Flash Player has its humble beginnings in the world of vector animations. And although it has added a lot of new functionality over the years, vector animations remain one of its strengths. Vectors generally require significantly less data than rasterized bitmaps. The latter requires data for each pixel, whereas the former only requires data for things such as line segment end points and fill color. That means that vectors are typically much more compact than bitmaps (except in the case of extremely complex vector artwork). Additionally, although bitmaps don't scale well, vectors do. Although vectors have many advantages, there are some potential drawbacks. In particular, we want to discuss issues related to translating complex vector artwork within Flash Player. Translating means moving the object in the x and y coordinate space. Every time a movie clip with vector artwork tweens across the Stage, Flash Player has to re-plot each line segment within the vector artwork. For the majority of vector shapes, that is still a relatively inexpensive operation. However, the more complex the vector artwork, the more points Flash Player has to re-plot. The result is that in some cases Flash Player maxes out on its use of system resources attempting to re-plot each point within the vector and it starts to run more slowly. Animations can then become noticeably slower and/or choppier. Contrast that with how Flash Player translates bitmaps. Flash Player treats bitmaps as single units. It does not attempt to shift the bitmap one pixel at a time. It simply translates the entire bitmap unit, which makes bitmap translation an inexpensive operation compared with complex vector artwork translation. Wouldn't it be nice if there were some way in which you could tell Flash Player to treat movie clips containing complex vector artwork as though they contained bitmaps? Starting with Flash Player 8, the MovieClip class enables that very functionality with the cacheAsBitmap property. The cacheAsBitmap property lets you tell Flash Player to take a snapshot of the vector artwork and use that snapshot as a bitmap surface for the movie clip. Flash Player then translates the movie clip as though it contained a bitmap. The result can potentially be smoother animations. The cacheAsBitmap property expects a Boolean value. The default value is false, so no bitmap surface is used. A value of true tells Flash Player to use a bitmap surface. exampleClip.cacheAsBitmap = true; Although caching bitmap surfaces is a useful solution in some cases, it is not a catch-all to use with every movie clip. Caching bitmap surfaces is not without some cost. When a movie clip has a cached bitmap surface, it must redraw the surface whenever the following events occur: If any of the preceding events occur with the frequency of a movie clip, caching a bitmap surface can be more detrimental than helpful. For example, if a movie clip rotates while translating, as long as cacheAsBitmap is true it is even more expensive than previously. In such a case, the movie clip has to rotate, translate, and then redraw the bitmap surface. Caching bitmap surfaces is a useful feature when used correctly. Use the feature only when you notice that it is required. For example, if you have complex vector artwork that tweens across the Stage and you notice that the animation slows down at that point, you can set the movie clip or movie clips containing the artwork to use a cached bitmap surface. In this next task, you'll make a snowfall scene animation using bitmap caching to optimize the animation of the snowflakes. The snowflakes are each movie clips containing complex vector artwork. As you'll see in Step 7, the animation can start to run slowly. So as a solution you'll set each movie clip to use a cached bitmap surface. 1. | Open snowflake1.fla from the Lesson09/Start directory.
The snowflake1.fla document contains the necessary elements. Specifically, it contains a movie clip symbol called Snowflake.
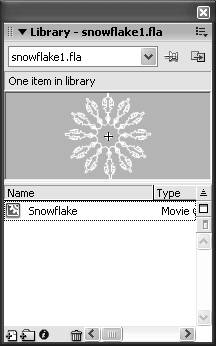 | 2. | Select the Snowflake movie clip symbol in the library, and open the Linkage Properties dialog box. Check the Export For ActionScript option. Use the default linkage identifier of Snowflake. Click OK.
To add instances of the symbol programmatically, you'll need to adjust the linkage properties for the movie clip. You can do so from the Linkage Properties dialog box. You can access the dialog box either by way of the library menu (select Linkage) or from the right-click/Ctrl-click context menu for the library symbol (select Linkage).
Set the symbol to Export For ActionScript so that you can attach instances using attachMovie().
