Building the Web Store New User Registration page can be accomplished just as easily under the ASP.NET server model as it is with the ASP server model. In fact, there are subtle differences. Most of the differences between ASP.NET and ASP lie in the code, behaviors, and the interfaces that those behaviors implement. Of course, these changes will be pointed out when necessary. If you are specifically interested in ASP.NET and want to put aside the old technology in ASP, this section covers everything you need to know as it relates to Insert, Updates, and Deletes using ADO.NET within ASP.NET. TIP ADO.NET is Microsoft's next-generation technology for accessing data within relational and nonrelational databases. Begin building the new pages by first taking a quick look at the Web Store database. The database reveals that you need to collect personal information and credit card information to process a visitor as a new user. Recall that the tables that make up customers' information lie within the customers and creditcards tables. Upon further inspection of those tables, you'll see that the following fields are necessary for new users to be created within the customers table: Field Name | Date Type | CustomerID | AutoNumber | FirstName | Text | LastName | Text | Username | Text | Password | Text | Email | Text | PhoneNumber | Number | BillingAddress | Text | BillingCity | Text | BillingState | Text | BillingZip | Text | ShippingAddress | Text | ShippingCity | Text | ShippingState | Text | ShippingZip | Text | To associate a credit card with a particular customer, the following information also would need to be collected within the creditcards table. Field Name | Date Type | CustomerID | AutoNumber | CreditCardType | Text | CreditCardExpiration | Text | Knowing the data that needs to reside within the database, you can now begin creating the New User Registration form to accommodate the data that will eventually be inserted. Creating the New User Registration Form Most ASP.NET driven Web applications simulate the fields within a database with Web controls. Because Web controls allow the user interaction with the Web application, they are the perfect channel for collecting information and subsequently inserting that information into the database. Before you can begin creating the New User Registration form, however, you must first create a table that will serve as the primary means for structuring the Web controls in a cohesive and usable fashion. Begin creating the table by following these steps: -
Create a new page from a template. Select New from the File menu, navigate to the Templates tab, and select the WebStore template. WARNING If you have not obtained the chapter files from www.dreamweavermxunleashed.com, you will not have the template available. -
Place your cursor inside the editable region and insert a new form by selecting it from the Insert menu. This guarantees that the table you are about to insert gets placed directly into the form. TIP Now switch to code view and insert the runat="server" into the form. Your ASP.NET page will not function correctly without this attribute. The result should look like this: <form name="form1" runat="server"></form> The method and action attributes are not required. -
Now decide how many rows your table will contain. A quick count of all the necessary fields within the customers and creditcards tables reveals that you need about 16 rows. Place your cursor in the editable region containing the form tag and select Table from the Insert menu. Your values should be structured so that you have 26 rows, 2 columns, and a width of 400 pixels. Everything else should be set to 0. -
