With all this interspersed code and all these server controls, it may be difficult for you to figure out what's what in ASP.NET pages, and where to put it. This "spaghetti code" was one of the problems facing traditional ASP developers. ASP.NET tries to simplify this as much as possible. There are two ways to write ASP.NET code: in code declaration blocks or in code render blocks. Thus, you should be able to pick out any ASP.NET code in a page easily. The first method is preferred for a number of reasons: It's compiled (unlike code render blocks), it eliminates the spaghetti code problem, and it's a better way to design your applications. Everything else, then, is HTML. Even the server controls that you used in Listing 2.1 are written in the page as plain HTML. (True, these controls are objects on the server side, but their interface on the browser is described using HTML.) Even the event specifier, as shown on line 17 of Listing 2.3, is HTML. There are also a number of ways to write pure HTML using ASP.NET, such as Response.Write or the shortcut <%= tag. Figure 2.6 shows the general way that you should try to structure your ASP.NET pages, with ASP.NET and HTML code grouped separately. If you use code render blocks in your HTML, it becomes much more difficult to maintain the ASP.NET code because it's all over the map. Figure 2.6. The ideal way to structure ASP.NET pages.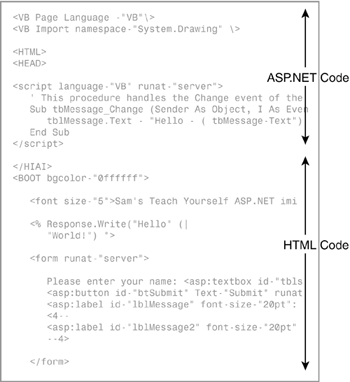 Do | Don't | Do separate your ASP.NET code from your HTML as much as possible by using code declaration blocks. | Don't use code render blocks to intersperse code or HTML output (using Response.Write) when another method may suffice. (And one often will!) | | Commenting Your Code Comments are lines in your code that don't affect its execution. These lines are inserted mainly for the benefit of the developer or anyone else who's trying to understand the code, although they can also be used to stop a piece of code from executing. The end user will never see the commented lines because they aren't sent to the browser. Commenting your code will help you immensely when you develop more complex applications later on. They allow you to put the logic of your code into plain English, which is very helpful if you forget what it does. In ASP.NET, there are three ways to comment your code. First, if you're familiar with HTML, you should know about the HTML commenting marks, <!-- and -->. These can be used anywhere to comment out HTML only. Within all ASP.NET code, you can use the commenting style of the programming language you're using. For example, with Visual Basic.NET, you can use a single quote to comment out one line at a time. Lines 3 and 4 of the following code snippet contain a comment. 1: <script language="VB" runat="server"> 2: sub Page_Load(obj as object, e as eventargs) 3: ' this method executes as soon as the ASP.NET page 4: ' loads 5: some code 6: end sub 7: </script> C# uses two slashes: 1: <script language="C#" runat="server"> 2: function Page_Load(object obj, eventargs e) { 3: // this method executes as soon as the ASP.NET page 4: // loads 5: ... Finally, everywhere but in code declaration blocks, you can use the server-side comment marks <%-- and --%>. Just as with HTML comment marks, these can be used to comment out multiple lines at once. Listing 2.7 shows all three commenting styles in action. Listing 2.7 Various Commenting Styles 1: <% 2: ' this is a Visual Basic comment 3: ' on multiple lines 4: %> 5: <!-- This is an HTML comment 6: on multiple lines --> 7: <%-- This is a server-side comment 8: on multiple lines --%> Caution If you try to use the server-side comment marks (<%-- and --%>) in a code declaration block, you'll receive errors. Code That Spans Multiple Lines With HTML, it's easy to write code that spans multiple lines. For instance, the following two blocks of code are equivalent: <b>Hello World!</b> <b>Hello World!</b> With ASP.NET and Visual Basic.NET, however, it's not so simple. If you try to use the following, you'll receive an error: <% Response.Write ("Hello World") %> This is shown in Figure 2.7. Figure 2.7. An error produced by spanning lines.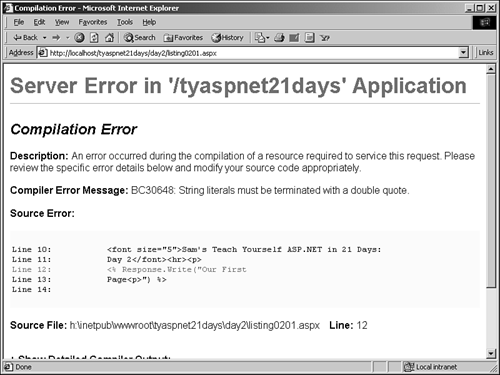 Visual Basic.NET has introduced the line continuation character, denoted by an underscore (_). Let's modify the previous code a bit: <% Response.Write _ ("Hello World") %> This now produces the right output, without any errors. You'll be seeing plenty of these line continuation characters throughout this book! C# isn't limited by line breaks because each complete line in C# must be suffixed by a semicolon (;) C# considers every line without a semicolon to be part of the following line. Thus, the following code snippets will work the same with C#: <% Response.Write ("Hello World"); Response.Write("Hello World"); %> There's another thing to be wary about when you're using line continuation characters they don't work in the middle of a string (even in C#). For example, the following will produce another error: <% Response.Write("Hello _ World") %> ASP.NET will interpret the character as an underscore in the text and will expect some more code. To break up a line containing a string, close the string first and then use the string concatenation character (the ampersand for VB.NET, or the plus symbol for C#) and the line continuation character: <% 'VB.NET Response.Write("Hello" & _ "World") 'C# Response.Write("Hello" + "World"); %> |