I just described components as movie clips with code in them. However, a movie clip with actions inside (maybe on a button or in a keyframe) doesn't automatically become a component. A Movie Clip symbol becomes a component when the component developer identifies parameters that can set differently for each instance. It's similar to how you can scale or tint any movie clip. In the case of components, you can set other properties in addition to the scale and tint. For example, you can set the label on each instance of a Button component (to change the word that appears on the button). The most common components are used for user interface (UI) elements such as buttons and menus. However, a component is just a clip with code and the ability to set initial values differently for each instance. You'll usually follow this order to use a component: 1. | Place it on the Stage.
| 2. | Populate it with data (for the Button component, you might change its label).
| 3. | Define the ActionScript that gets triggered when the component's event fires (say, how you want to handle the click event for the Button component).
| 4. | Place additional components that may work together (say, the RadioButton component, of which you'll always want two or more).
| We'll spread out all these steps into separate tasks in this hour, but this helps to know where you're headed. The first series of tasks this hour uses the ComboBox component. This is basically a drop-down menu from which the user can select any option, as shown in Figure 17.1. As with any component, you can define exactly how the ComboBox component is populated (that is, what's listed in the drop-down list) as well as how it will behave (that is, what it will do when the user makes a selection). You'll do just that in the next task. Figure 17.1. You can use the ComboBox component to let your users make selections. 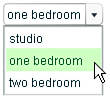
Try It Yourself: Manually Populate the ComboBox Component Later this hour you'll build on this task, but at this stage you'll just populate the component. Here are the steps: 1. | In a new file, open the Components panel by selecting Window, Components. It's possible to install sets of components, so make sure that the User Interface components section is fully expanded, as shown in Figure 17.2.
Figure 17.2. Make sure the User Interface components are fully expanded, as shown here. 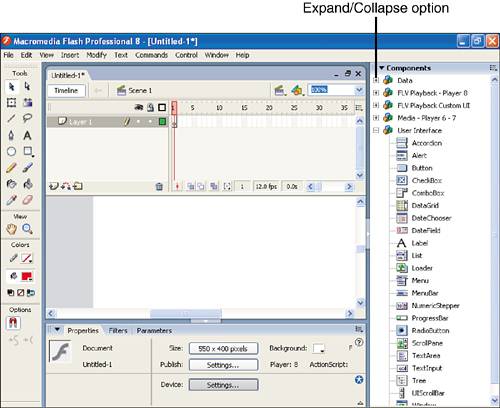
| 2. | Drag the ComboBox component onto the Stage as if you were dragging an instance from the Library. You can use the Free Transform tool to change its width, and you won't cause it to distort (but don't rotate or skew it). With the instance on the Stage still selected, open the Parameters panel. (If the Parameters panel is not in its default location adjacent to your Properties panel, select Window, Properties, Parameters.)
| 3. | Before you populate the parameters, give this instance a unique name so that later you can access it by using ActionScript. Name the instance myComboBox. Then click the Parameters tab so that you can populate this component uniquely.
| 4. | In the Parameters panel, select the line Labels. Click the magnifier button at the right of this line (see Figure 17.3).
Figure 17.3. Each named property (in the left column of the Parameters panel) can > be populated with unique values (in the right column). In the case of labels for a ComboBox component, you will enter several values. 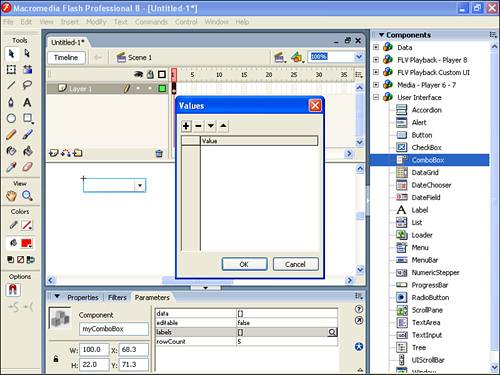
| 5. | In the Values dialog box that appears, click the plus button and then replace the text defaultValue with Macromedia. This will be the first item to appear in the ComboBox component. Repeat the process of clicking the plus button to create two more items one for Microsoft and another for Sams. You can click any value you have created and move it up or down in the list by pressing the arrow buttons. Click OK when you're finished.
| 6. | Test the movie, and you'll see the ComboBox component function. This is a pretty sophisticated piece of code, and all you had to do was provide unique data. You can likely use this in future projects. The only problem is that nothing happens when the user selects an item. That's because you've only set values for the "labels." In addition to populating each slot in the ComboBox with labels you must also supply a "data" for each slot. In addition, you need to write code that reacts to the user changing the ComboBox. You'll resolve all this in the task "Make the ComboBox Component Trigger Your ActionScript," later this hour.
| A common attribute of many components is the way they can contain both labels and data. The user sees the labels, but only your ActionScript can see the data. The data give you a convenient place to store additional details that the user doesn't need to see. In the case of the ComboBox component from the preceding task, the labels are what the user sees onscreen, and the data will be the actual web addresses to which we'll jump. In the end, you simply need to populate the data parameter with a list of three URLs that match the three companies listed in the labels parameter (which you just populated). You can do this manually in almost the same way you did in the preceding task, except that you enter values into the data parameter instead of the labels parameter. In order to demonstrate a different way that populates both data and labels, you'll do it by using ActionScript in the next task. Try It Yourself: Populate the ComboBox Component by Using ActionScript As the next step toward letting the user navigate using the ComboBox component, you'll populate both the data and labels by using ActionScript. Follow these steps: 1. | The following code will replace any labels you entered by hand via the Parameters tab of the Properties panel. It uses the addItem action, which accepts two parameters: the label and the data. Because your ComboBox component has the instance name myComboBox, you can put the following code into Frame 1. That is, select Frame 1, open the Actions panel, and then type this code:
var myComboBox:mx.controls.ComboBox; myComboBox.removeAll(); myComboBox.addItem("Macromedia", "http://www.macromedia.com/"); myComboBox.addItem("Microsoft", "http://www.microsoft.com/"); myComboBox.addItem("Sams", "http://www.samspublishing.com/"); The first line tells Flash that myComboBox is an instance of the ComboBox component. Actually, it helps you more than it helps Flash because anywhere you type myComboBox., the period will trigger a code hint for all the actions related to ComboBox components. The second line (removeAll()) will remove what you typed by hand. The last three lines each set a label and a corresponding value for data.
