To let the user set the value in the speed variable, Slapshot! uses the dialog box shown in Figure 2.2; the user can even open and use this dialog box when the game is playing. Figure 2.2. The Slapshot! application's dialog box. 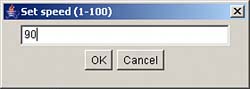
This dialog box is opened when the user selects the Set Speed… menu item, menuitem2. In the actionPerformed method, this item simply displays the dialog box texTDialog, an object of the OkCancelDialog class that was created in Slapshot!'s constructor. The user enters the new speed in the text field in the dialog box, which Slapshot! can recover using the dialog box's data member, converting the text the user enters to an integer using the Integer.parseInt method: [View full width] public class Slapshot extends Frame implements ActionListener, MouseListener, MouseMotionListener, Runnable { . . . OkCancelDialog textDialog; public void actionPerformed(ActionEvent e) { . . . if(e.getSource() == menuitem2){ textDialog.setVisible(true); if(!textDialog.data.equals("")){ int newSpeed = Integer.parseInt(textDialog.data); newSpeed = 101 - newSpeed; if(newSpeed >= 1 && newSpeed <= 100){ speed = newSpeed; } } } . . . }
So how do you create the OkCancelDialog dialog class? You can do that by extending the Java Dialog class when you create the OkCancelDialog class and by implementing the ActionListener interface so you can use clickable OK and Cancel buttons in the dialog box: class OkCancelDialog extends Dialog implements ActionListener { . . . } The AWT dialog boxes are based on the Dialog class, and you can see the significant methods of the Dialog class in Table 2.5. Table 2.5. The Significant Methods of the java.awt.Dialog ClassMethod | Does This |
---|
String getTitle() | Gets the title of the dialog | void hide() | Deprecated. Use the Component.setVisible (boolean) method instead | boolean isModal() | Returns true if this dialog is modal; returns false otherwise | boolean isResizable() | Returns true if this dialog may be resized by the user | void setModal(boolean b) | Specifies whether this dialog is modal | void setResizable(boolean resizable) | Specifies whether this dialog may be resized by the user | void setTitle(String title) | Specifies the title of this dialog | void show() | Deprecated. Use the Component.setVisible (boolean) method instead |
This dialog box sports an OK button, a Cancel button, and a text field where the user can enter the new game speed. Here's how those controls are set up: class OkCancelDialog extends Dialog implements ActionListener { Button ok, cancel; TextField text; public String data; OkCancelDialog(Frame hostFrame, String title, boolean dModal) { super(hostFrame, title, dModal); setSize(280, 100); setLayout(new FlowLayout()); text = new TextField(30); add(text); ok = new Button("OK"); add(ok); ok.addActionListener((ActionListener)this); cancel = new Button("Cancel"); add(cancel); cancel.addActionListener(this); data = new String(""); } . . . You can see the significant methods of the Button class in Table 2.6 and the significant methods of the TextField class in Table 2.7. Table 2.6. The Significant Methods of the java.awt.Button ClassMethod | Does This |
---|
void addActionListener(ActionListener l) | Adds the given action listener to the button so the listener will be notified of button events | ActionListener[] getActionListeners() | Returns an array of all action listeners that listen to this button | String getLabel() | Returns the text in the label of this button | void removeActionListener(ActionListener l) | Removes the given action listener, which means that it will not get events from this button | void setLabel(String label) | Sets the button's label to the string you pass this method |
Table 2.7. The Significant Methods of the java.awt.TextField ClassMethod | Does This |
---|
void addActionListener(ActionListener l) | Adds the given action listener to the text field so the listener will be notified of text field events | ActionListener[] getActionListeners() | Returns an array of all action listeners that listen to this text field | int getColumns() | Returns the number of columns currently in this text field | Dimension getMinimumSize() | Returns the minimum size allowed for this text field | Dimension getMinimumSize(int columns) | Returns the minimum size of a text field that has the number of columns you pass to this method | Dimension getPreferredSize() | Returns the text field's preferred size | Dimension getPreferredSize(int columns) | Returns the text field's preferred size, given the number of columns you pass to this method | Dimension minimumSize() | Deprecated. Use the getMinimumSize method instead | Dimension minimumSize(int columns) | Deprecated. Use the getMinimumSize(int) method instead | Dimension preferredSize() | Deprecated. Use the getPreferredSize method instead. | Dimension preferredSize(int columns) | Deprecated. Use the getPreferredSize(int) method instead. | void removeActionListener(ActionListener l) | Removes the given action listener, which means that it will not get events from this text field | void setColumns(int columns) | Sets the text field's number of columns | void setText(String t) | Sets the text that this text field will display |
When the user clicks the OK button, the dialog box stores the text from the text field in the member named data, or it stores an empty string in data if the user clicks the Cancel button: public void actionPerformed(ActionEvent event) { if(event.getSource() == ok){ data = text.getText(); } else { data = ""; } setVisible(false); } } Okay, you've gotten everything working nowexcept the pucks themselves, with their slide and drawPuckImage methods. And that's coming up next. |