Add all the appropriate content for personal information, billing information, and shipping information. You can merge cells along the way to create the headers. The result is shown in Figure 29.32. Figure 29.32. Add all the appropriate content to the new table.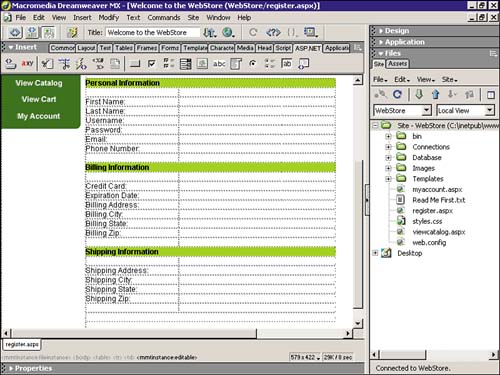 TIP You can apply the "body" class from the Styles panel to make your text a bit more attractive. -
You are now ready to begin adding all the Web controls for the New User Registration form. You can insert all the controls either by selecting them from the Objects menu or by selecting ASP.NET Object from the Insert menu and selecting the appropriate control. The Tag Editor for the specific control enables you to customize all the attributes, including the ID, which is necessary for all Web controls to function properly. The matrix below shows the fields, the appropriate Web controls that should be inserted, and the IDs that should be given to each of the Web controls. Field Name | Web Control | ID | First Name | Text Box | fname | Last Name | Text Box | lname | Username | Text Box | username | Password | Text Box | password | Email | Text Box | email | Phone Number | Text Box | phonenumber | Billing Address | Text Box | billingaddress | Billing City | Text Box | billingcity | Billing State | Text Box | billingstate | Billing Zip | Text Box | billingzip | Shipping Address | Text Box | shippingaddress | Shipping City | Text Box | shippingcity | Shipping State | Text Box | shippingstate | Shipping Zip | Text Box | shippingzip | Credit Card Type | Menu | cctype | Credit Card Expiration | Menu | ccexpiration | Submit Button | btnSubmit | | -
The result of inserting all the Web controls is shown in Figure 29.33. Figure 29.33. Insert all the Web controls for the New User Registration.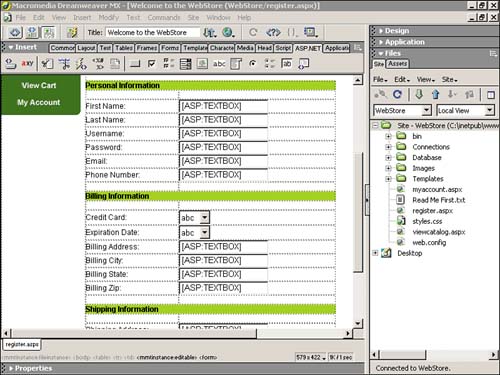 NOTE Ideally you would want the credit card types to come from a separate table within the database called creditcardtypes and dynamically set the drop-down list based on the values within that table. For the sake of simplicity, you can hard-code the values in for the credit card type and expiration date. -
Save the page as "register.aspx" and run it within the browser. The result will look similar to Figure 29.34. Figure 29.34. The New User Registration page allows the user to register for the Web store.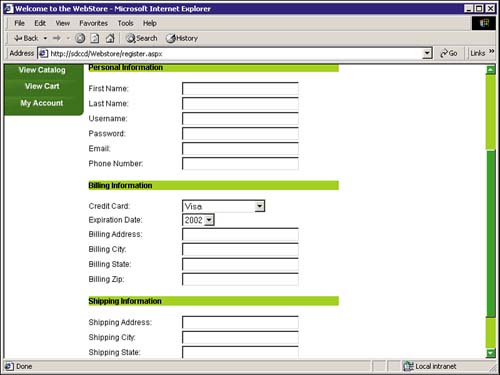 Save the page as "register.aspx" and run it within the browser. The result will look similar to Figure 29.34. Validating the Form with Validation Controls Now that you have the form created, you are ready to begin inserting the data into the database. But before you begin, you want to make sure that you have valid data going in. Validation controls can be used to trap any errors on the client before a request is sent to the ASP application. For instance, because the PhoneNumber field within the customers table accepts only numeric values, you would not want to allow a user to insert anything but numbers into the Phone Number text field. The use of Validation controls could allow you to check for invalid data and return an error message before the data is actually sent. There are numerous types of validation controls, including the following: RequiredFieldValidator The RequiredFieldValidator is used to make a particular control a required entry. CompareValidator The CompareValidator compares the value of a control with the value of another. RangeValidator The RangeValidator checks to make sure that the value of a control falls between a certain range. RegularExpressionValidator The RegularExpressionValidator checks for patterns using regular expressions. ValidationSummary Display a list of errors within one alert box using ValidationSummary. You can insert a RequiredFieldValidator control into your application by following these steps: -
