Variables, in short, are containers used to temporarily store values. These values can be numbers, text, or much more complex arrangements. Variables exist at the heart of any programming language, and comprehending them is key to using PHP. According to the PHP manual, there are eight types of variables in the language. These include four scalar (single-valued) typesBoolean (trUE or FALSE), integer, floating point (decimals), and strings (text); two nonscalar (multivalued)arrays and objects; plus resources (which you'll see when interacting with databases) and NULL (which is a special type that has no value). Regardless of what type you are creating, all variables in PHP follow certain syntactical rules: A variable's namealso called its identifiermust start with a dollar sign ($), for example, $name. The variable's name can contain a combination of strings, numbers, and the underscore, for example, $my_report1. The first character after the dollar sign must be either a letter or an underscore (it cannot be a number). Variable names in PHP are case-sensitive. This is a very important fact. It means that $name and $Name are entirely different variables. Variables can be assigned values using the equals sign (=), also called the assignment operator. This is just a cursory introduction to variables. You'll learn more about specific types (strings and numbers) as well as manipulating them later in this chapter. To begin working with variables, I'll make use of several predefined variables whose values are automatically set when a PHP script is run. To print out PHP's predefined variables 1. | Create a new HTML document in your text editor (Script 1.6).
<!DOCTYPE html PUBLIC "-//W3C// DTD XHTML 1.0 Transitional//EN "http://www.w3.org/TR/xhtml1/DTD/ xhtml1-transitional.dtd> <html xmlns="http://www.w3.org/1999/ xhtml xml:lang="en" lang="en"> <head> <meta http-equiv="content-type" content="text/html; charset=iso-8859-1 /> <title>Predefined Variables</ title> </head> <body> </body> </html> Script 1.6. This script prints three of PHP's predefined variables. 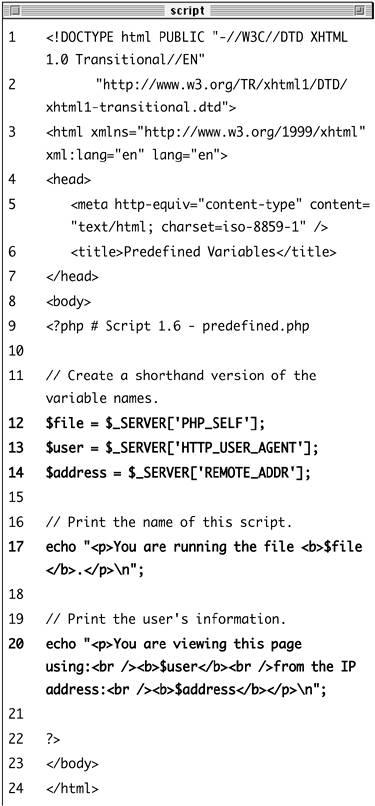
| 2. | Within the body tags, add your PHP tags and your first comment.
<?php # Script 1.6 - predefined.php ?> From here on out, my scripts will no longer comment on the creator, creation date, and so forth, although you should continue to do so. I will, however, make a comment listing the script number and filename for ease of cross-referencing (both in the book and when you download them from the book's supporting Web site, www.DMCInsights.com/phpmysql2).
| 3. | Create a shorthand version of the variables to be used in this script.
$file = $_SERVER['PHP_SELF']; $user = $_SERVER['HTTP_USER_AGENT']; $address = $_SERVER['REMOTE_ADDR']; This script will use three variables, each of which comes from the larger and predefined $_SERVER variable. $_SERVER refers to a mass of server-related information, like the name of the script being run ($_SERVER['PHP_SELF']), the Web browser and operating system of the user accessing the script ($_SERVER['HTTP_USER_AGENT']), and the IP address of the user accessing the script ($_SERVER['REMOTE_ADDR']).
Creating new variables with shorter names and then assigning them values from $_SERVER will make it easier to refer to the variables when printing them. (It also gets around some other issues you'll learn about in due time.)
| 4. | Print out the name of the script being run.
echo "<p>You are running the file <b>$file</b>.</p>\n; The first variable I'll use is $file, which has the same value as $_SERVER['PHP_SELF']. Again, this particular variable always refers to the current script being executed. Depending upon the server, this could be anything from just scriptname.php to /path/to/scriptname.php. Notice that this variable must be printed out within double quotation marks and that I also make use of the PHP newline (\n), which will add a line break in the generated HTML source. Some basic HTML tagsparagraph and boldare added to give the generated page some flair.
| 5. | Print out the information of the user accessing the script.
echo "<p>You are viewing this page using:<br /><b>$user</b><br />from the IP address:<br /><b>$address</ b></p>\n; Here I've used two more variables. To repeat what I said in the third step, $user correlates to $_SERVER['HTTP_USER_AGENT'] and refers to the operating system, browser type, and browser version being used to access the Web page. The second variable$addressmatches up with $_SERVER['REMOTE_ADDR'] and refers to the IP address of the user accessing the page.
Again, PHP newlines are used, and I've also thrown in some HTML breaks so that the resulting Web page has extra lines to it.
| 6. | Save your file as predefined.php, upload to your Web server, and test in your Web browser (Figure 1.13).
Figure 1.13. The predefined.php script reports back to the viewer information about the script and the Web browser being used to view it. 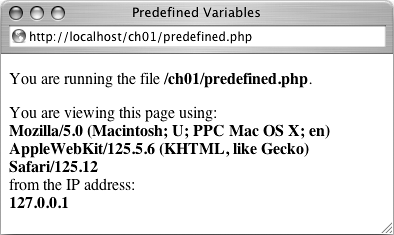
| Tips If possible, run this script using a different Web browser (Figure 1.14). Figure 1.14. This is the book's first truly dynamic script, in that the Web page changes depending upon the person viewing it (compare with Figure 1.13). 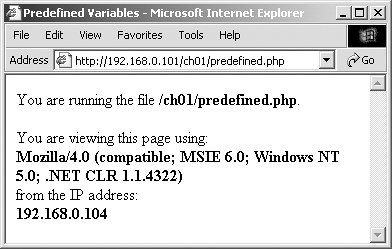
The most important consideration when creating variables is to use a consistent naming scheme. In this book you'll see that I use all lowercase letters for my variable names, with underscores separating words ($first_name). Some programmers prefer to use capitalization instead: $FirstName. PHP is very casual in how it treats variables, meaning that you don't need to initialize them (set an immediate value) or declare them (set a specific type), and you can convert a variable among the many types without problem.
|