ASP.NET, part of Microsoft's new strategy for delivering software as a service, is the next generation environment for developing server-based, dynamic applications. Utilizing the .NET Framework, ASP.NET provides developers with a rich set of controls with which to build, deploy, and maintain applications and Web Services. Introduction to .NET .NET is Microsoft's new strategy for delivering software as a service. By reinventing the Application Services Provider (ASP) business model, Microsoft hopes to achieve independence from the long-standing boxware paradigm. The key features that make up .NET include the following: .NET platform The .NET platform includes the .NET Framework and tools to build and operate the services, clients, and so on. .NET products .NET products currently include MSN.NET, Office .NET, and Visual Studio .NET, which provide developers a rich environment for creating Web services utilizing such programming environments as C++, Visual Basic .NET, ASP.NET, C#, and so on. .NET My Services An initiative formerly known as "Hailstorm," the .NET My Services are a set of user-centric XML Web services currently being provided by a whole host of partners, developers, and organizations hoping to build vertical market application for devices, applications, and the Internet. The collection of My Services currently extends to passport, messenger, contacts, email, calendar, profile, lists, wallet, location, document stores, application settings, favorite Web sites, devices owned, and preferences for receiving alerts. Figure 22.6 shows the MSN browser and some of the .NET My Services. Figure 22.6. The MSN browser is one of the .NET products and includes ways of interacting with a myriad of Web services. 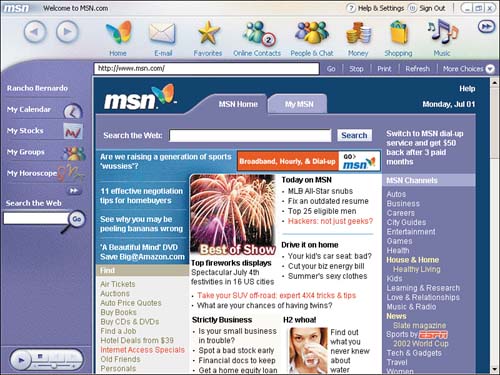 The .NET Framework The .NET Framework is the environment for which .NET applications are built. The .NET Framework specifically is designed for Web Services and supports development across a larger spectrum. The three main parts of the .NET Framework are Although the .NET Framework is just a small piece of the pie, it lays the foundation for .NET applications and Web Services. Remember, that the .NET Framework, although crucial in the .NET strategy, is only a small piece of the .NET platform and the .NET platform is just one-third of the .NET initiative. TIP Why is the .NET Framework relevant to Dreamweaver MX? If you are to build ASP.NET applications using Dreamweaver MX, you will first have to install the .NET Framework SDK. The .NET Framework SDK can be downloaded from Microsoft's Web site at www.microsoft.com. The Common Language Runtime One of the most important features within the .NET Framework is the Common Language Runtime (CLR). The CLR manages programming simplicity by exposing a unique compilation and execution flow. In the past, ASP supported VBScript and JScript as languages that could be used to write the applications. The CLR currently supports about a dozen, with the possibility for many more. The reason this can be accomplished is simple source code is compiled to Intermediate Language (IL) code, which in its simplest definition is just assembly code. Figure 22.7 shows how source code is compiled to IL code and metadata is created using the metadata engine. The IL and metadata are linked, resulting in a DLL or assembly. When executed, the IL code and any class that the application may use are combined using the class loader. The Just-In-Time (JIT) compiler produces more code that is passed onto the runtime for execution. Figure 22.7. The CLR involves a complex series of operations before final execution.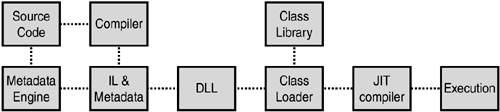 The .NET Framework Class Library The .NET languages are all about classes. Everything, right down to adding two numbers, implements a class in some form or fashion. The .NET Framework exposes a vast set of classes and namespaces appropriately named the Framework Class Library. These are contained within a set of namespaces called the Base Class Library that provides basic services for handling data, errors, garbage collection, strings, numbers, and more. In all, there are about 90 namespaces that contain about 3,400 classes. Understanding Namespaces As I mentioned, the Framework Class Library contains roughly 3,400 classes, all organized within a hierarchy of namespaces. If you think of the hierarchy as a tree of namespaces, System would be at the root, and all other namespaces would stem from that. For example, if you wanted to work with writing and reading text files, you would probably use the System.IO namespace. If you wanted to create a connection to an Access database, you would probably need the System.Data.OleDb namespace, and if you wanted to create a Web form utilizing Web controls, you would need to use the System.Web.UI.WebControls namespace. As you can see, namespaces are grouped through a hierarchy of namespaces, separated by dot syntax, and are relatively easy to use. NOTE Because there are so many namespaces, it is nearly impossible to remember which one contains the class that you need to expose. You can view a list of all the namespaces in the Framework Class Library by viewing the Reference Documentation for