You have already learned how to acquire input from users through the use of a TextBox control. This approach works well for a number of data-gathering needs, but what if you just want a simple piece of information, such as "Do you own a car?" or "What is your marital status?" In these cases, the choice of answers you want to provide is limited to two or, at most, a few fixed choices. If you set up a text box to handle only the words yes and no, your program will have a problem if users type Maybe or Yeah, or if they misspell a word. You can eliminate this problem, however, and make your programs easier to use by employing controls to display and accept choices. In the following sections, you will examine several controls used for making choices. You can offer these choices through the use of check boxes, option buttons, lists, and combo boxes: Check box Switches one or more options on or off Option buttons Selects a single choice from a group List box Displays a list of predefined choices Combo box Like a list box, but also allows the user to specify a choice other than the predefined ones Checked list box A list box that displays a check box by each item in the list, allowing the user to easily select multiple list items In addition, you will learn about the GroupBox control, which assists you in grouping other controls. The CheckBox Control You use the CheckBox control to get an answer of either "yes" or "no" from the user. This control works like a light switch. It is either on (checked) or off (unchecked); normally, there is no in between. When a check box is on, a check mark is displayed in the box. It indicates that the answer to the check box's corresponding question is "yes." When the check box is off, or unchecked, the box is empty, indicating an answer of "no." The Checked property of a CheckBox control is set to either True or False depending on whether the user has checked or unchecked the box. You can set the Checked property in code (chkTest.Checked = True) to modify the check box as your program is running. In addition to being checked or unchecked, a CheckBox control may possibly have a third state in which a check mark appears, but the background of the check box is gray. This is known as an indeterminate state and usually indicates that a partial choice (some, but not all, of a number of subchoices) has been made. For example, you may have a check box that designates whether a group of users has access to a particular application. Perhaps some, but not all, of the members of that user group are to be granted access. In that case, you could have a check box for the overall group; that check box would contain a grayed check, and there might be a Details or Members button that leads to a form where there are individual check boxes for each user. The Checked property does not report an indeterminate check box. Instead, you can utilize the CheckBox control's CheckState property, whose value may be Unchecked, Checked, or Indeterminate. Normally, your user cannot set a check box to the indeterminate state by clicking it; typically, you do so in program code by determining whether none, all, or some of the subchoices have been selected. If you do want to give the user the ability to directly select the indeterminate state, you can set the CheckBox control's ThreeState property to True. This allows the user to cycle between all three possible check box states, rather than the normal two (Unchecked and Checked). Note If the ThreeState property is set to True, the Checked property will return True if CheckState is either Checked or Indeterminate. Table 11.5 discusses commonly used properties that apply to CheckBox controls. Table 11.5. Common CheckBox Control Properties Property | Description |
---|
Appearance | If set to Button, the control looks like a Button control whose appearance toggles between pressed and unpressed. The default setting is Normal, which causes it to look like a normal check box. | AutoCheck | If for some reason you do not want your user to be able to check or uncheck the box by clicking on it, set AutoCheck to False. The default value of True causes the control's state to change whenever the user clicks it. | CheckAlign | Specifies where the check box is located within the boundaries of the control. There are nine possible locations any of the four edges, any of the four corners, and MiddleCenter. The default is MiddleLeft. Click the drop-down arrow to specify the location. | Checked | Indicates True or False, depending on whether the box is checked or unchecked. When a CheckBox control is first added to a form, the Checked property is set to False by default. | CheckState | Indicates the "check state" of the check box. Possible values are Unchecked, Checked, and Indeterminate (grayed). | FlatStyle | Determines the appearance of the check box and how it changes when the user moves the mouse over the button. The Standard setting (which is the default) causes it to be displayed like a typical check box. Other possible settings are Flat and