The AWT contains another set of graphical objects called components. Components are normally thought of as objects that you place inside of containers. You have been introduced to two types of components in the listings dealings with containers: buttons and text fields. Examples of common components include the following classes: TextField Button Label CheckBox Choice List List Menu Components All these classes extend the java.awt.component class. This class provides a number of methods that are useful to all the graphical components. The methods setLocation(), setForground(), setBackground(), addMouseListener(), and so on, come from this base class. TextField A TextField is a component that contains a single line of text for data entry. It can vary in length, but it can't extend for more than one line. The TextField class, java.awt.TextField, provides the following important methods: addActionListener() The listener class is one that receives notification when an action event occurs. setColumns() Sets the number of columns. setText() Sets or modifies the text. removeActionListener() Removes an action listener. getListeners() Returns an array of all listeners associated with this object. The first example simply places a TextField object in a frame. Listing 13.9 shows the code for the frame. Listing 13.9 The TestTextField.java File /* * TestTextField.java * * Created on July 30, 2002, 11:35 AM */ package com.samspublishing.jpp.ch13; import java.awt.*; import java.awt.event.*; /** * * @author Stephen Potts * @version */ public class TestTextFields extends Frame { TextField tfield1; TextField tfield2; /** Creates new TestTextField */ public TestTextFields() { tfield1 = new TextField(15); tfield2 = new TextField(20); tfield1.setEchoChar('*'); tfield2.setText("Some Sample Text"); tfield2.setFont(new Font("Courier",Font.BOLD,16)); tfield2.setEditable(false); tfield2.select(12,15); Panel p1 = new Panel(); p1.add(tfield1); p1.add(tfield2); add(p1); addWindowListener(new WinCloser()); setTitle("Using TextFields"); setBounds( 100, 100, 300, 300); setVisible(true); } public static void main(String[] args) { TestTextFields tp = new TestTextFields(); } } class WinCloser extends WindowAdapter { public void windowClosing(WindowEvent e) { System.exit(0); } } This example is designed to show some of the methods that the TextField class provides, as well as some of the methods that it inherits from its direct parent, the java.awt.TextComponent class. Text fields can be created with a preset number of columns to show at one time. tfield1 = new TextField(15); tfield2 = new TextField(20); We set an echo character so that sensitive information can't be viewed while it is being typed. tfield1.setEchoChar('*'); You can set the text programmatically to display default values. tfield2.setText("Some Sample Text"); You can also set the font. tfield2.setFont(new Font("Courier",Font.BOLD,16)); To set the field as not editable, you call this method. tfield2.setEditable(false); You can also select substrings of a field programmatically. tfield2.select(12,15); Running this example displays the result shown in Figure 13.9 (after you type a few characters in the first field). Figure 13.9. TextFields can be manipulated manually and programmatically. 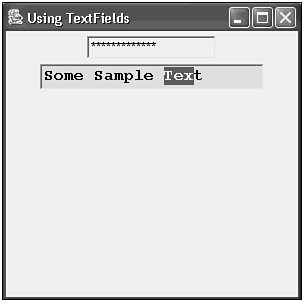 The highlighted portion of the second text field was set by the select() method. Adding Buttons One of the most popular graphical components is the button. The button class, java.awt.Button, class provides the following important methods: addActionListener() The listener class is one that receives notification when the button is clicked. setLabel() Sets the label text for the button. removeActionListener() Removes the association between this button and the listener class. Adding a button to a container is a fairly simple process. Listing 13.10 shows how we would add a button to a Frame. Listing 13.10 The TestButton.java File /* * TestButton.java * * Created on July 30, 2002, 2:36 PM */ package com.samspublishing.jpp.ch13; import java.awt.*; import java.awt.event.