As you may recall from our earlier discussion in Chapter 5, exceptions are errors that occur during program execution. Exceptions are thrown by functions within your program and can be caught and handled by Try ... Catch statements. Writing code to handle exceptions is important so your program can gracefully handle program errors at runtime. For an introduction to exceptions in Visual Basic, p.113 However, during the normal course of application development, exceptions are likely to occur before you have written code to handle them. In addition, you may want to disable your own exception handling code to troubleshoot program problems. In this section, we'll take a look at working with exceptions in the Visual Studio environment. Breaking for Unhandled Exceptions To simplify our discussion, let's pick an exception that is easy to understand: an invalid database password. If you try to establish a connection to a database and do not provide a correct password, the database driver may throw an exception. The following three lines of code attempt to connect to a SQL server database using an ADO.NET SQLConnection object: Dim cn As New SqlConnection() cn.ConnectionString = "Server=MyServer;UID=sa;pwd=WRONG;Database=pubs" cn.Open() The first line of code creates a new SQLConnection object and the second line sets its ConnectionString property. These two lines of code should execute without error. However, if the password is incorrect, the call to the Open method in the last line of code would throw an exception. If you are debugging in Visual Studio and have not written code to handle this exception, you will see a message similar to the one in Figure 26.1. Figure 26.1. Visual Studio displays a dialog box when unhandled exceptions occur during debugging. 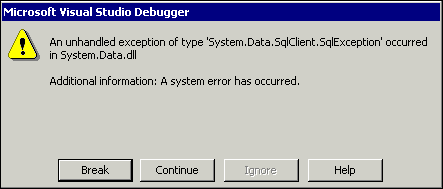 As you can see, the dialog box indicates that an unhandled SQL exception has occurred and gives the programmer a few choices: Break Pauses execution, leaving Visual Studio in break mode. While in break mode, you can examine variable values, enter commands in the Immediate window, and even press F5 to attempt to continue. In our example, you could change the value of the ConnectionString property to contain the correct password and successfully continue execution. Continue Continues execution, passing control to the exception handler. In our example, we have not written an exception handler, so Visual Studio just dumps detailed information to the Output window and ends the program. Ignore Continues execution, skipping over the exception handler. Visual Studio purposely disables this option in some cases, and most of the time, choosing it is probably not a good idea. In our example we cannot connect to our SQL database, so ignoring this fact certainly won't allow us to retrieve data from it! Breaking for Handled Exceptions By default, when an unhandled exception is thrown within the Visual Studio environment, several options are provided, such as entering break mode to troubleshoot the error. However, if you have written exception-handling code, Visual Studio will execute it when an exception occurs. For example, suppose we add a Try ... Catch statement to our sample code: Try cn.Open Catch ex As Exception MessageBox.Show("Error occurred: " & ex.ToString) Application.Exit End Try This code simply displays a message and ends the program when any exception occurs, essentially duplicating the effect of clicking the Continue button in Figure 26.1. As a developer you may not always want to automatically execute the exception handler, because it will end the program before you have the opportunity to troubleshoot the problem. Fortunately, Visual Studio allows you to customize its behavior using the Exceptions dialog box, pictured in Figure 26.2. Figure 26.2. The Exceptions dialog box can be displayed from the Debug menu or by pressing Ctrl+Alt+E. 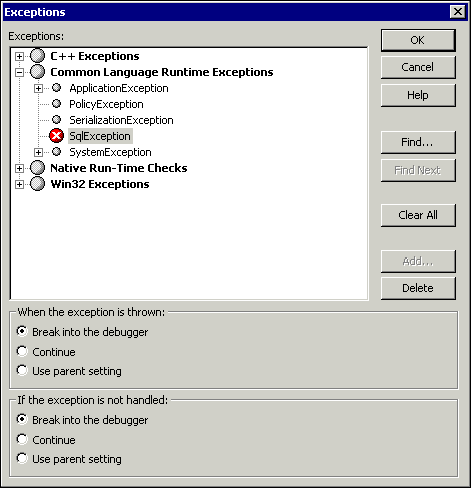 The Exceptions dialog box allows you to determine whether Visual Studio will break or continue based on the exception type. By default, Visual Studio will continue if you have written exception-handling code, or break if you have not. In our example, the type of exception being thrown is a SqlException. By using the Exceptions dialog box, we could tell Visual Studio to break on the exception even though we have written code to handle it, thus changing the default behavior. To cause Visual Studio to break on SQL exceptions regardless of whether they are handled in code, click the Add button and add SQLException to the exception list. Then, select the Break into the Debugger option, as shown in Figure 26.2. Note As you can see from Figure 26.2, the exception list is organized in a tree structure. Therefore, changing the break settings at the Common Language Runtime level would break on not only our exception but all other Common Language Runtime exceptions as well. Following the Call Stack Another useful troubleshooting tool is the Call Stack window. The Call Stack window, pictured in Figure 26.3, provides you the ability to follow the sequence of procedure calls in your program. This is especially important where procedures call other procedures that in turn call other procedures, and so on. The Call Stack window can be displayed from the Windows submenu of the Debug menu. Figure 26.3. The Call Stack window provides a trail of procedure calls so you can determine how you arrived at the statement that caused an error. 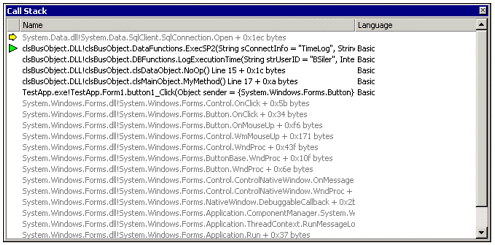 Each time a method is called, an entry with the method name and parameter values is added to the call stack. Note that you do not need to be troubleshooting an error to use the call stack; it can be displayed any time you are in break mode, such as when you are using breakpoints. For more on breakpoints, p. 129 |