In this section, we show you how to create a resource bundle. You create resource bundles for JSTL the same way you do for ordinary Java applications. Programmers create resource bundles for a variety of languages. For someone who is already familiar with English, it is quite easy to create a resource bundle for English or one of the other Romance languages. Non-Romance languages, such as the Asian languages, present some additional challenges, however. What Is a Resource Bundle? First, we'll describe a resource bundle and show you how to construct one. The method that we focus on involves creating compiled Java classes that extend the java.util.ListResourceBundle class. You must compile these class files and make them available to the classpath of your Web application. A Java resource bundle file contains a series of key-to-string mappings. The following demonstrates a simple Java resource bundle: package com.heaton.bundles; import java.util.*; public class Example extends ListResourceBundle { public Object[][] getContents() { return contents; } static final Object[][] contents = { {"count.one", "One"}, {"count.two", "Two"}, {"count.three", "Three"}, } } If you compiled this resource bundle and made it available to the classpath, you could use the following JSTL tags to display the three numbers: <fmt:setLocale value="en"/> <fmt:setBundle basename="com.heaton.bundles.Example" var="lang" scope="session"/> <fmt:message key="count.one" bundle="${lang}"/><br/> <fmt:message key="count.two" bundle="${lang}"/><br/> <fmt:message key="count.three" bundle="${lang}"/><br/> The power of resource bundles becomes apparent when you want to translate the site to another language. To accomplish this, you need only provide another resource bundle. You make no changes to the JSTL code; you only have to specify a different locale. The following code shows how the short resource bundle can be modified for Spanish: package com.heaton.bundles; import java.util.*; public class Example_es extends ListResourceBundle { public Object[][] getContents() { return contents; } static final Object[][] contents = { {"count.one", "Uno"}, {"count.two", "Dos"}, {"count.three", "Tres"}, } } As you can see, the name of the resource bundle has changed. Previously, the resource name was Example; now the name is Example_es. This is because the code es represents Spanish. Now a user can change the locale to Spanish, and the Spanish strings appear as follows: <fmt:setLocale value="es"/> Creating a Properties File Resource Bundle It is also possible to create a resource bundle using a properties file. A properties file in Java should contain the same base name as a compiled resource. For example, the forum's English properties file should be stored in a file named forum_en.properties. The format of a properties file is simple. The entire file consists of lines that are keys to the text data. The following code illustrates a simple properties file: # this is a comment property1 = value of property 1 property2 = value of property 2 property3 = value of property 3 Users access this file in the same way they would a compiled resource. The base name is used to identify the file. The suffix to this file must always be .properties for example, forum_en.properties. Creating a Resource Bundle for a Romance Language In this section, we show you how we created the resource bundles for our forum application. We first look at constructing a resource bundle for a Romance language. As you may already know, a Romance language is one based on Latin. Languages such as English, French, Italian, and Spanish all fall into this category. Romance languages use basically the same character set, known as the Latin character set. Because Java uses the Latin character set for source code, it is always easier to deal with a Romance language than a non-Romance language, such as Chinese. The resource bundles containing the English translation are Forum.java and Forum_en.java. Forum.java is the base translation that a program will use if it does not find the translation in the localized resource bundle. Listing 10.1 shows the English resource bundle used by our forum example. Listing 10.1 An English Resource Bundle (Forum.java)package com.heaton.bundles; import java.util.*; public class Forum extends ListResourceBundle { public Object[][] getContents() { return contents; } static final Object[][] contents = { {"lang.english", "English"}, {"lang.spanish", "Spanish"}, {"lang.chinese", "Chinese"}, {"button.ok", "OK"}, {"button.save", "Save"}, {"button.add", "Add"}, {"button.cancel", "Cancel"}, {"button.login", "Login"}, {"button.register", "Register"}, {"word.welcome", "Welcome"}, {"word.password", "Password"}, {"word.continue", "Continue"}, {"word.admin", "Administrator"}, {"word.exit", "Exit"}, {"word.action", "Action"}, {"word.user", "User"}, {"word.type", "Type"}, {"word.edit", "Edit"}, {"word.delete", "Delete"}, {"word.register", "Register"}, {"type.a","Admin User"}, {"type.g","Guest User(can't post)"}, {"type.r","Regular User"}, {"login.name", "Login Name"}, {"login.welcome","Welcome to the forum. Please login."