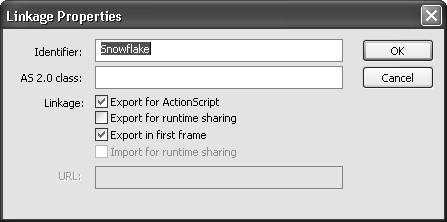 | 3. | Select the first keyframe on the Actions layer from the main Timeline, and open the Actions panel by pressing F9. Then add an import statement to import the Tween class.
import mx.transitions.Tween; You'll use the Tween class to animate the snowflakes. The Tween class is installed in the global Flash classpath with the default installation of Flash 8. However, it's in a package called mx.transitions. To reference the class simply as Tween throughout the rest of the code, you can use the import statement at the beginning of the code.
| 4. | Following the import statement, add the following line of code:
setInterval(this, "addSnowflake", 250); The setInterval() function tells Flash Player to call a function at a specified interval. In this case, you're telling Flash Player to call the addSnowflake() function every 250 milliseconds.
| 5. | Define the addSnowflake() function following the preceding code.
function addSnowflake():Void { // Add each snowflake to a unique depth. var depth:Number = this.getNextHighestDepth(); var snowflakeClip:MovieClip = this.attachMovie("Snowflake", ¬ "snowflakeClip" + depth, depth); // Make a random scale factor from 20 to 100. Then set the // _xscale and _yscale properties of the snowflake movie clip // to that scale factor so that the snowflakes have different // sizes. var scaleFactor:Number = Math.random() * 80 + 20; snowflakeClip._xscale = scaleFactor; snowflakeClip._yscale = scaleFactor; // Set the x coordinate of the snowflake to a random number // between 0 and 550. snowflakeClip._x = Math.random() * 550; // Use a Tween object to animate the snowflake such that it // falls from the top of the stage to the bottom of the stage. // Add a listener so that Flash Player gets notified when the // animation has completed. var snowflakeTween:Tween = new Tween(snowflakeClip, "_y", ¬ null, 0, 400, Math.random() * 50 + 100); snowflakeTween.addListener(this); } The inline comments provide the detailed description of the preceding code. Generally, the addSnowflake() function adds a new instance of the Snowflake symbol to the Stage. It scales the instance to a random value between 20 and 80 percent, and it places the instance at a random x coordinate along the top of the Stage. It then uses a Tween object to start an animation that causes the snowflake to fall from the top to the bottom of the Stage.
| 6. | Define the onMotionFinished() function following the addSnowflake() function.
function onMotionFinished(tweenObject:Tween):Void { tweenObject.obj.removeMovieClip(); } Because the main Timeline is the listener object for each Tween object, Flash Player automatically calls a function named onMotionFinished() when each tween completes. The function gets passed a reference to the Tween object that just completed the animation.
Within the onMotionFinished() function you can tell Flash Player to delete the corresponding movie clip using removeMovieClip(). Note that each Tween object has an obj property that references the targeted movie clip.
| 7. | Test the movie.
When you test the movie you'll see snowflakes falling. Initially the animation ought to playback smoothly. However, as more snowflakes are added to the scene you'll likely notice that the animation slows significantly.
| 8. | Edit the ActionScript code by adding the bolded line of code to the addSnowflake() function.
function addSnowflake():Void { var depth:Number = this.getNextHighestDepth(); var snowflakeClip:MovieClip = this.attachMovie("Snowflake", ¬ "snowflakeClip" + depth, depth); var scaleFactor:Number = Math.random() * 80 + 20; snowflakeClip._xscale = scaleFactor; snowflakeClip._yscale = scaleFactor; snowflakeClip._x = Math.random() * 550; snowflakeClip.cacheAsBitmap = true; var snowflakeTween:Tween = new Tween(snowflakeClip, "_y", ¬ null, 0, 400, Math.random() * 50 + 100); snowflakeTween.addListener(this); } The cacheAsBitmap property is false by default. By setting the property to true you're telling Flash Player to use a cached bitmap surface for the snowflake movie clip. That means Flash Player can translate the movie clips as bitmaps rather than as vectors.
| 9. | Test the movie again.
When you test the movie this time, you'll likely notice that the animation plays without slowing. The bitmap caching enables Flash Player to translate the snowflake movie clips as efficiently as possible.
| |