Popup. | Image | Specifies an image to be displayed on or in the control. | ImageAlign | Specifies where within the control the image specified by the Image property will be aligned. There are nine possible locations any of the four edges, any of the four corners, and MiddleCenter (the default). Click the drop-down arrow to specify the location. | Text | The text that is displayed as the radio button's caption. | TextAlign | Specifies where the text specified by the Text property is aligned within the boundaries of the control. There are nine possible locations any of the four edges, any of the four corners, and MiddleCenter. The default is MiddleLeft. Click the drop-down arrow to specify the location. | ThreeState | When set to True, the user can cycle through all three possible CheckState values (Unchecked, Checked, and Indeterminate) by clicking the check box. When set to the default value of False, the user can only toggle between Unchecked and Checked; she cannot set the CheckState property to Indeterminate. | Figure 11.5 shows various types of check boxes. Figure 11.5. A check box can indicate a "yes" or "no" response to a question. 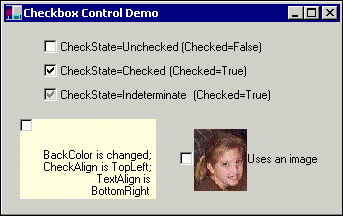 The RadioButton Control Radio buttons are like the buttons that select stations on a car radio; they exist in a group, and only one of them can be selected at a time. They are useful for presenting a fixed list of mutually exclusive choices. Only one of a group of radio buttons may be selected at any given time. As with CheckBox controls, RadioButton controls have a Checked property that indicates via True or False values whether the control is selected or not. Try it yourself: Draw several RadioButton controls on a form, and then run the program. Initially, each radio button's Checked property has the default value of False. However, notice that if you click any one of them to select it, and then subsequently select a different button in the group, the one that was selected will automatically become unselected. Figure 11.6 illustrates a group of RadioButton controls, only one of which is selected. Figure 11.6. Only one radio button in a group can be selected. 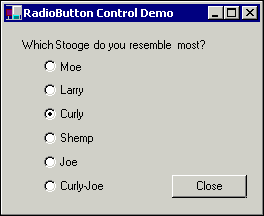 One major difference between RadioButton controls and CheckBox controls is that only one of the RadioButton controls in a group can be selected at any given time, whereas the selection state of CheckBox controls is independent of all other controls. How, then, do you define a group of radio buttons? The simplest type of group includes all the RadioButton controls that are placed directly on a form. However, a special type of control called a GroupBox control is available to assist in grouping radio buttons. You will learn about the GroupBox control in the next section. Note RadioButton and GroupBox controls are equivalent to the OptionButton and Frame controls, respectively, provided in previous versions of Visual Basic. To utilize RadioButton controls in your code, you typically write statements that examine the Checked property of the applicable radio buttons as needed. For example, suppose you have created a form that asks the user to enter registration information. You might have a group of three radio buttons, rbRepublican, rbDemocrat, and rbIndependent, to allow the user to specify his or her political preferences. Your procedure that processes the form could include code like the following: If rbRepublican.Checked Then Call ProcessConservative() ElseIf rbDemocrat.Checked Then Call ProcessLiberal() ElseIf rbIndependent.Checked Then Call ProcessMiddleOfRoad() Else Call ProcessNoPref() End If Alternatively, you may want to add code to the Click event handlers of each of your radio buttons if you want to perform some action as soon as the button is clicked. For example, you may be coding a trivia game that presents your users with a question and a number of multiple-choice answers. You could write code to score the question in the Click event handlers of each of the possible answers (represented by RadioButton controls). Of course, using this approach does not allow your user to change his mind once he has clicked one of the choices. Tip A common misconception about RadioButton controls is that there is a property that reports which one of a group of buttons is selected. While such a property might be handy, this isn't the case. One technique I use to emulate this functionality is to create a form-level variable to let me know which button is selected; the Click event handler of each individual button sets the value of the variable. For example, if I have a group of radio buttons to allow the user to tell me his favorite baseball team, each button's Click event handler could set the variable strFavoriteTeam to a different value. Table 11.6 shows properties that are commonly used in relation to RadioButton controls. Table 11.6. Common RadioButton Control Properties Property | Description |
---|
