You already learned how to load external content in Lessons 6 and 9. You saw that you can load JPEG, PNG, GIF, and SWF files into either movie clips or a loader component. What you haven't done yet is to let someone know that content is in fact loading, or, what's more, switched out what content goes into a Loader or movie clip instances based on a user interaction. You're about to do that in this next exercise. Here's the rationale: You could have a separate Loader component instance or movie clip instance in some other keyframe in a Timeline; when a user clicks a button, you go to that keyframe with the new instance and load the new content in the interface at that point. This is overcomplicated and an inefficient use of end user resources. Every instance of a Loader component or movie clip that you make creates a new object in the end user's memory, which consumes resources. It makes more sense then to reuse a single object as often as possible, which is the goal of this next exercise. Before we get started, you will be using two dynamic text fields to display information: One will display the download progress of the file being loaded into a Loader component instance; the other will display information about a section of the website, depending on which button was clicked. All this will happen in a one-frame Timeline and will be controlled with ActionScript. You already added Loader components to the Stage, drawn graphics, and made or used Button components, so the foundation of the file has already been created for you to save a little work and time. This leaves you free to focus on dynamic text fields and the ActionScript you have to write. 1. | Open lesson10/start/tour_start.fla from this book's CD-ROM and save it as tour.fla in your TechBookstore folder on your hard drive.
Take a moment to look at the construction of this file: You have an empty actions layer, which you'll add ActionScript to in a few moments. You have a loaderdisplay layer, in which you will create a dynamic text field to display the progress of download of any file going into the Loader component instance on the Stage. The components layer contains three buttons with the instance names btnReviews, btnCompany, and btnContact, respectively. It also contains the Loader component instance that you will load SWFs into. The frame layer just contains a graphic the same size as the Stage and another the same size as the Loader component instance on the Stage. The text fields layer contains some static text right at the moment, but you will also add a multi-line dynamic text field, which will display information retrieved from a text file using the LoadVars class.
| 2. | If you do not already have them in your TechBookstore directory, copy video1.swf, video2.swf, and video3.swf from the lesson10/assets directory into your TechBookstore folder on your hard drive. Also copy sectionText.txt into your Tech Bookstore directory from lesson10/assets.
These are the SWF files that you will be loading. If you look up their properties in Windows Explorer or do a quick Get Info on the Mac, you'll see that they're 1.8 megabytes each. That's embedded video for you! Because we can't really "stream" these files, we'll need to indicate information about their download progress to try and mitigate the file size with user expectation. While they're downloading, you'll give your users some text to read to keep occupied. Sneaky, but effective.
| 3. | Create a dynamic text field on the loaderDisplay layer, a multi-line dynamic text field on the text fields layer.
The loaderDisplay text field will indicate download progress. The text field in the text fields layer will display instructional text about how to use the website, loaded from a text file.
Select the Text tool and press and drag a fixed-width text field over top of the Loader component instance in the loaderDisplay layer. Set the text type to Dynamic. Set the font, font size, and justification to whatever you like; the solution file uses Arial Black, 12 pt, left-justified. Give the text field the instance name percentLoaded. Lock the loaderDisplay layer when you finish adjusting and positioning the text field.
Select the text fields layer and unlock it. Drag a large dynamic text field about 270 pixels wide by 280 pixels tall (it doesn't have to be exact). In the Property inspector, select Multiline from the Line Type drop-down list. Press the Render Text as HTML button so that it is highlighted. Give the text field the instance name displaySection. Reposition the text field so that it appears similar to the one in the following figure.
| 4. | Select frame 1 of the actions layer and open the Actions panel.
There are a number of steps to do in the ActionScript. First, you have to create a reusable function that will handle SWF file loading. Then you have to create an object that handles what should happen when a file is loading, and what should happen when it's done. You also have to make a LoadVars object to load information from a text file, which is nothing new to you by now, and event handlers for buttonsalso nothing new. Consider it practice.
| 5. | Create a new function to load SWF files into the Loader component on the Stage. Name it loadVideo.
If Script Assist mode is on, turn it off now. At the top of the Actions pane, type the following ActionScript:
//loads the video embedded in SWF files using the loader component function loadVideo(pathToFile:String,textToLoad:String):Void { videoLoader.contentPath=""; videoLoader.contentPath=pathToFile; displaySection.htmlText=loadSectionText[textToLoad]; } First, you start with a comment that describes the purpose of the function. Next, you create a new function named loadVideo that accepts two arguments. The first argument, pathToFile, represents the path to the external file on the hard drive or server when it is deployed. The second argument, textToLoad, represents a string of text that will be stored in the LoadVars object when you create it.
Next, videoLoader.contentPath="" is a way of clearing out any content that may already be in the Loader component instance on the Stage. The Loader component on the Stage has the instance name videoLoader, and here you are using ActionScript instead of the Property inspector to say what content should load into the component instance. The next line assigns the path to the file on the hard drive or server when the function is called.
The final line instructs the text field displaySection to treat the text coming into it as HTML-formatted, and then gets its information from the LoadVars object. The assignment loadSection[textToLoad] might look a little strange to you because of the square braces. The square braces tell Flash to fill in the value for textToLoad before the rest of the line is executed, so it's read correctly that way. Otherwise, Flash would be looking for a property in the LoadVars object literally named textToLoad, and not its intended value. When the function runs, the variable textToLoad is populated with its value, the open square brace is converted to a ".", and the close square brace vaporizes. Flash then sees loadSection.productsAndReviews, for example. This process, which is called dynamic evaluation, is a way of saying, "Do this first; then worry about the rest of the line." And you thought you'd never use algebra.
| 6. | Disable the button instances by default.
