Once you have PHP set to reveal what errors occur, you might want to adjust the level of error reporting. Your PHP installation as a whole or individual scripts can be set to report or ignore different levels of errors. Table 6.1 lists most of the levels, but they can generally be one of these three kinds: Notices, which do not stop the execution of a script and may not necessarily be a problem. Warnings, which indicate a problem but don't stop a script's execution. Errors, which stop a script from continuing (including the ever-common parse error, which prevent scripts from running at all). Table 6.1. PHP's error-reporting settings, to be used with the error_reporting() function or in the php.ini file.Error-Reporting Levels |
---|
NUMBER | CONSTANT | REPORT ON |
---|
1 | E_ERROR | Fatal run-time errors (that stop execution of the script). | 2 | E_WARNING | Run-time warnings (nonfatal errors). | 4 | E_PARSE | Parse errors. | 8 | E_NOTICE | Notices (things that could or could not be a problem). | 256 | E_USER_ERROR | User-generated error messages, generated by the trigger_error() function. | 512 | E_USER_WARNING | User-generated warnings, generated by the trigger_error() function. | 1024 | E_USER_NOTICE | User-generated notices, generated by the trigger_error() function. | 2047 | E_ALL | All errors and warnings. | 2048 | E_STRICT | E_ALL plus recommendations. |
As a rule of thumb, you'll want PHP to report on any kind of error while you're developing a site but report no specific errors once the site goes live. For security and aesthetic purposes, it's generally unwise for a public user to see PHP's detailed error messages. Frequently, error messagesparticularly those dealing with the databasewill reveal certain behind-the-scenes aspects of your Web application that are best not shown. While you hope all of these will be worked out during the development stages, that's rarely the case. You can follow the instructions in the previous section for altering the php.ini file specifically for error reporting or set this on a script-by-script basis using the error_reporting() function. This function is used to establish what type of errors PHP should report on within a specific page. The function takes either a number or a constant, using the values in Table 6.1 (the PHP manual lists a few others, related to the core of PHP itself). error_reporting(0); // Show no errors. A setting of 0 turns error reporting off entirely (errors will still occur; you just won't see them anymore). Conversely, error_reporting (E_ALL) will tell PHP to report on every error that occurs. The numbers can be added up to customize the level of error reporting, or you could use the bitwise operators| (or), ~ (not), & (and)with the constants. With this following setting any non-notice error will be shown: error_reporting (E_ALL & ~E_NOTICE); Because you'll probably want to adjust error reporting on a site-wide basis and change the level of reporting depending upon whether or not the site is live, you'll want to call the error_reporting() function in one file common to the entire Web site. To do this, a configuration file will be created for handling a dynamic application's errors. To adjust error reporting for an application 1. | Create a new PHP script in your text editor (Script 6.1).
<?php # Script 6.1 - config.inc.php Script 6.1. This configuration file sets error reporting on nearly the highest level. 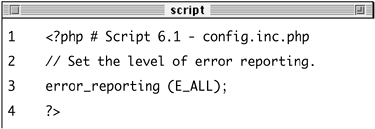
This is a plain PHP page; there will be no HTML in it at all.
| 2. | Set the error reporting on the highest level.
error_reporting (E_ALL); For development purposes, I'd like PHP to notify me of all errors and warnings. This line will accomplish that. Because E_ALL is a constant, it is not enclosed in quotation marks.
| 3. | Close the PHP script and save the file as config.inc.php.
?> You can give your file just about any extension, but .inc.php indicates that it's both an included file and a PHP file.
| 4. | To use the file in a Web application, include the following line at the top of every script:
include ('config.inc.php'); This configuration file will set the behavior for an entire application. Including it first in every PHP script will apply the error reporting to that script. You'll see this concept in action in Chapter 13, "ExampleUser Registration."
| Tips E_STRICT has been added in version 5 of PHP. Using it means that PHP will make recommendations for optimal coding and let you know when you're using outdated functions or variables. In current versions of PHP, error reporting is set to E_ALL & ~E_NOTICE (report on all errors except for notices) by default. The scripts written in this book were all programmed with PHP's error reporting on the highest level (in the hopes of catching every possible problem).
Suppressing Errors with @ Individual errors can be suppressed in PHP using the @ operator. For example, if you don't want PHP to report if it couldn't open a file, you would code @include ('config.inc'.php); Or if you don't want to see a "division by zero" error: $x = 8; $y = 0; $num = @($x/$y); The @ symbol will work only on expressions, like function calls or mathematical operations. You cannot use @ before conditionals, loops, function definitions, and so forth. As a rule of thumb, I recommend that @ be used on functions whose execution, should they fail, will not affect the functionality of the script as a whole. Or you can suppress PHP's errors when you will handle them more gracefully yourself. |
|