We will begin our discussion of controls by revisiting three controls the TextBox, Label, and Button controls that you have already worked with in earlier chapters. They are probably the controls that are used most often by Visual Basic developers. Figure 11.1 illustrates a typical data entry form utilizing each of these controls. Figure 11.1. Text boxes, labels, and buttons are frequently used controls. 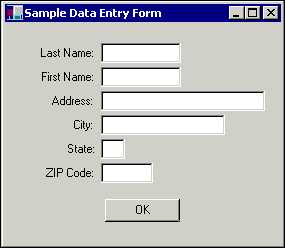 Note The basic controls presented here are discussed in more detail in Chapter 2. See the sections "Adding a TextBox Control," "Labeling Your Program's Controls," and "Adding Command Buttons" in that chapter. The TextBox Control Because much of what programs do is to retrieve, process, and display information in the form of text, you might guess (and you would be correct) that the major workhorse of many programs is the TextBox control. The text box allows you to display text; more importantly, however, it also provides an easy way for your users to enter and edit text and for your program to retrieve the information that was entered. A TextBox control presents your program's user with an area in which he can enter or modify text. That text can be accessed by your program through the TextBox control's Text property. Tip When your user is in the process of entering or editing text in a TextBox control, he can use standard Windows techniques to assist him. For example, he can select a portion of the text and press Ctrl+C to copy the text to the clipboard, Ctrl+X to cut the text, or Ctrl+V to paste text from the clipboard into the TextBox control. Alternatively, he can right-click inside the text box and select Cut, Copy, Paste, or Delete from the context menu that appears. Handling Multiple Lines of Text Normally, you use the text box to handle a single piece of information, such as a name or an address. If needed, however, the text box can handle thousands of characters of text. By default, the text box accepts a single line of text. This is adequate for many purposes, but occasionally your program needs to handle a larger amount of text. The TextBox control has two properties that are useful for handling larger amounts of text: the MultiLine and ScrollBars properties. The MultiLine property determines whether the information in a text box is displayed on a single line or wraps and scrolls to multiple lines. If the MultiLine property is set to True, information is displayed on multiple lines, and word wrapping is handled automatically. Users can press Enter while typing to force a new line. The ScrollBars property determines whether scrollbars are displayed in a text box, and if so, what types of scrollbars (Horizontal, Vertical, or Both). The default value of None causes no scrollbars to be displayed, although the user can still use the mouse or arrow keys to navigate to text that he can't see. The scrollbars are useful if more text is stored in the Text property than fits in the text box. The ScrollBars property has an effect on the text box only if its MultiLine property is set to True. Figure 11.2 shows several text boxes that illustrate the effects of the MultiLine and ScrollBars properties. Figure 11.2. You can use a text box to enter single lines of text or entire paragraphs. 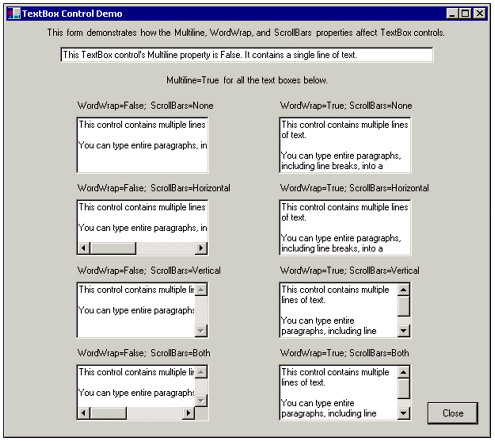 Validating Input When accepting freeform text input from a user, you often need to perform some data validation before proceeding. For example, if you have a text box for the quantity of an item ordered, you may want to see if the user entered a value other than a number into the text box. You can use a TextBox control's Validating event to validate the user's input. This event handler can contain code that validates the information in the associated control. It is triggered by another control whose CausesValidation property is set to True. The following is a typical Validating event handler that uses the IsNumeric function to ensure that the data entered into a text box is numeric: Private Sub txtQuantity_Validating(ByVal sender As Object, _ ByVal e As System.ComponentModel.CancelEventArgs) _ Handles txtQuantity.Validating If Not IsNumeric(txtQuantity.Text) Then MessageBox.Show("You must enter a number in the Quantity field.") txtQuantity.Focus() End If End Sub Table 11.2 lists the properties that you will use with TextBox controls most often. Table 11.2. Common TextBox Control Properties Property | Description |
---|
