To this point, every script in the book has consisted of a single file that contains all of the required HTML and PHP code. But as you develop more complex Web sites, you'll see that this methodology has many limitations. PHP can readily make use of external files, a capability that allows you to divide your scripts into their distinct parts. Frequently you will use external files to extract your HTML from your PHP or to separate out commonly used processes. PHP has four functions for using external files: include(), include_once(), require(), and require_once(). To use them, your PHP script would have a line like include_once('filename.php'); require('/path/to/filename.html'); Using any one of these functions has the end result of taking all the content of the included file and dropping it in the parent script (the one calling the function) at that juncture. An important attribute of included files is that PHP will treat the included code as HTML (i.e., send it directly to the browser) unless it contains code within the PHP tags. Previous versions of PHP had a different distinction between when you'd use include() and when you'd use require().Now the functions are exactly the same when working properly but behave differently when they fail. If an include() function doesn't work (it cannot include the file for some reason), a warning will be printed to the Web browser (Figure 3.1), but the script will continue to run. If require() fails, an error is printed and the script is halted (Figure 3.2). Figure 3.1. Two failed include() calls. 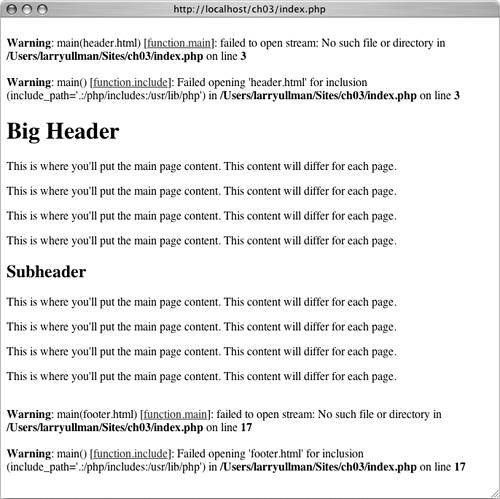 Figure 3.2. The first failure of a require() function will print an error and terminate the execution of the script. 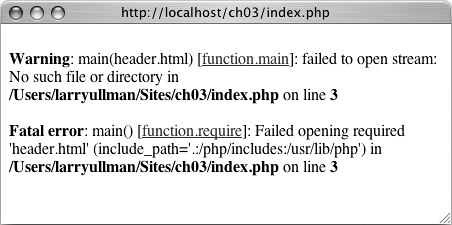
Both functions also have a *_once() version, which guarantees that the file in question is included only once regardless of how many times a script may (presumably inadvertently) attempt to include it. require_once('filename.php'); include_once('filename.php'); In this first example, I'll use included files to separate my HTML formatting from my PHP code. Then, the rest of the examples in this chapter will be able to have the same appearance without the need to rewrite the HTML every time. The concept results in a template system, an easy way to make large applications consistent and manageable. The focus in these examples is on the PHP code itself; you should also read the sidebar on "Site Structure" so that you understand the organization scheme of the files, and if you have any questions about the CSS (Cascading Style Sheets) or (X)HTML used in the example, see the references in Appendix C, "Resources." To included multiple files 1. | Design an HTML page in your text or WYSIWYG editor (Script 3.1 and Figure 3.3).