*; /** * * @author Stephen Potts * @version */ public class TestButton extends Frame implements ActionListener { Button btnExit; /** Creates new TestDialog */ public TestButton() { btnExit = new Button("Exit"); btnExit.setFont(new Font("Courier", Font.BOLD, 24)); btnExit.setBackground(Color.cyan); Cursor curs = new Cursor(Curson.HAND_CURSOR); btnExit.setCursor(curs); btnExit.addActionListener(this); add(btnExit); this.setLayout(new FlowLayout()); addWindowListener(new WinCloser()); setTitle("Using a Button and an ActionListener"); setBounds( 100, 100, 300, 300); setVisible(true); } public void actionPerformed(ActionEvent ae) { if (ae.getActionCommand().equals("Exit")) System.exit(0); } public static void main(String[] args) { TestButton td = new TestButton(); } } class WinCloser extends WindowAdapter { public void windowClosing(WindowEvent e) { System.exit(0); } } This example shows some of the interesting things that you can do with a button. Buttons that don't do anything when clicked are not of very much value. For that reason, applications that contain buttons always implement the ActionListener interface. This interface specifies that a method called actionPerformed() bepresent. public class TestButton extends Frame implements ActionListener A Button variable is declared. This does not create the Button object, just a variable that can be assigned the address of a Button object. Button btnExit; This is where the actual object is created. The string passed into the constructor is the label that will appear on the button's face. btnExit = new Button("Exit"); You can control the font in which the Button's label will appear. btnExit.setFont(new Font("Courier", Font.BOLD, 24)); You can also set the background color. Here we set it to Color.cyan. btnExit.setBackground(Color.cyan); The Cursor class controls the shape of the cursor when the mouse is over this object. We change it from the default arrow to the hand-shaped cursor called HAND_CURSOR. Cursor curs = new Cursor(Cursor.HAND_CURSOR); The setCursor() command takes a Cursor as a parameter. btnExit.setCursor(curs); The connection between the button that will contain the actionPerformed() method is made here. The keyword this means that the class that we are writing code for will provide this method. btnExit.addActionListener(this); The actionPerformed() method is called whenever the button is clicked. public void actionPerformed(ActionEvent ae) { The getActionCommand() method returns the value of the label that was given to it when it was instantiated. This is one way to determine which button in a class was clicked. Here there is only one button in the application, and it displays the string Exit. All that it does when this is clicked is terminate the application. if (ae.getActionCommand().equals("Exit")) System.exit(0); } The result from running this example is shown in Figure 13.10. Figure 13.10. Buttons trigger action events. 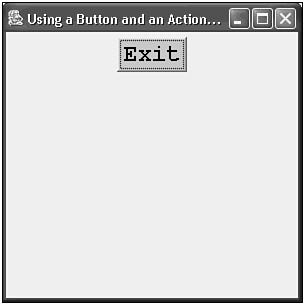 Notice that the color of the background is a cyan (blue), the font is larger and bolded, the cursor changes back and forth to a hand shape and back to a cursor when you move the mouse over the button. TextArea A TextArea object is a rectangular text field that can be longer than one line. It can also be set to read-only. TextAreas can have zero, one, or two scrollbars, which makes them useful for long pieces of text. Several methods can be used to set modify the text in a TextArea. append Adds a String to the end of the text. insert Adds the String at position x in the TextArea and shifts the rest of the text to the right. replaceRange Replaces the text between a start and an end point with a String that gets passed in. We see these methods in action in Listing 13.11. Listing 13.11 The TestTextArea.java File /* * TestTextArea.java * * Created on July 30, 2002, 2:36 PM */ package com.samspublishing.jpp.ch13; import java.awt.*; import java.awt.event.