Appearance | If set to Button, the control looks like a Button control whose appearance toggles between pressed and unpressed. The default setting is Normal, which causes it to look like a standard radio button. | AutoCheck | If for some reason you do not want your user to be able to select the radio button by clicking it, set AutoCheck to False. The default value of True causes the control to be selected when the user clicks it. | CheckAlign | Specifies where the button itself is located within the boundaries of the control. There are nine possible locations any of the four edges, any of the four corners, or MiddleCenter. The default is MiddleLeft. Click the drop-down arrow to specify the location. | Checked | Contains True or False, depending on whether the control is selected or not. When a RadioButton control is first added to a form, the Checked property is set to False by default. You can determine a default choice by setting the Checked property of one button to True. Remember, no more than one radio button in a group can have a Checked property of True at any given time. | FlatStyle | Determines the appearance of the radio button and how it changes when the user moves the mouse over it. The Standard setting (which is the default) causes it to be displayed like a typical radio button. Other possible settings include Flat and Popup. | Image | Specifies an image that is to be displayed on or in the control. | ImageAlign | Specifies where within the control the image specified by the Image property will be aligned. There are nine possible locations any of the four edges, any of the four corners, and MiddleCenter (the default). Click the drop-down arrow to specify the location. | Text | The text that is displayed as the radio button's caption. | TextAlign | Specifies where the text specified by the Text property is aligned within the boundaries of the control. There are nine possible locations any of the four edges, any of the four corners, and MiddleCenter. The default is MiddleLeft. Click the drop-down arrow to specify the location. | The GroupBox Control The GroupBox control, mentioned in the previous section, allows you to group controls such as radio buttons and check boxes. Think of a GroupBox control as a container for other controls. It is a rectangular box into which you can place any number of controls. All the RadioButton controls placed in a particular GroupBox control are treated as a group; that is, only one of the radio buttons in that group may be selected at a given time. However, radio buttons in other GroupBox controls (or placed directly on the form) may be selected as well, within the constraints of their own group(s). In addition to delineating groups of radio buttons, GroupBox controls are handy for creating visual groups of other types of controls as well. Figure 11.7 shows GroupBox controls that contain RadioButton and CheckBox controls. Note that within a group box any combination of check boxes, but only one radio button, may be selected. Figure 11.7. The GroupBox control lets you place controls in logical groups. 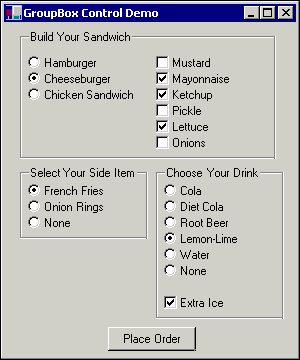 One property of note that applies to the GroupBox control is the Text property, which contains the text that appears as a caption at the top of the control's surrounding border. The ListBox Control As its name implies, you can use the ListBox control to present a list of choices. Figure 11.8 shows a simple list box used to pick a state abbreviation for use on a mailing label. This figure shows all the components that make up the list box. Figure 11.8. A simple list box contains a series of choices for the user. 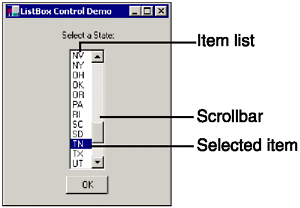 The key parts of the list box are the following: Item list This is the list of items from which the users can select. These items are added to the list in the design environment or by your program as it is running. Selected item This is the item that has been chosen by the user. Depending on the style of list you choose, a selected item is indicated by a highlight bar or by a check in the box next to the item. Scrollbar This part indicates that more items are available on the list than will fit in the box and provides the users with an easy way to view the additional items. To the user, using a list box is similar to choosing channels on a TV. The cable company decides which channels to put on the selection list. The customer then can pick any of these channels but can't add one to the list if she doesn't like any of the choices provided. With a list box, the choices are set up by you, the programmer; the user can select only from the items you decide should be available. When you first draw a list box on the form, it shows only the border of the box and the text ListBox1 (the default name of the first list box). No scrollbar is present and, of course, no list items are available. A vertical scrollbar is added to the list box automatically when you list more items than fit in the box. If the items in your list box are wider than the control displays, you can add a horizontal scrollbar by setting the HorizontalScrollbar property to True. Adding Items to a