In non-dynamic projects, sounds are placed directly on, and controlled from, the timeline. This means that if you want a sound to play in your movie, you must drag it from the library onto the timeline and then indicate when and how long it should play as well as how many times it should loop on playback. Although this may be a fine way of developing projects for some, we're ActionScripters which means we want control! That's why in this exercise we're going to show you how to leave those sounds in the library and call on them only when you need them. Using some more methods available to Sound objects, you'll learn how to add sounds and control their playback all on the fly. When you create a Sound object in Flash, one of the most powerful things you can do with it is attach a sound in essence pulling a sound from the library that can be played or halted any time you wish. To do this, you must assign identifier names to all of the sounds in the library. Once these sounds have identifier names, you can attach them to Sound objects and control their playback, even their volume and panning, as we discussed earlier in this lesson. For example, let's assume there's a music soundtrack in the project library with an Identifier name of "rockMusic." Using the following code, you could dynamically employ it in your project and control its playback: on (release) { music = new Sound (); music.attachSound ("rockMusic"); music.start (0, 5); } The first line of this script shows that it's executed when the button it's attached to is released. When executed, the first line creates a new Sound object named music . The next line attaches the "rockMusic" sound (in the library) to this Sound object. The next line starts the playback of this Sound object, which in effect starts the playback of the "rockMusic" soundtrack (because it's attached to this Sound object). The 0 in this action denotes how many seconds into the sound to start playback. For example, if the "rockMusic" soundtrack includes a guitar solo that begins playing 20 seconds into the soundtrack, setting this value to 20 would cause the sound to begin playback at the guitar solo as opposed to its actual beginning. The second value in this action, which we've set to 5, denotes how many times to loop the sound's playback. In this case, our soundtrack will play five times before stopping. In addition, you can set all of these values using variables or expressions opening a world of possibilities. In the following exercise, we'll show you how to attach a random sound from the library to a Sound object and trigger its playback whenever the mouse button is pressed. The number of times this sound loops will be random as well. We'll also set up our script so that pressing any key halts the sound's playback. -
Open basketball5.fla in the Lesson16/Assets folder. If you wish, you can simply continue to use the file you were working with at the end of the previous exercise. -
Choose Window > Library to open the Library panel. The library contains a folder called Dynamic Sounds. In this folder you'll find three sounds that have been imported into this project. These sounds only exist within the library; they have not been placed on our project's timeline yet. 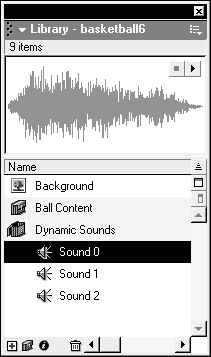 -
Click on Sound 0 to select it. From the Library Option menu, choose Linkage. The Symbol Linkage Properties dialog box appears. This is where you assign an identifier name to the sound. -
Choose Export for ActionScript from the Linkage options, and give this Sound an identifier name of Sound0. 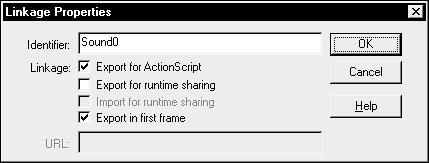 NOTE A discussion of the settings in this dialog box can be found in Lesson 14, Controlling Movie Clips Dynamically. The reason we've used an identifier name ending in a number is that our script generates a random number between 0 and 2: When 0 is generated, Sound0 will play; when 1 is generated, Sound1 will play; when 2 is generated, Sound2 will play. Thus, that number at the end of our identifier name is critical. When you've assigned an identifier name to the sound, Click OK. -
Repeat Steps 3 and 4 for Sound 1 and Sound 2 in the library. Give Sound 1 an identifier name of Sound1 and Sound 2 an identifier name of Sound2. NOTE Although library-item names can contain spaces, identifier names cannot. When assigning identifier names, follow the same naming rules that apply to variables. At this point, the three sounds in the Dynamic Sounds folder have been given identifier names of Sound0, Sound1, and Sound2. Next, we'll use them in a script. -
