Visual Studio .NET provides many tools you can use to debug your applications. In addition, Visual Basic .NET provides tools you can use, such as the Stop statement, the Debug and Trace objects, and conditional compilation. Table 9.1 provides a brief definition of each of these items. Table 9.1. Tools You Can Use to Debug Your Application Tool | Description | Breakpoints | Breakpoints allow you to drop into Break mode before a particular line of code executes. Once in Break mode, you can single-step through your code. You can set as many breakpoints as you like, and your breakpoints are preserved with your project. | Debug class | This class provides methods that allow you to interactively debug your application at development time. You can output text to any of a number of listeners as you're debugging your application. The default listener is the Output window, although you can add other listeners as well, such as the Event Log, text files, or the Console window. | Conditional statements | These statements allow you to include or exclude statements at compile time. Excluded statements simply don't exist within your compiled applications. | Debugging tools | Visual Studio .NET provides a host of windows that give you all sorts of information about variables, breakpoints, memory, the call stack, and much more. | Stop statement | A Stop statement acts as a permanent runtime breakpoint. Unlike breakpoints, however, you cannot leave Stop statements in your compiled applications, because your applications will simply stop when the code execution reaches the Stop statement. Stop statements are included mostly for backward compatibility because breakpoints are now persisted with your projects. (In VB6, developers lost their breakpoints when they shut down the development environment, so developers often used Stop statements to preserve their breakpoints.) | Trace class | This class provides runtime tracing support, allowing you to write text to a number of listeners as your application runs on client machines. You can send information to any number of different listeners, such as the Event Log, text files, or the Console window. (This topic is beyond the scope of this chapter. For more information, see the .NET Framework documentation.) | The following subsections introduce you to these various tools. Using Breakpoints Often, as you're testing an application on your own machine, you won't want to step through every line of code (using the Step Into or Step Over techniques) in order to arrive at the point where you think an error might be lurking. Instead, you can set a breakpoint in your source code to indicate to Visual Studio .NET where it should stop executing and drop into Break mode. To insert a breakpoint, you can simply click the mouse in the left margin, next to the line of code on which you'd like to drop into Break mode. Figure 9.2 shows a procedure including a breakpoint. Figure 9.2. Click in the margin to insert a breakpoint into your code.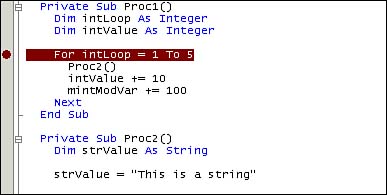 After a breakpoint is set, you can start and run the application normally. When Visual Basic hits the line of code marked with the breakpoint, it automatically puts you in Break mode. Breakpoints are saved with your project, so after you close Visual Studio .NET and restart it, your breakpoints will still be available to you. The Breakpoints Window To make it easier for you to see all your breakpoints, Visual Studio .NET provides the Breakpoints window. Select Debug, Windows, Breakpoints to see the window shown in Figure 9.3. Figure 9.3. The Breakpoints window shows all the breakpoints in your solution.