Finding an Object's Style Property Values Part 1 of this book showed you how to set a variety of styles using CSS. Many of the properties set using CSS can be directly accessed using the DOM properties shown in Table 14.1. However, many of the style properties do not have direct DOM correlations, so we need to look at how to use the object ID to get at those values. Figure 14.8 shows how to access those style values. Figure 14.8. The general syntax for finding an element's CSS property value. 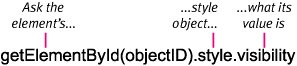 As an example of finding style property values, let's take a look at the visibility property. All objects that have a position set also have a visibility state: hidden or visible (see Chapter 8). The default state is visible. In this example (Figure 14.9), when the page first loads the visibility property is set for the object on the page. Clicking the visible object will cause an alert to appear showing you its visibility value. Figure 14.9. The Cheshire Cat is visible, but for how long? To find the visibility of an object: | | 1. | function initPage(objectID, state) {...} Add the initPage() function to your JavaScript (Code 14.5). This function sets the initial visibility of objects when the page first loads. Unfortunately, browsers cannot access the style that is initially set in the CSS; they're aware of the state only after it has been set dynamically. If you do not set this first, the value shows as null.
Code 14.5. The function findVisibility() determines the current visibility state of an individual object in the window. | | | 2. | function findVisibility(objectID) {...} Add the function findVisibility() to your JavaScript. This function uses the ID of the object to be addressedpassed to it as the variable objectIDto find the object on the page. It then uses this ID to access the current visibility property value set for the object. Based on that value, the function returns either visible or hidden.
| 3. | function showVisibility(evt) {...} Create a JavaScript function showVisibility() that determines the ID of the initiating object from the evt:
var objectID = (evt.target) ? evt.target.id : ((evt.srcElement) ? evt.srcElement.id : null); This function then passes that value to the function findVisibility() and displays the value that function returns.
| 4. | #object1 {...} Set up the CSS rules for your object. In your HTML, you will be using this ID to define a <div> tag as an object.
| 5. | onload="initPage('object1', 'visible');" In the <body> tag, use the initPage() function to initialize the visibility of all the objects for which you need to know the initial visibility. It then displays those values in an alert.
| 6. | onclick="showVisibility(event);" Add an event handler to trigger the function you created in Step 3, and pass to it the event.
| Tips You can also use JavaScript to change the visibility state, as explained in "Making Objects Appear and Disappear" in Chapter 16. In Chapter 18, we'll explore ways to dynamically set CSS after the page has loaded. An alternate (though no less complex) method for finding the visibility state of any object without first setting the value using JavaScript is presented in "Finding a Style Property's Value" in Chapter 18. |