A Multilingual Forum Application You should now have the necessary resource bundles for our forum application. Let's see how to convert the forum example shown in Chapter 7 to a multilingual application. The majority of the work involves changing all the English text to <fmt:message> tags. We won't show you every converted JSP file; rather, we'll just examine the index.jsp file in order to demonstrate how to use the <fmt:message> tags. The index page for our forum application now allows you to easily switch between the three languages. The default language, English, is shown in Figure 10.3. 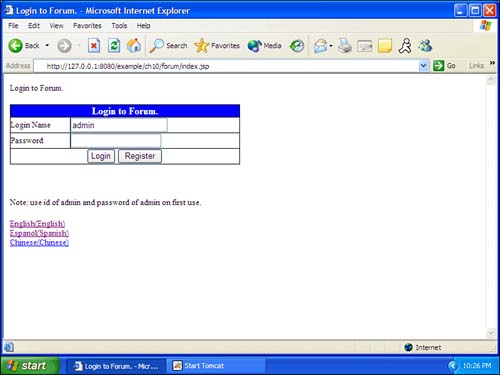 Simply clicking on the Spanish link will cause the site to redisplay in Spanish. Figure 10.4 shows this transformation. 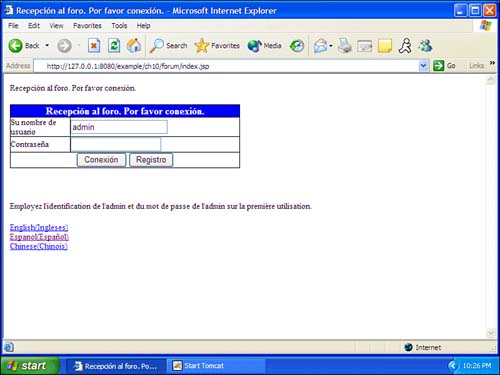 If you click the Chinese link, the site will be displayed in Chinese. You'll notice that the Chinese characters display just fine in the text regions and buttons. However, those characters do not display properly in the browser's title bar. This is because very few browsers can correctly display Chinese characters contained in the <title></title> tags of a page. Romance languages will display just fine. Because of this, you may want to replace all the HTML <title> tags with generic English titles that will not be translated. Figure 10.5 shows the example running in Chinese. 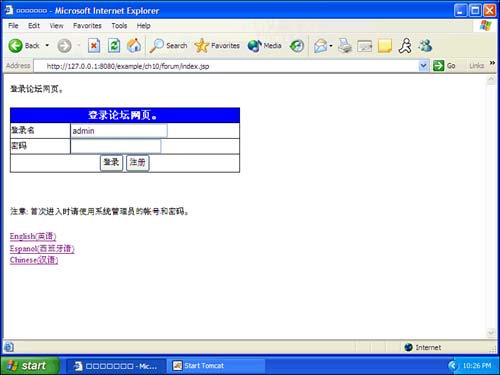 Now that you've seen how the forum looks in each of the three languages, let's examine how this was actually done. The only file that changes significantly is the index.jsp file. The other files change only in that they now specify UTF-8 as the character encoding, and they use <fmt:format> to display strings. Listing 10.4 shows the index.jsp file from Chapter 7, rewritten for multilingual processing. Listing 10.4 A Multilingual index.jsp (index.jsp)<%@ page contentType="text/html; charset=UTF-8" %> <%@ taglib uri="http://java.sun.com/jstl/fmt" prefix="fmt" %> <%@ taglib uri="http://java.sun.com/jstl/core" prefix="c" %> <%@ taglib uri="http://java.sun.com/jstl/core-rt" prefix="c-rt" %> <%@ taglib uri="http://java.sun.com/jstl/sql" prefix="sql" %> <c:if test="${dataSource==null}"> <sql:setDataSource var="dataSource" driver="org.gjt.mm.mysql. Driver" url="jdbc:mysql://localhost/forum?user=forumuser" scope="session" /> </c:if> <c:if test="${lang==null}"> <fmt:setBundle basename="com.heaton.bundles.Forum" var="lang" scope="session"/> </c:if> <c:if test="${param.lang!=null}"> <fmt:setLocale value="${param.lang}"/> <fmt:setBundle basename="com.heaton.bundles.Forum" var="lang" scope="session"/> <c:redirect url="index.jsp"/> </c:if> <c:if test="${pageContext.request.method=='POST'}"> <c:if test="${param.reg!=null}"> <c:redirect url="register.jsp" /> </c:if> <sql:query var="users" dataSource="${dataSource}">select c_ uid,c_type from t_users where c_uid = ? and c_pwd = ? <sql:param value="${param.uid}" /> <sql:param value="${param.pwd}" /> </sql:query> <c:choose> <c:when test="${users.rowCount<1}"> <h3 color="red"><fmt:message key="login.badpw" bundle="${lang}"/></h3> <sql:update var="result" dataSource="${dataSource}">update t_users set c_bad = c_bad + 1 where c_uid = ? <sql:param value="${param.uid}" /> </sql:update> </c:when> <c:otherwise> <% Cookie mycookie = new Cookie("login",request. getParameter("uid")); mycookie.setMaxAge(0x7ffffff); response.addCookie(mycookie); %> <c:forEach var="aUser" items="${users.rows}"> <c:set var="userID" value="${aUser.c_uid}" scope="session" /> <c:set var="userType" value="${aUser.c_type}" scope="session" /> </c:forEach> <c:redirect url="welcome.jsp" /> </c:otherwise> </c:choose> </c:if> <c-rt:if test="<%=request.getCookies()!