Select the basketball movie clip instance, then open the Actions panel. After the line of script that creates the bounce Sound object we worked with earlier, add the following line of script: dynaSounds = new Sound(); This creates a new Sound object called dynaSounds . As with the bounce Sound object, this Sound object is created when the basketball movie clip instance is loaded into the scene. When this occurs, there will be two Sound objects in our project, one that controls the bouncing sound and another (the one we just added) to randomly play the three sounds in the library. You'll notice that when we created this Sound object, we didn't associate it with a timeline. This means that our new Sound object will be associated with the entire project a "'universal" Sound object, so to speak. -
Add the following lines to the end of the current script: onClipEvent (mouseDown) { randomSound = random (3); randomLoop = random (2) + 1; dynaSounds.attachSound ("Sound" + randomSound); dynaSounds.start (0, randomLoop); } 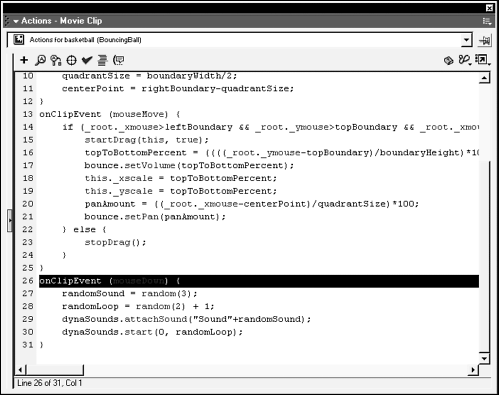 The mouseDown event handler causes these lines of script to be triggered whenever the mouse is pressed down anywhere on the stage. Here's what this script does: First, the expression random(3); generates one of three values between 0 and 2 and then assigns this value to the randomSound variable. This random value will be used further down in the script to determine which sound from the library will be attached to the dynaSounds Sound object and then played. The next line in our script contains another expression for generating one of two random values. This random value is then assigned to the randomLoop variable. This random value will be used further down in the script to determine how many times our sound should loop. You'll notice that this expression differs slightly from the first: We've added + 1 to it. Without it, random (2) will only generate a value of 0 or 1, leaving randomLoop with a value of only 0 or 1. By adding + 1 to the expression, the value generated by random(2) will have 1 added to it, giving us the possible values of 1 (0 + 1) or 2 (1 + 1). Since this variable is used to determine how many times our sound loops, values of 1 or 2 will cause the sound to play once or twice. Zero is a useless value in this circumstance. POWER TIP You can use this trick whenever you wish to generate a random value between a specific range of numbers. For example, the expression random(51) + 50; will generate a random number between 50 and 100. Here's the formula: -
Determine the highest number you want generated (maybe 500) -
Determine the lowest number you want generated (maybe 150) -
Subtract the lowest number from the highest (in our example we get 350) -
Add 1 to this result (in our example we get 351). This step is necessary to offset the fact that the random() function starts generating numbers at 0 -
Create your expression (in our example this would be random(351) + 150 , which will generate a random number between 150 and 500) After its execution, the script will have generated two random numbers. These values will now be used in the remaining two lines. dynaSounds.attachSound ("Sound" + randomSound); Using an expression, this action attaches a sound from the library to the dynaSounds Sound object, based on the current value of randomSound . If the current value of randomSound is 2, this would be the same as writing this line of script as: dynaSounds.attachSound ("Sound2"); With each click of the mouse, a new number is generated and thus the sound attached to this Sound object can change. Finally, to play this Sound object, the following line is used: dynaSounds.start (0, randomLoop); This will play the current sound attached to this Sound object. For the two parameters of this action, 0 will cause the sound to playback from its actual beginning, and the current value of randomLoop determines how many times the sound will loop (once or twice). -
Add the following lines to the end of the current script: onClipEvent (keyDown) { dynaSounds.stop(); } 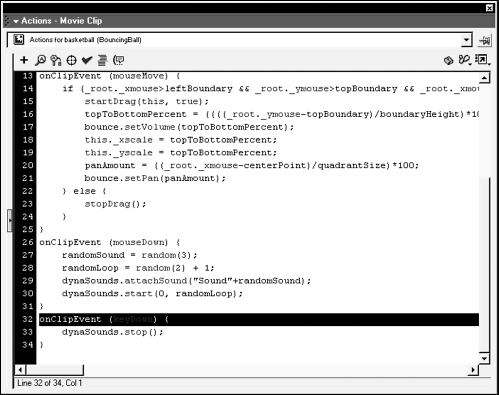 The keyDown event handler causes this line of script to be triggered whenever a key is pressed. When this action is executed, playback of the dynaSounds Sound object is stopped, regardless of where it is in its playback. -
Choose Control > Test Movie to see how the movie operates. In the testing environment, clicking the mouse causes a random sound from the library to play. If you press a key while the sound is playing, the sound will stop. -
Close the testing environment to return to the authoring environment. Save the current file as basketball6.fla. This completes the project! You should now be able to see how dynamic sound control enables you to add realism to your projects and in the process makes them more memorable and enjoyable. You can do all kinds of things with sounds, including loading them from an external source (which we'll describe in Lesson 17, Loading External Content. |