Now we are ready to begin the process of designing our sample application, a loan payment calculator. The steps in creating this project are very similar to those used in the previous chapter. First, we will design the user input screen by placing fields on a Web form. Next, we will write Visual Basic code to perform the payment calculation and present the results to the user. Finally, we will run and test the program. Designing the Web Form As you learned in the previous chapter, Visual Basic comes with many predefined objects called controls. Each has a specific purpose and can be used as needed in your applications. The first step in adding a control to your application is displaying the Toolbox window. You do this by choosing Toolbox from the View menu, or clicking the word Toolbox on the left of the screen. Figure 3.4 shows the Toolbox window. Figure 3.4. The controls available for use in Web Applications are in a section of the Toolbox labeled "Web Forms." 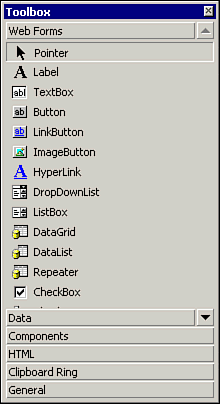 For the loan calculator project, we will be using the following controls: TextBox This control allows the user to input text or numeric values. In this project we will be using four text boxes. Label A label control is used for displaying text. The Loan Calculator will use four label controls to identify the text boxes. LinkButton This control allows the user to initiate a program action. We will use one LinkButton so the user can process the payment calculation. As you may have noticed, the Web Forms controls are very similar to their Windows counterparts. As you work with Web applications, you will find some subtle differences. Adding the First TextBox To add the first TextBox control to the project, draw it on the Web form by following these steps: -
Display the Toolbox window. -
Single-click the TextBox control. -
Position the mouse over a blank area on the Web form designer. The mouse pointer will change to a crosshair. -
Click and hold the mouse button. Drag the mouse to draw a rectangular box. -
Release the mouse button. A TextBox control should now appear on the Web form. Note Freehand drawing and movement of controls on a Web form is only possible in the grid layout mode. If you are using linear layout mode, you need to double-click the control or drag it from the toolbox to the form. Now that we have drawn the TextBox control, we need to set some key properties. As you may recall from Chapter 2, every control has a unique name, which you use to manipulate the control with Visual Basic code. By default, Visual Studio assigns sequentially numbered names such as TextBox1, TextBox2, and so on. However, you should always change the names of your text boxes to something more meaningful. To change the name of the text box you just created, you will need to set its ID property by performing these steps: -
To select the text box, single-click it with the mouse. A series of dots around the border will appear. -
Press the F4 key to display the Properties window. -
Find the ID property in the list, shown in Figure 3.5. Figure 3.5. The ID property of a Web text box corresponds to the Name property of a windows text box. 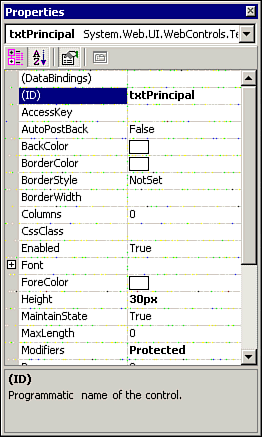 -
Set the value of the ID property to txtPrincipal. This designates the text box for the principal loan amount. Adding the First Label A text box appears as a rectangular box with space for the user to type information. For the user to know the purpose of a text box on the screen, we need to label it. A similar control, the Label control, is typically used to label text boxes. To label the txtPrincipal text box perform the following steps: -
Display the Toolbox window. -
Single-click the Label control. -
Position the mouse over a blank area on the Web form designer. The mouse pointer will change to a crosshair. -
Click and hold the mouse button. Drag the mouse to draw a rectangular box. -