}, {"login.title","Login to Forum."}, {"login.note","Note: use id of admin and password of admin on first use. "}, {"login.badpw","Sorry, we have no one registered with that name."}, {"reg.thanks","Thanks for joining the forum. Please choose a login name and a password. Please enter your password twice to verify."}, {"reg.verify","Verify Password"}, {"reg.needlogin","Must enter a login id!"}, {"reg.needpw","Must enter a password!"}, {"reg.badpw","Passwords do not match!"}, {"welcome.id","Login ID"}, {"welcome.times","Times on"}, {"welcome.first","First Login"}, {"welcome.last","Last Login"}, {"welcome.failed","Failed Logins"}, {"welcome.posted","Messages Posted"}, {"welcome.type","User Type"}, {"main.welcome","Select one of the following forums. Each form contains messages on the given topic."}, {"main.please","Please select a forum."}, {"main.forumdeleted","Forum Deleted."}, {"admin.welcome1","From this screen you can edit forums, delete forums or create new forums."}, {"admin.welcome2","You may also edit users."}, {"admin.prompt","The following forums are available:"}, {"admin.newforum","New Forum"}, {"admin.editusers","Edit Users"}, {"editforum.title","Edit Forum"}, {"editforum.ins1","Forum code is a unique code for this forum."}, {"editforum.ins2","Sequence number determines the order forums will list."}, {"editforum.code","Forum Code"}, {"editforum.name","Forum Name"}, {"editforum.number","Sequence Number"}, {"newforum.title","Add New Forum"}, {"editusers.title","Edit Users"}, {"editusers.ins","The following users exist in your forum."}, {"editusers.deleted","User deleted."}, {"edituser.title","User Editor"}, {"edituser.ins","This page allows you to edit a user. The only attribute you can change is the user's type."}, {"forum.none","No messages posted yet."}, {"forum.post","Post a new message"}, {"forum.from","From:"}, {"forum.date","Date:"}, {"forum.subject","Subject:"}, {"post.ins","Posting to forum:"}, {"post.title","Post Message"} }; } As you can see in Listing 10.1, every string ever displayed in the forum application has been segregated to this file. The forum example will now refer to this file to get the text when English is the current language. Listing 10.2 shows the forum application translated to Spanish. I apologize in advance for the translation. It was made through a combination of a Spanish dictionary, a Spanish computer dictionary, and AltaVista's Babelfish. While the translation may not be perfect, it does demonstrate how to load another Romance language as a resource bundle. TIP Babelfish, which you can find at http://world.altavista.com/, is a useful Internet-based translation service. For a quick prototype translation, you'll find Babelfish useful. However, using AltaVista's translation system on a production system can result in sentences being interpreted incorrectly. Here's an example: "The spirit is willing but the flesh is weak" translated to Spanish gives us, "El alcohol est dispuesto, pero la carne es d bil." Translating this Spanish back to English should give the original sentence, yet here's the result: "The alcohol is arranged but the meat is weak." As you can see, the service cannot determine which synonyms to use in place of spirit and flesh. Listing 10.2 A Spanish Resource Bundle (Forum_es.java)package com.heaton.bundles; import java.util.*; public class Forum_es extends ListResourceBundle { public Object[][] getContents() { return contents; } static final Object[][] contents = { {"lang.english", "Ingleses"}, {"lang.spanish", "Espa ol"}, {"lang.chinese", "Chinois"}, {"button.ok", "Ok"}, {"button.save", "Guardar"}, {"button.add", "Ampliar"}, {"button.cancel", "Cancelar"}, {"button.login", "Hacer la conexi n"}, {"button.register", "Registro"}, {"word.welcome", "Bienvenue"}, {"word.password", "Contrase a"}, {"word.continue", "Seguir"}, {"word.admin", "Operador del Sistema"}, {"word.exit", "Aalir"}, {"word.action", "Hacer"}, {"word.user", "Persona"}, {"word.type", "Tipo"}, {"word.edit", "Corrigen"}, {"word.delete", "Eliminar"}, {"word.register", "Registro"}, {"type.a","Operador del Sistema"}, {"type.g","Persona Invitado"}, {"type.r","Persona Regular"}, {"login.name", "Su nombre de usuario"}, {"login.welcome","Recepci n al foro. Por favor conexi n."}, {"login.title","Recepci n al foro. Por favor conexi n. "}, {"login.note"," Employez l'identification de l'admin et du mot de passe de l'admin sur la premi re utilisation."}, {"login.badpw","Apesadumbrados, tenemos nadie registrados con ese nombre."}, {"reg.thanks","Gracias por ensamblar el foro. Elija por favor un nombre de la conexi n y una palabra de paso. Incorpore por favor su palabra de paso dos veces para verificar."}, {"reg.verify","V rifiez Le Mot de passe"}, {"reg.needlogin","Debe incorporar una identificaci n de la conexi n!"}, {"reg.needpw","Debe incorporar una palabra de paso!"