the .NET Framework. Although for the most part you will have to Import most namespaces into your ASP.NET pages, some you will not. ASP.NET implicitly imports a group of namespaces known as the ASP.NET Base Classes. These include the following: System Contains all the data types as well as classes that relate to working with numbers and time. System.Collections Contains classes for working with collection types such as arrays. System.Collections.Specialized Contains classes for working with specialized collections such as string collections. System.Configuration Contains classes for working with the Web.Config file and other configuration settings. System.Text Contains classes for creating and manipulating strings. System.Text.RegularExpressions Contains classes for doing regular expression matches. System.Web Contains the classes for working with the Web, including traditional request and response properties. System.Web.Caching Contains the classes used for caching content with a page. System.Web.Security Contains classes used for implementing authentication. System.Web.SessionState Contains classes used to implement the state of a session. System.Web.UI Contains classes used in the construction of ASP.NET pages. System.Web.UI.HTMLControls Contains the classes for HTML controls. System.Web.UI.WebControls Contains the classes for Web controls. ASP.NET Page Structure Before you can begin writing ASP.NET pages, it is important to discuss how the page is even constructed. Aside from containing code (server-side execution and dynamic content) and markup for structure, ASP.NET pages also contain seven distinct elements. These are In the following sections, we will discuss the four most important ones. Directives Directives are used within an ASP.NET page to tell the compiler how it should compile the page. The most useful directives are Page and Import, but you can use any of the following as well: Control, Register, Assembly, and OutputCache. Directives are inserted at the top of an ASP.NET page and look like the following: <%@Page attribute=value %> ASP.NET Controls ASP.NET controls provide a way for you to develop dynamic Web applications without having to embed clumsy and antiquated scripts. Because ASP.NET controls render the code that the user will actually see, ASP.NET controls can be considered the building blocks for the dynamic and interactive portions of your Web applications. ASP.NET controls take the form of HTML Controls HTML controls are constructed by simply adding the runat="server" attribute within any ordinary HTML tag. HTML controls are discussed later in the chapter. Web Controls With Web controls, Microsoft basically re-invented HTML. Web controls provide all the dynamic input and output for the ASP.NET page. Custom Controls Although ASP.NET comes bundled with ready-to-use controls, at times you might want to create your own custom controls to interact with. Validation Controls Validation controls are used in the validation of form objects. Rich Controls Rich controls include the calendar control, XML control, and the ad rotator control. Data Controls Data controls provide a means of interacting with data from a database. Data controls include the DataList, DataGrid, and Repeater. Mobile Controls Mobile controls provide a means for creating rich Web applications for handheld devices. Code Render Blocks Code render blocks allow the user to define code when the page is rendered, not compiled. Code render blocks can be written similar to the following: <% Dim strText As String = "My Message" Response.Write(strText) %> Literal Text and HTML Tags The final and most important element that you can include in your page is plain old HTML. HTML lays the foundation for your ASP.NET page and is what ultimately controls the structure and design of the content within it. Configuring the Web Store to Run ASP.NET Dreamweaver MX provides excellent integration for ASP.NET, including a library of controls available from the Tag Chooser, drag-and-drop objects from the Objects panel, ASP.NET objects from the Insert menu, DataSets from the Bindings panel, and many server behaviors to help you get started producing dynamic content including the DataGrid, DataList, and Repeaters controls. To configure the Web Store application to run under ASP.NET, follow the three steps outlined next: -
Define a new site for the Web Store application. -
Choose a document type. -
Configure the application testing server. Define a New Site for the Web Store Application One of the most important things you can do with any Web application, whether it is dynamic or static, is to define a new site for it. Creating a new site ensures link integrity, helps when performing global find and replaces and link changing, and provides the benefits of site reports. You can define a new site for the Web Store application by following the steps outlined next: -
Start Dreamweaver MX and open the Application panel. Figure 22.8 shows the three steps to creating an application within Dreamweaver MX. Figure 22.8. Creating a new site involves three steps: defining the location of the site, choosing a server technology, and picking a testing server.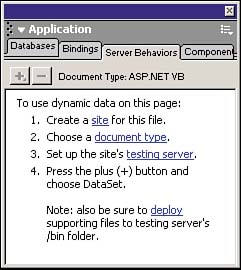 -
