For the most part, once you create the fetchData() Ajax function, you can leave it alone. This function will form the workhorse of your Ajax code, but it is also general enough to handle a variety of situations. Building on the code from the previous sections in this chapter, we'll put together an external JavaScript file that can be included on any Web page that lets you access server pages. You can then create a control function to prepare the data before invoking the fetchData() function and a filterData() function tailored to the specific needs of the Web page in which it is included. In this example (Figure 20.8), a message pulled from a page on the server is displayed. Figure 20.8. The text has been fetched from the server using the code in the AjaxsBasics.js library file. 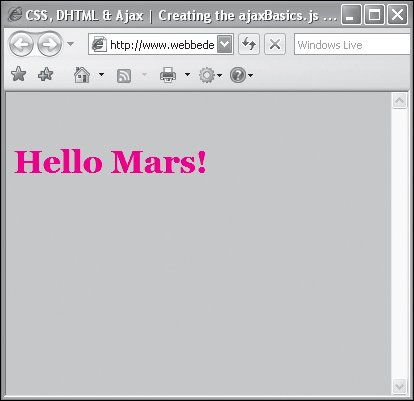 To set up a globally available Ajax function library: 1. | ajaxBasics.js Create a JavaScript file and save it as ajaxBasics.js (Code 20.6). This file will contain the final version of the fetchData() function presented in the previous section ("Filtering the Data").
Code 20.6. ajaxBasics.js: This external JavaScript file contains the fetchData() function so that you do not have to add it directly to every page in the site. [View full width] // ajaxBasics.js: Include this file when creating Ajax enabled functions. function fetchData(url,dataToSend,objectID){ var pageRequest = false if (window.XMLHttpRequest) pageRequest = new XMLHttpRequest(); else if (window.ActiveXObject) pageRequest = new ActiveXObject("Microsoft.XMLHTTP"); else return false; pageRequest.onreadystatechange = function() { filterData(pageRequest,objectID); } if (dataToSend) { var sendData = 'sendData=' + dataToSend; pageRequest.open('POST',url,true); pageRequest.setRequestHeader('Content-Type', 'application/x-www-form-urlencoded'); pageRequest.send(sendData); } else { pageRequest.open('GET',url,true); pageRequest.send(null); } } function filterData(pageRequest,objectID){ var object = document.getElementById(objectID); if (pageRequest.readyState == 4) { if (pageRequest.status==200) object.innerHTML = pageRequest.responseText ; else if (pageRequest.status == 404) object.innerHTML = 'Sorry, that information is not currently available.'; else object.innerHTML = 'Sorry, there seems to be some kind of problem.'; } else return; } | | 2. | dataPage.php Create your data page and save it as dataPage.php (Code 20.3).
In this example, I'm using the same data page as in the "To fetch a data response from the server," earlier in this chapter.
| | | 3. | index.html Create an HTML file and save it as index.html (Code 20.7). The rest of the steps in this section apply to this file.
Code 20.7. index.html: The prepData() function triggers the fetchData() function. [View full width] <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR /xhtml1/DTD/xhtml1-strict.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8" /> <title>CSS, DHTML & Ajax | Creating the ajaxBasics.js Library</title> <style type="text/css" media="screen"> h1 { color: red; } </style> <script src="ajaxBasics.js" type="text/javascript"></script> <script type="text/javascript"> function prepData(requestedData) { fetchData('dataPage.php', requestedData, 'message'); } </script> </head> <body onload="prepData(4);"> <div id="message"> </div> </body></html> | | 4. | <script src="ajaxBasics.js" type="text/javascript"></script Call the ajaxBasics.js file from Step 1. This will reference the code without having to embed it on every Web page it needs to be used with.
| 5. | function prepData(requestedData) {…} Add the prepData() function to your JavaScript.
This is the control function that invokes the fetchData() function located in the external JavaScript file ajaxBasics.js. Although you could bypass this and pass the information directly to the fetchData() function, you will often want to process the raw data coming from a function before sending it to the data page.
In this example, I hardcoded the URL data page and the ID for the object where the response is displayed, so that all the triggering function has to include is the value to be sent to the data page.
| 6. | function filterData(pageRequest, objectID){…} Add the filterData() function to your JavaScript.
This function is almost identical to the filterData() function presented in the previous section ("Filtering the Data"), but it removes the status updates for the state changes, which are generally not necessary except when you need to debug your code.
| | | 7. | onload="prepData(4);"> Add an event handler to your HTML to trigger the prepData() function.
In this example, I set up a simple onload event handler so that the function is triggered as soon as the page loads, passing it the value being sent to the data page (in this case, hardcoded as 4).
| 8. | <div id="message"> </div> Finally, set up the object that will contain the final results fetched using our Ajax function.
| Tip Ajax APIs and Design Patterns Ajax Patterns (ajaxpatterns.org): A wiki devoted to Ajax design patterns. The site contains a collection of public articles on everything Ajax. Yahoo! UI Library (developer.yahoo.com/yui/): A set of utilities and controls that lets you write Web-based applications that take advantage of existing Yahoo! functionality. Yahoo! Maps Web Services (developer.yahoo.com/maps/ajax/): lets you embed dynamic maps directly into your Web page. Google Search Ajax API (code.google.com/apis/ajaxsearch/): lets you integrate dynamic Google search directly into any Web page. |
|