At this point, you're getting down to the last few steps that you need to take to complete this application. One of the last tasks for you to complete is to send information out of Flash using the forms you created in Lesson 8. That's the subject of the next exercise. After that, you'll create a simple progress bar to let users know that Tech Bookstore website is loading when they first come to the page. First things first, though, and that's submitting data. In Flash 8 Basic, there are several ways to send information out of Flash, all of which require some level of ActionScript. You can use the LoadVars object to send information out of Flash (and you will in this exercise), getURL, XML, and for more complex applications, Flash Remoting. Which method you choose depends on the application you are building, but all of them have one thing in common: There must be some technology on a web server somewhere that is waiting for the data you're sending out, and what's more, understands the data and what to do with it. This back-end work is really outside of the scope of this book because it requires an understanding of technologies that are much different from Flash. If you're a designer, it might make sense to team up with a developer who can help you develop the back-end technology to receive and process data for the projects you'll be working on in real life. Sending Data Out of the Feedback Form In the next exercise, you'll create a LoadVars object that will collect information from your form and then send it to a server. The server already understands what to do with the information, but that's really not our concern at this point. What we care about is getting the information out. You will be submitting information to your e-mail address using a script pre-created on an actual web server. You should still be working with bookstore14.fla in this lesson. 1. | Select Frame 50 of the feedback layer.
You will place the ActionScript that collects and send the data out of Flash inside each movie clip Timeline. Frame 50 is where your mcQuestionForm and mcFeedbackForm movie clips are located.
| 2. | Double-click mcFeedbackFrom. Add a hidden TextInput instance on the Stage to hold a value for the e-mail address that you will send the data to.
You are double-clicking mcFeedbackForm on the Stage to enter the movie clip's Timeline, in which you will place ActionScript and a hidden form element. You'll need to make sure that the feedback layer in the main document Timeline is unlocked so that you can edit the mcFeedbackForm movie clip.
Drag an instance of the TextInput component onto the Stage in the form layer. Using the Property inspector, set the text parameter to the e-mail address that you want to e-mail the feedback to, such as you@yourdomain.com. Enter your own e-mail address (or an account you can actually check) here.
Open the Component inspector panel by choosing Window > Component Inspector, and select the parameters tab. Set the visible parameter to false to hide the instance on the Stage. The visible parameter is not available on the Property inspector, which is why you are using the Component inspector panel to change its value. Assign an instance name of tiEmailTo to the TextInput instance using the Property inspector.
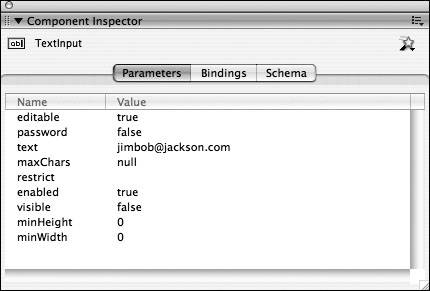 | 3. | Add the following ActionScript into the Actions panel on Frame 1 of the actions layer in the mcFeedbackForm movie clip Timeline.
Select Frame 1 of the actions layer, still inside the mcFeedbackForm Timeline, and type in the following code into the Actions panel. This ActionScript is used to send data entered into the form to an e-mail address. An explanation of what this code does follows the listing.
//Sends the information out of Flash bSend.onRelease = function() { var targetLoadVars:LoadVars = new LoadVars(); var myLoadVars:LoadVars = new LoadVars(); myLoadVars.emailFrom = tiEmail.text; myLoadVars.emailTo = tiEmailTo.text; myLoadVars.subject = tiSubject.text; myLoadVars.message = taMessage.text; myLoadVars.sendAndLoad ("http://www.flash-mx.com/ws/ ¬ submit_feedback.cfm", targetLoadVars, "POST"); targetLoadVars.onLoad = function() { trace(this.success); }; gotoAndStop("thankyou"); }; The script you just typed might look a little intimidating, but it really isn't so bad once you know what it means. Ultimately, you're gathering information, storing it in sort of a programming version of an expand-a-file folder, and sending it out. You start with adding a comment, for reasons you've already learned. Commenting is your friend, so don't forget about it.