From either the Database, Binding, or Server Behaviors panel, select Site from step one. -
After you have selected the Site link, select the Advanced tab from the Site Definition window. Figure 22.9 shows the site definition information. Figure 22.9. Use the Site Definition window to define properties for your Web application.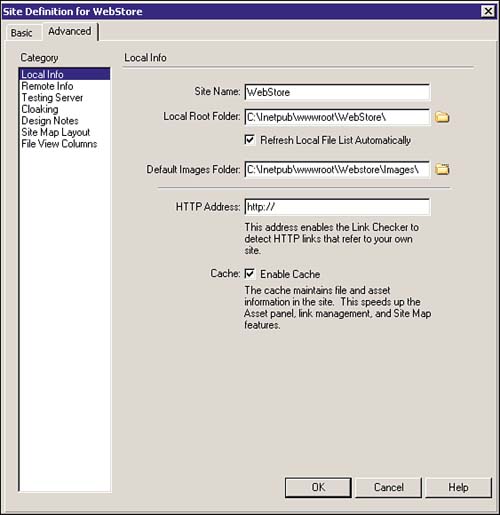 -
Enter Web Store for the Site Name. -
Navigate to your local root folder where your application resides. If you have not already done so, create a Project folder somewhere on your computer called Web Store and another folder within that called Images. This folder will serve as the repository for all the files that will reside in your application. -
Refresh File List Automatically should be checked. -
Navigate to the Images folder within the Web Store folder. -
Enable Site Cache should be checked. -
Press OK. Figure 22.10 shows how you should now have a check mark next to step one in any of the three tabs (Database, Bindings, Server Behaviors) within the Application panel. Figure 22.10. After you have defined your site, a check mark will appear after the first step.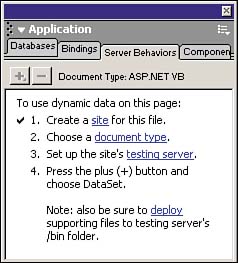 Choose a Document Type The document type will serve as the object model for the type of application you want to create and run. To define a document type, follow these steps: -
Choose Document Type from step 2 in either the Database, Bindings, or Server Behaviors tab within the Application panel. CAUTION Sometimes step 2 may already be checked after you've defined your site. Dreamweaver MX does not know the model that you plan to use and will default to the last one picked. You still need to perform this step. -
When the Document Type dialog box appears as shown in Figure 22.11, select ASP.NET VB. Figure 22.11. Select ASP.NET VB as the Document Type.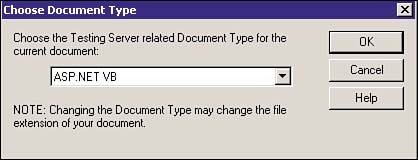 -
Select OK. Configure the Application Testing Server The final step to defining the Web Store application is setting up the testing server. Remember, in a Microsoft server environment, Web applications are run in the C:\Inetpub\wwwroot directory. Before you can define the testing server, you must properly create the project folder within the C:\Inetpub\wwwroot directory. To do so, follow these steps: -
Navigate to C:\Inetpub\wwwroot. -
Create a new folder called Web Store. -
Inside of Web Store, create a new folder called Images. You are now ready to define the testing server. -
Choose Testing Server from step 3 in either the Database, Bindings, or Server Behaviors tab within the Application panel. -
The site definition for Web Store window will appear. -
Select ASP.NET VB from the Server Model drop-down list. -
Select Local/Network from the Access drop-down list. -
Navigate to C:\Inetpub\wwwroot\Webstore as the testing server folder. Click Select. -
Refresh File List Automatically should be checked. -
http://localhost/ should be set as the URL prefix. -
Select OK. There will also be a reminder that Dreamweaver MX will deploy what it calls "supporting files" to the bin directory. Select Deploy to bring up the Deploy Supporting Files to Testing Server dialog box. Remember, this is accomplished because of the CLR. The CLR compiles the source code and any metadata into IL code, which is then linked into a DLL or assembly within the bin directory. The file remains there until execution. Using ASP.NET with Dreamweaver MX Like ASP, ASP.NET integration begins with the Application panel. Most of the functionality that you will use whether it's creating a database connection, working with DataSets, or displaying dynamic content within DataGrids or DataLists will spawn from the Application panel, most notably the Database, Bindings, and Server Behaviors tabs. The features that make up the ASP.NET integration include the following: The Database panel The Database panel provides you with a way of defining a database connection to either a SQL Server database or a supported OLE DB type. The Data Bindings panel The Data Bindings panel allows you to work with DataSets and Stored Procedures. You can also find more data sources from the Macromedia Exchange by selecting Get More Data Sources. The Server Behaviors panel The Server Behaviors panel gives you the opportunity to work with Data Sources such as DataSets and Stored Procedures, Data Controls in DataGrids, DataLists, and Repeaters, and various other record-handling mechanisms. The Components Panel The Components panel allows you to work with Web Services. The Tag Chooser The Tag Chooser gives you access to controls such as Web controls, validation controls, Macromedia server controls, and ASP.NET specific style information and templates. ASP.NET Objects ASP.NET objects are available either through the Insert menu or the Objects panel. You can insert various predefined ASP.NET objects including data handling objects, namespace objects, and various other controls. The Web.Config File The Web.Config file is usually automatically created for you after you define a database connection. Residing in the root directory of your project folder, the Web.Config file contains crucial configuration information that the compiler relies on. |