1.4. Define Multiple Validation Groups on a Page In ASP.NET 1.x, all controls in a single page are validated together. For example, suppose you have a Search button and a Get Title button, as shown in Figure 1-15. If you use a RequiredFieldValidator validation control on the ISBN text box, then clicking the Search button will not result in a postback if the ISBN text box is empty. This is because the entire page is invalidated as long as one control fails the validation. Figure 1-15. Multiple forms on a page 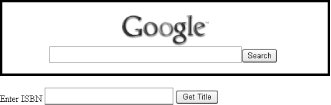 Note: By grouping controls into validation groups, you can now independently validate collections of controls. In ASP.NET 2.0, group validation allows controls in a page to be grouped logically so that a postback in one group is not dependent on another. 1.4.1. How do I do that? The best way to explore this new feature is to create a form with multiple postback controls, add a validator to one of them, and then assign each control to a separate group. In this lab, you'll implement the form shown in Figure 1-15. Launch Visual Studio 2005 and create a new web site project. Name the project C:\ASPNET20\chap01-Validation. Populate the default Web Form with the Panel, TextBox, and Button controls shown in Figure 1-16. Figure 1-16. A page with multiple controls 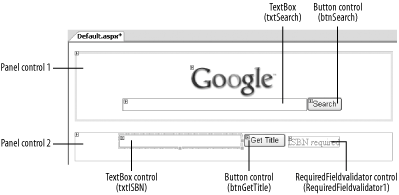
In the top panel (Panel control 1), you'll configure the Search button to post the search string to the server, which in turn will redirect to Google's site. To do so, double-click the Search button (btnSearch) and enter the following code on the code-behind to redirect the search string to Google's site: Sub btnSearch_Click(ByVal sender As Object, _ ByVal e As System.EventArgs) _ Handles btnSearch.Click Dim queryStr As String = HttpUtility.UrlEncode(txtSearch.Text) Response.Redirect("http://www.google.com/search?q=" & queryStr) End Sub The TextBox control contained in the bottom panel (Panel control 2) will take in an ISBN number and perhaps perform some processing (like retrieve details of a book from Amazon.com). Associate the RequiredFieldValidator control with the ISBN text box (you can configure it in Source View): <asp:RequiredFieldValidator Runat="server" ErrorMessage="RequiredFieldValidator" ControlToValidate="txtISBN" SetFocusOnError="True"> ISBN required</asp:RequiredFieldValidator> To divide the controls into two separate validation groups, switch to Source View and use the ValidationGroup attribute, as shown in the following code sample (highlighted in bold): ... <asp:TextBox Runat="server" Width="320px" Height="22px"> </asp:TextBox> <asp:Button Runat="server" Text="Search" ValidationGroup="SearchGroup" /> ... <asp:TextBox Runat="server" Width="212px" Height="22px"> </asp:TextBox> <asp:Button Runat="server" Text="Get Title" ValidationGroup="BooksGroup" /> <asp:RequiredFieldValidator Runat="server" ErrorMessage="RequiredFieldValidator" ControlToValidate="txtISBN" ValidationGroup="BooksGroup">ISBN required </asp:RequiredFieldValidator> ... Here, the Search button is assigned the SearchGroup validation group, while the Get Title button and the RequiredFieldValidator are both assigned to the BooksGroup validation group. Press F5 to test the application. Now you can click the Search and Get Title buttons independently of each other. Tip: Controls that do not set the ValidationGroup attribute belong to the default group, thus maintaining backward compatibility with ASP.NET 1.x. 1.4.2. What about... ...setting the focus on the field on error? All validation controls in ASP.NET 2.0 now support the SetFocusOnError property. Simply set a validator control's SetFocusOnError attribute to True so that the browser will set the focus on the control on error. In the previous example, if the RequiredFieldValidator control has the SetFocusOnError attribute: <asp:RequiredFieldValidator Runat="server" ErrorMessage="RequiredFieldValidator" ControlToValidate="txtISBN" ValidationGroup="BooksGroup" SetFocusOnError="True"> Then the ISBN text box will get the focus if you click the Get Title button when the ISBN text box is empty. 1.4.3. Where can I learn more? If you are interested in simulating the validation group behavior of ASP.NET 2.0 in ASP.NET 1.x, check out http://weblogs.asp.net/skoganti/archive/2004/12/05/275457.aspx. |