There are three major categories of errors that you will deal with in your Visual Basic applications. Compile-Time Errors. Some errors are so blatant that Visual Basic will refuse to compile your application. Generally, such errors are due to simple syntax issues that can be corrected with a few keystrokes. But you can also enable features in your program that will increase the number of errors recognized by the compiler. For instance, if you set Option Strict to On in your application or source code files, implicit narrowing conversions will generate compile-time errors. ' ----- Assume: Option Strict On Dim bigData As Long = 5& Dim smallData As Integer ' ----- The next line will not compile. smallData = bigData Visual Studio 2005 includes new features that help you locate and resolve compile-time errors. Such errors are marked with a "blue squiggle" below the offending syntax. Some errors also prompt Visual Studio to display corrective options through a pop-up window, as shown in Figure 9-1. Figure 9-1. Error correction options for a narrowing conversion Run-Time Errors. Run-time errors occur when a combination of data and code causes an invalid condition in what otherwise appears to be valid code. Such errors frequently occur when a user enters incorrect data into the application, but your own code can also generate run-time errors. Adequate checking of all incoming data will greatly reduce this class of errors. Consider the following block of code: Public Function GetNumber() As Integer ' ----- Prompt the user for a number. ' Return zero if the user clicks Cancel. Dim useAmount As String ' ----- InputBox returns a string with whatever ' the user types in. useAmount = InputBox("Enter number.") If (IsNumeric(useAmount) = True) Then ' ----- Convert to an integer and return it. Return CInt(useAmount) Else ' ----- Invalid data. Return zero. Return 0 End If End Function This code looks pretty reasonable, and in most cases, it is. It prompts the user for a number, converts valid numbers to integer format, and returns the result. The IsNumeric function will weed out any invalid non-numeric entries. Calling this function will, in fact, return valid integers for entered numeric values, and zero for invalid entries. But what happens when a fascist dictator tries to use this code? As history has shown, a fascist dictator will enter a value such as "342304923940234." Because it's a valid number, it will pass the IsNumeric test with flying colors, but since it exceeds the size of the Integer data type, it will generate the dreaded run-time error shown in Figure 9-2. Figure 9-2. An error message only a fascist dictator could love 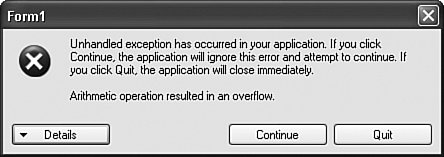 Without additional error-handling code or checks for valid data limits, the GetNumber routine generates this run-time error, and then causes the entire program to abort. Between committing war crimes and entering invalid numeric values, there seems to be no end to the evil that fascist dictators will do. Logic Errors. Logic errors are the third, and the most insidious, type of error. They are caused by you, the programmer; you can't blame the user on this one. From process-flow issues to incorrect calculations, logic errors are the bane of software development, and result in more required debugging time than the other two types of errors combined. Logic errors are too personal and too varied to directly address in this book. Many logic errors can be forced out of your code by adding sufficient checks for invalid data, and by adequately testing your application under a variety of conditions and circumstances. You won't have that much difficulty dealing with compile-time errors. A general understanding of Visual Basic and .NET programming concepts, and a regular use of the tools included with Visual Studio 2005, will help you quickly locate and eliminate them. The bigger issue is: What do you do with run-time errors? Even if you check all possible data and external resource conditions, it's impossible to prevent all run-time errors. You never know when a network connection will suddenly go down, or the user will trip over the printer cable, or a scratch on a DVD will generate data corruption. Any time you deal with resources that exist outside of your source code, you are taking a chance that run-time errors will occur. Figure 9-2 showed you what Visual Basic does when it encounters a run-time error: It displays to the user a generic error dialog, and offers a chance to ignore the error (possible corruption of any unsaved data) or exit the program immediately (complete loss of any unsaved data). Although both of these user actions leave much to the imagination, they don't instill consumer confidence in your coding skills. Trust me on this: The user will blame you for any errors generated by your application, even if the true problem was far removed from your code. Fortunately, Visual Basic includes three tools to help you deal completely with run-time errors, if and when they occur. These three Visual Basic featuresunstructured error handling, structured error handling, and unhandled error handlingcan all be used in any Visual Basic application to protect the user's dataand the userfrom unwanted errors. |