*; /** * * @author Stephen Potts * @version */ public class TestTextArea extends Frame implements ActionListener { Button btnExit; Button btnAppend; Button btnInsert; Button btnReplace; TextArea taLetter; public TestTextArea() { btnAppend = new Button("Append"); btnAppend.addActionListener(this); btnInsert = new Button("Insert"); btnInsert.addActionListener(this); btnReplace = new Button("Replace"); btnReplace.addActionListener(this); btnExit = new Button("Exit"); btnExit.addActionListener(this); taLetter = new TextArea("",10,30, TextArea.SCROLLBARS_VERTICAL_ONLY); taLetter.append("I am writing this letter to inform you that you"); taLetter.append(" have been drafted into the United States Army."); taLetter.append(" You will report to Fort Bragg, North Carolina "); taLetter.append("on July 20, 1966. You will be assigned to "); taLetter.append("Vietnam."); add(btnAppend); add(btnInsert); add(btnReplace); add(btnExit); add(taLetter); this.setLayout(new FlowLayout()); addWindowListener(new WinCloser()); setTitle("Using a TextArea Object"); setBounds( 100, 100, 400, 400); setVisible(true); } public void actionPerformed(ActionEvent ae) { if (ae.getActionCommand().equals("Append")) taLetter.append("\n\nSincerely, \n The Draft Board"); if (ae.getActionCommand().equals("Insert")) taLetter.insert("Dear Steve,\n ", 0); if (ae.getActionCommand().equals("Replace")) taLetter.replaceRange("Dear Jerry,\n ", 0, 12); if (ae.getActionCommand().equals("Exit")) System.exit(0); } public static void main(String[] args) { TestTextArea tta = new TestTextArea(); } } class WinCloser extends WindowAdapter { public void windowClosing(WindowEvent e) { System.exit(0); } } The TextArea object is declared to be empty, with 10 rows, 30 columns, and a vertical scrollbar. taLetter = new TextArea("",10,30, TextArea.SCROLLBARS_VERTICAL_ONLY); The append command is used to create the body of the letter. taLetter.append("I am writing this letter to inform you that you"); taLetter.append(" have been drafted into the United States Army."); taLetter.append(" You will report to Fort Bragg, North Carolina "); taLetter.append("on July 20, 1966. You will be assigned to "); taLetter.append("Vietnam."); Each of the buttons performs a different function. The Append button adds a Sincerely line. if (ae.getActionCommand().equals("Append")) taLetter.append("\n\nSincerely, \n The Draft Board"); The Insert button adds a salutation. if (ae.getActionCommand().equals("Insert")) taLetter.insert("Dear Steve,\n ", 0); The Replace button replaces the salutation with a different name. if (ae.getActionCommand().equals("Replace")) taLetter.replaceRange("Dear Jerry,\n ", 0, 12); The result of running this example is shown in Figure 13.11. Figure 13.11. Buttons can be used to trigger method calls on the TextArea object. 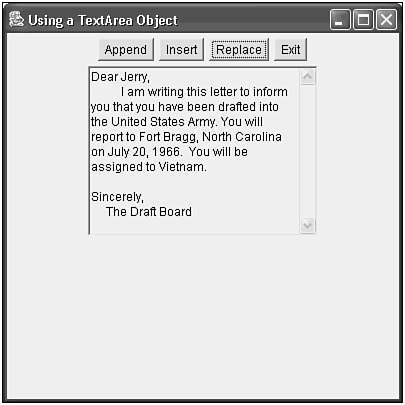 Note how the \n causes new lines to be inserted in the text. You can use this technique to perform some crude formatting. CheckBox A Checkbox is a switch that can either be checked on or off. You can group Checkboxes together into a group where they can act like the buttons on a radio. Clicking one of them will unclick the other ones automatically. Checkboxes are useful as toggle switches and for representing choices where only one of them would make sense, such as a choice of bread, wheat or rye. The listener class that handles events generated by Checkboxes is the ItemListener interface. This interface requires that the itemStateChanged() method be implemented. The JVM will call this method for you whenever an item changes that has a listener registered for it. Listing 13.12 shows how this works. Listing 13.12 The TestCheckboxes.java File /* * TestCheckboxes.java * * Created on July 30, 2002, 2:36 PM */ package com.samspublishing.jpp.ch13; import java.awt.*; import java.awt.event.