In general, a loop continues to perform iterations until its condition is no longer true . There are two actions you can use to change this behavior: continue and break . With the continue action, you can stop the current iteration (that is, no actions in that iteration will be executed) and jump straight to the next iteration in a loop. Take, for example, the following: total = 0; i = 0; while (++i <= 20) { if (i == 10) { continue; } total += i; } The while statement in this script loops from 1 to 20, and with each iteration, adds the current value of i to a variable named total except when i equals 10. When i equals 10, the continue action is invoked, which means no more actions are executed on that iteration, and the loop skips to the eleventh iteration. This would create a set of numbers that read "1 2 3 4 5 6 7 8 9 11 12 13 14 15 16 17 18 19 20." Note that there's no number 10, indicating that no action occurred on the tenth loop. The break action is used to exit a loop even if the condition that keeps the loop working remains true . Take, for example, the following: total = 0; i = 0; while (++i <= 20) { total += i; if (total >= 10) { break; } } This script increases the value of a variable named total by 1 with each iteration. When the value of total is 10 or greater (as checked by an if statement, as shown), a break action occurs and the while statement halts, even though it's set to loop 20 times. In this exercise, you'll use continue and break in a simple search routine. -
Open phoneNumberSearch1.fla in the Lesson10/Assets folder. This file contains two layers: Actions and Search Assets. The Actions layer will contain the search routine for this project. The Search Assets layer contains the text fields, button, and graphics for this exercise. 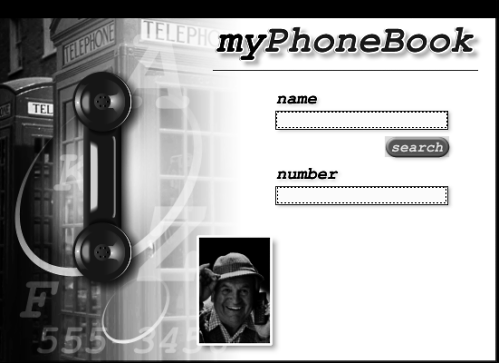 In this exercise, you'll produce a simple application that lets you enter a name in a search field to return the phone number for that individual. There are two text fields on screen, one with an instance name of name that will be used to enter the name to search, and the other with an instance name of result that will be used to display the search result. There is an invisible button over the Search button graphic that will be used to call a search function. -
With the Actions panel open, select the first frame in the Actions layer and add the following script: info = [["John","919-555-5698"],["Kelly","232-555-3333"],["Ross","434-555- 5655"]]; This creates a two-dimensional array called info , which contains three elements, each of which is its own array, or sub-array. The first element of each sub-array is a name, and the second element of each sub-array is a phone number. 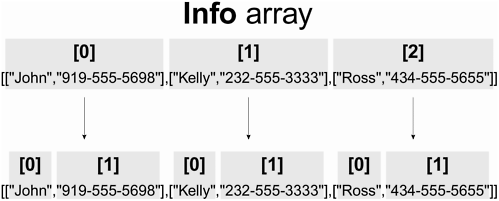 To access the first name in this array, you would use info[0][0] ; the first phone number would be accessed using info[0][1] . This represents John's name and number. This syntax will play an important role in the search function we're about to script. -
Add the following function definition just under the info array: function search () { matchFound = false; i = -1; while (++i < info.length) { } } You've begun to define the function that will search the info array for a specific phone number. The first action in this function creates a variable called matchFound and assigns it an initial value of false . (We'll show you how this variable will be used in a moment.) We've set up the while statement to loop once for every element in the info array. -
Add the following actions to the while loop in the search() function: if (info[i][0].toLowerCase() != name.text.toLowerCase()) { continue; } result.text = info[i][1]; matchFound = true; break; } With each iteration of the loop, the above if statement uses the current value of i to determine whether there are any mismatches between names in the info array (made all lowercase) and the user-entered name in the name text field (forced to lowercase). When a mismatch is encountered, the continue action within the if statement is evoked and you skip to the next loop. By using toLowerCase() to convert any names to lowercase, the search becomes case-insensitive. If a name in the info array and the name text field match, continue is not invoked and the actions after the if statement are executed: Using the value of i at the time a match was found, the first action sets the value of the variable result.text to the matching phone number; matchFound is set to true ; and the break action is executed to halt the loop. To demonstrate how this works, imagine that someone has entered Kelly into the name text field. The location of "Kelly" in the info array is as follows: info[1][0] On the first iteration of the loop, the value of i is 0 , which means the if statement in the loop would look like the following: if (info[0][0].toLowerCase() != name.text.toLowerCase()) { continue; } Here the statement asks if "john" (the name at info[0][0] , made lowercase) is not equal to "kelly'" (entered into the name text field, made lowercase). Since "john" does not equal "kelly," the continue action is invoked and the next loop begins. Because i is incremented by one with each loop, on the next iteration, the if statement will look like the following: if (info[1][0].toLowerCase() != name.text.toLowerCase()) { continue; } Here the statement is asking if "kelly" (the name at info[1][0] , made lowercase) is not equal to "kelly" (entered into the name text field, made lowercase). Since "kelly" does equal "kelly," the continue action is skipped and the next three actions are executed. The first action sets the value of result.text to info[i][1] . Because i has a value of 1 when this is executed, result.text is set to info[1][1] Kelly's phone number, which is now displayed. Next, matchFound is set to true and the break action exits the loop. TIP Although the break action is not a necessity, it helps shorten search times. Think of how much time you could save by using a break action to avoid unnecessary loops in a 10,000-name array! -
Add this final if statement as the last action in the search() function: if (!matchFound) { result.text = "No Match"; } This statement, which is not part of the loop, checks to see whether matchFound still has a value of false (as it was set initially) once the loop is complete. If it does, the action in this if statement sets the value of result.text to "No Match." The syntax !matchFound is a shorthand way of writing matchFound == false . -
With the Actions panel open, select the invisible button over "search" and add the following script: on (release, keyPress "<Enter>") { search(); } 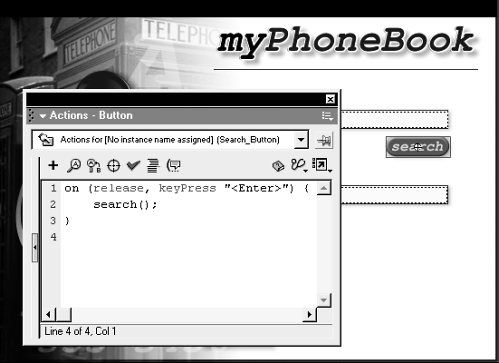 If the mouse is released or the Enter key pressed, the search() function will be called. -
Chose Control > Test Movie. Enter John, Kelly, or Ross into the search field and press the Search button. Enter any other name and then press the Search button. A phone number should appear when a name is valid, and "No Match" should appear when a name is not contained within the info array. -
Close the test movie and save your work as phoneNumberSearch2.fla. In this exercise, you used the loop exceptions continue and break to create a search routine. You'll soon find out that in practice, the break command is more common than the continue command: This is because programmers often use if statements rather than continue to bypass actions in a loop. |