List Box Obviously, for a list box to be functional, you need to add items to its list. There are several ways to accomplish this, either at design time or at runtime. You may want to create a sample Windows Application project and add a ListBox control to Form1.vb in order to test each method. Adding Items at Design Time To add items to a list box at design time, make sure the ListBox control on the form is selected; then look for the Items property in the Properties window. Actually, Items is a collection to which members can be added. If you click the ellipsis (…) next to the Items property, you will be presented with the String Collection Editor window, as shown in Figure 11.9. You can use the String Collection Editor to enter the items that you want to be displayed in your list box. Put each item on a separate line. Click the OK button to close the editor; you can now see the items you added to the list box at both design time and runtime. Figure 11.9. The String Collection Editor allows you to enter items into a list box at design time. 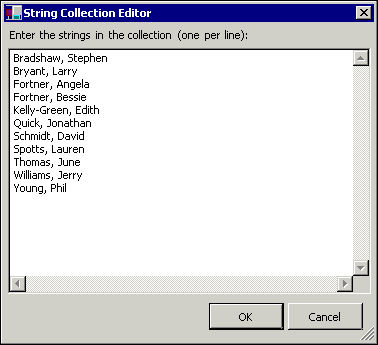 Adding Items at Runtime with the Add Method If you want to add items to your list box at runtime, you can do so by invoking the Add method of the ListBox control's Items collection at runtime. Typically, you place such initialization code after the InitializeComponent call in your form's predefined New procedure, which is executed when an instance of your form is created. The following code illustrates a form's Sub New, which has been modified to add six employee names to a ListBox control named lstAribanistas in this manner: Public Sub New() MyBase.New Form1 = Me 'This call is required by the Win Form Designer. InitializeComponent() 'TODO: Add any initialization after the InitializeComponent() call lstAribanistas.Items.Add("Newsom, Steve") lstAribanistas.Items.Add("Morrison, Robert") lstAribanistas.Items.Add("James, Bob") lstAribanistas.Items.Add("Watson, Mike") lstAribanistas.Items.Add("Brown, Zondra") lstAribanistas.Items.Add("Johnson, Cathy") lstAribanistas.Items.Add("Burton, Eric") lstAribanistas.Items.Add("Wright, Mamon") lstAribanistas.Items.Add("Phillips, Jerry") End Sub Figure 11.10 shows this list box at runtime. Figure 11.10. A list box can show a number of choices. 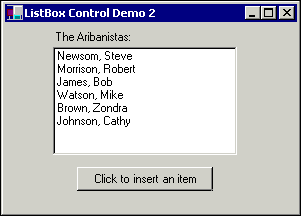 Inserting Items at Runtime with the Insert Method If you want to add an item to a specific position in an existing ListBox control's list, you can use the Insert method of the ListBox control's Items collection. The Insert method allows you to specify the specific position within the list as well as the text to insert. Note that when specifying the position, numbering begins with zero (0); the first item in the list is position 0, the second is position 1, and so forth. The following code shows how to add a new item in position 3 (the fourth position) of a list box: lstAribanistas.Items.Insert(3, "Phillips, Jerry") You can see the results of this new addition to the list box in Figure 11.11. Figure 11.11. A new item has been added to the list box. 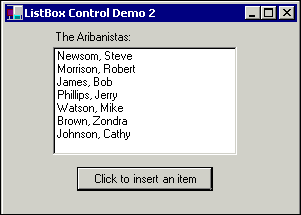 Assigning an Array to a List Box You can also assign an entire array of variables to the All property of the Items collection of a list box. This will cause the list box to be populated with the members of the array. The following code demonstrates this technique: Dim strDays() As String = {"Sunday", "Monday", "Tuesday", "Wednesday", _ "Thursday", "Friday", "Saturday"} lstWeekDays.Items.All = strDays Figure 11.12 shows this list box that has been populated from an array. Figure 11.12. You can use an array to populate a list box all at once. 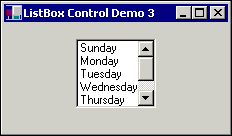 Removing Items from a List Box There may be times when you want to remove individual items, or even the entire list of items, from a list box. Visual Basic provides methods for each task, as discussed next. Removing Individual Items with the Remove Method The ListBox control's Items collection has a Remove method whose purpose is to delete a specific item from the list box. You do so by specifying the desired item's position in the list box, specifying the text of the item, or indicating that you want the currently selected item removed (using the SelectedItem property, which contains the index of the item that is currently highlighted). The following code demonstrates all three methods: 'Remove the third item (index 2): lstTest.Items.Remove(2) 'Remove the item whose text is "Nashville" lstTest.Items.Remove("Nashville") 'Remove the currently selected item: lstTest.Items.Remove(lstTest.SelectedItem) Note that either of the first two methods will generate an error if you attempt to remove an item that does not exist. The third method will generate an error if no item is selected. The following illustrates a sample KeyDown procedure that can be used to delete an item from a list box when the user presses the Delete key while a particular item is selected. Note that the KeyCode property of the KeyEventArgs class specifies