*; /** * * @author Stephen Potts * @version */ public class TestCheckboxes extends Frame implements ItemListener { Checkbox cbWhiteBread; Checkbox cbWheatBread; Checkbox cbRyeBread; Checkbox cbToasted; TextField tField; public TestCheckboxes() { cbWhiteBread = new Checkbox("White Bread"); cbWhiteBread.setState(false); cbWhiteBread.addItemListener(this); cbWheatBread = new Checkbox("Wheat Bread"); cbWheatBread.setState(false); cbWheatBread.addItemListener(this); cbRyeBread = new Checkbox("Rye Bread"); cbRyeBread.setState(false); cbRyeBread.addItemListener(this); cbToasted= new Checkbox("Toasted"); cbToasted.setState(false); cbToasted.addItemListener(this); tField = new TextField(30); setLayout(new FlowLayout()); add(cbWhiteBread); add(cbWheatBread); add(cbRyeBread); add(cbToasted); add(tField); addWindowListener(new WinCloser()); setTitle("Using Checkboxes"); setBounds( 100, 100, 300, 300); setVisible(true); } public void itemStateChanged(ItemEvent ie) { Checkbox cb = (Checkbox)ie.getItemSelectable(); if( cb.getState()) tField.setText(cb.getLabel()); else tField.setText("Not " + cb.getLabel()); } public static void main(String[] args) { TestCheckboxes tcb = new TestCheckboxes(); } } class WinCloser extends WindowAdapter { public void windowClosing(WindowEvent e) { System.exit(0); } } We implement the ItemListener interface to receive ItemEvents. public class TestCheckboxes extends Frame implements ItemListener Each Checkbox is given a label, set to an unchecked state, and given an ItemListener. cbWhiteBread = new Checkbox("White Bread"); cbWhiteBread.setState(false); cbWhiteBread.addItemListener(this); cbWheatBread = new Checkbox("Wheat Bread"); cbWheatBread.setState(false); cbWheatBread.addItemListener(this); cbRyeBread = new Checkbox("Rye Bread"); cbRyeBread.setState(false); cbRyeBread.addItemListener(this); cbToasted= new Checkbox("Toasted"); cbToasted.setState(false); cbToasted.addItemListener(this); The itemStateChanged() method is called by the JVM whenever a registered Checkbox's state changes from true to false, and vice versa. public void itemStateChanged(ItemEvent ie) { We obtain a handle to the Checkbox that triggered the event. Checkbox cb = (Checkbox)ie.getItemSelectable(); We use that object handle to determine the current state and to get the label so that we can display it. if( cb.getState()) tField.setText(cb.getLabel()); else tField.setText("Not " + cb.getLabel()); } The result of running this example is shown in Figure 13.12. Figure 13.12. Checkboxes are useful for values that are either true or false and on or off. 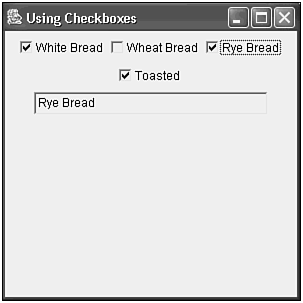 Notice that multiple bread types can be checked at the same time, even though these choices are mutually exclusive. In the following section, you will learn how to constrain them to only one choice. CheckboxGroup The CheckBoxGroup class enables you to limit the number of check boxes that the user can select to one at a time. Checking another will automatically uncheck the others. Listing 13.13 shows a modified version of Listing 13.12 and adds a CheckboxGroup. Listing 13.13 The TestCheckboxGroup.java File /* * TestCheckboxGroups.java * * Created on July 30, 2002, 2:36 PM */ package com.samspublishing.jpp.ch13; import java.awt.*; import java.awt.event.*; /** * * @author Stephen Potts * @version */ public class TestCheckboxGroups extends Frame implements ItemListener { Checkbox cbWhiteBread; Checkbox cbWheatBread; Checkbox cbRyeBread; Checkbox cbToasted; TextField tField; /** Creates new TestCheckboxGroups */ public TestCheckboxGroups() { CheckboxGroup cbgBread = new CheckboxGroup(); cbWhiteBread = new Checkbox("White Bread", cbgBread, false); cbWhiteBread.addItemListener(this); cbWheatBread = new Checkbox("Wheat Bread", cbgBread, false); cbWheatBread.addItemListener(this); cbRyeBread = new Checkbox("Rye Bread", cbgBread, false); cbRyeBread.addItemListener(this); cbToasted= new Checkbox("Toasted"); cbToasted.setState(false); cbToasted.addItemListener(this); tField = new TextField(30); setLayout(new FlowLayout()); add(cbWhiteBread); add(cbWheatBread); add(cbRyeBread); add(cbToasted); add(tField); addWindowListener(new WinCloser()); setTitle("Using Checkboxes"); setBounds( 100, 100, 300, 300); setVisible(true); } public void itemStateChanged(ItemEvent ie) { Checkbox cb = (Checkbox)ie.getItemSelectable(); if( cb.getState()) tField.setText(cb.getLabel()); else tField.setText("Not " + cb.getLabel()); } public static void main(String[] args) { TestCheckboxGroups tcb = new TestCheckboxGroups(); } } class WinCloser extends WindowAdapter { public void windowClosing(WindowEvent e) { System.exit(0); } } The CheckboxGroup is a class that you declare like any other. Here we declared it as a local object