Script 3.1. The HTML template for this chapter's Web pages. Download the layout.css file it uses from the book's supporting Web site. 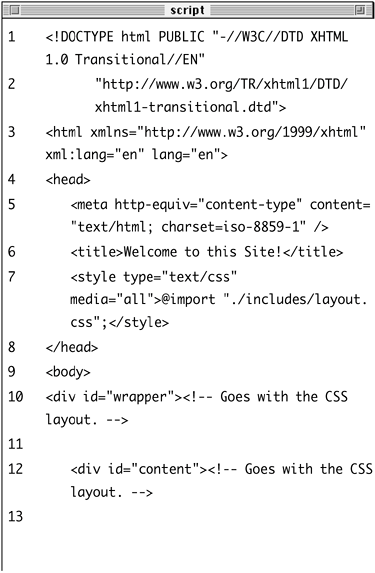 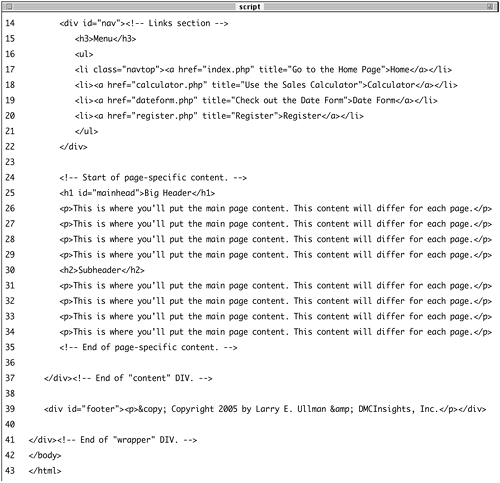 Figure 3.3. The HTML and CSS design as it appears in the Web browser (without using any PHP). 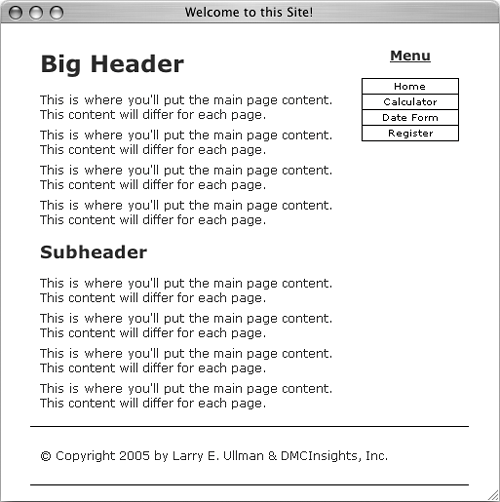
To start creating a template for a Web site, design the layout like a standard HTML page, independent of any PHP code. The section of the layout that will change from page to page is enclosed within HTML comments indicating such.
Note: in order to save space, the CSS file for this example (which controls the layout) is not included in the book. You can download the file through the book's supporting Web site (see the site's Extras page) or do without it (the template will still work, it just won't look as nice).
| 2. | Copy everything from the first line of the layout's source to just before the page-specific content and paste it in a new document (Script 3.2).
<!DOCTYPE html PUBLIC "-//W3C// DTD XHTML 1.0 Transitional//EN "http://www.w3.org/TR/xhtml1/DTD/ xhtml1-transitional.dtd> <html xmlns="http://www.w3.org/1999/ xhtml xml:lang="en" lang="en"> <head> <meta http-equiv="content-type" content="text/html; charset=iso-8859-1 /> <title>Welcome to this Site!</title> <style type="text/css" media= "all>@import "./includes/ layout.css;</style> </head> <body> <div > <div > <div > <h3>Menu</h3> <ul> <li ><a href= "index.php title="Go to the Home Page>Home</a> </li> <li><a href="calculator php title="Use the Sales Calculator>Calculator</a> </li> <li><a href="dateform.php" title="Check out the Date Form>Date Form</a></li> <li><a href="register.php" title="Register>Register< /a></li> </ul> </div> <!-- Script 3.2 - header.html --> Script 3.2. The initial HTML for each Web page will be stored in a header file. 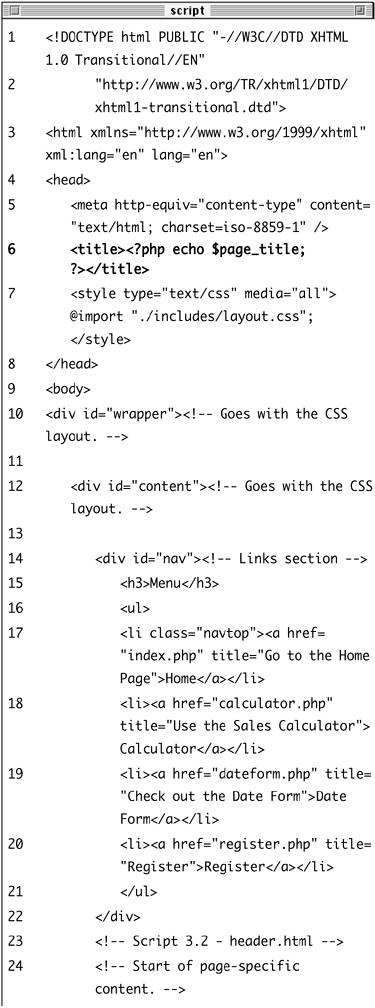
This first file will contain the initial HTML tags (from DOCTYPE through the head and into the beginning of the page body). It also has the code that makes the column of links on the right side of the browser window (see Figure 3.3).
| 3. | Change the page's title line to read
<title><?php echo $page_title; ?> </title> I'll want the page title (which appears at the top of the Web browser; see Figure 3.3) to be changeable on a page-by-page basis. To do so, I set this as a variable that will be printed out by PHP.
| 4. | Save the file as header.html.