which key the user pressed and is compared to the Delete member of the Keys enumeration. This tells the procedure whether the Delete key was pressed; if so, the Remove method is invoked to delete the selected item: Private Sub lstTest_KeyDown(ByVal sender As Object, _ ByVal e As System.Windows.Forms.KeyEventArgs) _ Handles lstTest.KeyDown If e.KeyCode = keys.Delete Then lstTest.Items.Remove(lsttest.SelectedIndex) End If End Sub Removing All Items with the Clear Method You can remove all the items in a list box's Items collection by invoking the Clear method, as follows: lstTest.Items.Clear Accessing Members of the Items Collection In addition to providing methods to allow you to add and remove list box items, the Items collection gives you access to the contents of the list. You can access the members of the Items collection using an index, much as you access the elements of a variable array. When utilizing the index, remember that the first item in the list box has an index of 0. Therefore, the first item of a ListBox control named lstTest would be accessible through lstTest.Items(0), the second item through lstTest.Items(1), and so on. If you do not know how many items are in the list, the Count property of the Items collection will provide this information. The following code uses a message box to display, one by one, the items in a ListBox control's list: Dim i As Integer For i = 0 To lstTest.Items.Count - 1 'Remember that the index begins with 0 for the first item, ' so the counter variable must start at 0 and go up to ' one less than the Count property. MsgBox(lstTest.Items(i)) Next i Accessing the Currently Selected Item If the user has selected an item in the list, the ListBox control provides you with two properties that allow you to access the selected item. The SelectedIndex property tells you the index of the selected item, whereas the SelectedItem property contains the actual item that has been selected. To illustrate, assume that you have a list box that contains city names. Memphis is in the second position (index 1) and has been selected by the user. The code MsgBox lstTest.SelectedIndex would return a value of 1, telling you that the second item in the list has been selected. MsgBox lstTest.SelectedItem would provide you with the actual contents of that position, Memphis. Note SelectedIndex returns a value of -1 if no item is selected. Another way to retrieve the value of the list box item selected by the user is to check the list box's Text property. The Text property contains the item from whichever line of the list box has been selected. If no list box item has been selected, the Text property contains an empty string (""). Determining the Initial Selection Depending on your application, you might want to set the initial item for a list box. For example, you can set the choice in a program that needs to know your citizenship. You can provide a list of choices but set the initial value to United States because that would be the selection of most people in this country. You set the initial value by using the SelectedIndex property of the Items collection. For example, if United States is the fourth entry in the list, you can use the following code to cause that item to be preselected (recall that SelectedIndex begins its counting with zero): lstCitizenship.Items.SelectedIndex = 3 This statement causes the fourth item in the list (with an index of 3) to be displayed when the list box is first shown. You can set the initial choice in this manner for a list box, a checked list box, or a combo box. You also can set the initial selection of a list box by setting its Text property to the value of the desired list item, as follows: lstCitizenship.Text = "United States" Sorting List Box Items You have seen how to modify a list by adding and removing items. But what do you do if you want your entire list sorted in alphabetic order? Fortunately, this task is simple. To sort the list, you simply set the ListBox control's Sorted property to True. Then, no matter in which order you enter your items, they appear in alphabetic order to the users. The indexes of the list items are adjusted as they are added so that they remain in order. Note Because the Sorted property causes list items to be placed in alphabetical order, numeric items may not appear to sort properly. For example, a list containing the numbers 1, 2, 3, 11, 23, 100, and 200 would be sorted in the order 1, 100, 11, 2, 200, 23, 3, which is correct if the values are treated as text instead of numbers. This may be confusing to your users. Working with Multiple Selections Sometimes you need to let the users select more than one item from a list. The list box supports this capability with the SelectionMode property. This property has four possible settings: One, None, MultiSimple, and MultiExtended: The default setting, One, means that multiple selections are not permitted, and the list box can accept only one selection at a time. If one item is selected and the user clicks a second item, the first item is