Release the mouse button. The new Label control should now appear on the Web form. Using the mouse, drag the controls around on the screen so that the Label control is positioned to the left of the text box, as shown in Figure 3.6. Figure 3.6. In Visual Studio .NET, the Caption property of a label has been replaced by the Text property. 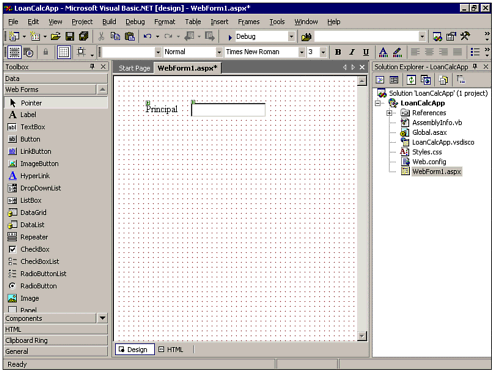 As with the text box, we will need to set the ID property of the label to something more meaningful than the default value. Go ahead and change it to lblPrincipal. Also you need to set the Text property of the label. The Text property determines what the label displays on the screen when the program is running. By default a label displays the word Label. Using the Properties window, change this property to the word Principal. Your screen should look similar to Figure 3.6. Adding the Remaining Labels and Text Boxes To draw the three additional text boxes and labels, you could simply display the Toolbox window and repeat the previous steps. However, it is a little quicker to use the copy and paste method. Copying and pasting controls is just like copying and pasting text in a word processor. First, select the object(s) you want to copy and press Ctrl+C. Then, press Ctrl+V to create a new copy. You will still have to set the individual properties of each new control, but the copy and paste method saves the time of drawing the control and keeps the size of controls consistent. Using the copy and paste method, create three additional Label and TextBox controls. Set the property values as listed in Tables 3.1 and 3.2. After creating all the text boxes and labels, arrange the controls so that your form looks similar to Figure 3.7. Figure 3.7. Arrange the labels to the left of the text boxes, so that each label indicates to the user what information is in the associated text box. 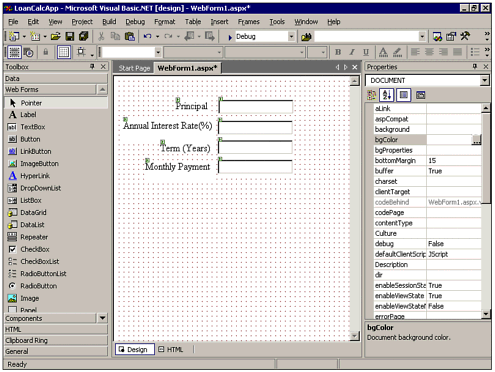 Table 3.1. Additional Label Controls Name (ID Property) | Text (Text Property) |
---|
lblIntRate | Annual Interest Rate (%) | lblTerm | Term (Years) | lblPayment | Monthly Payment | Table 3.2. Additional TextBox Controls Name (ID Property) |
---|
txtIntRate | txtTerm | txtPayment | With the addition of all the text boxes and labels, we have provided a means for the program to accept input from the user (the principal, interest rate, and term) as well as display a result (the monthly payment). Note We could have used a Label control for the monthly payment amount, because it is used for displaying data only. However, it was easier to use a text box for visual consistency. Adding a Link Button In the previous chapter we added a Button control so that the user could tell the program to process the calculation. In the case of the Web, we will use a control called the LinkButton, which works like a regular button but looks like a Web hyperlink. Note There is another control, called the Hyperlink, not to be confused with the LinkButton control, which we will be using to execute VB code on the Web server. To add the link button, perform these steps: -
Display the ToolBox window. -
Single-click the LinkButton control. -
Move the mouse pointer to a blank area of the Web form designer. The mouse pointer will change to a crosshair. -
Hold down the mouse button and draw a LinkButton control. -
Position the LinkButton control so that it is centered below the text boxes and Label control. -
Change the ID property of the link button to cmdCalculate. (The cmd prefix is my personal naming convention for buttons; it stands for command.) -
