13.8. Dialogs can be used to confirm user actions. 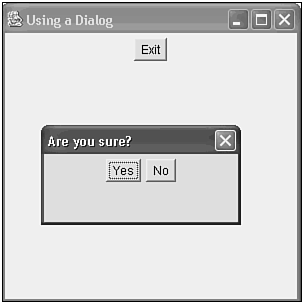 Clicking on the "Yes" button will cause the dialog and the Frame to close. |