All of the examples in this chapter and the preceding one used two separate scripts for handling HTML forms: one that displayed the form and another that received it. While there's certainly nothing wrong with this method, there are advantages to having the entire process in one script. To have one page both display and handle a form, use a conditional. if (/* form has been submitted */) { // Handle it. } else { // Display it. } To determine if the form has been submitted, I normally check that a $_POST variable is set (assuming that the form uses the POST method, of course). For example, you can check $_POST['submitted'], assuming that's the name of a hidden input type in the form. if (isset($_POST['submitted'])) { // Handle it. } else { // Display it. } If you want a page to handle a form and then display it again (e.g., to add a record to a database and then give an option to add another), use if (isset($_POST['submitted'])) { // Handle it. } // Display the form. Using the preceding code, a script will handle a form if it has been submitted and display the form every time the page is loaded. To demonstrate this important technique, I'll start by creating a sales calculator. Later in this chapter this same method will be used with a registration page. To handle HTML forms 1. | Create a new PHP document in your text editor (Script 3.5).
<?php # Script 3.5 - calculator.php $page_title = 'Widget Cost Calculator; include ('./includes/header.html'); Script 3.5. The calculator.php script both displays a simple form and handles the form data, performing the calculations. 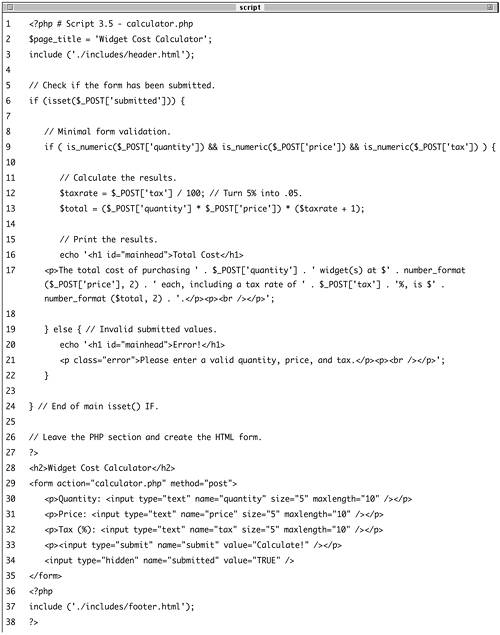 This, and all the remaining examples in the chapter, will use the same templating system as the index.php page. The beginning syntax of each page will therefore be the same, but the page titles will differ.
| 2. | Write the conditional for handling the form.
if (isset($_POST['submitted'])) { As I mentioned previously, if the $_POST['submitted'] variable is set, I know that the form has been submitted and I can process it. This variable will be created by a hidden input in the form that will act as a submission indicator.
| 3. | Validate the form.
if ( is_numeric($_POST['quantity']) && is_numeric($_POST['price]) && is_numeric($_POST['tax]) ) { The validation here is very simple: it merely checks that three submitted variables are all numeric types. You can certainly elaborate on this, perhaps checking that the quantity is an integer (in fact, in Chapter 10, "Web Application Security," you'll find a variation on this script that does just that).
If the validation passes all of the tests, the calculations will be made; otherwise, the user will be asked to try again.
| 4. | Perform the calculations.
$taxrate = $_POST['tax'] / 100; $total = ($_POST['quantity'] * $_POST['price]) * ($taxrate + 1); The first line changes the tax value from a percentage (say, 5%) to a decimal (.05), which will be needed in the subsequent calculation. The second line starts by multiplying the quantity times the price. This is then multiplied by the tax rate (.05) plus 1 (1.05). This last little trick is a quick way to calculate the total cost of something with tax (instead of multiplying the tax rate times the total and then adding this amount to the total).
| 5. | Print the results.
echo '<h1 >Total Cost</h1> <p>The total cost of purchasing ' . $_POST['quantity] . ' widget(s) at $' . number_format ($_POST ['price], 2) . ' each, including a tax rate of ' . $_POST['tax] . '%, is $' . number_format ($total, 2) . '.</p><p><br /></p>'; All of the values are printed out, formatting the price and total with the number_format() function.
| 6. | Complete the conditionals and close the PHP tag.