in the constructor. CheckboxGroup cbgBread = new CheckboxGroup(); The work of the CheckboxGroup class is all done when the Checkboxes are being instantiated. By adding the name of the group in the Checkbox constructor, you associate the Checkbox with the group. cbWhiteBread = new Checkbox("White Bread", cbgBread, false); cbWhiteBread.addItemListener(this); cbWheatBread = new Checkbox("Wheat Bread", cbgBread, false); cbWheatBread.addItemListener(this); cbRyeBread = new Checkbox("Rye Bread", cbgBread, false); cbRyeBread.addItemListener(this); This has no affect on any Checkboxes that are not associated with the group explicitly. cbToasted= new Checkbox("Toasted"); The cbToasted checkbox functions independently of the others that were added to the group. The result of running this example is shown in Figure 13.13. Figure 13.13. CheckboxGroups are used to associate Checkbox objects. 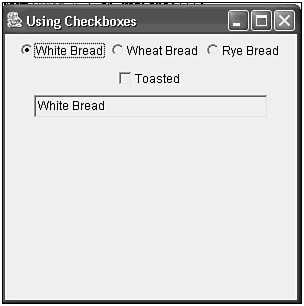 Notice that the appearance of the Checkboxes that were added to the group is now different from the appearance of the lone checkbox that is not a part of the group. Programming with a Choice Control The Choice object is a pull-down menu or list of items. It only shows the list of choices available when it has the focus, but it shows the current choice at all times. The Choice object generates an ItemEvent when a change is made to the object. The getSelectedItem() method provides the information about which item was selected. Listing 13.14 shows an example that contains a Choice object. Listing 13.14 The TestChoices.java File /* * TestChoices.java * * Created on July 30, 2002, 2:36 PM */ package com.samspublishing.jpp.ch13; import java.awt.*; import java.awt.event.*; /** * * @author Stephen Potts * @version */ public class TestChoices extends Frame implements ItemListener { Choice clBread; TextField tField; /** Creates new TestChoices*/ public TestChoices() { clBread = new Choice(); clBread.add("White Bread"); clBread.add("Wheat Bread"); clBread.add("Rye Bread"); clBread.addItemListener(this); tField = new TextField(30); setLayout(new FlowLayout()); add(clBread); add(tField); addWindowListener(new WinCloser()); setTitle("Using Choices"); setBounds( 100, 100, 300, 300); setVisible(true); } public void itemStateChanged(ItemEvent ie) { Choice selBread = (Choice)ie.getItemSelectable(); tField.setText("You selected " + selBread.getSelectedItem()); } public static void main(String[] args) { TestChoices tc = new TestChoices(); } } class WinCloser extends WindowAdapter { public void windowClosing(WindowEvent e) { System.exit(0); } } The first step in using a Choice object is to instantiate the Choice class. clBread = new Choice(); The add() method places the items on the list. clBread.add("White Bread"); clBread.add("Wheat Bread"); clBread.add("Rye Bread"); An ItemListener interface is needed to receive the ItemEvents that are generated by the Choice object. clBread.addItemListener(this); The itemStateChange() method provides the event handling for this object. public void itemStateChanged(ItemEvent ie) { You first get a handle to the Choice object itself. Choice selBread = (Choice)ie.getItemSelectable(); Now you can use that handle to call the getSelectedItem() method. tField.setText("You selected " + selBread.getSelectedItem()); The result of running this example is shown in Figure 13.14. Figure 13.14. Choice objects are useful when you want to allow users to choose one item from a list. 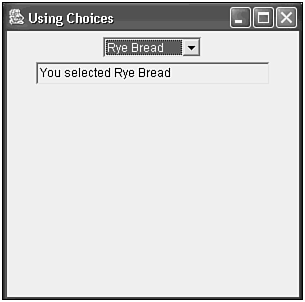 Notice that only one item can be chosen. If you want to allow multiple choices, you can use the List class, the topic of the following section. Programming with a List Control A List object is similar to a Choice object, except that the List allows more than one item to be displayed at a time. It also allows more than one item to be selected at the same time. Listing 13.15 shows an example of this. Listing 13.15 The TextLists.java File /* * TestLists.java * * Created on July 30, 2002, 2:36 PM */ package com.samspublishing.jpp.ch13; import java.awt.*; import java.awt.event.