In the preceding section, we had several event sources report to the same event listener. In this section, we will do the opposite. All AWT event sources support a multicast model for listeners. This means that the same event can be sent to more than one listener object. Multicasting is useful if an event is potentially of interest to many parties. Simply add multiple listeners to an event source to give all registered listeners a chance to react to the events. According to the SDK documentation, "The API makes no guarantees about the order in which the events are delivered to a set of registered listeners for a given event on a given source." Therefore, don't implement program logic that depends on the delivery order. | Here we will show a simple application of multicasting. We will have a frame that can spawn multiple windows with the New button. And, it can close all windows with the Close all button see Figure 8-10. Figure 8-10. All frames listen to the Close all command 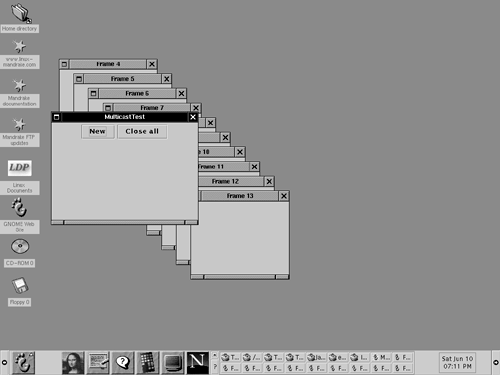 The listener to the New button of the MulticastPanel is the newListener object constructed in the MulticastPanel constructor it makes a new frame whenever the button is clicked. But the Close all button of the MulticastPanel has multiple listeners. Each time the BlankFrame constructors executes, it adds another action listener to the Close all button. Each of those listeners is responsible for closing a single frame in its actionPerformed method. When the user clicks the Close all button, each of the listeners is activated, and each of them closes its frame. Furthermore, the actionPerformed method removes the listener from the Close all button, since it is no longer needed once the frame is closed. Example 8-6 shows the source code. Example 8-6 MulticastTest.java 1. import java.awt.*; 2. import java.awt.event.*; 3. import javax.swing.*; 4. 5. public class MulticastTest 6. { 7. public static void main(String[] args) 8. { 9. MulticastFrame frame = new MulticastFrame(); 10. frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); 11. frame.show(); 12. } 13. } 14. 15. /** 16. A frame with buttons to make and close secondary frames 17. */ 18. class MulticastFrame extends JFrame 19. { 20. public MulticastFrame() 21. { 22. setTitle("MulticastTest"); 23. setSize(DEFAULT_WIDTH, DEFAULT_HEIGHT); 24. 25. // add panel to frame 26. 27. MulticastPanel panel = new MulticastPanel(); 28. Container contentPane = getContentPane(); 29. contentPane.add(panel); 30. } 31. 32. public static final int DEFAULT_WIDTH = 300; 33. public static final int DEFAULT_HEIGHT = 200; 34. } 35. 36. /** 37. A panel with buttons to create and close sample frames. 38. */ 39. class MulticastPanel extends JPanel 40. { 41. public MulticastPanel() 42. { 43. // add "New" button 44. 45. JButton newButton = new JButton("New"); 46. add(newButton); 47. final JButton closeAllButton = new JButton("Close all"); 48. add(closeAllButton); 49. 50. ActionListener newListener = new 51. ActionListener() 52. { 53. public void actionPerformed(ActionEvent event) 54. { 55. BlankFrame frame 56. = new BlankFrame(closeAllButton); 57. frame.show(); 58. } 59. }; 60. 61. newButton.addActionListener(newListener); 62. } 63. } 64. 65. /** 66. A blank frame that can be closed by clicking a button. 67. */ 68. class BlankFrame extends JFrame 69. { 70. /** 71. Constructs a blank frame 72. @param closeButton the button to close this frame 73. */ 74. public BlankFrame(final JButton closeButton) 75. { 76. counter++; 77. setTitle("Frame " + counter); 78. setSize(DEFAULT_WIDTH, DEFAULT_HEIGHT); 79. setLocation(SPACING * counter, SPACING * counter); 80. 81. closeListener = new 82. ActionListener() 83. { 84. public void actionPerformed(ActionEvent event) 85. { 86. closeButton.removeActionListener(closeListener); 87. dispose(); 88. } 89. }; 90. closeButton.addActionListener(closeListener); 91. } 92. 93. private ActionListener closeListener; 94. public static final int DEFAULT_WIDTH = 200; 95. public static final int DEFAULT_HEIGHT = 150; 96. public static final int SPACING = 40; 97. private static int counter = 0; 98. } 99. |