The next step is to add an onRelease event handler for your send button. This handler is called when a user clicks on the bSend Button instance. Then you create two LoadVars objects to send the data out. The first LoadVars object is used to hold the variables that the server-side script will return after it is done executing. The second LoadVars object holds all the variables that are sent to the server-side script.
After the LoadVars objects are created, the code copies the four text field values (tiEmail, tiEmailTo, tiSubject, and taMessage) into the myLoadVars variable. When the myLoadVars object is sent to a URL, all these variables are included. Then a server-side script can be used to process the variables. A server-side script is code written using a language such as PHP, ColdFusion, or ASP. The script interacts with web pages and is used to perform a particular task. The script sits on a server and can be used to load data, interact with a database, and perform other similar tasksdepending on what the code is written to do.
The following line of code is where Flash posts the values within the myLoadVars object to your server-side script:
myLoadVars.sendAndLoad("http://www.flash-mx.com/ws/ submit_feedback.cfm", ¬ targetLoadVars, "POST"); The values in the LoadVars object are sent to http://www.flash-mx.com/ws/submit_feedback.cfm. Any results sent from the server-side script will be saved into the targetLoadVars object. The final parameter in the sendAndLoad() function is POST. POST tells Flash how to send the data to the server-side script. When sending the LoadVars object using the POST method, all fields are sent to the server-side script as form variables. Form variables are variables that are sent in the HTTP header, which is not visible to the visitor. This is suitable for long sets of variables, and more importantly, is necessary, because that's how the script is expecting data to come.
Note The other option for the method is GET instead of POST. The GET method sends the values to your server-side script as URL variables along the query string. The query string, which is the part of the URL in the browser's address bar after the question mark, is suitable for sending short variables. For example, in the URL http://www.TrainingFromTheSource.com/index.cfm?name=jdehaan, the query string is name=jdehaan. The final section of ActionScript from the code block you typed in is used for debugging in the testing environment only. The trace statement appears in the Output panel when the data has been sent from Flash to the server-side script. This lets you know that Flash has sent the data when you are working in the testing environment. The trace statements are used only for development and testing purposes, and should be removed before you publish the file and upload it to a server for a website (sometimes known as the production environment).
Finally, you see the following code:
targetLoadVars.onLoad = function() { trace(this.success); }; This code is triggered when Flash receives a reply from the server-side script, which sometimes takes a short period of time before it's executed as Flash waits for a reply. Currently, the server returns a string value of success or failure, which will be displayed in the Output panel (again, for testing purposes). You will be able to test this form after you have the buttons and menus working for the Tech Bookstore at the end of this lesson. In the real world, you would redirect a user to the "thank you" page only if the data were successfully submitted and generated error messages for the user if it were not. In this application, you don't provide feedback for the end user if there is an error. The success page, however, is targeted in the line: gotoAndStop("thankyou"); which directs the playhead to the thankyou frame label.
| 4. | Save the changes you made to bookstore14.fla.
Save your file and test it by selecting Control > Test Movie. Go to the feedback section of the site and fill out the feedback form. Press the send button, and if you specified your own e-mail address in Step 2, you should see an e-mail in your inbox sent from Flash.
Note If your back button for some reason does not work, go to the "thankyou" frame, select it, and open the Actions panel. You added an ActionScript using Script Assist in Lesson 8. If you are in Script Assist mode, toggle it off, and change line 2 to read this._parent.gotoAndStop("form"), which tells the button to control the playhead in the movie clip Timeline a little bit more specifically. This refers to the button; parent refers to the movie clip Timeline. If everything works, save the file and leave it open. You will send data out of the Questionnaire form next.
| Sending Data Out of the Questionnaire Form In this exercise, you will use LoadVars to send values to a server, similar to the way you send information out of the feedback form you completed in the previous exercise. The questionnaire is more complex than the feedback form because you are not only dealing with text fields this time. The form has a NumericStepper component, List component, TextArea component, and two CheckBox components for which you have to get the values. In this exercise, you will use ActionScript to send the variables to a server-side script, which then processes the results so they can be computed later. 1. | In bookstore14.fla, make sure you are in Frame 50 of the main document Timeline. Double-click mcQuestionForm to open it in symbol-editing mode. Enter instance names for the component instances that are on the Stage.