Change the Text property of the link button to Calculate Payment. Congratulations you have just finished designing your first Web form. Next, you will write the code to make the program work. Writing the Visual Basic Code Now that we have designed the user interface for the Web form, we need to add Visual Basic code to make the program do something useful. The event that will trigger the loan calculation is the user clicking the cmdCalculate link. Therefore, we will add code that executes every time the click event occurs. To display the Code window associated with your Web form, right-click the WebForm1.aspx file in the Solution Explorer and choose View Code. The code editor will display the file WebForm1.aspx.vb, which contains the server-side code associated with your Web form. Note In this chapter the application code resides in a file with a .vb extension on the Web server. There is also a second code component to a Web form that resides on the client and contains the HTML and script code. We will cover this later in the book. Using the leftmost drop-down box at the top of the code editor window, select the cmdCalculate object. Open the rightmost drop-down box and select the Click procedure. You should see the following lines of code added to the window, which represent the beginning and end of the Click procedure. Public Sub cmdCalculate_Click(ByVal sender As Object,ByVal e As System.EventArgs) Handles cmdCalculate.Click End Sub Enter the following code so that the cmdCalculate_Click method appears as shown in Listing 3.1. Listing 3.1 cmdCalculate.zip Loan Payment Calculator Public Sub cmdCalculate_Click(ByVal sender As Object,_ ByVal e As System.EventArgs) Handles cmdCalculate.Click Dim decPrincipal, decIntRate As Decimal Dim intTerm As Integer Dim dblPayment As Double 'Store the principal in the variable cPrincipal decPrincipal = txtPrincipal.Text.ToDecimal 'Convert interest rate to its decimal equivalent ' i.e. 12.75 becomes 0.1275 decIntRate = txtIntRate.Text.ToDecimal / 100 'Convert annual interest rate to monthly ' by dividing by 12 (months in a year) decIntRate = decIntRate / 12 'Convert number of years to number of months ' by multiplying by 12 (months in a year) intTerm = txtTerm.Text.ToInt16 * 12 'Calculate and display the monthly payment. ' The Format function makes the displayed number look good. dblPayment = decPrincipal *_ (decIntrate / (1 - (1 + decIntrate) ^ -intTerm)) txtPayment.Text = Format(dblPayment, "#.00") End Sub Note The loan payment calculator uses the same code as the previous chapter. Please read the section "Writing Program Code" for an explanation of how the code works. Testing Your Web Application Click the start button to compile the program. The Internet Explorer browser should appear and display your Web form, as pictured in Figure 3.8. Enter some sample numeric values in the text boxes and click the link to calculate the loan payment. Note that the Web version of the Loan Calculator works just like its Windows counterpart from Chapter 2. One advantage of the Web version is that you should now be able to go to any other machine on your network even a PC without Visual Studio installed and run the application from your Internet browser. Figure 3.8. Web applications allow you to create applications that can run in a browser, eliminating the need for complex client installations. 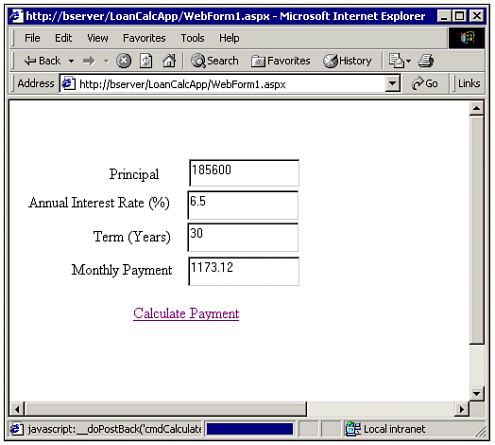 Note If Visual Studio gives you an error about not being able to attach to a debug session, you may need to install the remote debugging feature on the Web server. See the troubleshooting section at the end of this chapter for more details. However, you can still run the Web application manually by opening Internet Explorer and accessing the address http://servername/LoanCalcApp/WebForm1.aspx. Users of previous versions of Visual Basic will especially appreciate the fact that Visual Studio allows you to create a functional Web site using the same techniques you would for any other VB program! As we will see later in the book, many of the other VB features you are familiar with, such as interactive debugging, can be used on the Web. |