Most JSTL tags operate on scoped variables. You create JSTL variables by using the set command. A typical set command is shown here: <c:set var="variableName" value="the value"/> This command assigns the value the value to the scoped variable, which is named variableName . If you are familiar with JSP scriptlet programming, you may be interested to know that the scoped variables in JSTL are stored by the same mechanism as JSP scriptlet scoped variables. Just as in JSP scriptlet programming, these variables are stored using the javax.servlet.jsp.PageContext class. Because of this, JSTL scoped variables are accessible to JSP using the PageContext class. Because JSTL variables are called scoped variables, the concept of scope must enter the picture at some point. The programming term scope refers to the scope for which a variable is valid. In Java, the scope of an automatic, or local, variable is only within the function that initializes the variable. In the following code snippet, the variable i 's scope is only within the mymethod() method: void mymethod() { int i; for(i=0;i<10;i++) { System.out.println("Count:"+i); } } However, JSTL does not use methods. The scope of a JSTL variable cannot be determined from its context in the same way a Java variable can. Therefore, you must specify the scope of every variable you create. If you fail to specify the scope for a variable, that variable's scope will default to page-level scope. The following tag will create a variable named variableName for session-level scope: <c:set var="variableName" value="the value" scope="session"/> JSTL and scriptlet code support four different levels of scope the same four levels of scope that JSP provides. These four levels are page scope, request scope, session scope, and application scope. Table 2.1 summarizes these scopes. Table 2.1. Variable Scopes Page | For the current page | Very low | Request | For the current request | Low | Session | For the current session | High | Application | Accessible everywhere | Low | Let's examine each level of scope and the differences among them. We begin with page scope. Page Scope Page scope is the closest that JSTL comes to automatic, or local, variables. If a variable is created with page scope, its value is valid only inside the current page. If the current page includes (using the JSP include directive) other pages, the page-scoped variable will not be available in these included pages. Further, the page-scoped variable loses its value after the page is displayed to the user. If this user requests that the same page be loaded again, the page-scoped variables are reset. Page-scoped variables are specific to each load, or display, of the page. They should be used whenever possible because they present the least amount of overhead to the Web server. Programmers typically use page-scoped variables to hold temporary counters and other variables while a page processes. To create a page-scoped variable, use the following syntax: <c:set var="scopeVarPage" value="Page Value" scope="page" /> The scope attribute specified on the previous line is optional because page is the default scope. Let's look at a sample program that demonstrates the differences in page, request, session, and application scopes. Listing 2.1 shows the main page for the scope example. Listing 2.1 The Main Scoped Sample Index (index.jsp)<%@ taglib uri="http://java.sun.com/jstl/core" prefix="c" %> <c:set var="scopeVarPage" value="Page Value" scope="page" /> <c:set var="scopeVarRequest" value="Request Value" scope="request" /> <c:set var="scopeVarSession" value="Session Value" scope="session" /> <c:set var="scopeVarApplication" value="Application Value" scope="application" /> <html> <head> <title>Scope Example</title> </head> <body> <h3>Main File: index.jsp</h3> <table border="1"> <tr> <th>Scoped Variable</th> <th>Current Value</th> </tr> <tr> <td> <b>Page Scope</b> (scopeVarPage)</td> <td>  <c:out value="${scopeVarPage}" /> </td> </tr> <tr> <td> <b>Request Scope</b> (scopeVarRequest)</td> <td>  <c:out value="${scopeVarRequest}" /> </td> </tr> <tr> <td> <b>Session Scope</b> (scopeVarSession)</td> <td>  <c:out value="${scopeVarSession}" /> </td> </tr> <tr> <td> <b>Application Scope</b> (applicationVarPage)</td> <td>  <c:out