A new feature I've added to this registration example in this edition of the book is the activation process. When a person registers, an activation code will be associated with their account. This will also be sent as a link in the registration confirmation email. The user then has to click the link in the email, which will take them to the site's activation page. The link will pass to the activation script the user's ID number and the activation code. If these two values match those in the database, the activation code will be removed from the record, indicating an active account. The login page, developed next, will allow only users who have activated their account to log in. To create the activation page 1. | Begin a new PHP script in your text editor or IDE (Script 13.7).
<?php # Script 13.7 - activate.php require_once ('./includes/config. inc.php); $page_title = 'Activate Your Account'; include ('./includes/header.html'); Script 13.7. To activate an account, the user must come to this page, passing it their user ID number and activation code. 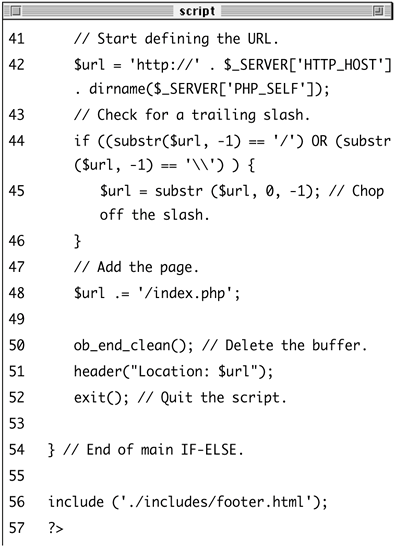
| 2. | Validate the values that should be received by the page.
if (isset($_GET['x'])) { $x = (int) $_GET['x']; } else { $x = 0; } if (isset($_GET['y'])) { $y = $_GET['y']; } else { $y = 0; } As I mentioned, if the user clicks the link in the registration confirmation email, they'll pass two values to this page: the user ID and the activation code. I first check for the presence of x (the user's ID); if it is there, I type-cast it as an integer, just in case. For y (the activation code), I just check for its existence. In either case, if the value is not present, then the variable is set to 0.
| 3. | If $x and $y have the correct values, activate the user.
if ( ($x > 0) && (strlen($y) == 32)) { require_once ('../mysql_connect. php); $query = "UPDATE users SET active= NULL WHERE (user_id=$x AND active='" . escape_data($y) . "') LIMIT 1; $result = mysql_query ($query) or trigger_error("Query: $query\n <br />MySQL Error: " . mysql_ error()); The conditional checks that $x is a positive integer and that $y is 32 characters long, which is the length of the string returned by the md5() function in register.php. If both conditions are trUE, an UPDATE query is run. This query removes the activation code from the user's record by setting the active column to NULL.
| 4. | Report upon the success of the query.
if (mysql_affected_rows() == 1) { echo "<h3>Your account is now active. You may now log in. </h3>"; } else { echo '<p><font color="red" size="+1>Your account could not be activated. Please re-check the link or contact the system administrator.</font></p>'; } If one row was affected by the query, then the user's account is now active and a message says as much (Figure 13.16). If no rows are affected, the user is notified of the problem (Figure 13.17). This would most likely happen if someone tried to fake the x and y values or if there's a problem in following the link from the email to the Web browser.
Figure 13.16. If the database could be updated using the provided user ID and activation code, the user is notified that their account is now active. 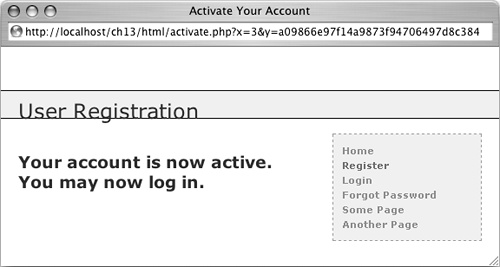
Figure 13.17. If an account is not activated by the query, the user is told of the problem. 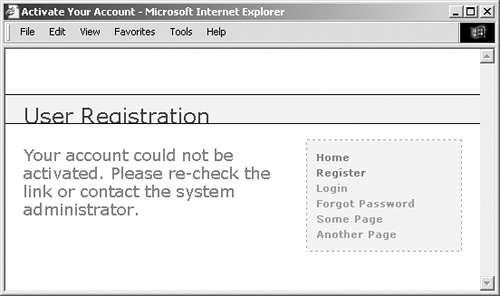
| 5. | Complete the main conditional.
mysql_close(); } else { $url = 'http://' . $_SERVER ['HTTP_HOST] . dirname($_SERVER ['PHP_SELF]); if ((substr($url, -1) == '/') OR (substr($url, -1) == '\\') ) { $url = substr ($url, 0, -1); } $url .= '/index.php'; ob_end_clean(); header("Location: $url"); exit(); } The else clause takes effect if $x and $y are not of the proper value and length. In such a case, the user is just redirected to the index page.
| 6. | Complete the page.
include ('./includes/footer.html'); ?>
| 7. | Save the file as activate.php, upload to your Web server, and test by clicking the link in the registration email (Figure 13.18).
Figure 13.18. The registration confirmation email includes a link to activate the account. 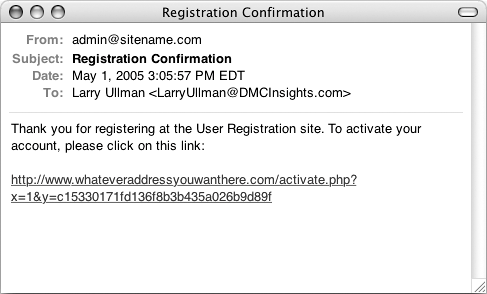
| Tips I specifically use the vague x and y as the names in the URL for security purposes. While someone may figure out that the one is an ID number and the other is a code, it's sometimes best not to be explicit about such things. You may want to add a From parameter to your mail() call in register.php so that you can indicate who sent the confirmation email.
|