At its most basic; Ajax can be used to retrieve a chunk of content from the server. It doesn't pass any information back to the server; it simply retrieves a file, which can then be used by the Web page. This content file can be in virtually any format, as long as when it is inserted into the page the browser can interpret it. In this example (Figure 20.2), we'll simply fetch a small chunk of code (<h1>Hello World</h1>) when the visitor clicks the conspicuous control and then place that code onto the page (Figure 20.3). Figure 20.2. When the page loads, only the control is displayed. 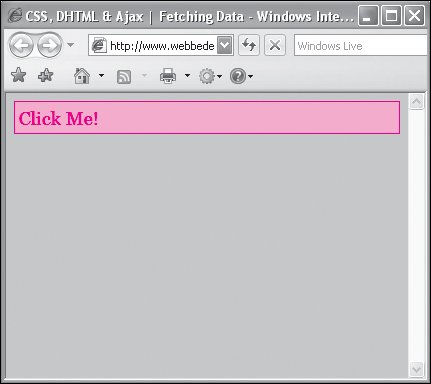 Figure 20.3. Clicking the control causes the browser to fetch the code from the server and place it onto the page. 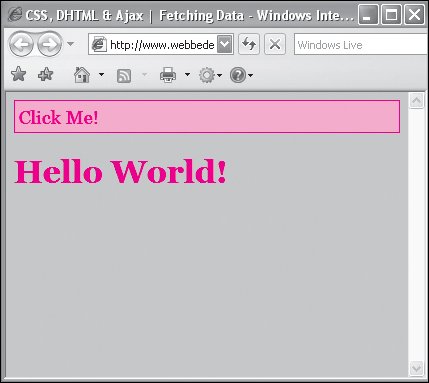 To fetch data from the server: 1. | dataPage.php Create your data page and save it as dataPage.php (Code 20.1).
Code 20.1. dataPage.php: The data page is on the server and contains a simple line of HTML code that can be inserted into any Web page. This file is used to hold the content that is going to be fetched by the Ajax function on our Web page.
For this example, I used the PHP extension, but this is really nothing more than a text file since there is nothing but HTML code in it. This file could be an HTML, ASP, JSP or any other file format that a server can store.
| | | 2. | index.html Create an HTML file and save it as index.html (Code 20.2). The rest of the steps in this example apply to this file.
Code 20.2. index.html: The fetchData() function requests a file from the server based on the URL passed to it and then displays that URL's content directly in the page. [View full width] <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR /xhtml1/DTD/xhtml1-strict.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8" /> <title>CSS, DHTML & Ajax | Fetching Data</title> <style type="text/css" media="screen"> h1 { color: red; } #control { color: red; background-color: #fcc; border: 1px solid red; padding: 4px; } </style> <script type="text/javascript"> function fetchData(url,objectID){ var pageRequest = false; if (window.XMLHttpRequest)pageRequest = new XMLHttpRequest(); else if (window.ActiveXObject) pageRequest = new ActiveXObject("Microsoft.XMLHTTP"); else return false; pageRequest.onreadystatechange = function() { var object = document.getElementById(objectID); object.innerHTML = pageRequest.responseText; } pageRequest.open('GET',url,true); pageRequest.send(null); } </script> </head> <body> <div id="control" onclick="fetchData('dataPage.php', 'message');"> Click Me! </div> <div id="message"> </div> </body> </html> | | 3. | function fetchData(url, objectID){…} Add the function fetchData() to a JavaScript block.
This function will be used to grab whatever code is located at the URL specified by the variable url and place it in the object specified by objectID. Steps 4 through 8 apply to this function.
| | | 4. | var pageRequest = false; Initialize the variable pageRequest and set it to false.
This variable is used as the conduit to exchange information between the Web page (index.html) and the data page (dataPage.php).
| 5. | pageRequest = new XMLHttpRequest(); If the Web page is loaded in a browser that uses the XMLHttpRequest method for making requests, such as Mozilla, Safari, or Internet Explorer 7, add code to initialize the pageRequest variable as an XMLHttpRequest object.
| | | 6. | pageRequest = new ActiveXObject("Microsoft.XMLHTTP"); If the Web page is loaded in a browser that uses the ActiveXObject method for making requests, add code to initialize the pageRequest variable as an ActiveXObject object:
| 7. | pageRequest.onreadystatechange = function() {…} Tell the function what to do when the status of the page request object changes as the data is fetched and loaded into the pageRequest object. In this example, we simply place content returned from the data page (responseText) into an object on our page:
object.innerHTML = pageRequest.responseText; | 8. | pageRequest.open('GET', url, true); Now we add the real action to our function. By initiating the open() method with the pageRequest object, we can get information from the file specified by the url variable, but no data is being sent:
pageRequest.send(null);
| 9. | onclick="fetchData('dataPage.php','message');" Add an event handler to your HTML to trigger the fetchData() function.
In this example, I set up a simple onclick event handler so that the function is triggered when the visitor clicks the control, passing it the URL for the page where the data is located (dataPage.php), as well as the ID for the object that the results are to be placed into (message).
| 10. | <div id="message"> </div> Finally, set up the object that will contain the final results fetched using our Ajax function from Step 3.
| |