value="${scopeVarApplication}" /> </td> </tr> </table> <br /> <br /> <jsp:include page="included.jsp" /> <br /> <br /> <a href="linked.jsp">[Click Here to View: linked.jsp]</a> </body> </html> When the user first views Listing 2.1, four scoped variables are created. The variables scopeVarPage , scopeVarRequest, scopeVarSession,and scopeVarApplication are each defined with their respective scope. Next, the values of each variable are displayed when the user first loads this page. This sample program works by displaying these four variables at different stages in the program. The scope of a variable can be determined by seeing how long it holds its values. The main index.jsp page shown in Listing 2.1 uses the following JSP include directive: <jsp:include page="included.jsp" /> Often, you will find that you want to reuse JSP code. To allow code to be shared, JSP includes a directive that allows a page to insert the code of another JSP page. To the user, this is seamless, and the included page's HTML is inserted directly into the page that contains the include statement. Of course, an included file can contain JSP. Listing 2.2 shows an example called included.jsp. As you can see, Listing 2.2 displays the values. This allows you to see which of the scoped variables hold their value during the include. Observe that the page-scoped variable loses its value, as seen in Figure 2.1. Listing 2.2 The Included Scoped Example (included.jsp)<%@ taglib uri="http://java.sun.com/jstl/core" prefix="c" %> <table border="1"> <tr> <th>Scoped Variable</th> <th>Current Value</th> </tr> <tr> <td> <b>Page Scope</b> (scopeVarPage)</td> <td>  <c:out value="${scopeVarPage}" /> </td> </tr> <tr> <td> <b>Request Scope</b> (scopeVarRequest)</td> <td>  <c:out value="${scopeVarRequest}" /> </td> </tr> <tr> <td> <b>Session Scope</b> (scopeVarSession)</td> <td>  <c:out value="${scopeVarSession}" /> </td> </tr> <tr> <td> <b>Application Scope</b> (applicationVarPage)</td> <td>  <c:out value="${scopeVarApplication}" /> </td> </tr> </table> 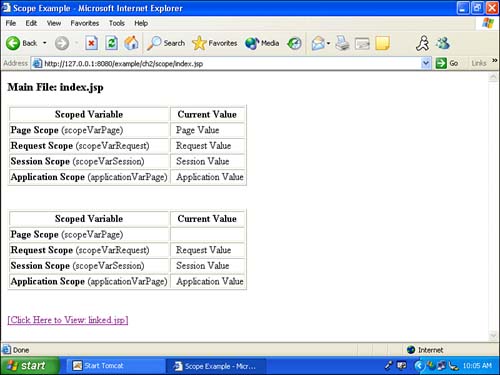 The index.jsp file shown in Listing 2.1 also gives the user the opportunity to link to a second page using a hyperlink. The following code provides a link to a second JSP page called linked.jsp, which is shown in Listing 2.3: <a href="linked.jsp">[Click Here to View: linked.jsp]</a> Moving to the linked page allows you to see which variables hold their values. Observe that the page-scoped variable loses its value on a linked page as well, as seen in Figure 2.2. Listing 2.3 The Linked Scoped Example (linked.jsp)<%@ taglib uri="http://java.sun.com/jstl/core" prefix="c" %> <html> <head> <title>Scope Example</title> </head> <body> <h3>Linked File: linked.jsp</h3> <table border="1"> <tr> <th>Scoped Variable</th> <th>Current Value</th> </tr> <tr> <td> <b>Page Scope</b> (scopeVarPage)</td> <td>  <c:out value="${scopeVarPage}" /> </td> </tr> <tr> <td> <b>Request Scope</b> (scopeVarRequest)</td> <td>  <c:out value="${scopeVarRequest}" /> </td> </tr> <tr> <td> <b>Session Scope</b> (scopeVarSession)</td> <td>  <c:out value="${scopeVarSession}" /> </td> </tr> <tr> <td> <b>Application Scope</b> (applicationVarPage)</td> <td>  <c:out value="${scopeVarApplication}" /> </td> </tr> </table> </body> </html> 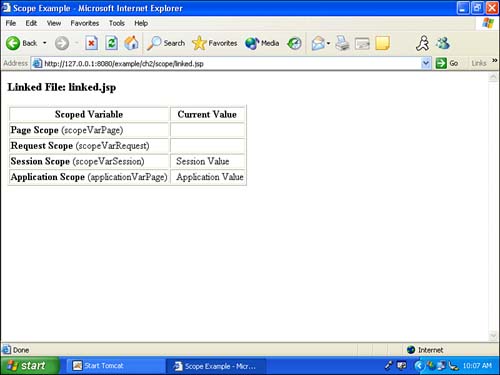 Figures 2.1 and 2.2 show the output from these two pages. The browser in Figure 2.1 is viewing the index.jsp page, which includes both Listings 2.1 and 2.2. Figure 2.2 is showing only linked.jsp (Listing 2.3). As you can see, page scope is somewhat limited. On the other hand, the request scope, which we examine next, holds its values over a somewhat larger scope. Request Scope A variable that is created with request scope will remain valid for the entire request. A request begins when a Web browser requests a single page, and ends when the Web server has transmitted that page. If you examine Figure 2.1, you will note that the request-scoped variable maintains its value in the included page, yet does not hold its value to the linked page. This is because the linked page is covered by a second request. When users click the hyperlink that takes them to the linked page, that click is a second request. Request-scoped variables do not introduce much additional overhead beyond the