=null%>"> <c-rt:forEach var="aCookie" items="<%=request.getCookies()%>"> <c:if test="${aCookie.name=='login'}"> <c:set var="uid" value="${aCookie.value}" /> </c:if> </c-rt:forEach> </c-rt:if> <html> <head> <title><fmt:message key="login.title" bundle="${lang}"/></title> </head> <body> <fmt:message key="login.welcome" bundle="${lang}"/><br> <form method="POST"> <table border="1" cellpadding="0" cellspacing="0" style="border-collapse: collapse" bordercolor="#111111" width="49%" > <tr> <td width="100%" colspan="2" bgcolor="#0000FF"> <p align="center"> <b> <font color="#FFFFFF" size="4"> <fmt:message key="login.title" bundle="${lang}"/> </font> </b> </p> </td> </tr> <tr> <td width="26%"><fmt:message key="login.name" bundle="${lang}"/></td> <td width="74%"> <input type="text" name="uid" value="<c:out value="${uid}"/>" size="20" /> </td> </tr> <tr> <td width="26%"> <fmt:message key="word.password" bundle="${lang}"/></td> <td width="74%"> <input type="password" name="pwd" size="20" /> </td> </tr> <tr> <td width="100%" colspan="2"> <p align="center"> <input type="submit" value="<fmt:message key="button.login" bundle="${lang}"/>" name="Login" /> <input type="submit" value="<fmt:message key="button.register" bundle="${lang}"/>" name="reg" /> </p> </td> </tr> </table> <p> </p> </form> <p><fmt:message key="login.note" bundle="${lang}"/> </p> <a href="index.jsp?lang=en">English(<fmt:message key="lang.english" bundle="${lang}"/>)</a><br> <a href="index.jsp?lang=es">Espanol(<fmt:message key="lang.spanish" bundle="${lang}"/>)</a><br> <a href="index.jsp?lang=zh">Chinese(<fmt:message key="lang.chinese" bundle="${lang}"/>)</a><br> </body> </html> Notice the first line of the file: <%@ page contentType="text/html; charset=UTF-8" %> This line specifies that the file is using UTF-8 encoding. Without this line, the Chinese characters will not display correctly. To switch between the languages, we've provided several hyperlinks near the bottom that will take you back to the index file with the language value contained in the parameter param. This will allow the following code to correctly change the language: <c:if test="${lang==null}"> <fmt:setBundle basename="com.heaton.bundles.Forum" var="lang" scope="session"/> </c:if> First, we check to see whether any language bundle is loaded. This handles the default first view when English is selected. The above line loads the bundle into the scoped variable lang: <c:if test="${param.lang!=null}"> <fmt:setLocale value="${param.lang}"/> <fmt:setBundle basename="com.heaton.bundles.Forum" var="lang" scope="session"/> <c:redirect url="index.jsp"/> </c:if> Next, we check to see whether a new language was specified. If a language was specified, we set it to the current locale. We then reload the resource bundle, reflecting the change in locale. Finally, to make the browser redisplay the index page, we redirect to that page using a <c:redirect> tag. |