Let's take a step back and quickly look at exactly how ASP.NET applications are handled by the CLR. You need to know what the CLR does so that you can anticipate any actions it will take on our code. Intermediate Language When an ASP.NET page is compiled, it's translated into the Microsoft Intermediate Language (MSIL). This is an efficient CPU-neutral set of instructions for controlling applications. Essentially, it's the programming code you wrote in a more compact form. You can't run or execute MSIL itself, but it can be easily converted to machine-native code for actual execution. This MSIL code is stored in a portable executable (PE) file. Execution When your application is ready to be executed, the MSIL is converted to machine-native language by a just-in-time (JIT) compiler that is part of the CLR. Machine-native code is much more efficient to run than uncompiled code. Because MSIL is CPU-independent, the JIT compiler must translate it into the language of the specific machine the user is on. Consequently, there must be many JIT compilers one for each different type of machine.  | When the PE file is compiled to native code, the JIT compiler verifies that it's type-safe. That is, it doesn't allow objects to reference memory locations that they aren't allowed to. In other words, if you create one object of type X, the JIT compiler prevents you from using that object as if it were of type Y. This ensures that each application is isolated and doesn't interfere with other applications, preventing memory corruption and crashes. The JIT compiler only allows type-safe code to pass the verification. You'll see many more examples of this as you develop ASP.NET pages. It's a very common situation. | Processing  | The traditional method used by operating systems to handle applications involves processes. A process runs one application, and it determines the resources available to that application. The process boundaries make sure that applications are isolated from each other if one process (or application) fails, the rest can continue without any hiccups. Figure 2.9 shows a typical list of processes running on Windows 2000. | Figure 2.9. A typical list of processes running on Windows 2000.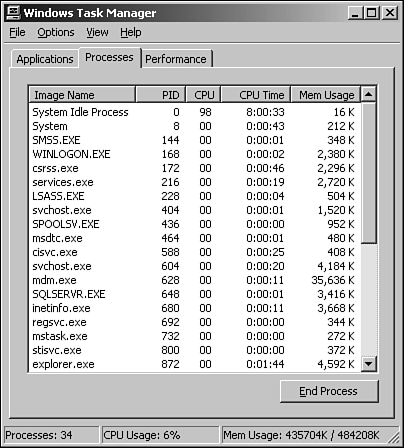 Imagine that an operating system is a beehive. Each bee does its own thing and doesn't interfere with the others. If the bees did interfere with each other, they might crash or bump each other around. Each bee can be considered a process because it runs a honey-collecting "program" and knows where the boundaries of the flowerbed are. If one bee crashes and burns, the rest of the colony can continue without problems.  | This method can still be rather costly in terms of performance and stability, however. The .NET Framework introduces application domains, which are new, smaller units of processing. Because the CLR knows that all of the code it will execute is type-safe, it can spend less time monitoring the processes. A single application can have multiple application domains if needed, which means even better fault-tolerance. | Imagine that beehive again, only now each honey-collecting program uses multiple bees. If one of these bees crashes, wanders off course, or gets eaten by another bug, the honey-collecting program can still go on and the failed bee will soon be replaced by another. This is the way .NET treats its applications. Assemblies  | The assembly is the basic unit of sharing and reusability in the CLR. Assemblies contain other files that represent a common unit, such as ASP.NET pages, PE files, images, or VB.NET source files. A similar object in the traditional Windows framework is a dynamic linked library (DLL). Assemblies, however, allow the .NET Framework to enforce much tighter security and versioning for applications, which in turn allow for much more stable code. The CLR can only execute code that's contained in an assembly. Therefore, even ASP.NET pages are placed in assemblies that are created dynamically when the page is requested. | Side-by-Side Execution The CLR provides the ability to run multiple versions of the same assemblies at once. This is like running multiple versions of a DLL at once in the traditional framework which was impossible but highly sought after. This topic will become important during Week 3 as you develop more sophisticated ASP.NET applications. Note Before you ask, side-by-side execution is not why ASP and ASP.NET pages can run at the same time. Rather, the reason is that each type of page is handled by a different IIS ISAPI filter. ISAPI (Internet Server Application Programming Interface) programs respond when the Web server receives an HTTP request. Filters can be used for any Web server event, such as a Read or Write event. These filters are very useful for Web applications. What Does the CLR Mean for ASP.NET? In terms of ASP.NET development, the CLR gives developers a lot less to worry about. It manages memory much better than the traditional method, and it offers ASP.NET applications much better performance and stability. With better fault isolation, you no longer need to restart IIS or even reboot the server when a Web application crashes. ASP.NET is much more stable and efficient than previous methods of Web development. |