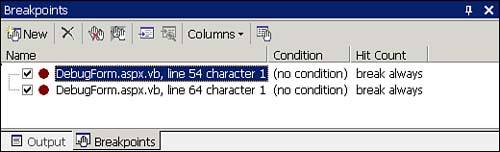 This window provides a list of all the breakpoints you have set in your application. If you double-click a breakpoint, the window will take you directly to that breakpoint within your source code. Breakpoints Toolbar The Breakpoints window contains a toolbar that provides a series of buttons you'll find useful while debugging. Table 9.2 lists and describes the buttons, from left to right, for you. Table 9.2. The Breakpoints Window Toolbar Includes These Buttons Tool | Description | New | Creates a new breakpoint and allows you to configure the conditions under which the program will stop on this breakpoint. See the next section for more information on setting up conditional breakpoints. | Delete | Deletes the currently selected breakpoint. | Clear All Breakpoints | Deletes all breakpoints from the current solution. | Disable All Breakpoints | Disables all breakpoints until you enable them again. | Go To Source Code | Moves your cursor to the line in your source code where the currently selected breakpoint is located. | Go To Disassembly | Opens the disassembly window to the line where the currently selected breakpoint is located. | Columns | Allows you to choose which columns you wish to display in the Breakpoint window. You can display Name, Condition, Hit Count, Language, Function, File, Address, Data, and Program. | Properties | Allows you to configure the conditions under which the program will stop on the currently selected breakpoint. See the next section for information on setting conditional breakpoints. | Conditions on Breakpoints If you right-click any breakpoint in the Breakpoints window and select Properties from the context menu (or select the Properties button on the window's toolbar), you can set and retrieve attributes about the selected breakpoint. If you want to create a new breakpoint, setting conditional attributes, you can right-click any breakpoint and select New Breakpoint from the context menu or click New on the window's toolbar. (The New Breakpoint dialog box is essentially identical to the Breakpoint Properties dialog box.) Figure 9.4 shows the New Breakpoint dialog box. Figure 9.4. The New Breakpoint dialog box shows you information about a breakpoint. A similar dialog box allows you to create a new breakpoint.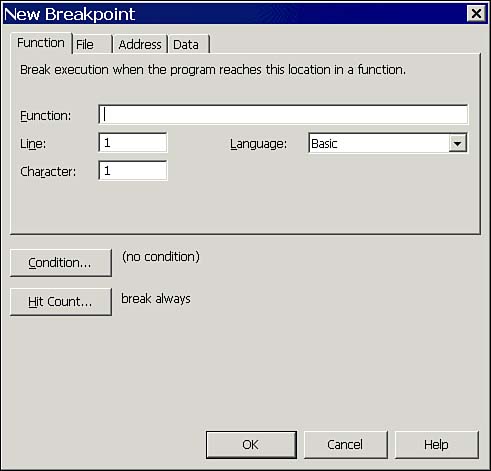 The Function tab (shown in Figure 9.4) allows you to determine in which function you want to create the breakpoint. You can specify the line number and even the character number where you want the breakpoint. (Allowing you to set the character number takes into account Visual Basic's ability to have multiple statements on the same line, separated with a colon [:] character.) The File tab allows you to set a breakpoint within a specific file in your application by line number and character. Figure 9.5 shows the New Breakpoint dialog box with the File tab selected. Figure 9.5. The File tab of the New Breakpoint dialog box allows you to set the file and line number at which you wish to set a breakpoint.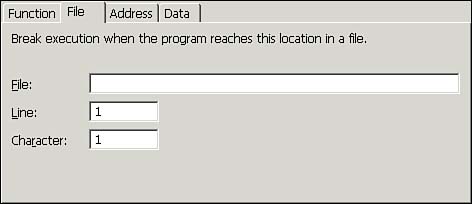 The Address tab of the New Breakpoint dialog box lets you set the actual address of the instruction where you wish to set the breakpoint. You're unlikely to use this option, shown in Figure 9.6, from within Visual Basic .NET. Figure 9.6. The Address tab of the New Breakpoint dialog box lets you set the instruction address at which to break.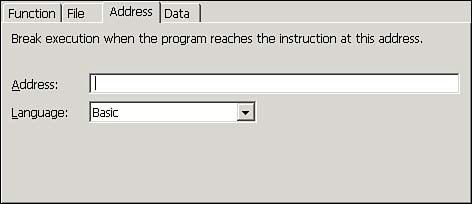 The Data tab of the New Breakpoint dialog box allows you to enter Break mode when a specified variable changes its value. This can be very useful for tracking down where in your program a global variable changes its value. Figure 9.7 shows the Data tab. Figure 9.7. The Data tab of the New Breakpoint dialog box allows you to break when a specified variable changes its value.