In a synchronous method call, the thread that executes the method call waits until the called method finishes execution. Such calls can make the user interface nonresponsive if the client program is waiting for a long process to finish executing on the server. This is especially true for remote method calls where additional time is involved because the calls are made across the network. The .NET Framework has a solution for this in the form of asynchronous methods. An asynchronous method call invokes the method and then returns the control to the calling program immediately, leaving the invoked method to complete its execution.  | For asynchronous method calls, the remote objects need not explicitly support the asynchronous behavior. It is up to the caller to decide whether a particular remote call is asynchronous. |
In the .NET Framework, asynchronous programming is implemented with the help of delegate types. Delegates are types that are capable of storing references to methods of a specific signature. A delegate declared as shown here is capable of invoking methods that take a string parameter and return a string type: delegate string LongProcessDelegate(string param); If there is a method definition, such as the following: string LongProcess(string param) { ... } then the LongProcessDelegate can hold references to this method like this: LongProcessDelegate delLongProcess; delLongProcess = new LongProcessDelegate(LongProcess); After you have the delegate object available, you can call its BeginInvoke() method to call the LongProcess() method asynchronously, such as in the following: IAsyncResult ar = delLongProcess.BeginInvoke("Test", null, null); Here the IAsyncResult interface is used to monitor an asynchronous call and relate the beginning and the end of an asynchronous method call. When you use the BeginInvoke() method, control immediately comes back to the next statement while the LongProcess() method might still be executing. To return the value of an asynchronous method call, you can call the EndInvoke() method on the same delegate, such as in the following example: String result = delLongProcess.EndInvoke(ar); However, it is important to know where to place this method call because when you call the EndInvoke() method, if the LongProcess() method has not yet completed execution, the EndInvoke() method causes the current thread to wait for the completion of LongProcess() method. Poor use of the EndInvoke() method, such as placing it just next to the BeginInvoke() method, can cause an asynchronous method call to result in a synchronous method call. One of the alternatives to this problem is to use the IsCompleted property of the IAsyncResult object to check whether the method has completed the execution, and then to call the EndInvoke() method only in such a case. string result; if(ar.IsCompleted) { result = delLongProcess.EndInvoke(ar); } But regular polling of the IAsyncResult.IsCompleted property requires additional work at the client side. You can also use a WaitHandle object to manage asynchronous remote method calls. When you are ready to wait for the results of the remote method call, you can retrieve a WaitHandle object from IAsyncResult object's AsyncWaitHandle property: ar.AsyncWaitHandle.WaitOne(); String result = delLongProcess.EndInvoke(ar); The WaitOne() method of WaitHandle object causes the thread to pause until the results are ready. The WaitHandle object has other methods that are useful if you have multiple outstanding asynchronous remote method calls. You can wait for all the method calls to come back by using the static WaitHandle.WaitAll() method, or for the first one to return by using the static WaitHandle.WaitAny() method. Therefore, the WaitHandle object essentially lets you turn the asynchronous process back into a synchronous process. In fact, there is a better technique for managing asynchronous method invocation: using callback methods. In this technique, you can register a method that is automatically invoked as soon as the remote method finishes execution. You can then place a call to the EndInvoke() method inside the callback method to collect the result of remote method execution. To implement the callback technique with an asynchronous method invocation, you need to take the following steps in the client program: Define a callback method that you want to execute when the remote method has finished execution. Create an object of delegate type AsyncCallback to store the reference to the method created in Step 1. Create an instance of the remote object on which you want to invoke remote method calls. Declare a delegate type capable of storing references to the remote method. Using the object in Step 3, create a new instance of the delegate declared in Step 4 to refer to the remote method. Call the BeginInvoke() method on the delegate created in Step 5, passing any arguments and the AsyncCallback object. Wait for the server object to call your callback method when the method has completed. 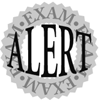 | The Soapsuds tool (soapsuds.exe) available in the .NET Framework SDK enables you to easily distribute the interface of a Web service interface to a large number of clients. Given the URL for a remotable object, the Soapsuds tool can automatically generate an interface assembly for the remote object. All the clients need to know is the URL of the remotable object. However, the Soapsuds tool can be used only with the HTTP channel. If you are using the TCP channel, you still need to depend on manually creating and distributing the interfaces for remotable classes. |
|