*; /** * * @author Stephen Potts * @version */ public class TestLists extends Frame implements ItemListener { List lstBread; TextField tField; /** Creates new TestLists*/ public TestLists() { lstBread = new List(3, true); lstBread.add("White Bread"); lstBread.add("Wheat Bread"); lstBread.add("Rye Bread"); lstBread.addItemListener(this); tField = new TextField(" ",30); setLayout(new FlowLayout()); add(lstBread); add(tField); addWindowListener(new WinCloser()); setTitle("Using Lists"); setBounds( 100, 100, 300, 300); setVisible(true); } public void itemStateChanged(ItemEvent ie) { List selBread = (List)ie.getItemSelectable(); if (selBread.getSelectedItem()!= null) tField.setText("You selected " + selBread.getSelectedItem()); else { tField.setText(""); for (int i=0; i< selBread.getItemCount() ; i++) { if (selBread.isIndexSelected(i)) { String oldString = tField.getText(); tField.setText( oldString + " " + selBread.getItem(i)); } } } } public static void main(String[] args) { TestLists tc = new TestLists(); } } class WinCloser extends WindowAdapter { public void windowClosing(WindowEvent e) { System.exit(0); } } You instantiate the List with an integer for the number of rows that you want to display, and a Boolean that indicates that you want to allow multiple selections. lstBread = new List(3, true); The add() method places the items on the list lstBread.add("White Bread"); lstBread.add("Wheat Bread"); lstBread.add("Rye Bread"); The List needs an implemented ItemListener interface to handle the events that it generates. lstBread.addItemListener(this); The fact that the List allows multiple selections complicates event handling. public void itemStateChanged(ItemEvent ie) { We first get a handle to the List object. List selBread = (List)ie.getItemSelectable(); If one item is selected, the getSelectedItem() method returns it. Otherwise, it returns null. if (selBread.getSelectedItem()!= null) tField.setText("You selected " + selBread.getSelectedItem()); else { We need to look at each item in the List to see if it is selected. tField.setText(""); for (int i=0; i< selBread.getItemCount() ; i++) { If it is, we need to append it to the String being displayed. Because no append method exists for TextFields, we have to do this manually. if (selBread.isIndexSelected(i)) { String oldString = tField.getText(); tField.setText( oldString + " " + selBread.getItem(i)); } The result of running this is shown in Figure 13.15. Figure 13.15. List objects are useful when you want to enable users to choose more than one item from a list. 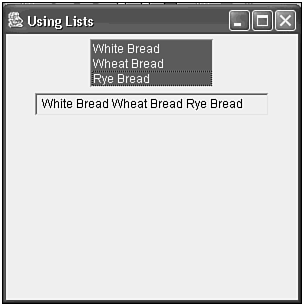 Notice that you are able to choose multiple items in the list without pressing the Ctrl key. MenuBar One of the most useful features of a GUI is its menu system. The menu system is composed of a MenuBar. Only one MenuBar can be attached to a frame. All other menu processing must be initiated via the MenuBar. MenuBars are containers for Menu classes. A Menu is a pull-down list that allows only one selection at a time. Listing 13.16 shows an example with a MenuBar class and three menus. /* * TestMenuBars.java * * Created on July 30, 2002, 2:36 PM */ package com.samspublishing.jpp.ch13; import java.awt.*; import java.awt.event.*; /** * * @author Stephen Potts * @version */ public class TestMenuBars extends Frame { MenuBar mBar; Menu breadMenu, toastMenu; Menu helpMenu; TextField tField; /** Creates new TestMenuBars*/ public TestMenuBars() { breadMenu = new Menu("Bread"); breadMenu.add("White"); breadMenu.add("Wheat"); breadMenu.add("Rye"); toastMenu = new Menu("Toast"); toastMenu.add("Light"); toastMenu.add("Medium"); toastMenu.add("Dark"); mBar = new MenuBar(); mBar.add(breadMenu); mBar.add(toastMenu); helpMenu = new Menu("Help"); helpMenu.add("help"); mBar.setHelpMenu(helpMenu); tField = new TextField(" ",30); setLayout(new FlowLayout()); add(tField); setMenuBar(mBar); addWindowListener(new WinCloser()); setTitle("Using Menu Bars"); setBounds( 100, 100, 300, 300); setVisible(true); } public static void main(String[] args) { TestMenuBars tmb = new TestMenuBars(); } } class WinCloser extends WindowAdapter { public void windowClosing(WindowEvent e) { System.exit(0); } } We will create a menu for the bread, the