The MEDIATOR pattern also imposes policy. However, whereas FACADE imposes its policy in a visible and constraining way, MEDIATOR imposes its policies in a hidden and unconstraining way. For example, the QuickEntryMediator class in Listing 23-1 sits quietly behind the scenes and binds a text-entry field to a list. When you type in the text-entry field, the first list element that matches what you have typed is highlighted. This lets you type abbreviations and quickly select a list item. Listing 23-1. QuickEntryMediator.cs using System; using System.Windows.Forms; /// <summary> /// QuickEntryMediator. This class takes a TextBox and a /// ListBox. It assumes that the user will type /// characters into the TextBox that are prefixes of /// entries in the ListBox. It automatically selects the /// first item in the ListBox that matches the current /// prefix in the TextBox. /// /// If the TextField is null, or the prefix does not /// match any element in the ListBox, then the ListBox /// selection is cleared. /// /// There are no methods to call for this object. You /// simply create it, and forget it. (But don't let it /// be garbage collected...) /// /// Example: /// /// TextBox t = new TextBox(); /// ListBox l = new ListBox(); /// /// QuickEntryMediator qem = new QuickEntryMediator(t,l); /// // that's all folks. /// /// Originally written in Java /// by Robert C. Martin, Robert S. Koss /// on 30 Jun, 1999 2113 (SLAC) /// Translated to C# by Micah Martin /// on May 23, 2005 (On the Train) /// </summary> public class QuickEntryMediator { private TextBox itsTextBox; private ListBox itsList; public QuickEntryMediator(TextBox t, ListBox l) { itsTextBox = t; itsList = l; itsTextBox.TextChanged += new EventHandler(TextFieldChanged); } private void TextFieldChanged(object source, EventArgs args) { string prefix = itsTextBox.Text; if (prefix.Length == 0) { itsList.ClearSelected(); return; } ListBox.ObjectCollection listItems = itsList.Items; bool found = false; for (int i = 0; found == false && i < listItems.Count; i++) { Object o = listItems[i]; String s = o.ToString(); if (s.StartsWith(prefix)) { itsList.SetSelected(i, true); found = true; } } if (!found) { itsList.ClearSelected(); } } } | The structure of the QuickEntryMediator is shown in Figure 23-2. An instance of QuickEntryMediator is constructed with a ListBox and a TextBox. The Quick-EntryMediator registers an EventHandler with the TextBox. This EventHandler invokes the TextFieldChanged method whenever there is a change in the text. This method then finds a ListBox element that is prefixed by the text and selects it. Figure 23-2. QuickEntryMediator 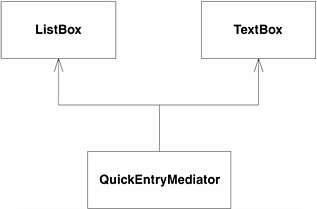 The users of the ListBox and TextField have no idea that this MEDIATOR exists. It quietly sits there, imposing its policy on those objects without their permission or knowledge. |