Text | The text contained in the control. You can set the Text property either at design time using the Properties window or at runtime using program code such as txtManager.Text = "Chris Cawein". Your user can change the Text property at runtime by simply typing in the text box. | Multiline | Determines whether the text in the control can extend to more than one line. The default value is False. | ScrollBars | For multiline text boxes, this property determines whether horizontal and vertical scrollbars are displayed to help the user navigate the text area. The default value is None. Other possible values are Horizontal, Vertical, and Both. | AutoSize | When set to True (the default), the control's height will be automatically maintained based on the control's font size. AutoSize does not apply if the control's Multiline property is set to True. Notice that when AutoSize is True, any sizing handle that would normally modify the control's height is disabled at design time. | AcceptsReturn | If Multiline is set to True, this property determines whether a Return character (the Enter key) is accepted as input in the text box. The default value is False. | AcceptsTab | If Multiline is set to True, this property determines whether a Tab character is accepted as input in the text box. The default value is False. | BorderStyle | Set to Fixed3D (the default), FixedSingle, or None to determine the appearance of the border surrounding the text box. | CharacterCasing | Text entered into the text box can be automatically converted into either all uppercase or all lowercase characters by setting the CharacterCasing property to Upper or Lower, respectively. The default value is Normal. | MaxLength | Sets the maximum number of characters that may be entered into the text box. The default value is 0, which specifies that there is no maximum. | PasswordChar | Specifying a character for the PasswordChar property causes that character to be displayed instead of the text contained in the control. Typically, an asterisk is used to prevent the display of a password as it is being entered. This property is only functional if the TextBox control's Multiline property is set to False. | ReadOnly | When set toTrue, the text in the control cannot be changed by the user. The default value is False. | SelectionLength | The number of characters in the TextBox control's Text property that are selected (highlighted). Used in conjunction with the SelectionStart property. | SelectionStart | The position of the first character in the TextBox control's Text property that is selected (highlighted). Used in conjunction with the SelectionLength property. | TextAlign | Determines how text is aligned within the control. The possible values are Left(the default), Right, and Center. | WordWrap | If Multiline is True for this control, WordWrap determines whether content that is too wide for the control is automatically wrapped to the next line. The default is True. | The Label Control You use a Label control to display information that the user cannot change. Typically, Label controls are used to identify ("label") input areas or other information that is displayed on a form. A Label control's Text property determines what text is displayed in it. Tip When assigning a label's Text property, you can force a new line by including a carriage return and line feed combination. This technique, as well as its nomenclature, is a throwback to the ancient days of manual typewriters. When a manual typewriter user reached the end of a line, she had to move the paper up manually to the next line (a line feed) and return the carriage to the beginning of that line (a carriage return). In Visual Basic .NET, you can insert a carriage return/line feed combination by inserting ASCII characters 13 and 10 into the caption at the point where the line should break. Visual Basic supplies a predefined constant, vbCrLf, to help you accomplish this task: Label1.Text = "First Line" & vbCrLf & "Second Line" Table 11.3 shows you properties that are commonly used for Label controls. Table 11.3. Common Label Control Properties Property | Description |
---|
Text | The text displayed in the label. As with TextBox controls, you can set the Text property either at design time using the Properties window or at runtime using program code such as lblDirectorName.Text = "Jerry Williams". However, your user cannot change the Text property of a Label control. | AutoSize | When set to True, the label's size will be automatically maintained based on its contents and font size. The default value is False. | BorderStyle | Set to None (the default), FixedSingle, or Fixed3D to determine the appearance of the border surrounding the label. | Image | Specifies an image that is to be displayed on the label. | ImageAlign | Specifies where on the label the image specified by the Image property will be aligned. There are nine possible locations any of the four edges, any of the four corners, and MiddleCenter (the default). Click the dropdown arrow to specify the location. | UseMnemonic | If set to True (the default), an ampersand (&) in the label's Text property will designate the next letter as an access key for a control whose TabIndex property is one higher than the label's TabIndex property. For example, if a Label control whose TabIndex property is 11 has UseMnemonic set to True, and its Text property contains A&ddress, pressing Alt+D at runtime would shift the focus to a TextBox control whose TabIndex property is 12. | Figure 11.3 shows how some of these properties affect the appearance of Label controls. Figure 11.3. Label controls can exhibit a wide range of appearances. 