Just as with ASP.NET pages, mobile pages consist of Web forms and server controls. Recall that ASP.NET pages can have server controls, script blocks, and HTML. In a mobile Web page, however, only server controls and script blocks are allowed; they cannot contain any HTML. Let's take a quick look at a short example, shown in Listing 23.1. Listing 23.1 Your First Mobile Page 1: <%@ Page Inherits="System.Web.UI.MobileControls.MobilePage" Language="VB" %> 2: <%@ Register TagPrefix="Mobile" Namespace="System.Web.UI.MobileControls" Assembly="System.Web.Mobile" %> 3: 4: <Mobile:Form BackColor="#ccddcc" runat="server" 5: FontName="Times" FontSize="3"> 6: <Mobile:Label runat="server" Text="Hello World!"/> 7: </Mobile:Form>  | Save this listing as listing2301.aspx. At first glance, this looks like any other ASP.NET page that inherits from a code-behind (Day 19, "Separating Code from Content") and implements a user control (Day 6, "Learning More About Web Forms"). Recall that the Inherits attribute on line 1 specifies a class that this page should inherit from (instead of the usual System.Web.UI.Page class). All mobile pages must inherit from this class so that ASP.NET knows that you're building a special type of ASP.NET page. | Line 2 looks like the registration for a user control. In essence, that's what it is. The namespace System.Web.UI.MobileControls contains all the mobile server controls that you'll be using in your mobile pages it resides in a file named System.Web.Mobile.dll. This means that mobile server controls are not directly part of ASP.NET; you have to register them explicitly. Note the TagPrefix set to Mobile; I'll touch on this next. Note Lines 1 and 2 highlight the two lines of code that must be in every mobile Web page: <%@ Page Inherits="System.Web.UI.MobileControls.MobilePage" Language="VB"%> <%@ Register TagPrefix="Mobile" Namespace="System.Web.UI.MobileControls" %> Don't forget these lines in your pages! On line 4, you start a mobile Web form, which is similar to an ASP.NET Web form, except that instead of using the tag prefix ASP, you use Mobile, as declared on line 2. Note that this form also must have the runat="server" attribute, which gives it the same capabilities as regular Web forms. In the declaration of this form, you specify a few additional properties, such as BackColor and FontName (the values chosen here are used to reflect what a cell phone user would see on her LCD screen). On line 6, you declare a mobile Label control, which works just like a regular Label control. Note that it also uses the Mobile tag prefix, and contains the runat="server" attribute. Finally, on line 7, you close the mobile Web form. When viewed from the browser, this listing produces Figure 23.3. Figure 23.3. The page as shown from a cell phone…sort of.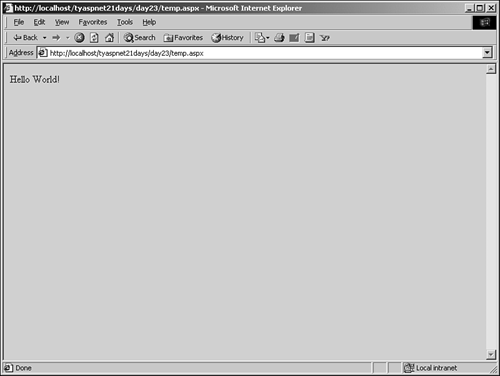 You'll examine more of the mobile server controls later today, but they are typically very similar to their counterparts in ASP.NET. Table 23.1 shows all the controls available to mobile Web forms. Table 23.1. The Mobile Web Server Controls Control | Description | AdRotator | Displays ads specified from an XML advertisement file. | Calendar | Displays an interactive calendar. | Command | Provides a way to invoke event handlers; similar to a button in ASP.NET. | CompareValidator | Compares the value in one control to a constant value or another control. | CustomValidator | Allows you to provide your own custom validation routines. | DeviceSpecific | Used for templates on mobile Web forms. | Form | A container for controls in a mobile page. | Image | Displays an image. | Label | Displays noninteractive text. | Link | Creates a hyperlink to another form or page. | List | Renders a list of items. | MobilePage | The base class for all mobile ASP.NET pages. | ObjectList | Similar to a List control, but lists data types. | Panel | A container for other mobile controls. | PhoneCall | Displays a device-dependent user interface for automatically calling or displaying telephone numbers. | RangeValidator | Validates the value in a control against a specified range of values. | RegularExpressionValidator | Validates the value in a control against a regular expression string. | RequiredFieldValidator | Ensures that a value is entered into a control (that is, ensures that the control is not left blank). | SelectionList | Similar to the ASP.NET CheckBox, ListBox, and RadioButton controls. | StyleSheet | Used to organize styles for controls; does not provide a visual display. | TextBox | Displays a single-line text box for user entry. Use the Type property to determine which kind of box to display: Text (works normally), Password (hides input with asterisks), or Numeric (restricts input to numeric values, useful for cell phones). | TextView | Displays a large amount of noneditable text. Similar to the Label control. | ValidationSummary | Displays a summary of all validation errors that have occurred on the page. | Note Note that many of the properties of these controls are dependent on the viewing device. For example, setting the Type property on a TextBox to Numeric should theoretically allow only numbers to be entered as