} else { echo '<h1 >Error!</h1> <p >Please enter a valid quantity, price, and tax.</p><p><br /></p>'; } } ?> The else clause completes the validation conditional, printing an error if the three submitted values aren't all numeric. The final closing curly brace closes the isset($_POST['submitted']) conditional. Finally, the PHP section is closed so that the form can be created without using print() or echo().
| 7. | Display the HTML form.
<h2>Widget Cost Calculator</h2> <form action="calculator.php" method="post> <p>Quantity: <input type="text" name="quantity size="5" maxlength="10 /></p> <p>Price: <input type="text" name="price size="5" maxlength="10 /></p> <p>Tax (%): <input type="text" name="tax size="5" maxlength="10 /></p> <p><input type="submit" name="submit value="Calculate!" /></p> <input type="hidden" name="submitted value="TRUE" /> </form> The form itself is fairly obvious, containing only two new tricks. First, the action attribute uses this script's name, so that the form submits back to this page instead of to another. Second, there is a hidden input called submitted with a value of TRUE. This is the flag variable whose existence will be checked to determine whether or not to handle the form (see the main condition).
| 8. | Include the footer file.
<?php include ('./includes/footer.html'); ?>
| 9. | Save the file as calculator.php, upload to your Web server, and test in your Web browser (Figures 3.7, 3.8, and 3.9).
Figure 3.7. The HTML form, upon first viewing it in the Web browser. 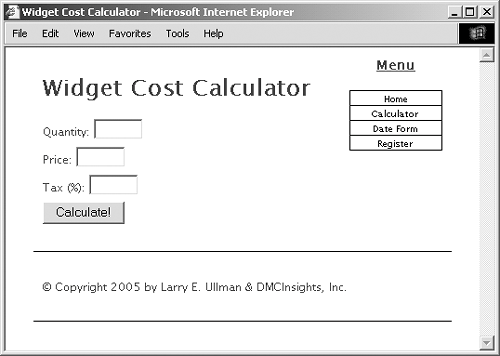 Figure 3.8. The page performs the calculations, reports on the results, and then redisplays the form. 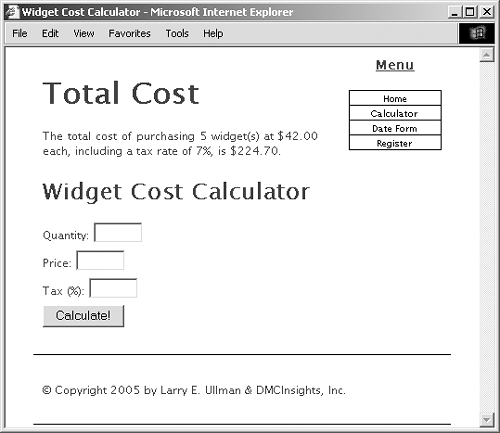 Figure 3.9. If one of the submitted values is not numeric, an error message is displayed. 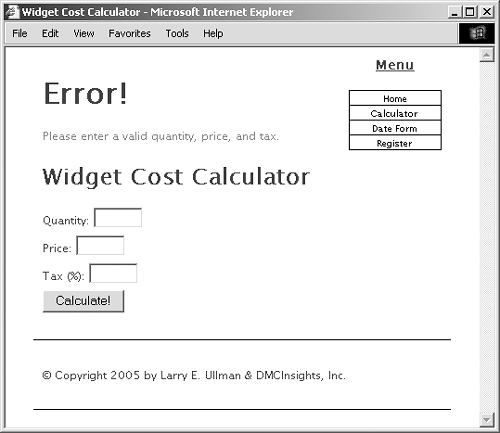
| Tips Another common method for checking if a form has been submitted is to see if the submit button's variable$_POST['submit'] hereis set. The only downside to this method is that it won't work if the user submits the form by pressing Return or Enter. If you use an image for your submit button, you'll also want to use a hidden input to test for the form's submission. You can also have a form submit back to itself by having PHP print the name of the current scriptstored in $_SERVER['PHP_SELF']as the action attribute. <form action="<?php echo $_SERVER['PHP_SELF']; ?>" method="post">
By doing so, the form will always submit back to this same page, even if you later change the name of the script.
|