If you want to allow a user to select a date, displaying a calendar is the right way to do it. You could fashion your own calendar using an HTML table and a lot of scripting code (many developers have done this, in many different ways), but it's a lot of effort. Fortunately, ASP.NET handles this effort for you. The Calendar control, shown in Figure 22.2 (see the sample page CalendarControl.aspx) displays a neatly formatted, bindable table-like view that provides for almost any "look" you need. (Actually, if you view the source for the page containing the Calendar control in the browser, you'll see that it actually is an HTML table, albeit a somewhat complex one.) Figure 22.2. Use the Calendar control to select a date.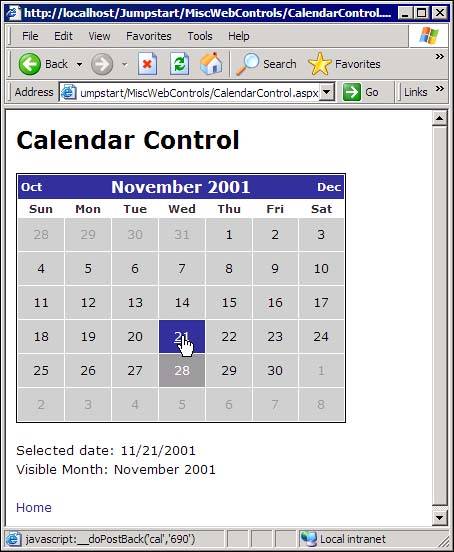 The Calendar control uses client-side script to trigger a postback to the server each time you click any of the dates, or other links, on the calendar. As you can see, in the status bar in Figure 22.2, each date calls a JavaScript procedure that raises an event on the server, thus allowing you to run code each time the user clicks a date. When the user clicks a date, the SelectionChanged event is triggered. In this event procedure, you might retrieve the SelectedDate property of the Calendar control and use that date: Private Sub cal_SelectionChanged( _ ByVal sender As System.Object, _ ByVal e As System.EventArgs) _ Handles cal.SelectionChanged lblDate.Text = _ String.Format("Selected date: {0:d}", _ cal.SelectedDate) End Sub TIP This example uses a format specifier in the String.Format placeholder: {0:d}. The formatting string, d, indicates that the date should be formatted using the short date format of the current locale. For more information on formatting strings, see the online help for the String.Format method. If you click one of the links at the top of the control that moves you to a new month, you trigger the Calendar control's VisibleMonthChanged event. This event procedure receives, in its second parameter, a variable of the MonthChangedEventArgs type. This variable provides two unique properties, NewDate and PreviousDate, that allow you to determine the first day of the month that's currently showing and the first day of the month that was previously showing. The sample page uses the NewDate property to display the current month: Private Sub cal_VisibleMonthChanged( _ ByVal sender As System.Object, _ ByVal e As System.Web.UI.WebControls. _ MonthChangedEventArgs) _ Handles cal.VisibleMonthChanged lblMonth.Text = _ String.Format("Visible Month: {0:MMMM yyyy}", _ e.NewDate) End Sub TIP This example uses a user-defined formatting specification, MMMM yyyy, to display only the month name and the year. Again, see the online help for String.Format for complete information on its formatting capabilities. Initializing the Calendar The Calendar control provides two properties, SelectedDate and TodaysDate, that are closely related. The SelectedDate property (and its cousin, SelectedDates) allows you to specify or retrieve the selected date that is, the date the user has selected. (The SelectedDates property allows you to set or retrieve a group of selected dates.) The TodaysDate property allows you to set or retrieve the date the calendar considers to be the current date, and this property will contain the server's date if you don't specify a date. It's quite possible, and highly probable, that these two properties will contain different values. When you first load a page containing the Calendar control, however, you might like them to both contain the same value. (If you don't specify a value for the SelectedDate property, it contains the date 1/1/0001. If you don't specify a value for the TodaysDate property, it contains the current date on the server.) To avoid any confusion that might occur if you want to allow a user to select the current date without actually clicking anything, you might want to initialize both these properties to the same date as your page loads: Private Sub Page_Load( _ ByVal sender As System.Object, _ ByVal e As System.EventArgs) _ Handles MyBase.Load cal.SelectedDate = cal.TodaysDate End Sub |