Okay, we've covered most of the basic GDI+ features used to draw images. It's all now just a matter of issuing the drawing commands for shapes, images, and text on a graphics surface. Most of the time, you'll stick with the methods included on the Graphics object, all 12 bazillion of them. Perhaps I over-counted, but there are quite a few. Here's just a sampling. Clear method. Clear the background with a specific color. CopyFromScreen method. If the "Prnt Scrn" button on your keyboard falls off, this is the method for you. DrawArc method. Draw a portion of an arc along the edge of an ellipse. Zero degrees starts at three o'clock. Positive arc sweep values move in a clockwise direction; use negative sweep values to move counterclockwise. DrawBezier and DrawBeziers methods. Draw one Bezier spline, or a continuing curve made up of multiple connected splines. DrawCurve, DrawClosedCurve, and FillClosedCurve methods. Draw "cardinal" curves, with an optional brush fill. DrawEllipse and FillEllipse methods. Draw an ellipse or a circle (which is a variation of an ellipse). DrawIcon, DrawIconUnstretched, DrawImage, DrawImageUnscaled, and DrawImageUnscaledAndClipped methods. Different ways of drawing images and icons. DrawLine and DrawLines methods. Draw one or more lines with lots of options for making the lines snazzy. DrawPath and FillPath methods. I'll discuss "graphic paths" a little later. DrawPie and FillPie methods. Draw a pie-slice border along the edge of an ellipse. DrawPolygon and FillPolygon methods. Draw a regular or irregular geometric shape based on a set of points. DrawRectangle, DrawRectangles, FillRectangle, and FillRectangles methods. Draw squares and rectangles. DrawString method. We used this before to output text to the canvas. FillRegion method. I'll discuss regions later in the chapter. Here's some sample drawing code. ' ----- Line from (10, 10) to (40, 40). e.Graphics.DrawLine(Pens.Black, 10, 10, 40, 40) ' ----- 90degree clockwise arc for 40-pixel diameter circle. e.Graphics.DrawArc(Pens.Black, 50, 10, 40, 40, 0, -90) ' ----- Filled 40x40 rectangle with a dashed line. e.Graphics.FillRectangle(Brushes.Honeydew, 120, 10, 40, 40) Dim dashedPen As New Pen(Color.Black, 2) dashedPen.DashStyle = Drawing2D.DashStyle.Dash e.Graphics.DrawRectangle(dashedPen, 120, 10, 40, 40) dashedPen.Dispose() ' ----- A slice of elliptical pie. e.Graphics.FillPie(Brushes.BurlyWood, 180, 10, 80, 40, _ 180, 120) And so on. You get the idea. Figure 17-9 shows the output for this code. Figure 17-9. Some simple drawings 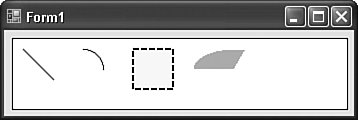 |