Switch to code view and navigate to the bottom of the page until you find the <asp:button> control. Enter the following attribute to turn validation on for the form: causesvalidation="True". The line of code should result in the following: <asp:button causesvalidation="True" Text="submit" runat="server"></asp: button> -
Switch back to design view and place your cursor next to the First Name text box control. Switch back to code view. -
Select Tag from the Insert Menu. Navigate to ASP.NET Objects, select Validation Server Controls, and highlight RequiredFieldValidator, as shown in Figure 29.35. Choose Insert. Figure 29.35. Insert the RequiredFieldValidator control.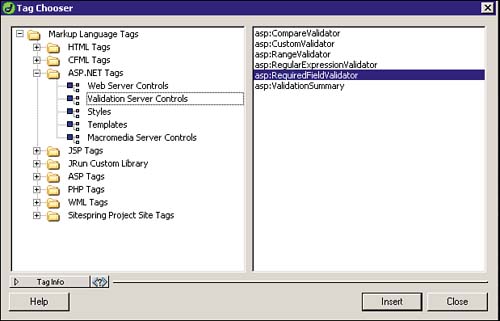 -
Give your validation control an ID, the name of the control to validate, and a custom error message as shown in Figure 29.36. Choose OK. Figure 29.36. Enter all the appropriate information for your validation control.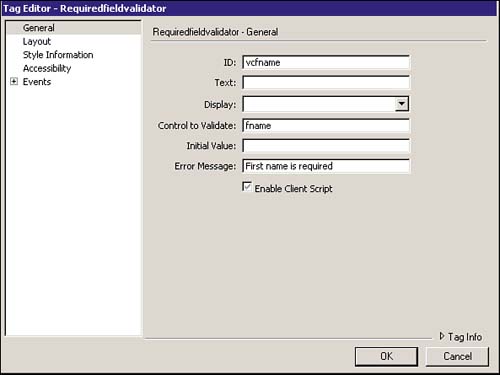 -
Select Close. Your new validation control should now be inserted. -
Save your work and run it in the browser. Not typing a value for First Name will result in an error message, as shown in Figure 29.37. Figure 29.37. Forgetting to type in a value results in an error message.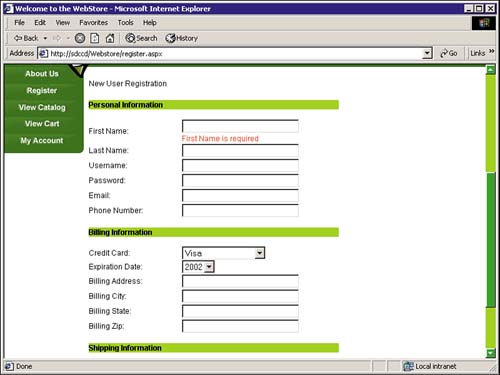 -
Repeat the process until all controls have a RequiredFieldValidator associated with them. Inserting a New User Now that your form is created and all your controls will be validated, you are ready to insert the data into the database. Dreamweaver MX provides a server behavior in the Insert Record that allows for quick and intuitive insertions into the database. To insert the user's record into the database, follow the steps outlined next: -
Select Insert Record from the Server Behaviors panel. -
The Insert Record dialog box appears. The Insert Record dialog box enables you to select a connection, a table, or a view to insert the data into, a page to redirect to after the data has been sent, the form to retrieve the values from, and a columns box allowing you to match up the appropriate Web control with its respective field. For the most part, Dreamweaver MX will find the match unless they are named differently, as is the case with fname and lname. If some do not match, select the appropriate match from the value drop-down list, as shown in Figure 29.38. Figure 29.38. Match up the appropriate Web control with its corresponding field in the database.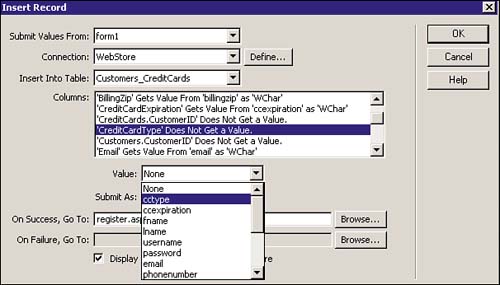 NOTE Unlike with the ASP server model, the Insert Record server behavior within the ASP.NET server model supports insertions into View. Remember the queries that you created and saved in Access that joined multiple tables? These are also known as views. Dreamweaver MX can use these views to insert data into multiple tables. The views appear within the Insert into Table drop-down list at the bottom. Notice that they are the same names as the saved queries within your Access database. -
Select OK. -
Save your work and run it in the browser. After you enter information into the fields and click Submit, it should redirect back to the same page. TIP You may want to create another page called "confirm_newuser.aspx". This page would alert users that their information has been successfully added. After you do that, you can change the redirect value within the Insert Record dialog box to that page. -
Look at the customers and creditcards tables within the database. Your values should appear as they do in Figure 29.39. Figure 29.39. The values from the form should go directly into the database.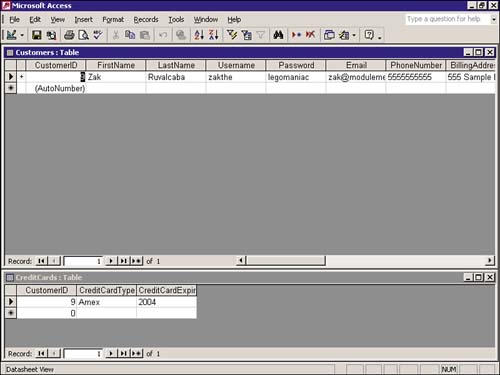 |