Underneath all your current code, type the following ActionScript:
//set initial button states btnReviews.enabled=false; btnCompany.enabled=false; btnContact.enabled=false; Again, you start out with an explanatory comment. Buttons and Button components have a property called enabled, which has a true or false value. When enabled is set to false, a user cannot click on the button; if it is a Button component, it is dimmed. In this case, we want to disable the buttons until the LoadVars object we will create has fully loaded its text file. Otherwise, people will click the buttons and not see the text if it hasn't loaded yet. This is an example of usability.
| 7. | Assign default text to the displaySection text field.
Underneath all your current code, type the following lines of ActionScript:
//makes some default text displaySection.htmlText="<p>Press a button below to view more information ¬ about a section of the website</p>"; This assigns HTML-formatted text to the displaySection text field that tells a user what to do next. If you save and test your file at this point, you will see the text appear in the text field, and your buttons will be dimmed.
| 8. | Create a LoadVars object, load a text file, and enable the buttons when the text file is fully loaded.
Underneath all your current script, type the following:
//creates the load vars object for loading the section text var loadSectionText:LoadVars=new LoadVars(); loadSectionText.load("sectionText.txt"); loadSectionText.onLoad=function() { btnReviews.enabled=true; btnCompany.enabled=true; btnContact.enabled=true; } You have worked with the LoadVars object before. The only thing really different here is instead of using it to display text right away, you are using it to make your buttons clickable again. Pretty clever, huh?
Again, this is an example of usability. When users click a button, they expect something to happen. By turning off the buttons until the information fully loads, you're preventing users from getting frustrated when they click something and the application just sits there.
| 9. | Create a listener object that responds to progress and complete events. Add the listener object to the Loader component instance.
You were briefly introduced to the idea of listener objects in Lesson 9. Here, you want single object to handle two tasks. It has to listen for someone to tell it a file is loading; that's the progress event. The "someone" is the Loader instance. We'll get to that in a second. Also, you want it to do something when a file has finished loading. That's the complete event.
Lastly, the listener object barely cares who talks to it, as long as someone addresses it directly. Kind of like a New Yorker. People can scream and whoop and holler all around New Yorkers, and they couldn't care less. However, if someone comes right up to them and says, "Can you tell me the way to Times Square?" they can point you in the right direction if they're of a mind to (but don't trust the young ones, they always get you turned around). In ActionScript, you'll have to tell the Loader component to let your listener object know when it is loading a file or when a file is finished loading, so it won't be ignored.
Underneath all your code, write the following lines of ActionScript:
//creates a listener object to handle progress indication and //clears the progress indication when the video is fully loaded. var myLoadIt:Object=new Object(); myLoadIt.progress=function() { percentLoaded.autosize="left"; percentLoaded.text=Math.round(videoLoader.percentLoaded)+"% of the ¬ video has loaded"; } myLoadIt.complete=function() { percentLoaded.text=""; } videoLoader.addEventListener("progress",myLoadIt); videoLoader.addEventListener("complete",myLoadIt); Be proud of yourself! You're doing very well so far.
As always, you start off with a comment. Then you create a generic object called myLoadIt from the Object class. The Object class is THE class in Flash, because everything is based from it. It's kind of dumb, however, because it doesn't really have its own properties or methods to speak of, which is what makes it great for a listener object. Think of it as modeling clay: Once you have it, you can shape it into anything you want.
After you have the object built, you tell it to respond to anything that tells it a file load is in progress. When it knows that's what's happening, it looks at the percent of data loaded into the videoLoader component instance on the Stage, rounds the number, and then displays that number n the percentLoaded text field you create earlier. You use concatenation (remember, the ActionScript glue stick) to add an extra piece of text to the information displaying in the text field so the end user understands what's happening.
The last two lines use the addEventListener method of the Loader component to tell videoLoader to let your listener object know when it's loading or finished loading a file.
Whew! One last thing to do and then you can test it.
| 10. | Create event handlers for your buttons to load different SWF files containing embedded video.
Underneath all your current script, type the following lines of ActionScript:
//buttons cause video and text to display btnReviews.onRelease=function() { loadVideo("video1.swf","productsAndReviews"); } btnCompany.onRelease=function() { loadVideo("video2.swf","companyAndNews"); } btnContact.onRelease=function() { loadVideo("video3.swf","contactAndMap"); } You already created event handlers for buttons. What's different with these event handlers is that you are calling your loadVideo function, and passing to the function the path to the video and the text to display from the LoadVars object you created. Also, you're loading each SWF file into a single Loader component instance, replacing current content with new.
Note If the Loader component is already loading content when a new button is selected, the current download will be stopped in favor of the new download. | 11. | Save and test your file. Simulate a download to see the progress indication.
Save tour.fla and select Control > Test Movie. If the Bandwidth Profiler is not turned on, select View > Bandwidth Profiler in the Test Movie Environment.
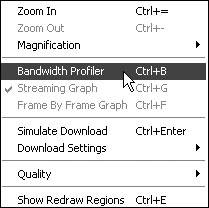 When you test a movie and it loads information in from the hard drive, it appears as if the content is loading quickly, but this gives you a false sense of security. To simulate a download, select View Download Settings, and then choose an option. Then select View > Simulate Download. Your progress indicator should show the percentages of each SWF file loaded when you click a video, and the download begins. When the download is finished, the text field displaying the percentage loaded is purged.
| 12. | Save and close tour.fla.
In the next set of exercises, you will be loading content into the main Tech Bookstore website. You'll be using techniques similar to what you just learned, with a few slight modifications.
| |