Open bookstore14.fla from the TechBookstore folder on your hard drive that you worked on earlier in this lesson, if it is not already open. Make sure you are in Frame 50 of the feedback layer. Double-click mcQuestionForm to open the instance in symbol-editing mode. Lock the actions layer to prevent accidentally adding any symbols to that layer.
You first need to give instance names to the components that are on the Stage. Give the NumericStepper an instance name of nsNumYears, and the ComboBox an instance name of cbNavigation. Assign an instance name of taBooks to the TextArea component. Then assign the Designer CheckBox an instance name of chDesigner and the Developer CheckBox instance an instance name of chDeveloper. Finally, give the Send button an instance name of bSend if it is not already named.
| 2. | Add ActionScript code to Frame 1 of the actions layer using the Actions panel.
Select Frame 1 of the actions layer in the mcQuestionForm instance and open or expand the Actions panel (F9). Type in the following code:
//sends questionnaire data out and to the server bSend.onRelease = function(){ var targetLoadVars:LoadVars = new LoadVars(); var myLoadVars:LoadVars = new LoadVars(); myLoadVars.surveyExperience = nsNumYears.value; myLoadVars.surveyNavigation = cbNavigation.selectedItem.label; myLoadVars.surveyBooks = taBooks.text; myLoadVars.surveyDesigner = chDesigner.selected; myLoadVars.surveyDeveloper = chDeveloper.selected; myLoadVars.sendAndLoad("http://www.flash-mx.com/ws/submit_survey.cfm", ¬ targetLoadVars, "POST"); trace("send"); targetLoadVars.onLoad = function() { trace(this.success); }; gotoAndStop("thankyou"); }; This code is similar to the previous exercise, in which you used LoadVars to send the feedback to the server-side script. There are a couple of major differences between the two scripts. Because the questionnaire uses several different component types instead of the TextInput and TextArea, grabbing the values from the component and adding them to the LoadVars object is slightly more complicated.
Each component is different, so they each have a different way to access the value of the component. For example, the TextArea and TextInput components store the current value in text, whereas the CheckBox component doesn't have a value, but instead has a Boolean (Yes/No) parameter called selected and denotes whether the component is checked or not. You access the current value of the NumericStepper component by accessing its value parameter.
The List component uses the parameter selectedItem, which is actually an object within itself and has two values: data and labels. You defined the labels and data values earlier in Lesson 8 after you dragged the List component onto the Stage. The value for labels is simply the label for the currently selected item, and the value for data is the value you defined in the Property inspector or Component inspector panel for the currently selected instance.
So how do you know all this stuff? The hardest part of ActionScript is knowing what does what, and learning how to manipulate information with prebuilt things such as components and movie clips and buttons. Aside from the volumes and references written about ActionScript 2.0, you have documentation right in Flash that explains what does what. The Help menu can be pulled up with the keyboard shortcut F1, or you can go to Help > Flash Help. There are gobs of entries about components all by themselves, where you can look up and learn more about how they work. Don't forget about help. It's a real lifesaver.
Just like the feedback form, you also have trace statements in the code to notify you when the request was sent to the server, and when the server responds, it will return success. These two trace statements can be safely removed when you are convinced that the application is working properly.
| 3. | Save the changes to the FLA file.
After you have finished, choose File > Save to save your modifications to the file. Test your file with Control > Test movie and fill out the questionnaire. When you click Send, the values from each of the components are stored in a LoadVars object and are sent to a ColdFusion page.
| |