Included files can use just about any extension for the filename. Some programmers like to use .inc to indicate that a file is used as an include. In this case, you could also use.inc.html, which would indicate that it's both an include and an HTML file (to distinguish it from includes full of PHP code).
| 5. | Copy everything in the original template from the end of the page-specific content to the end of the page and paste it in a new file (Script 3.3).
<!-- Script 3.3 - footer.html --> </div> <div ><p>© Copyright 2005 by Larry E. Ullman & DMCInsights, Inc.</p></div> </div> </body> </html> Script 3.3. The concluding HTML for each Web page will be stored in this footer file. 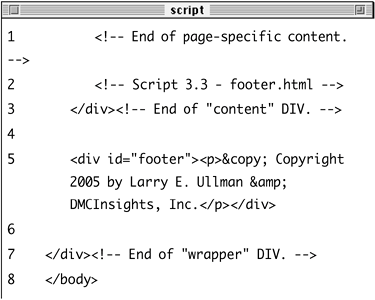
The footer file contains the remaining formatting for the page body, including the page's footer, and then closes the HTML document.
| 6. | Save the file as footer.html.
| 7. | Create a new PHP document in your text editor (Script 3.4).
<?php # Script 3.4 - index.php Script 3.4. This script generates a complete Web page using a template stored in external files. 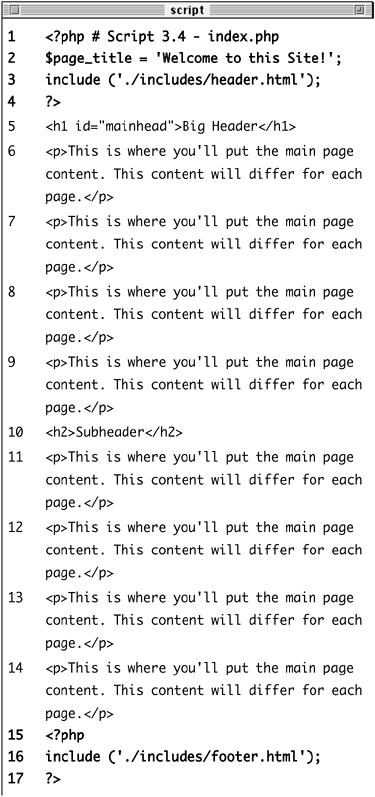
Since this script will use the included files for most of its HTML formatting, I can begin and end with the PHP tags rather than HTML.
| 8. | Set the $page_title variable and include the HTML header.
$page_title = 'Welcome to this Site!'; include ('./includes/header.html'); The $page_title will allow me to set a new title for each page that uses this template system. Since I establish the variable before I include the header file, the header file will have access to that variable. Remember that this include() line has the effect of dropping the contents of the included file into this page at this spot.
| 9. | Close the PHP tags and copy over the page-specific content from the template.
?> <h1 >Big Header</h1> <p>This is where you'll put the main page content. This content will differ for each page.</p> <p>This is where you'll put the main page content. This content will differ for each page.</p> <p>This is where you'll put the main page content. This content will differ for each page.</p> <p>This is where you'll put the main page content. This content will differ for each page.</p> <h2>Subheader</h2> <p>This is where you'll put the main page content. This content will differ for each page.</p> <p>This is where you'll put the main page content. This content will differ for each page.</p> <p>This is where you'll put the main page content. This content will differ for each page.</p> <p>This is where you'll put the main page content. This content will differ for each page.</p> Site Structure When you begin using multiple files in your Web applications, the overall site structure becomes more important. When laying out your site, there are three considerations: Ease of maintenance Security Ease of user navigation Using external files for holding standard procedures (i.e., PHP code), CSS, JavaScript, and the HTML design will greatly improve the ease of maintaining your site because commonly edited code is placed in one central location. I'll frequently make an includes or templates directory to store these files apart from the main scripts. I recommend using the .inc or .html file extension for documents where security is not an issue (such as HTML templates) and .php for those that contain more sensitive data (such as database access information). You can also use both .inc and .html or .php so that a file is clearly indicated as an include of a certain type: functions.inc.php or header.inc.html. Finally, try to structure your sites so that they are easy for your users to navigate, both by clicking links and by manually typing a URL. Try to avoid creating too many nested folders or using hard-to-type directory names and filenames containing upper- and lowercase letters and all manner of punctuation. |
This information could be sent to the browser using echo(), but since there's no dynamic content here, it'll be easier and more efficient to exit the PHP tags temporarily.
| 10. | Create a final PHP section and include the footer file.
