This small application may not seem like much, but it shows you the foundation upon which all other ASP.NET applications will be built. You've learned about the event-driven model, as well as Web controls, which are an integral part of ASP.NET. This application will teach you how ASP.NET and the rest of the .NET Framework interact, which will show you just how powerful this new Framework is. Let's take a look at some more of the technical details, including where these items fall into the .NET framework. These details are important for developing ASP.NET applications and need to be covered early on. More on ASP.NET Compilation You can think of the .NET Framework as a set of blueprints for objects. For example, the System.Web.UI.Page object blueprint tells you the basics of what should be in an ASP.NET page and how it should function. When you build and compile an ASP.NET page, then, it becomes an actual object based on the System.Web.UI.Page blueprint. It contains its own properties and methods, all derived from System.Web.UI.Page. Each ASP.NET page is simply another object based on this blueprint. In this way, each ASP.NET page extends the .NET Framework by providing some custom functionality. Your applications then become a defining part of the framework. You're beginning to see just how powerful the hierarchical framework is and how it can be made much more powerful with your additions. If you find some feature lacking in the original framework, you can simply build it and add it as though it were always there! There's also a blueprint for pure HTML called the System.Web.UI.LiteralControl class. Therefore, HTML is part of the .NET Framework as well. The objects based on the System.Web.UI.LiteralControl blueprint hold all literal values and are rendered to the Web browser as HTML text. This means that the HTML also has properties, methods, and events, just as other objects do. Importing Namespaces Each namespace in the .NET Framework is essentially a collection of blueprints. ASP.NET comes with its own blueprints, but sometimes this set is not enough. Therefore, you must reference other sets from your ASP.NET pages to build different types of objects. You can access these additional objects and methods by using the Import keyword: <%@ Import Namespace="System.Drawing" %> This line imports all of the classes of the System.Drawing namespace, such as the font and image classes. You can now use these blueprints for custom objects. You can also import your own user-defined namespaces. By default, the following namespaces are imported into every ASP.NET page automatically: These namespaces are part of ASP.NET. You don't have to import them explicitly, nor will you ever see the commands used to import them. ASP.NET knows that these namespaces are needed, so they're just there for you to use. Figure 2.8 shows a small sampling of the namespaces available in the .NET Framework. Figure 2.8. A small sampling of the .NET namespaces.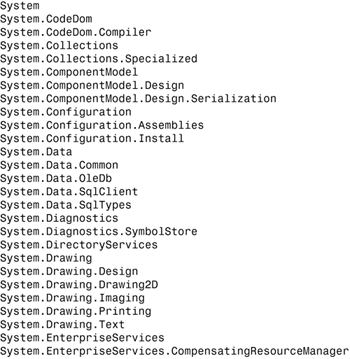 Importing a namespace does not import the namespaces underneath it, however, as you can guess from Figure 2.8. Only classes that belong to that interface are imported. Note You don't need to import a namespace to use its objects; it's just an easier way to do so. For instance, you can use the following in your ASP.NET page: dim objFile as File (Don't worry about the syntax just yet. The namespace portion is the important thing for now.) The File is part of the System.IO namespace. Not importing this namespace wouldn't prevent you from using this object, however. You could use the following instead: dim objFile as System.IO.File In other words, you reference the object by using its entire namespace name. This tells ASP.NET exactly where to look to find this blueprint. Importing namespaces simply allows you to use the shorthand version for your blueprint names. Occasionally, though, if there are two classes with the same name in two different namespaces, you will have to use the full namespace name anyway. For a full listing of all the .NET namespaces and their properties, be sure to check out the extensive .NET Framework documentation that's bundled with the ASP.NET environment you're using. |