automatically deselected. A setting of None specifies that the user cannot select items in the list at all. With a setting of MultiSimple, a user can use the mouse to select and deselect multiple items. If one item is selected and he clicks another item, that second item will be selected in addition to the first item. The other setting of the MultiSelect property, MultiExtended, is more complex. In this mode, users can use standard Windows techniques to select multiple items. They can select a range of items by clicking the first item in the range and then, while holding down the Shift key, clicking the last item in the range; all items between the first and last item are selected. To add or delete a single item to or from the selection, users hold down the Ctrl key while clicking the item. Getting the selections from a multiple-selection list box is a little different from getting them for a single selection. Because the SelectedItem and SelectedIndex properties work only for a single selection, you can't use them. Instead, the ListBox control provides two other collections, SelectedItems and SelectedIndices, which provide an array of item values and indices, respectively, containing only the items that have been selected. Each supports a Count property so you can know how many items have been selected. To illustrate this, the following code uses the SelectedItems collection to display all selected items in message boxes: Dim i As Integer For i = 0 To lstTest.SelectedItems.Count - 1 MessageBox.Show(lstTest.SelectedItems(i)) Next i Table 11.7 is a listing of commonly used properties that apply to ListBox and CheckedListBox controls. Table 11.7. Common ListBox and CheckedListBox Control Properties Property | Description |
---|
BorderStyle | How the border is drawn around the control. The default setting, Fixed3D, shows a three-dimensional border. BorderStyle can also be set to FixedSingle, which gives it a flatter appearance. | MultiColumn | When set to True, displays the list items in multiple columns. The default value is False. | ColumnWidth | Determines how wide each column is (in pixels) when MultiColumn is set to True. | HorizontalScrollbars | Determines if the ListBox control will display a horizontal scrollbar if its contents are wider than the control. The default value is False. | HorizontalExtent | If HorizontalScrollbars is True, this property sets the width (in pixels) by which the list box can be scrolled horizontally. | Items | The actual items contained in the list. See the text for a description of the Items collection. | ScrollAlwaysVisible | When set to False (the default), the vertical scrollbar appears only when needed (that is, when the list contains more items than will fit in its height). A setting of True causes the vertical scrollbar to be shown regardless of the size of the list. | SelectionMode | Determines if and how the control allows more than one item to be selected at the same time. See the text for a full description. | Tip You can add code to a list box control's Click or DoubleClick event handler to cause an action to occur when a user clicks or double-clicks an item in the list. This causes the list box to act like a mini-menu. In the event handler code, you can examine the value of the ListBox control's SelectedIndex or Text property to see which item the user clicked. This technique is often used to allow the user to double-click an item in a list to view some details about the item. The CheckedListBox Control The CheckedListBox control is almost identical to the ListBox control, except in the way its list items are presented. In a CheckedListBox control, each item in the list has a check box to the left of the item's text. The user can check or uncheck individual items in the list. One thing to keep in mind is that a checked item is different from a selected item. The CheckedListBox control does not support the Multi- settings of the SelectionMode property (although they may appear in the Properties window), so your user cannot select multiple items (although she can check multiple items). Figure 11.13 illustrates a typical CheckedListBox control. Figure 11.13. A checked list box lets the user mark entries to select them. 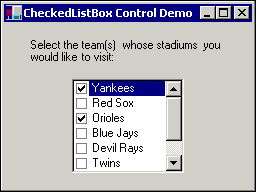 Determining Checked Items To figure out which items in a CheckedListBox control have been checked, you need to use the GetItemChecked method for each item in the control's list. The following code will display message boxes containing each checked item in the CheckedListBox control clbTest: Dim i As Integer For i = 0 To clbTest.Items.Count - 1 If clbTest.GetItemChecked(i) = True Then msgbox(clbTest.Items(i)) End If Next i The properties presented in Table 11.8 are noteworthy for the CheckedListBox control in addition to the ListBox properties you saw in Table 11.7. Table 11.8. Common CheckedListBox Control Properties Property | Description |
---|