page-scoped variables. If you need to store variables that will be accessible to pages imported with the include statement, you must use request-scoped variables. The following line of code creates a request-scoped variable: <c:set var="scopeVarRequest" value="Page Value" scope="request" /> Session Scope Session-scoped variables remain valid for the duration of the current Web session. These variables are tied to individual sessions. As you can see in Figures 2.1 and 2.2, the session-scoped variables keep their values both in included files and linked files. To fully understand session-scoped variables, you need to know exactly what a session is. In the following lines of code, we created a cookie named JSESSIONID. This cookie will be transmitted back to the Web server with every request made by this client. The cookie allows the Web server to tie a user's browser to that user's session. HTTP/1.1 200 OK Content-Type: text/html;charset=ISO-8859-1 Connection: close Date: Sun, 14 Apr 2002 19:01:40 GMT Server: Apache Tomcat/4.0.3 (HTTP/1.1 Connector) Set-Cookie: JSESSIONID=960A80E2D931F2D671843E6D6EC8A834;Path=/ A session is an area of memory that stores values for each currently logged-in Web browser. Session-scoped variables are stored in this area. The following line of code creates a session-scoped variable: <c:set var="scopeVarSession" value="The Value" scope="session" /> A session ends when one of two things happens. First, a JSP page can specifically terminate a session by calling the HttpSession class's invalidate() method. Second, a JSP page can terminate a session using a timeout, which is the method by which most sessions are ultimately terminated. If the session is not used for a predefined amount of time, the session will be discarded. By default, Tomcat uses a 30-minute timeout, but you can change this value in the web.xml file. Use the following lines to set the timeout value: <!-- ==================== Default Session Configuration ======= --> <!-- You can set the default session timeout (in minutes) for --> <!-- all newly created sessions by modifying the value below. --> <session-config> <session-timeout>30</session-timeout> </session-config> Because the Web server must allocate memory for each current Web session, session-scoped variables require considerable overhead. Over-dependence on session-scoped variables can considerably increase the processing needs of your Web application and can lead to degraded performance. Application Scope Application-scoped variables are as close as JSTL comes to global variables. When you create an application-scoped variable, it can be accessed from any page and any browser session. This makes application-scoped variables useful for information that must be shared at a global level by JSP pages. If you look at Figures 2.1 and 2.2, you will notice that application-scoped variables are viewable from all three pages. Notice in Figure 2.1 that session-scoped variables are also accessible in all three locations. So, now we must consider what separates application-scoped variables from session-scoped variables. If you open a new browser and go directly to the linked page, you will see the difference between session and application variables. By going directly to the linked file, http://127.0.0.1:8080/example/ch2/scope/linked.jsp, you bypass the variables being set near the beginning of the index.jsp file. Figure 2.3 shows the results of this direct navigation from a second browser. 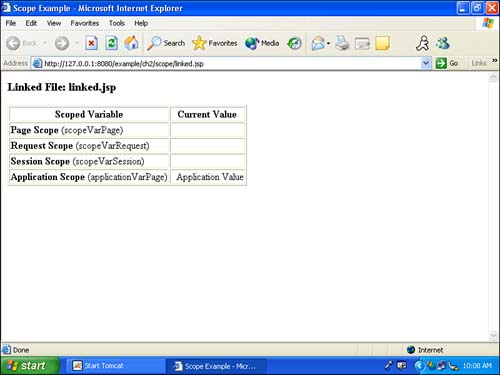 Notice that the only variable that is set in Figure 2.3 is the application-scoped variable. This is because your new Web browser created a new session. This new session is separate from the previous session; as a result, no session-scoped variables are kept. However, the application-scoped variable remains. This variable will keep its value for every Web session on the Web server. However, if the Web server is restarted, the application-scoped variable will lose its value. Application-scoped variables consume little overhead because they need to be stored only once and are global to the entire Web application. To create an application-scoped variable, use the following line of code: <c:set var="scopeVarApplication" value="The Value" scope="application" /> |