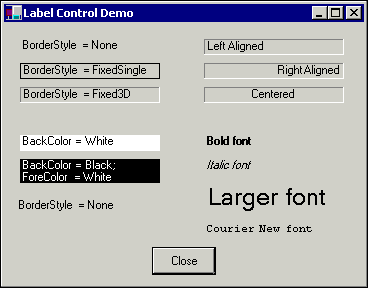 Note If you use an ampersand (&) in a control's Text property to assign an access key, you should be aware that Windows 2000's default settings do not cause a visible underscore to be displayed under the access key until the user presses Alt. The Button Control A control important to practically every application that you will develop is the Button control. This control lets users initiate actions by using the mouse to click a button. You set up a Button control by drawing the button on a form and then setting its Text property to the text that you want displayed on the button's face. Some typical Button controls are illustrated in Figure 11.4. Figure 11.4. Button controls are one way a user can dictate program actions. 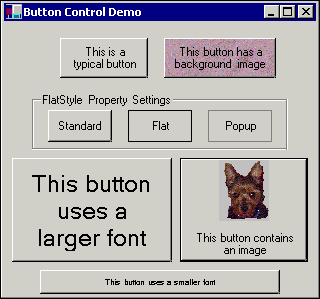 Writing Code for a Button As you have seen in several examples now, the main purpose of a Button control is to execute program code when the button is clicked. To activate this functionality, you write a Click event handler that Visual Basic automatically invokes each time the button is clicked. Double-click a Button control at design time to create or modify the button's Click event handler in the Code window. The following is a typical Click event handler for a button named btnExit: Private Sub btnExit_Click(ByVal sender As System.Object, _ ByVal e As System.EventArgs) Handles btnExit.Click ActiveForm.Close() End Sub Using the Keyboard with a Button Although users most often use buttons by clicking them with the mouse, some users prefer accessing commands through the keyboard. This is often the case for data-entry programs. To accommodate these users, you can make your program trigger buttons when certain keys are pressed. You do so by assigning an access key to the button. When an access key is defined, the user can hold down Alt and press the access key to trigger the Button control's Click event. You assign an access key when you set the Button control's Text property. Simply place an ampersand (&) in front of the letter of the key you want to use. For example, if you want the users to be able to press Alt+P to invoke a Print button, you set the Text property to &Print. The ampersand does not show up on the button, but the letter for the access key is underlined. The caption Print then appears on the button. Tip If for some reason you need to display an ampersand on the face of a button, simply use two of them in a row in the Text property; for example, Save && Exit produces the caption Save & Exit. Creating an Accept Button One button on a form can be designated as that form's Accept button. When this is the case, the user can simply press Enter while the focus is on any control (except another command button or a text box) whose MultiLine property is to trigger the default button. This action invokes the default button's Click event handler, just as if the users had clicked it with the mouse, and is commonly used for data entry forms. To set up a button as the Accept button, set the AcceptButton property of the form (not of the button itself) to the name of the button that should act as the Accept button. Only one button on a form can be the Accept button. Creating a Cancel Button You can also designate one button on a form as the Cancel button, which is similar to the Accept button but works with the Esc key. If the user presses Esc while working with the form, the Cancel button's Click event handler will be invoked. To make a button into a Cancel button, set the CancelButton property of the form (not of the button itself) to the name of the button that should act as the Cancel button. As with Accept buttons, only one button on a form can be a Cancel button. Table 11.4 presents commonly used Button control properties. Table 11.4. Common Button Control Properties Property | Description |
---|
Text | The text displayed on the face of the button. You can set the Text property either at design time using the Properties window or at runtime using program code such as txtLastName.Text = "Cawein". Including an ampersand (&) before one letter of the Text property will define that letter to be the button's Access key. This means that the user can initiate the button's Click event by pressing Alt plus the Access key. For example, if a button's Text property contains &Calculate, pressing Alt+C at runtime will invoke the button's Click event handler, just as if the button had been clicked with the mouse. | FlatStyle | Determines the appearance of the button and how it changes when the user moves the mouse over the button. The Standard setting (which is the default) causes the button to be displayed like a typical Windows button. A setting of Flat causes the button to appear flat against the form at all times. (The text displayed on the button changes color when hovered over.) A setting of Popup makes the button appear flat until it is hovered over, at which time it changes to look like a standard button. | Image | Specifies an image that is to be displayed on the button. | ImageAlign | Specifies where on the button the image specified by the Image property will be aligned. There are nine possible locations any of the four edges, any of the four corners, and MiddleCenter (the default). Click the dropdown arrow to specify the location. | TextAlign | Determines how text is aligned on the button. Like the ImageAlign property, there are nine possible values; MiddleCenter is the default. | |