toast first breadMenu = new Menu("Bread"); breadMenu.add("White"); breadMenu.add("Wheat"); breadMenu.add("Rye"); toastMenu = new Menu("Toast"); toastMenu.add("Light"); toastMenu.add("Medium"); toastMenu.add("Dark"); We instantiate the MenuBar then add the Menu objects to it. mBar = new MenuBar(); mBar.add(breadMenu); mBar.add(toastMenu); We create the Help menu last. helpMenu = new Menu("Help"); helpMenu.add("help"); The Help menu is a special case, so we use the setHelpMenu() method instead of the add() method to attach it to the MenuBar. mBar.setHelpMenu(helpMenu); The setMenuBar() method attaches the MenuBar to the Frame. setMenuBar(mBar); Running this example displays the result shown in Figure 13.16. Figure 13.16. The MenuBar is a container for Menu objects. 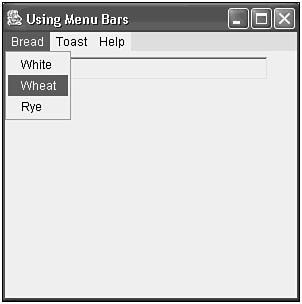 Notice how easy it was to create menus and menu bars. The reason for this is that the AWT provides all the graphics management and behavior needed to display the menus. All that you have to provide is the specification of what you want to see. This is a very good example of object-oriented programming. MenuItem As you probably guessed, a MenuItem class represents an item on a Menu. The primary job of the MenuItem is to generate an ActionEvent when it is chosen. You have the option of enabling and disabling the MenuItem object using the enable() and disable() methods. Listing 13.16 shows an example that uses MenuItems. Listing 13.16 The TestMenuItems.java File /* * TestMenuItems.java * * Created on July 30, 2002, 2:36 PM */ package com.samspublishing.jpp.ch13; import java.awt.*; import java.awt.event.*; /** * * @author Stephen Potts * @version */ public class TestMenuItems extends Frame implements ActionListener { MenuBar mBar; Menu breadMenu, toastMenu; Menu helpMenu; TextField tField; /** Creates new TestMenuItems*/ public TestMenuItems() { breadMenu = new Menu("Bread"); MenuItem tempMenuItem; tempMenuItem = new MenuItem("White"); tempMenuItem.addActionListener(this); breadMenu.add(tempMenuItem); tempMenuItem = new MenuItem("Wheat"); tempMenuItem.addActionListener(this); breadMenu.add(tempMenuItem); tempMenuItem = new MenuItem("Rye"); tempMenuItem.addActionListener(this); breadMenu.add(tempMenuItem); toastMenu = new Menu("Toast"); tempMenuItem = new MenuItem("Light"); tempMenuItem.addActionListener(this); toastMenu.add(tempMenuItem); tempMenuItem = new MenuItem("Medium"); tempMenuItem.addActionListener(this); toastMenu.add(tempMenuItem); tempMenuItem = new MenuItem("Dark"); tempMenuItem.addActionListener(this); toastMenu.add(tempMenuItem); mBar = new MenuBar(); mBar.add(breadMenu); mBar.add(toastMenu); helpMenu = new Menu("Help"); helpMenu.add(new MenuItem("help")); mBar.setHelpMenu(helpMenu); tField = new TextField(" ",30); setLayout(new FlowLayout()); add(tField); setMenuBar(mBar); addWindowListener(new WinCloser()); setTitle("Using Menu Bars"); setBounds( 100, 100, 300, 300); setVisible(true); } public void actionPerformed(ActionEvent ae) { String cmd = ae.getActionCommand(); tField.setText("you selected: " + cmd); } public static void main(String[] args) { TestMenuItems tmi = new TestMenuItems(); } } class WinCloser extends WindowAdapter { public void windowClosing(WindowEvent e) { System.exit(0); } } Adding a MenuItem consists of a declaration, an instantiation, and the addition of an action listener. MenuItem tempMenuItem; tempMenuItem = new MenuItem("White"); tempMenuItem.addActionListener(this); The item is then added to the menu. breadMenu.add(tempMenuItem); The getActionCommand() method can also be used to discover which of the menu items was actually selected by the user. public void actionPerformed(ActionEvent ae) { String cmd = ae.getActionCommand(); tField.setText("you selected: " + cmd); } This provides you with a string that can be tested to determine the action that is appropriate. The result of running this example is shown in Figure 13.17. Figure 13.17. The MenuItems generate events that your program can respond to. Notice how the most recently selected menu item's title is displayed in the TextField on the form. 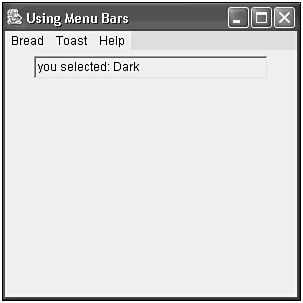 |