In this project we will create a simple Billiards application in which the user can hit a ball with a stick. It's a very simple demonstration of how to use event handlers in a project. 1. | Open Billiards1.fla in the Lesson07/Start folder.
This FLA file has the following four layers: Actions, Ball, Stick and Background. The Actions layer is where we will place all the actions for this project. The Ball layer contains a MovieClip with an instance named ball, and the Stick layer contains a MovieClip with an instance named stick. The Background layer contains our green billiards table background image.
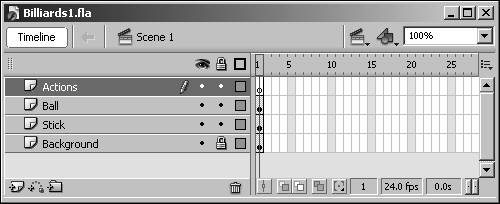 After our project is done, the user can drag the stick to the right and let up to hit the ball. Then the ball will roll a distance as determined by the distance the stick was pulled back, which simulates the action of hitting the ball with the stick.
| 2. | With the Actions panel open, select Frame 1 of the Actions layer and add the following script:
var stickStart:Number = stick._x; var stickStop:Number = stick._x + 100; var ballStart:Number = ball._x; var ballStop:Number = ball._x - 350; var isBallRolling:Boolean = false; var rollDistance:Number = 0; In this script we set some initial variables that we'll be using from inside our event handlers. The first four variables will help us track the positioning of the stick and ball. The first variable, named stickStart, represents the initial position of the stick MovieClip on the x axis. The second variable, named stickStop, represents the maximum position the stick can be moved on the x axis. The third variable, named ballStart, represents the initial position of the ball on the x axis. The fourth variable, named ballStop, represents the maximum distance the ball can move on the x axis.
The fifth variable, named isBallRolling, is a Boolean value that represents whether the ball is currently rolling or moving. It is initially set to false, but after we hit the ball it is set to true until it has completed its move.
The final variable, named rollDistance, is set when the ball is initially hit. As the ball moves, this value is reduced until it is less than or equal to zero. When it reaches that point, we know the move is complete.
| 3. | Add the following event handler after the script you added in Step 2:
stick.onPress = function() { if(!isBallRolling) { ball._x = ballStart; this.startDrag(false, stickStart, this._y, stickStop, this._y); } } This first event handler is added to the stick MovieClip and enables us to drag the stick away from the ball. We want the code in this event handler to execute only if the ball is not currently rolling, so we place it inside an if statement. Inside the if statement, we reset the ball position and then start the drag.
However, if you run this script, you'll notice that the drag doesn't stop because we need to stop the drag manually when the stick's onRelease event is fired.
| 4. | Add the following event handler after the script you added in Step 3:
stick.onRelease = function() { if(!isBallRolling) { this.stopDrag(); var power:Number = Math.round(this._x - stickStart) / 100; rollDistance = Math.round((ballStart - ballStop) * power); this._x = stickStart; isBallRolling = true; } } This event handler is also added to the stick MovieClip. When the mouse is released, this event is fired. Once again, we want this code to run only if the ball is not currently rolling, so we again place our code inside an if statement.
Inside the if statement we first want to stop the drag. Then we need to calculate the distance we want the ball to travel based on the distance the stick was pulled back.
We start this calculation by determining the percent the stick was pulled back. We store this value in a function-level variable named power. Then we need to set the initial value of the rollDistance variable using our ballStart, ballStop, and power variables.
Then we reset the position of the stick to the start position and set the isBallRolling variable to TRue. In our next script you'll see that by setting this variable to true, our ball will begin moving.
| 5. | Add the following event handler after the script you added in Step 4:
ball.onEnterFrame = function() { if(isBallRolling) { this._x -= 5; rollDistance -= 5; if(rollDistance <= 0) { isBallRolling = false; } } } This event handler will be added to the ball MovieClip instance. The onEnterFrame event handler is called each time the frame is rendered and is executed at the same rate as your Movie's frame rate. Our Movie's frame rate value is set to 24 frames per second (fps). Therefore, our onEnterFrame event will be fired 24 times per second.
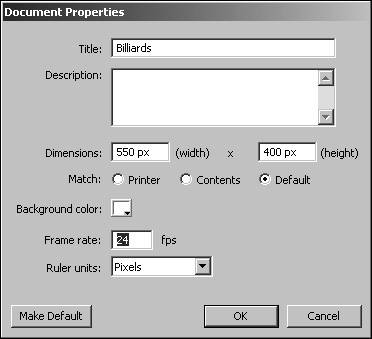 Because we want to execute the code inside this event handler only if the ball is currently rolling, we will wrap the code in an if statement. We will start subtracting 5 from the values of the ball's _x property and the rollDistance variable. This will move the ball five pixels to the left each time the onEnterFrame event is fired. We then check whether the rollDistance variable is less than or equal to 0. If it is, we set the isBallRolling variable to false, which stops the ball from rolling any farther and enables another shot to be made.
| 6. | From the menu, choose Control > Test Movie to see the movie in action.
Interact with the project by clicking the stick MovieClip, dragging it to the right, and then releasing it. You should see the ball move to the left, and the distance should be proportionate to the distance you pull the stick back.
| 7. | Close the test movie to return to the authoring environment. Save your project as Billiards2.fla.
This completes the exercise. You should now have a basic understanding of event handlers and the ways you can use them to enhance your projects.
| |