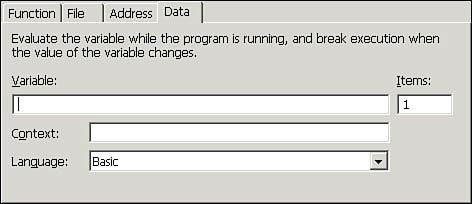 Setting a Condition Sometimes, you'll want to trigger a breakpoint only if some specific condition is met. You can click the Condition button on the New Breakpoint dialog box (or the same button on the Breakpoint Properties dialog box) to enter a condition. Clicking the button displays the dialog box shown in Figure 9.8. Figure 9.8. The Breakpoint Condition dialog box allows you to specify a condition, such as intNumber = 5 or boolValue = True.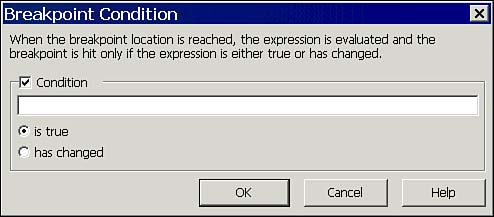 In the Breakpoint Condition dialog box, you can enter expressions and force the program to drop into Break mode either when your expression is true or when the expression changes its value. For example, you might specify an expression such as intNumber = 10 or boolValue = True. If you want to track a variable's value changing, you can enter the variable's name and select Has Changed. Anytime the value of that variable changes, Visual Studio .NET will place you into Break mode. Specifying a Hit Count Sometimes you may not want to step through a loop 50 times just to find an error on the 50th iteration through the loop. To avoid this drudgery, you can select Hit Count on the New Breakpoint dialog box and enter the hit count on which you'd like to drop into Break mode. When you click this button, you will see the dialog box shown in Figure 9.9. Figure 9.9. The Breakpoint Hit Count dialog box lets you control exactly when you enter Break mode.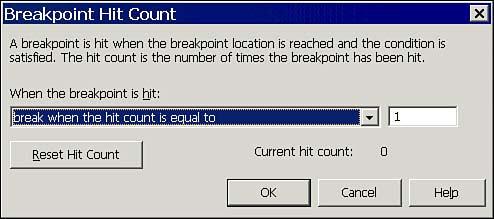 The Breakpoint Hit Count dialog box supplies four options you can select from the drop-down list on the dialog box. Table 9.3 outlines each of these options. Table 9.3. Choose One of These Options to Determine How and When You Drop into Break Mode Option | Description | Break always | Like a standard breakpoint, selecting this option will stop execution each time the breakpoint is reached. | Break when the hit count is equal to | Selecting this option indicates that when the breakpoint has been the number of times you've specified, you'll drop into Break mode. | Break when the hit count is a multiple of | Selecting this option indicates that when the breakpoint has been hit a number of times that is an even multiple of the value you've specified, you'll drop into Break mode. For example, if you specify 5, you'll drop into Break mode the 5th, 10th, 15th, and so on, times you hit the breakpoint. | Break when the hit count is greater than or equal to | Selecting this option indicates that when the breakpoint has been hit at least the number of times you've specified, you'll drop into Break mode each time you hit the breakpoint after that. For example, if you specify 5, you'll drop into Break mode on the 5th, 6th, 7th, and so on, times you hit the breakpoint. | Setting the Next Statement to Execute Sometimes when debugging your application in Break mode, you might need to skip a section of code or back up and execute the same code again. Visual Studio .NET allows this, as long as the line you want to move to is within the current procedure. While in Break mode, simply click and drag the yellow arrow within the left margin to a new location within the same procedure to set the instruction pointer to the newly selected line of code. Figure 9.10 shows the action of dragging the yellow arrow to select a new line of code to execute. Figure 9.10. Click and drag the yellow arrow to set the new instruction pointer.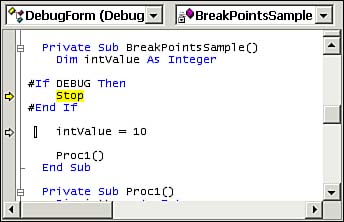 |