input. In a Web browser, this restriction is not enforceable without complex client-side scripting. It is enforceable, however, in cell phones. Take note of your viewing audience (see "Device-Specific Output and Template Sets," later today). The Way Mobile Forms Work Aside from a few small changes, Listing 23.1 looks and works just as an ASP.NET page does. Mobile forms can, however, get a bit more complex. Let's examine the design of mobile devices a bit further. If you've ever used a cell phone or similar device, you know that interacting with one is not exactly the same as interacting with a PC. Due to the small size of the screen and the form of the input devices, applications must present information in a different way. Specifically, information is gathered into small chunks that can be displayed on a few "pages" of the device. There isn't a lot of text to display, there are hardly any complex images, and users make choices by selecting from a menu. Compare this to Web pages, where text is a very prominent feature, images are abundant, and most user interaction is accomplished by clicking on links or buttons, and you can see why a different paradigm is needed. Also, navigation in mobile devices isn't as easy as in a browser; users can't simply type in the URL to a new page, and loading new pages often takes a very long time due to the limited bandwidth of these devices. To address this, mobile Web forms have several differences from ASP.NET Web forms. The first, and probably largest, is that mobile pages can contain as many server-side forms as necessary, whereas ASP.NET pages can only contain one. Each form can contain different information and controls to display; this minimizes the need to load new pages into the device. A single page can post back onto itself, and depending on the criteria, the page can choose to display a different form. Thus, a mobile Web form and a mobile page are two highly distinguishable entities. A single mobile page can contain an entire application's worth of mobile forms. Figure 23.4 illustrates the use of multiple forms on one mobile page. Figure 23.4. An entire application can be contained in one mobile page, but several mobile forms.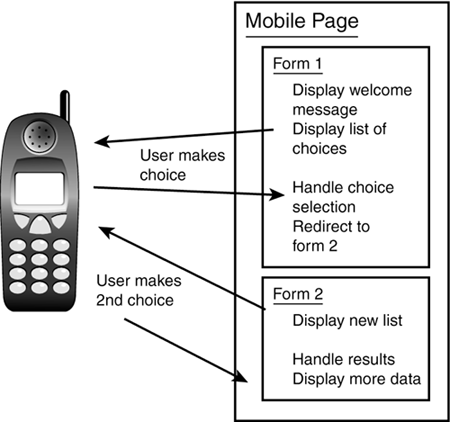 Mobile Web forms also focus more on presenting information to the user as menus and lists, rather than long paragraphs of text and links. Menus and lists are the easiest elements for users to interact with in the mediocre input controller world of wireless devices, so it is only logical that emphasis would be placed on these constructs. There are other minor differences that you'll come across as you're developing your pages. There are numerous similarities as well, such as event handling and user input validation, which work the same as with ASP.NET pages. Let's build some more examples. Building a More Apropos Interface Before I go into more examples, though, you might have noticed that Figure 23.3 isn't exactly the ideal interface for developing mobile applications. The screen might be too large to show developers what their pages will actually look like. Therefore, let's develop a different interface, one that better reflects the target audience. Listing 23.2 shows a page that presents your mobile pages in an Iframe a type of HTML frame that can contain different URLs within the containing page. Listing 23.2 Developing a Better Suited Interface 1: <script Language="VB" runat="server"> 2: sub Page_Load(Sender as Object, e as EventArgs) 3: frmScreen.Attributes("src") = Request.Params("page") 4: end sub 5: </script> 6: <html><body> 7: <p> <p> 8: <center> 9: <table border=1 width=100 height=100> 10: <tr> 11: <td valign="top"> 12: <iframe width="125" height="100" 13: marginwidth="2" marginheight="2" 14: runat="server"></iframe> 15: </td> 16: </tr> 17: </table> 18: </center> 19: </body></html> Save this listing as Frame.aspx. This page is mostly plain HTML that displays an IFrame, shown on line 12. The IFrame does not map to a predefined ASP.NET server control, but that doesn't stop you from making it into a generic control (represented by the HtmlGenericControl; see Appendix C, "ASP.NET Controls: Properties and Methods," for more information). Notice that the src property, which determines which page to display in the IFrame, is set to the value returned from a Request parameter on line 3. This code retrieves a variable named page from the querystring this variable holds the actual mobile page you want to display. Now request this page from your browser with the following URL: http://localhost/tyaspnet21days/day23/Frame.aspx?page=listing2301.aspx Note that the querystring contains the mobile page you developed earlier. This URL should produce the display shown in Figure 23.5. Figure 23.5. The IFrame allows you to display a mobile page in a way more representative of your target audience.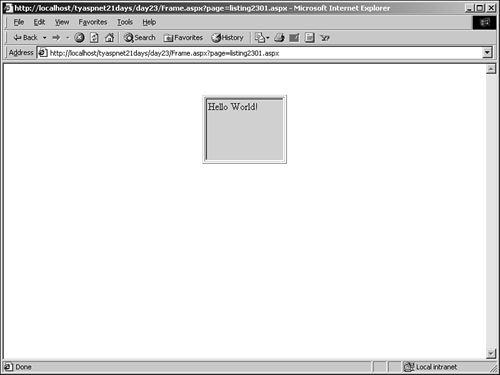 Now that looks more like it! It's not exactly a representation of a cell phone, but this will allow you to better see how your pages will look in smaller devices. The rest of the examples in this chapter use Frame.aspx with a particular querystring to display the output. |