CheckOnClick | When set to True, when the user clicks an unselected item, the item is selected and its check box is checked (or unchecked if it was already checked). The default value is False; in this case, the user must click an item once to select it, and then click it again to check it. This technique is confusing, so you should consider setting this property to True. | ThreeDCheckBox | Determines whether the check box displayed by each list item has a 3D appearance. A setting of False (the default) displays flat check boxes. | The ComboBox Control As its name implies,the ComboBox control combines the functionality of two other controls list boxes and text boxes. The ComboBox control functions much like a list box. However, in addition to being able to select from predefined choices, the user can enter freeform text as well (depending on how the ComboBox control is configured). The ComboBox control supports three distinct styles, each of which provides slightly different functionality for the user. The style displayed by a ComboBox control is determined by its Style property. Each of the styles is discussed in the following sections. The Drop-Down Combo Box The default configuration,of a ComboBox control is the drop-down combo box, which is specified by setting the control's Style property to DropDown. This type of combo box presents the user with what appears to be a normal text box combined with a drop-down list (see Figure 11.14). The user can either type an entry into the text box portion of the control or click the drop-down arrow to select an item from the list portion of the control. In either case, the item she typs or selects is stored in the control's Text property. Figure 11.14. The drop-down combo box lets the user click to reveal and select from the list or type an alternative item in the text box. 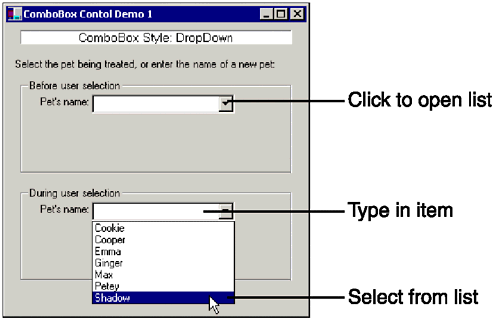 The Simple Combo Box Setting a ComboBox ,control's Style property to Simple causes the control to look like a text box on top of a list box (see Figure 11.15). As with the drop-down style, the user can either type information into the text box or select an item from the list portion. If he selects an item from the list, that item is put into the text box portion. Again, in either case, the item is stored in the control's Text property. Figure 11.15. The simple combo box displays a text area and a list at the same time. 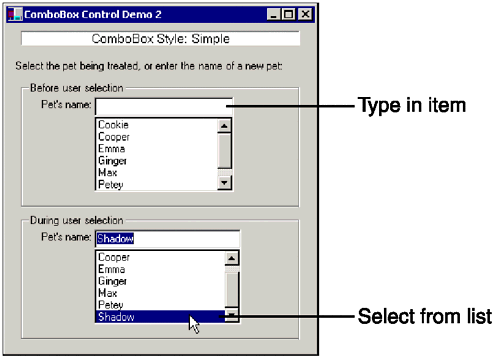 The Drop-Down List Combo Box This final combo box ,style, which is specified by setting the Style property to DropDownList, displays a drop-down list without a text box for the user to supply a freeform entry (see Figure 11.16). He can only select an item from the predefined list. In essence, this style works just like a list box. It saves screen space, however, as the control itself only displays one line. The list is contained in the drop-down portion, which is only visible when activated. Figure 11.16. The drop-down list style of combo box works like a list box but saves screen space. 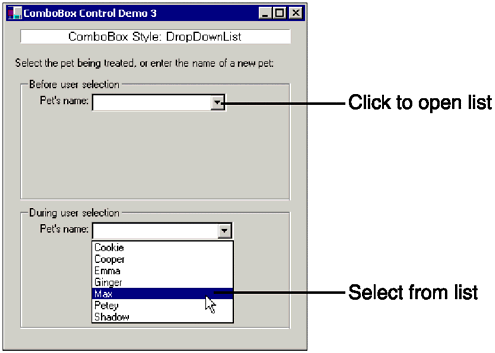 Note You can only change the Height property for a ComboBox control if its Style property is Simple. The height of combo ,boxes of the other two styles DropDown and DropDownList will be automatically configured, since the list portion is not normally visible. Managing Combo Box Items The combo box ,has much in common with the list box. Both use the Add, Remove, and Clear methods of the Items collection to modify the contents of the list. Both can present a sorted or an unsorted list. The techniques used to manage members of the list portion of a combo box are identical to those used for a list box, as discussed earlier in this chapter. Caution The initial value of a combo box's Text property is the name of the combo box. If you do not want this name to appear in your combo box on startup, set a default selection using the techniques described earlier in this chapter, or set the Text property in code. Alternatively, you can simply delete the contents of the Text property while you are in the design environment. Working with Choices Not on the List The real power ,of the combo box is its ability to allow users to enter choices other than those on the list. This capability is available with two styles of combo boxes the simple combo box and the drop-down combo box. Both styles provide a list of items from which you can select, and both allow you to enter other values. The difference between the two styles is the way in which you access items already in the list. With a simple combo box, your users can access the items in the list using the mouse or the arrow keys. If the users don't find what they want on the list, they can type new choices. The drop-down combo box works like a combination of the drop-down list and the simple combo box. You select an item from the list the same way you would for a drop-down list, but you also can enter a value that is not on the list. In any case, ,the value your user enters into a combo box whether he enters it by clicking a predefined option or by typing a new value into the text box can be accessed through the ComboBox control's Text property. |