1.3. Set the Focus of Controls In earlier versions of ASP.NET, assigning focus to a control involves writing client-side script (such as JavaScript). In ASP.NET 2.0, this process has been much simplified, and you can now set the focus of a control via its Focus( ) method. Consider the example shown in Figure 1-10. If the user clicks on the Single radio button, you would expect the focus to now be assigned to the Age text box. If the Married option is selected, the Spouse Name text box should get the focus. Figure 1-10. Setting the focus of controls 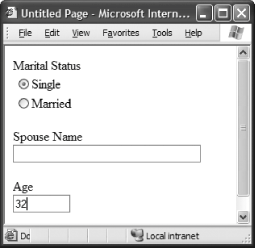 Note: Look, no JavaScript! Now you set the focus of controls using the new Focus property. 1.3.1. How do I do that? To try out the new Focus( ) method, work through the following steps to implement the form shown in Figure 1-10. In Visual Studio 2005, create a new ASP.NET 2.0 web application and name it C:\ASPNET20\chap01-ControlFocus. Add a radio button list and two text box controls to the default Web Form and give them the names shown in Figure 1-11. Figure 1-11. Populating the default form with the various controls 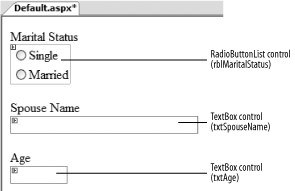
Populate the RadioButtonList with two items: Single and Married. To do so, switch to Source View and add the following bold lines: <asp:RadioButtonList runat="server" AutoPostBack="True"> <asp:ListItem>Single</asp:ListItem> <asp:ListItem>Married</asp:ListItem> </asp:RadioButtonList><br /> To ensure that the Spouse Name text box gets the focus when the user selects the Married radio button, and the Age text box gets the focus when the user selects the Single radio button, you need to set the AutoPostBack property of the RadioButtonList control (rblMaritalStatus) to TRue. This can be done either in the Tasks Menu of the RadioButtonList control (check the Enable AutoPostBack option) or via the Properties window. To implement the desired focus rules described in the previous step, double-click the RadioButtonList control to reveal the code-behind, and add the following code to the page: Protected Sub rblMaritalStatus_SelectedIndexChanged( _ ByVal sender As Object, _ ByVal e As System.EventArgs) _ Handles rblMaritialStatus.SelectedIndexChanged If rblMaritialStatus.SelectedValue = "Single" Then txtAge.Focus( ) Else txtSpouseName.Focus( ) End If End Sub Tip: You can also set the focus of a control through the SetFocus( ) method of the Page class. The syntax is: Page.SetFocus(controlName) 1.3.2. What about... ...setting a default button on a Web Form? In ASP.NET 2.0, you can set a default button on a form. For example, Figure 1-12 shows a page with two TextBox controls and two Button controls. You can configure the Submit button to be the default button so it is automatically clicked when a user presses the Enter key. Figure 1-12. Setting the Submit button as the default button of the form 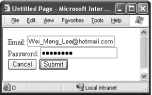 To try this out on your own, follow these steps: Note: In ASP.NET 2.0, you can now set a default button on a page so that when the user presses the Enter key the button is automatically invoked. Create a form like the one shown in Figure 1-13. Figure 1-13. A Web Form with two TextBox controls and two Button controls 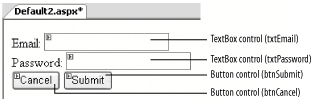
Switch the Web Form to Source View and then add the lines of code shown in bold. The defaultfocus attribute sets the control that will be assigned the focus when the form is loaded. The defaultbutton attribute sets the button to be activated when the Enter key is pressed: <form runat="server" defaultbutton="btnSubmit" defaultfocus="txtEmail" > <div> Email: <asp:TextBox runat="server" Width="174px"></asp:TextBox> <br /> Password: <asp:TextBox runat="server" Width="153px" TextMode="Password"></asp:TextBox> <br /> <asp:Button runat="server" Text="Cancel" /> <asp:Button runat="server" Text="Submit" /><br /> <br /> </div> </form> Press F5 to load the form; note that the focus is on the Email text box (see Figure 1-14), and the Submit key is set as the default button. Figure 1-14. The Email text box has the focus, and the Submit button is the default button 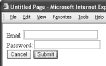 1.3.3. Where can I learn more? The Focus( ) method in ASP.NET 2.0 works on the server side; hence, every time you need to set the focus of a control, you need to initiate a postback. If you want to set the focus of controls on the client side without a postback, check out the following two links: |