If you've ever written any computer code, then you have had errors. Things never work exactly the way you plan, so you need contingency plans. With Ajax, the risks for things going wrong increases, because you cannot predict the reliability of the connection between visitors' computers and the Web server where the data is coming from. The code presented in the previous two sections assumes the data will arrive as planned. However, if it doesn't, the page simply waits obediently until the visitor gets bored and/or frustrated and then abandons the ship. You do not want that to happen. Building off the fetchData() function from the previous section ("Fetching a Response"), in this section we'll add the ability to specify another function to be used to process and filter the messages and data coming from the server and display the current status and final data response on the Web page. If there is a problem retrieving the data from the server, the function will alert visitors that something went wrong, rather than leave them hanging. In this example (Figure 20.7), when the page first loads, it immediately fetches a message from the server based on the value passed to the data page on the server. Figure 20.7. The text has been fetched from the server after the page loads. 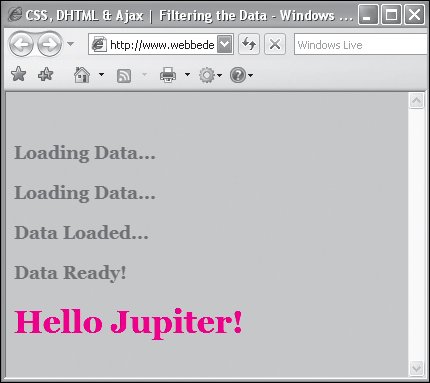 To filter a data response from the server: 1. | dataPage.php Create your data page and save it as dataPage.php (Code 20.3).
In this example, I'm using the same data page as in the previous section ("Fetching a Response").
| | | 2. | index.html Create an HTML file and save it as index.html (Code 20.5). The rest of the steps in this section apply to this file.
Code 20.5. index.html: The fetchData() function passes a value to the data page on the server and then passes the request object (pageRequest) to the function filterData() every time there is a change in the readyState. [View full width] <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR /xhtml1/DTD/xhtml1-strict.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8" /> <title>CSS, DHTML & Ajax | Filtering the Data</title> <style type="text/css" media="screen"> h1 { color: red; } h3 { color: #666; } </style> <script type="text/javascript"> function fetchData(url,dataToSend,objectID){ var pageRequest = false if (window.XMLHttpRequest) pageRequest = new XMLHttpRequest(); else if (window.ActiveXObject) pageRequest = new ActiveXObject("Microsoft.XMLHTTP"); else return false; pageRequest.onreadystatechange = function() { filterData(pageRequest,objectID); } if (dataToSend) { var sendData = 'sendData=' + dataToSend; pageRequest.open('POST',url,true); pageRequest.setRequestHeader('Content-Type', 'application/x-www-form-urlencoded'); pageRequest.send(sendData); } else { pageRequest.open('GET',url,true); pageRequest.send(null); } } function filterData(pageRequest,objectID){ var object = document.getElementById(objectID); if (pageRequest.readyState == 0) object.innerHTML += '<h3>Fetching Data… </h3>'; if (pageRequest.readyState == 1) object.innerHTML += '<h3>Loading Data… </h3>'; if (pageRequest.readyState == 2) object.innerHTML += '<h3>Data Loaded… </h3>'; if (pageRequest.readyState == 3) object.innerHTML += '<h3>Data Ready!</h3>'; if (pageRequest.readyState == 4) {if (pageRequest.status==200) object.innerHTML += pageRequest.responseText ; else if (pageRequest.status == 404) object.innerHTML += 'Sorry, that information is not currently available.'; else object.innerHTML += 'Sorry, there seems to be some kind of problem.'; } } </script> </head> <body onload="fetchData('dataPage.php',5,'message');"> <div id="message"> </div> </body></html> | | 3. | function fetchData (url, dataToSend, objectID) {…} Add the function fetchData() to a JavaScript block.
This function is identical to the fetchData() function presented in the previous section, but specifies the function to be executed when the request state changes.
For this example, I've instructed the function to trigger the filterData() function every time the state of the pageRequest object changes, passing to the function the pageRequest object and the objectID variable:
filterData(pageRequest, objectID);
| 4. | function filterData(pageRequest, objectID){…} Add the filterData() function to your JavaScript.
This function process the pageRequest object's current readyState value (Table 20.3) and acts accordingly. Steps 5 through 8 apply to this function.
Table 20.3. ReadyState ValuesVALUE | DESCRIPTION |
---|
0 | Uninitialized. The object has no data. | 1 | Loading. The object has data loading. | 2 | Loaded. The object has finished loading data. | 3 | Interactive. Data is still loading, but the object can be interacted with. | 4 | Complete. Data is fully loaded and ready for use. |
| 5. | var object = document.getElementById(objectID); Get the object that the data will eventually be placed into, based on the objectID variable.
| | | 6. | if (pageRequest.readyState == 0) Add conditionals to test for each of the possible ready states, and write a message into the HTML object.
For this example, I simply add a message specifying the current state:
object.innerHTML += '<h3>Fetching Data… </h3>'; Notice that I used plus and equal signs (+=) to append each message to the object, rather than replace it. I did this because the messages would pass so quickly in this example you would never see anything but the final results. Generally, you will want to replace += with a simple equal sign (=).
| 7. | if (pageRequest.readyState == 4) {…} For the fourth readyState value (at which point the data is ready for display), we need to do a bit more than display the results in our message object.
To display the results (responseText), we need to check that the status of the request is "OK" (status equals 200):
pageRequest.status==200
If the page is not found (status equals 404), we need to alert the visitor that the data isn't available:
pageRequest.status == 404
If all else fails, we need to display a message in case some other problem occurs.
| 8. | onload = "fetchData('dataPage.php', 5, 'message');" Add an event handler to your HTML to trigger the fetchData() function.
In this example, I set up an onload event handler in the body so that the function is triggered as soon as the page loads, passing it the URL for the page where my data is located (dataPage.php), the value being sent to the data page (in this case, hard coded as 5), and the ID for the object I want the results placed into (message).
| 9. | <div id="message"> </div> Finally, set up the object that will contain the final results fetched using our Ajax function from Step 3.
| Tip |