To illustrate deadlocking, Edsger Dijkstra introduced a "Dining Philosopher" metaphor that has become a classical way of introducing resource allocation and deadlocking. The metaphor (with variations) goes like this: Five philosophers, who spend their lives alternately thinking and eating, are sitting around a table. In the center of the round table is an infinite supply of food. Before each philosopher is a plate, and between each pair of plates is a single chopstick. Once a philosopher quits thinking, he or she attempts to eat. In order to eat, a philosopher must have possession of the chopstick to the left and right of the plate. 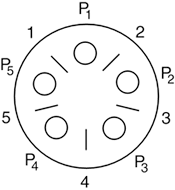 Your challenge is to design a program that creates five threads one to represent each philosopher that continually perform the tasks of thinking and eating. The program should implement a synchronization scheme that allows each thread to periodically acquire two chopsticks so that it can eat for a fixed or random (your choice) amount of time. After eating, a philosopher should release its chopsticks and think for a while before trying to eat again. Hint: Use Monitor.Wait() to try to acquire chopsticks, and Monitor.PulseAll() to notify all threads that chopsticks are being released. |