}, {"reg.badpw","Las palabras de paso no corresponden con!"}, {"welcome.id","Identificaci n De la Conexi n"}, {"welcome.times"," pocas encendido"}, {"welcome.first","Premi re Ouverture"}, {"welcome.last","Conexi n Pasada"}, {"welcome.failed","Falladas Conexiones"}, {"welcome.posted","Los Mensajes Fijaron"}, {"welcome.type","Tipo Del Utilizador"}, {"main.welcome","Seleccione uno de los foros siguientes. Cada forma contiene mensajes en el asunto dado."}, {"main.please","Seleccione por favor un foro."}, {"main.forumdeleted","Foro Suprimido."}, {"admin.welcome1","De esta pantalla usted puede corregir foros, suprimir foros o crear foros nuevos."}, {"admin.welcome2","Usted puede tambi n corregir a usuarios. "}, {"admin.prompt","Los foros siguientes est n disponibles: "}, {"admin.newforum","Foro Nuevo"}, {"admin.editusers"," ditez Les Utilisateurs"}, {"editforum.title","Corrija El Foro"}, {"editforum.ins1","El c digo del foro es un c digo nico para este foro."}, {"editforum.ins2","El n mero de serie determina los foros de la orden enumerar ."}, {"editforum.code","C digo Del Foro"}, {"editforum.name","Nom De Forum"}, {"editforum.number","N mero De Serie"}, {"newforum.title","Ajoutez Le Nouveau Forum"}, {"editusers.title","Corrija A Utilizadores"}, {"editusers.ins","Los utilizadores siguientes existen en su foro."}, {"editusers.deleted","Utilizador suprimido."}, {"edituser.title","Redactor Del Usuario"}, {"edituser.ins","Esta paginaci n permite que usted corrija a un utilizador. El nico atributo que usted puede cambiar es el tipo del utilizador. "}, {"forum.none","Ningunos mensajes fijados con todo."}, {"forum.post","Fije un nuevo mensaje"}, {"forum.from","De:"}, {"forum.date","Fecha:"}, {"forum.subject","Tema:"}, {"post.ins","Posting to forum:"}, {"post.title","Mensaje Del Poste"} }; } As you can see in Listing 10.2, every string in the resource bundle has been translated to a Spanish string. This is an ordinary text file that you can edit using Notepad, vi, or another editor. Creating a Resource Bundle for a Non-Romance Language We'll now show you how to translate a resource bundle into a non-Romance language. For this example, we use the Chinese language. NOTE The method I used to translate this file to Chinese is typical of how you might translate actual resource bundles. I used a third party; the translation was done by Jenny Yao, a student in one of the Java classes that I teach. I provided Jenny with only the English translation of the Forum.java file. After a couple of emails to verify the correct meanings of some terms, she emailed me a version of my resource bundle translated to Chinese. This is an important feature of JSTL. It is only necessary to provide your translator with the resource bundle; you do not need to provide any of your Web application's source code. This has several benefits. For example, you don't have to worry about your translator inadvertently changing some of the JSTL code and causing an error. In addition, keeping your JSTL code inside your company and not disclosing it to third-party translation services adds a layer of security. The Chinese version of the resource bundle is contained in the file Forum_zh.java. You cannot simply open this file with Notepad to view it. If you're using Windows, we suggest that you use WordPad to view the file, as shown in Figure 10.1. You can obtain this file from the Sams Web site. 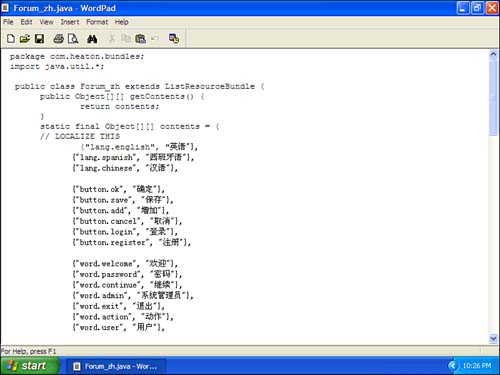 It is also important to note that you must have the Chinese fonts installed in order for WordPad to display your file correctly. In Windows XP, select Settings, Control Panel, Regional and Language Options. In the resulting dialog box, click the Advanced tab and select the option Simplified Chinese, as shown in Figure 10.2. 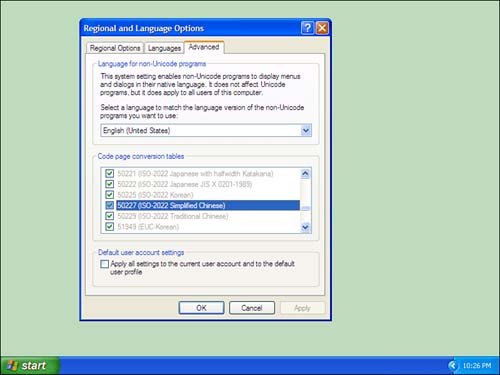 Compiling the Resource Bundles Now that we've created four resource bundles to use with our forum application, we must compile the bundles. Listing 10.3 contains a simple build script named build.sh (build.bat for Windows) that will build these files. The same script file will work under either Windows or Unix. Listing 10.3 A Resource Bundle Build Script (build.sh)javac -encoding UTF-16 ./com/heaton/bundles/Forum_zh.java javac ./com/heaton/bundles/Forum_es.java javac ./com/heaton/bundles/Forum.java javac ./com/heaton/bundles/Forum_en.java jar cvf bundle.jar ./com/heaton/bundles/*.class As you can see, the Chinese file, Forum_zh.java, is given special handling. This is because it cannot be compiled by Java unless we specify the encoding. For this file, we used UTF-16 encoding, as revealed in the first line of Listing 10.3. If all goes well, this script creates a file named bundle.jar, which you should place in Tomcat's lib directory so that it is exposed to the classpath. You can download a zipped version of the Tomcat root directory from the Sams Web site; this version has all of the files in the correct locations for every example in this book. |