| 2. | Test the movie at this point, but it will appear no different than it did at the end of the preceding task. In the next task you will include the ActionScript to actually jump to those URLs. As you probably can imagine, this involves the getURL action. However, the next task will show you exactly when to trigger that script as well as how to determine the parameter (so you go to the right website).
| Although populating a component manually or by using ActionScript is important so that the user sees your content, you'll almost always need to tie the component to your movie by letting it trigger scripts. In the case of the ComboBox component, when the user makes a selection, you want to trigger the getURL action. Just as a button can respond to a variety of different events (such as press and release), each component has one or more events to which a script can respond. There are two basic ways to assign a script to a component's events: by using the Behaviors panel or the ActionScript panel to attach code directly to the component or by using the addEventListener() action. Personally, I recommend using addEventListener(), despite the fact that it's slightly more complex. If you use this method, when you have several components (say, a row of radio buttons), you won't have to physically add code to each one. Because both approaches are fairly easy, we'll look at both in the following task. Try It Yourself: Make the ComboBox Component Trigger Your ActionScript In this task you'll add ActionScript that the ComboBox component triggers each time the user makes a change. Here are the steps: 1. | With the file from the preceding task, select the instance of your ComboBox component and open the Actions panel. Begin by typing on(. You should immediately see a code completion hint that lists all the various events available for this component, as Figure 17.4 shows.
Figure 17.4. Typing on( triggers a code completion hint, which lists all available events for the selected component. 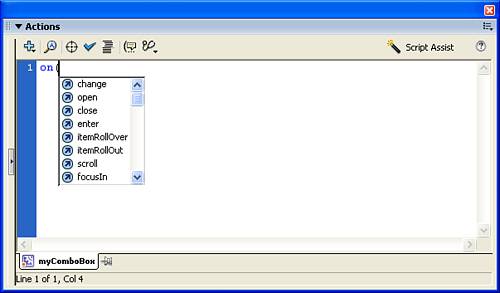
| 2. | Select the event change, which triggers every time the user changes the ComboBox component. Notice that there are many other events that you can trap. Make sure the skeleton for the on(click) event looks like this:
on(change){ } By the Way: Trapping Events Trap means that you can prepare a script that triggers when a particular event (that you want to trap) occurs. |
| 3. | You need to add a getURL action after line 1 and before line 2. Click after line 1 and press Enter. Then, from the plus button, select Global Functions, Browser/Network, getURL. The code hint should appear to help you fill it in, but for now just use Macromedia's address. The code should look like this:
on(change){ getURL("http://www.macromedia.com/"); } If you select Control Test Movie now, it will work only insofar as you'll hyperlink to Macromedia, no matter which selection you make.
| 4. | Open the Actions panel and make some edits to the script the behavior produced. Replace the entire parameter in the getURL action (quotation marks and all) with code that says "the data for the item that's currently selected in this ComboBox component." Naturally, that's pseudo-code. In the Actions panel, change the code to read as follows:
on (change) { getURL(this.selectedItem.data); } Although that code might look a bit complex, it uses dot syntax, as discussed last hour. In English you might go from specific to general, like "the data of the selected item in this component." But in script it goes from general to specific: this.selectedItem.data.
| 5. | When you test the movie now, it should work great. Whenever the user changes the ComboBox component selection, the currently selected item's data value (set way back in each addItem() action) will be used as the getURL action's first parameter.
| Code hints helped you in the preceding task because they displayed the available events for the ComboBox component. Naturally, you'll also find complete details in the Help panel. Another way to trigger code hints is by declaring the instance name of your component, like this: var myComboBox:mx.controls.ComboBox; In effect, this tells Flash that the name myComboBox is going to be a ComboBox component, so you want all code hints that come with it. To see how you can do the whole preceding task by using ActionScript (that is, not putting any code "on" the ComboBox itself), check out the following code. All you need is to have a ComboBox instance named myComboBox and the following code: var myComboBox:mx.controls.ComboBox; myComboBox.removeAll(); myComboBox.addItem("Macromedia", "http://www.macromedia.com/"); myComboBox.addItem("Microsoft", "http://www.microsoft.com/"); myComboBox.addItem("Sams", "http://www.samspublishing.com/"); myComboBox.addEventListener("change",doChange); function doChange(evt){ getURL(evt.target.selectedItem.data); } The last four lines replace what you did in the preceding task, but they use script (that is, you don't want any code ComboBox component instance itself). You don't have to use this all-script approach. It's just that, as you'll see with the RadioButton component in particular, it's easier to add an event listener to several buttons than to put code on each one. Plus, a custom function such as doChange() can also serve as the clearinghouse for several different buttons. Notice the code uses the selectedItem of evt.target (not this or myComboBox) that is, the particular component instance that changed. You'll see this in a minute, but I wanted to show you that there's always an all-script alternative to putting code right on components. |