<?php include ('./includes/footer.html'); ?>
| 11. | Save the file as index.php and upload to your Web server.
| 12. | Create an includes directory in the same folder as index.php. Then place header.html, footer.html, and layout.ccs (downloaded from www.DMCInsights.com/phpmysql2), into this includes directory.
| 13. | Test the template system by going to the index.php page in your Web browser (Figure 3.4).
Figure 3.4. Now the same layout (see Figure 3.3) has been created using external files in PHP. 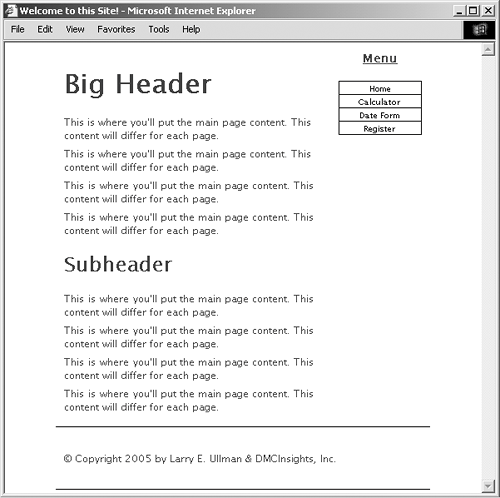 The index.php page is the final result of this template system. You do not need to access any of the included files directly, as index.php will take care of incorporating their contents.
| 14. | If desired, view the HTML source of the page (Figure 3.5).
Figure 3.5. The generated HTML source of the Web page should replicate the code in the original template (refer to Script 3.1). 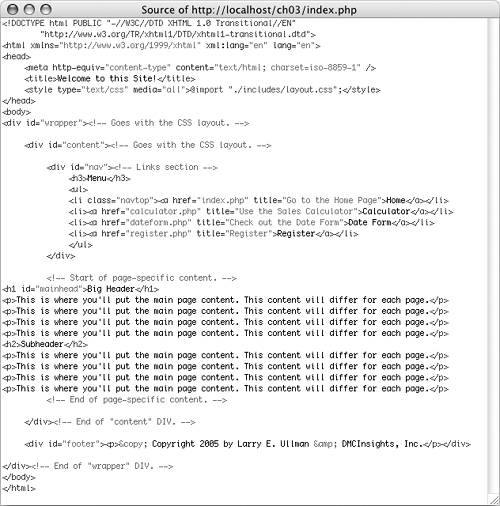
| Tips In the php.ini file, you can adjust the include_path setting, which dictates where PHP is and is not allowed to retrieve included files. As you'll see in Chapter 7, "Using PHP with MySQL," any included file that contains sensitive information (like database access) should be stored outside of the Web document directory. As a best practice, use the ./filename syntax when referring to files within the same directory as the parent (including) file, as I've done here. A file stored in a directory above the parent file would be included using the path ../filename, and a file stored in a directory below the parent file would use ./directory/filename. You can include your files using relative pathsas I have hereor absolute paths: include ('/path/to/filename');
Since require() has more impact on a script when it fails, I recommend using it for mission-critical includes (like those that connect to a database) and use include() for cosmetic ones. The *_once() versions provide for nice redundancy checking in complex applications, but they may be unnecessary in simple sites. Because of the way CSS works, if you don't use the CSS file or if the browser doesn't read the CSS, the generated result is still functional, just not aesthetically as pleasing (see Figure 3.6). Figure 3.6. This is the HTML layout without using the corresponding CSS file (compare with Figure 3.4). 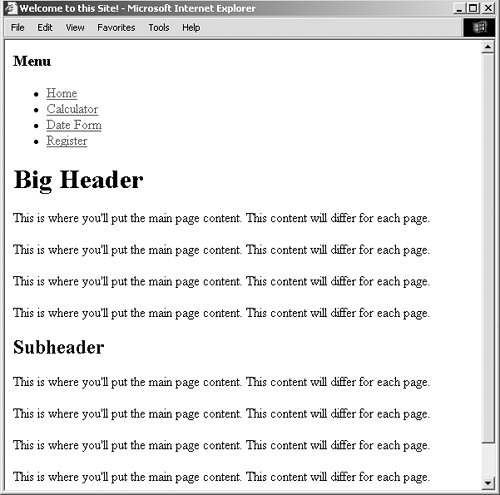
|